rubio-radio 0.0.1
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +7 -0
- data/LICENSE.txt +21 -0
- data/README.md +27 -0
- data/exe/rubio +30 -0
- data/lib/rubio/player.rb +57 -0
- data/lib/rubio/radio.rb +108 -0
- data/lib/rubio/radio_browser.rb +26 -0
- data/lib/rubio/station.rb +18 -0
- data/lib/rubio/version.rb +5 -0
- data/lib/rubio.rb +12 -0
- metadata +67 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: 76a2b9f65b8a23dc9b85e85c94815701339e14e3f7fda6d15fd49e2668e5efae
|
4
|
+
data.tar.gz: fa6cb61dda6e988098619c91c4bb32e0d764aaff9cd1b907cbe2207391ca3ced
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: bc3eaa0c4894f9924d7acfa63e8d877b51c93096996f17351927dcd03b4c8cf2c5b0d02a014d8c8655df8b734be6bda824dd07c27b36766b3459e82b4e861bc9
|
7
|
+
data.tar.gz: 2b044184d2978cbe1686c78a5453b01fc66530df583703bf20a390b86d4d35a5676749564cc3436a068e328f90239aef459d0c86e1362a626aa08561faf9c8c9
|
data/LICENSE.txt
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
The MIT License (MIT)
|
2
|
+
|
3
|
+
Copyright (c) 2020 kojix2
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in
|
13
|
+
all copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
21
|
+
THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,27 @@
|
|
1
|
+
# Rubio
|
2
|
+
|
3
|
+
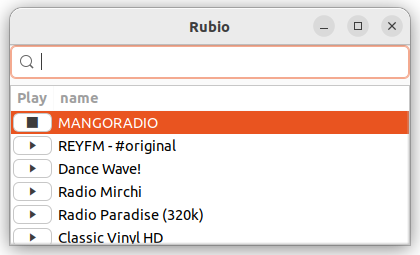
|
4
|
+
|
5
|
+
:bowtie: Alpha
|
6
|
+
## installation
|
7
|
+
|
8
|
+
Requirements : [cvlc](https://github.com/videolan/vlc)
|
9
|
+
|
10
|
+
```
|
11
|
+
gem install rubio-radio
|
12
|
+
```
|
13
|
+
## Usage
|
14
|
+
|
15
|
+
```
|
16
|
+
rubio
|
17
|
+
```
|
18
|
+
|
19
|
+
Default player is `cvlc`. But you can use any command line player that can take URL of radio station as its first argument.
|
20
|
+
|
21
|
+
```
|
22
|
+
rubio --backend mpg123
|
23
|
+
```
|
24
|
+
|
25
|
+
## LICENSE
|
26
|
+
|
27
|
+
MIT
|
data/exe/rubio
ADDED
@@ -0,0 +1,30 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
# frozen_string_literal: true
|
3
|
+
|
4
|
+
require 'rubio'
|
5
|
+
|
6
|
+
require 'optparse'
|
7
|
+
backend = 'cvlc'
|
8
|
+
debug = false
|
9
|
+
opt = OptionParser.new
|
10
|
+
opt.on('--vlc') { backend = 'cvlc' }
|
11
|
+
opt.on('--mpg123') { backend = 'mpg123' }
|
12
|
+
opt.on('--backend CMD') { |b| backend = b }
|
13
|
+
opt.on('--debug') { debug = true }
|
14
|
+
opt.on('--help') do
|
15
|
+
puts opt
|
16
|
+
exit
|
17
|
+
end
|
18
|
+
opt.on('--version') do
|
19
|
+
puts Rubio::VERSION
|
20
|
+
exit
|
21
|
+
end
|
22
|
+
opt.parse!(ARGV)
|
23
|
+
|
24
|
+
radio = Rubio::Radio.new(backend, debug: debug)
|
25
|
+
begin
|
26
|
+
radio.launch
|
27
|
+
rescue StandardError => e
|
28
|
+
radio&.player&.stop_all
|
29
|
+
raise e
|
30
|
+
end
|
data/lib/rubio/player.rb
ADDED
@@ -0,0 +1,57 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Rubio
|
4
|
+
class Player
|
5
|
+
attr_accessor :backend, :pid, :thr, :status, :history
|
6
|
+
|
7
|
+
def initialize(backend = 'cvlc')
|
8
|
+
raise unless backend.is_a?(String)
|
9
|
+
|
10
|
+
@backend = backend
|
11
|
+
@pid = nil
|
12
|
+
@thr = nil
|
13
|
+
@status = []
|
14
|
+
@history = []
|
15
|
+
end
|
16
|
+
|
17
|
+
def alive?
|
18
|
+
return false if @thr.nil?
|
19
|
+
|
20
|
+
@thr.alive?
|
21
|
+
end
|
22
|
+
|
23
|
+
def stop?
|
24
|
+
@thr.nil? || @thr.stop?
|
25
|
+
end
|
26
|
+
|
27
|
+
def play(url)
|
28
|
+
# do not include spaces in the command line
|
29
|
+
# if a space exist :
|
30
|
+
# * sh -c command url # this process with @pid will be killed
|
31
|
+
# * cmmand url # will not be killd because pid is differennt
|
32
|
+
# if no space : command url
|
33
|
+
# * cmmand url # will be killed by @pid
|
34
|
+
raise if url.match(/\s/)
|
35
|
+
|
36
|
+
@pid = spawn(*backend.split(' '), url)
|
37
|
+
@thr = Process.detach(@pid)
|
38
|
+
@status = [@pid, @thr]
|
39
|
+
@history << @status
|
40
|
+
end
|
41
|
+
|
42
|
+
def stop
|
43
|
+
return unless alive?
|
44
|
+
|
45
|
+
r = Process.kill(:TERM, pid)
|
46
|
+
@thr = nil
|
47
|
+
@pid = nil
|
48
|
+
r
|
49
|
+
end
|
50
|
+
|
51
|
+
def stop_all
|
52
|
+
@history.each do |pid, thr|
|
53
|
+
Process.kill(:TERM, pid) if thr.alive?
|
54
|
+
end
|
55
|
+
end
|
56
|
+
end
|
57
|
+
end
|
data/lib/rubio/radio.rb
ADDED
@@ -0,0 +1,108 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
require 'glimmer-dsl-libui'
|
4
|
+
|
5
|
+
module Rubio
|
6
|
+
class Radio
|
7
|
+
include Glimmer
|
8
|
+
|
9
|
+
attr_reader :stations, :player
|
10
|
+
|
11
|
+
def initialize(backend, debug: false)
|
12
|
+
@stations_all, @table = RadioBrowser.topvote(1000)
|
13
|
+
@stations = @stations_all.dup
|
14
|
+
@station_uuid = nil
|
15
|
+
@player = Player.new(backend)
|
16
|
+
|
17
|
+
monitor_thread(debug)
|
18
|
+
end
|
19
|
+
|
20
|
+
def monitor_thread(debug)
|
21
|
+
Glimmer::LibUI.timer(1) do
|
22
|
+
p @player.history if debug
|
23
|
+
next if @station_uuid.nil? || @player.alive?
|
24
|
+
|
25
|
+
message_box("player '#{@player.backend}' stopped!", @player.thr.to_s)
|
26
|
+
stop_uuid(@station_uuid)
|
27
|
+
@station_uuid = nil
|
28
|
+
true
|
29
|
+
end
|
30
|
+
end
|
31
|
+
|
32
|
+
def selected_station_at(idx)
|
33
|
+
raise unless idx.is_a?(Integer)
|
34
|
+
|
35
|
+
station_uuid = stations[idx].stationuuid
|
36
|
+
stop_uuid(@station_uuid)
|
37
|
+
if @station_uuid == station_uuid
|
38
|
+
@station_uuid = nil
|
39
|
+
else
|
40
|
+
play_uuid(station_uuid)
|
41
|
+
@station_uuid = station_uuid
|
42
|
+
end
|
43
|
+
end
|
44
|
+
|
45
|
+
def play_uuid(station_uuid)
|
46
|
+
station = uuid_to_station(station_uuid)
|
47
|
+
begin
|
48
|
+
@player.play(station.url)
|
49
|
+
rescue StandardError => e
|
50
|
+
message_box(e.message)
|
51
|
+
raise e
|
52
|
+
end
|
53
|
+
station.playing = true
|
54
|
+
end
|
55
|
+
|
56
|
+
def stop_uuid(station_uuid)
|
57
|
+
return if station_uuid.nil?
|
58
|
+
|
59
|
+
station = uuid_to_station(station_uuid)
|
60
|
+
@player.stop
|
61
|
+
station.playing = false
|
62
|
+
end
|
63
|
+
|
64
|
+
def launch
|
65
|
+
window('Rubio', 400, 200) do
|
66
|
+
vertical_box do
|
67
|
+
horizontal_box do
|
68
|
+
stretchy false
|
69
|
+
search_entry do |se|
|
70
|
+
on_changed do
|
71
|
+
filter_value = se.text
|
72
|
+
if filter_value.empty?
|
73
|
+
@stations.replace @stations_all.dup
|
74
|
+
else
|
75
|
+
@stations.replace(@stations_all.filter do |row_data|
|
76
|
+
row_data.name.downcase.include?(filter_value.downcase)
|
77
|
+
end)
|
78
|
+
end
|
79
|
+
end
|
80
|
+
end
|
81
|
+
end
|
82
|
+
horizontal_box do
|
83
|
+
table do
|
84
|
+
button_column('Play') do
|
85
|
+
on_clicked do |row|
|
86
|
+
selected_station_at(row)
|
87
|
+
end
|
88
|
+
end
|
89
|
+
text_column('name')
|
90
|
+
text_column('language')
|
91
|
+
cell_rows <= [self, :stations]
|
92
|
+
end
|
93
|
+
end
|
94
|
+
end
|
95
|
+
on_closing do
|
96
|
+
@player.stop_all
|
97
|
+
end
|
98
|
+
end.show
|
99
|
+
end
|
100
|
+
|
101
|
+
private
|
102
|
+
|
103
|
+
def uuid_to_station(uuid)
|
104
|
+
idx = @table[uuid]
|
105
|
+
@stations_all[idx]
|
106
|
+
end
|
107
|
+
end
|
108
|
+
end
|
@@ -0,0 +1,26 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
require 'json'
|
4
|
+
require 'open-uri'
|
5
|
+
|
6
|
+
module Rubio
|
7
|
+
# https://www.radio-browser.info
|
8
|
+
module RadioBrowser
|
9
|
+
module_function
|
10
|
+
|
11
|
+
def base_url
|
12
|
+
'http://all.api.radio-browser.info/json/'
|
13
|
+
end
|
14
|
+
|
15
|
+
def topvote(n = 100)
|
16
|
+
content = URI.parse(base_url + "stations/topvote/#{n}")
|
17
|
+
table = {} # uuid => index
|
18
|
+
result = []
|
19
|
+
JSON[content.read].each_with_index do |s, i|
|
20
|
+
table[s['stationuuid']] = i
|
21
|
+
result << Station.new(s['stationuuid'], s['name'], s['language'], s['url_resolved'])
|
22
|
+
end
|
23
|
+
[result, table]
|
24
|
+
end
|
25
|
+
end
|
26
|
+
end
|
@@ -0,0 +1,18 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Rubio
|
4
|
+
BaseStation = Struct.new(:stationuuid, :name, :language, :url)
|
5
|
+
|
6
|
+
class Station < BaseStation
|
7
|
+
attr_accessor :playing
|
8
|
+
|
9
|
+
def initialize(*args, **kwargs)
|
10
|
+
super(*args, **kwargs)
|
11
|
+
@playing = false
|
12
|
+
end
|
13
|
+
|
14
|
+
def play
|
15
|
+
@playing ? '■' : '▶'
|
16
|
+
end
|
17
|
+
end
|
18
|
+
end
|
data/lib/rubio.rb
ADDED
@@ -0,0 +1,12 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
require 'glimmer-dsl-libui'
|
4
|
+
|
5
|
+
require_relative 'rubio/version'
|
6
|
+
require_relative 'rubio/station'
|
7
|
+
require_relative 'rubio/radio_browser'
|
8
|
+
require_relative 'rubio/player'
|
9
|
+
require_relative 'rubio/radio'
|
10
|
+
|
11
|
+
module Rubio
|
12
|
+
end
|
metadata
ADDED
@@ -0,0 +1,67 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: rubio-radio
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.1
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- kojix2
|
8
|
+
autorequire:
|
9
|
+
bindir: exe
|
10
|
+
cert_chain: []
|
11
|
+
date: 2022-06-04 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: glimmer-dsl-libui
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - ">="
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '0'
|
20
|
+
type: :runtime
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - ">="
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '0'
|
27
|
+
description: Rubio is a simple radio player written in Ruby.
|
28
|
+
email:
|
29
|
+
- 2xijok@gmail.com
|
30
|
+
executables:
|
31
|
+
- rubio
|
32
|
+
extensions: []
|
33
|
+
extra_rdoc_files: []
|
34
|
+
files:
|
35
|
+
- LICENSE.txt
|
36
|
+
- README.md
|
37
|
+
- exe/rubio
|
38
|
+
- lib/rubio.rb
|
39
|
+
- lib/rubio/player.rb
|
40
|
+
- lib/rubio/radio.rb
|
41
|
+
- lib/rubio/radio_browser.rb
|
42
|
+
- lib/rubio/station.rb
|
43
|
+
- lib/rubio/version.rb
|
44
|
+
homepage: https://github.com/kojix2/rubio
|
45
|
+
licenses:
|
46
|
+
- MIT
|
47
|
+
metadata: {}
|
48
|
+
post_install_message:
|
49
|
+
rdoc_options: []
|
50
|
+
require_paths:
|
51
|
+
- lib
|
52
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
53
|
+
requirements:
|
54
|
+
- - ">="
|
55
|
+
- !ruby/object:Gem::Version
|
56
|
+
version: '0'
|
57
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
58
|
+
requirements:
|
59
|
+
- - ">="
|
60
|
+
- !ruby/object:Gem::Version
|
61
|
+
version: '0'
|
62
|
+
requirements: []
|
63
|
+
rubygems_version: 3.3.7
|
64
|
+
signing_key:
|
65
|
+
specification_version: 4
|
66
|
+
summary: Rubio - A simple radio player
|
67
|
+
test_files: []
|