rubiks_cube 1.1.0 → 1.2.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/Gemfile.lock +1 -1
- data/README.md +43 -23
- data/lib/rubiks_cube.rb +1 -0
- data/lib/rubiks_cube/cube.rb +6 -0
- data/lib/rubiks_cube/sticker_state_transform.rb +41 -0
- data/lib/rubiks_cube/version.rb +1 -1
- data/spec/rubiks_cube/cube_spec.rb +6 -0
- data/spec/rubiks_cube/sticker_state_transform_spec.rb +24 -0
- metadata +5 -3
- data/img/cube_instructions.jpg +0 -0
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: ea7804b38177c1c4c7075d2e4cd1db3c4de4d511
|
4
|
+
data.tar.gz: eb603bea15bfc45f9eee766563170d3c867838bb
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 7c12b08b5ffb2bf6394799ed19a5092e596ec985c59f8a14530f021bc8fd505a16d25cb8c20443538f7b69c201d203231e08e4030a27acedb05fbc8c727d3fbc
|
7
|
+
data.tar.gz: c0e10419fe026a25b542ae3bfde7be090882114bbd20e19dd974ebebfabe0c5147dcc1a7ea234eeef09bfb76fc835abf600858d753bbcef180e6a84c0c8d5b6e
|
data/Gemfile.lock
CHANGED
data/README.md
CHANGED
@@ -81,19 +81,20 @@ If you have a scrambled Rubik's Cube sitting in front of you right now, you
|
|
81
81
|
may not know how to get it back to the solved position. That's OK! We can set
|
82
82
|
the Rubik's Cube state manually and learn how to solve it.
|
83
83
|
|
84
|
-
|
85
|
-
|
84
|
+
Starting with the **F** face, enter the color of each sticker from top-left to
|
85
|
+
bottom-right. You may use any characters you like to describe the color of your
|
86
|
+
cube, but make sure all six colors are unique. Whitespace is not important.
|
86
87
|
|
87
|
-
|
88
|
-
not move when you rotate a side. The is the basis for entering cube state
|
89
|
-
manually. If the center of a face is red, then that face will be red when the
|
90
|
-
cube is solved.
|
88
|
+
The cube state must be entered in the following order:
|
91
89
|
|
92
|
-
|
93
|
-
|
94
|
-
|
90
|
+
1. **F**: Front Face
|
91
|
+
1. **R**: Right Face
|
92
|
+
1. **B**: Back Face
|
93
|
+
1. **L**: Left Face
|
94
|
+
1. **U**: Up Face (top)
|
95
|
+
1. **D**: Down Face (bottom)
|
95
96
|
|
96
|
-
|
97
|
+
See the [examples](#examples) below for more help.
|
97
98
|
|
98
99
|
### Examples
|
99
100
|
|
@@ -111,8 +112,14 @@ require 'rubiks_cube'
|
|
111
112
|
|
112
113
|
cube = RubiksCube::Cube.new
|
113
114
|
|
114
|
-
cube.state
|
115
|
-
|
115
|
+
cube.state = <<-STATE
|
116
|
+
G G G G G G G G G
|
117
|
+
Y Y Y Y Y Y Y Y Y
|
118
|
+
B B B B B B B B B
|
119
|
+
W W W W W W W W W
|
120
|
+
R R R R R R R R R
|
121
|
+
O O O O O O O O O
|
122
|
+
STATE
|
116
123
|
|
117
124
|
cube.solved? #=> true
|
118
125
|
```
|
@@ -121,15 +128,21 @@ cube.solved? #=> true
|
|
121
128
|
|
122
129
|
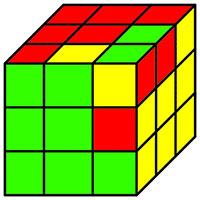
|
123
130
|
|
124
|
-
|
125
|
-
this state if we like:
|
131
|
+
Our cube is now slightly scrambled.
|
126
132
|
|
127
133
|
```ruby
|
128
134
|
require 'rubiks_cube'
|
129
135
|
|
130
|
-
cube = RubiksCube::Cube.new
|
131
|
-
|
132
|
-
|
136
|
+
cube = RubiksCube::Cube.new
|
137
|
+
|
138
|
+
cube.state = <<-STATE
|
139
|
+
G G Y G G R G G G
|
140
|
+
R R Y Y Y Y Y Y Y
|
141
|
+
B B B B B B B B W
|
142
|
+
W W W W W W O W W
|
143
|
+
R R R R R G R Y G
|
144
|
+
O O O O O O B O O
|
145
|
+
STATE
|
133
146
|
|
134
147
|
cube.solved? #=> false
|
135
148
|
```
|
@@ -138,8 +151,8 @@ cube.solved? #=> false
|
|
138
151
|
|
139
152
|
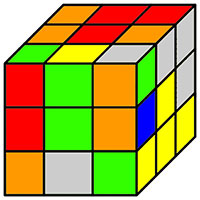
|
140
153
|
|
141
|
-
|
142
|
-
|
154
|
+
Here's a fully scrambled cube. This looks hard to solve. We can start our cube
|
155
|
+
in this state if we like and have the solver tell us a solution.
|
143
156
|
|
144
157
|
Here's the scramble we used to mix the cube if you'd like to try it yourself:
|
145
158
|
|
@@ -150,9 +163,16 @@ U D B2 U B D2 B2 F' R' U2 F U' L2 F L2 B2 L2 R' U R' U' D R2 F2 B2
|
|
150
163
|
```ruby
|
151
164
|
require 'rubiks_cube'
|
152
165
|
|
153
|
-
cube = RubiksCube::Cube.new
|
154
|
-
|
155
|
-
|
166
|
+
cube = RubiksCube::Cube.new
|
167
|
+
|
168
|
+
cube.state = <<-STATE
|
169
|
+
R G O R G O O W G
|
170
|
+
G W W B Y W Y Y Y
|
171
|
+
G B B G B W R Y B
|
172
|
+
Y G Y B W G R Y W
|
173
|
+
O R R O R O G Y W
|
174
|
+
B R O R O O W B B
|
175
|
+
STATE
|
156
176
|
|
157
177
|
cube.solved? #=> false
|
158
178
|
```
|
@@ -273,7 +293,7 @@ at first. Each face is represented by a letter:
|
|
273
293
|
- **F**: Front Face
|
274
294
|
- **B**: Back Face
|
275
295
|
- **U**: Up Face (top)
|
276
|
-
- **D**:
|
296
|
+
- **D**: Down Face (bottom)
|
277
297
|
|
278
298
|
When we see a letter in an algorithm, then we turn that face 90 degrees
|
279
299
|
clockwise. To determine which direction is clockwise, rotate the cube so that
|
data/lib/rubiks_cube.rb
CHANGED
data/lib/rubiks_cube/cube.rb
CHANGED
@@ -0,0 +1,41 @@
|
|
1
|
+
module RubiksCube
|
2
|
+
# Transform manual sticker state entry to cube state
|
3
|
+
class StickerStateTransform
|
4
|
+
attr_reader :state
|
5
|
+
|
6
|
+
CENTER_LOCATIONS = [ 4, 13, 22, 31, 40, 49 ]
|
7
|
+
|
8
|
+
CORNER_LOCATIONS = [
|
9
|
+
42, 0 , 29, 44, 9, 2 , 38, 18, 11, 36, 27, 20,
|
10
|
+
45, 35, 6 , 47, 8, 15, 53, 17, 24, 51, 26, 33
|
11
|
+
]
|
12
|
+
|
13
|
+
EDGE_LOCATIONS = [
|
14
|
+
43, 1 , 41, 10, 37, 19, 39, 28,
|
15
|
+
3 , 32, 5 , 12, 21, 14, 23, 30,
|
16
|
+
46, 7 , 50, 16, 52, 25, 48, 34
|
17
|
+
]
|
18
|
+
|
19
|
+
def initialize(state)
|
20
|
+
@state = state.gsub(/\s/, '').split('')
|
21
|
+
end
|
22
|
+
|
23
|
+
def to_cube
|
24
|
+
(edges + corners).join(' ').gsub(/./) { |c| color_mapping.fetch(c, c) }
|
25
|
+
end
|
26
|
+
|
27
|
+
private
|
28
|
+
|
29
|
+
def color_mapping
|
30
|
+
Hash[state.values_at(*CENTER_LOCATIONS).zip %w(F R B L U D)]
|
31
|
+
end
|
32
|
+
|
33
|
+
def edges
|
34
|
+
state.values_at(*EDGE_LOCATIONS).each_slice(2).map(&:join)
|
35
|
+
end
|
36
|
+
|
37
|
+
def corners
|
38
|
+
state.values_at(*CORNER_LOCATIONS).each_slice(3).map(&:join)
|
39
|
+
end
|
40
|
+
end
|
41
|
+
end
|
data/lib/rubiks_cube/version.rb
CHANGED
@@ -23,6 +23,12 @@ describe RubiksCube::Cube do
|
|
23
23
|
end
|
24
24
|
end
|
25
25
|
|
26
|
+
describe '#state=' do
|
27
|
+
it 'changes the state of the cube' do
|
28
|
+
expect { subject.state = 'a b c' }.to change { subject.state }
|
29
|
+
end
|
30
|
+
end
|
31
|
+
|
26
32
|
describe '==' do
|
27
33
|
it 'returns true when two cubes have the same state' do
|
28
34
|
expect(subject).to eq described_class.new(state)
|
@@ -0,0 +1,24 @@
|
|
1
|
+
require 'spec_helper'
|
2
|
+
|
3
|
+
describe RubiksCube::StickerStateTransform do
|
4
|
+
subject { described_class.new state }
|
5
|
+
|
6
|
+
let(:state) { nil }
|
7
|
+
|
8
|
+
describe '#to_cube' do
|
9
|
+
context 'when initialized with sticker state' do
|
10
|
+
let(:state) {[
|
11
|
+
"g b b b w b o g b \
|
12
|
+
|
13
|
+
o w b o o g r r w r y y o y r r g o r w o y r w",
|
14
|
+
"gw bg o yggowywyywrbbyrg "
|
15
|
+
].join(' ')}
|
16
|
+
|
17
|
+
it 'transforms to cube state' do
|
18
|
+
expect(subject.to_cube).to eq(
|
19
|
+
"BD RF RB UF DF DR RU LB BU DL LU LF FUR FRD BLD ULB BDR FDL UFL BRU"
|
20
|
+
)
|
21
|
+
end
|
22
|
+
end
|
23
|
+
end
|
24
|
+
end
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: rubiks_cube
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 1.
|
4
|
+
version: 1.2.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Chris Hunt
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2013-09-
|
11
|
+
date: 2013-09-23 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: bundler
|
@@ -97,7 +97,6 @@ files:
|
|
97
97
|
- Rakefile
|
98
98
|
- img/cube_algorithm.jpg
|
99
99
|
- img/cube_blank.jpg
|
100
|
-
- img/cube_instructions.jpg
|
101
100
|
- img/cube_m_slice.jpg
|
102
101
|
- img/cube_orientation.jpg
|
103
102
|
- img/cube_permutation.jpg
|
@@ -110,6 +109,7 @@ files:
|
|
110
109
|
- lib/rubiks_cube/cube.rb
|
111
110
|
- lib/rubiks_cube/cubie.rb
|
112
111
|
- lib/rubiks_cube/solution.rb
|
112
|
+
- lib/rubiks_cube/sticker_state_transform.rb
|
113
113
|
- lib/rubiks_cube/two_cycle_solution.rb
|
114
114
|
- lib/rubiks_cube/version.rb
|
115
115
|
- rubiks_cube.gemspec
|
@@ -117,6 +117,7 @@ files:
|
|
117
117
|
- spec/rubiks_cube/cube_spec.rb
|
118
118
|
- spec/rubiks_cube/cubie_spec.rb
|
119
119
|
- spec/rubiks_cube/solution_spec.rb
|
120
|
+
- spec/rubiks_cube/sticker_state_transform_spec.rb
|
120
121
|
- spec/rubiks_cube/two_cycle_solution_spec.rb
|
121
122
|
- spec/spec_helper.rb
|
122
123
|
homepage: https://github.com/chrishunt/rubiks-cube
|
@@ -148,5 +149,6 @@ test_files:
|
|
148
149
|
- spec/rubiks_cube/cube_spec.rb
|
149
150
|
- spec/rubiks_cube/cubie_spec.rb
|
150
151
|
- spec/rubiks_cube/solution_spec.rb
|
152
|
+
- spec/rubiks_cube/sticker_state_transform_spec.rb
|
151
153
|
- spec/rubiks_cube/two_cycle_solution_spec.rb
|
152
154
|
- spec/spec_helper.rb
|
data/img/cube_instructions.jpg
DELETED
Binary file
|