rbtree-jruby 0.1.0 → 0.2.0
Sign up to get free protection for your applications and to get access to all the features.
- data/Gemfile.lock +1 -1
- data/README.md +11 -0
- data/VERSION +1 -1
- data/benchmark/bm.rb +26 -0
- data/benchmark/bm1.rb +30 -0
- data/benchmark/result.png +0 -0
- data/benchmark/result.txt +114 -0
- data/java/src/rbtree/ext/MultiRBTree.java +1170 -1080
- data/lib/rbtree/ext/multi_r_b_tree.jar +0 -0
- metadata +6 -8
- data/java/src/rbtree/ext/Color.java +0 -6
- data/java/src/rbtree/ext/NilNode.java +0 -24
- data/java/src/rbtree/ext/Node.java +0 -98
- data/lib/rbtree/ext/multi_red_black_tree.jar +0 -0
- data/lib/rbtree/ext/rbtree.jar +0 -0
- data/lib/rbtree/ext/red_black_tree.jar +0 -0
data/Gemfile.lock
CHANGED
data/README.md
CHANGED
@@ -55,6 +55,17 @@ duplications of keys but MultiRBTree does.
|
|
55
55
|
end # => ["a", 20] ["a", 40] ["c", 10]
|
56
56
|
```
|
57
57
|
|
58
|
+
Benchmarks
|
59
|
+
-----------
|
60
|
+
|
61
|
+
Benchmark takes from headius' [redblack](https://github.com/headius/redblack) project.
|
62
|
+
Compared to [rbtree-pure](https://github.com/pwnall/rbtree-pure) and
|
63
|
+
[rbtree c extension](https://github.com/skade/rbtree).
|
64
|
+
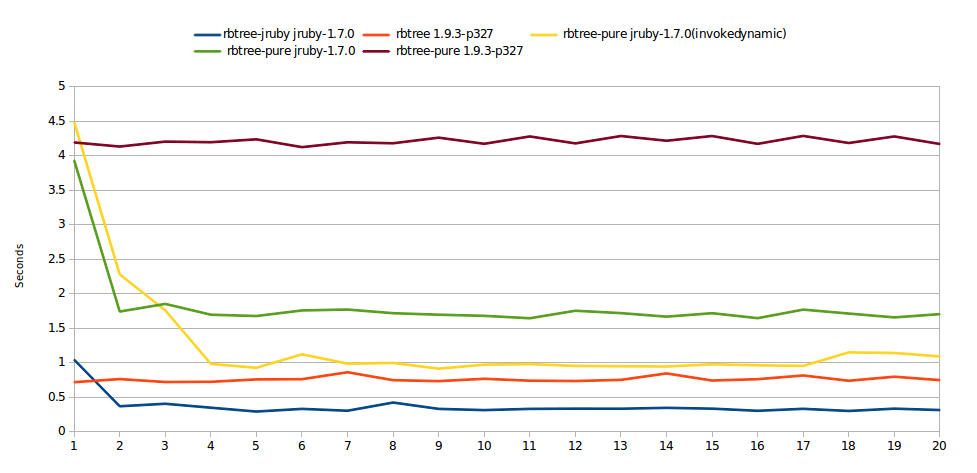
|
65
|
+
|
66
|
+
It's interesting to see jruby with invokedynamic almost gets C extension speed, and
|
67
|
+
jruby native extension is even faster than it's counterpart.
|
68
|
+
For more details, checkout [benchmark directory](https://github.com/isaiah/rbtree-jruby/tree/master/benchmark).
|
58
69
|
|
59
70
|
Requirement
|
60
71
|
-----------
|
data/VERSION
CHANGED
@@ -1 +1 @@
|
|
1
|
-
0.
|
1
|
+
0.2.0
|
data/benchmark/bm.rb
ADDED
@@ -0,0 +1,26 @@
|
|
1
|
+
#$:.unshift(File.join(File.dirname(__FILE__), "..", "lib"))
|
2
|
+
require 'rbtree'
|
3
|
+
require "benchmark"
|
4
|
+
|
5
|
+
def rbt_bm
|
6
|
+
n = 1_000
|
7
|
+
a1 = []; n.times { a1 << rand(999_999) }
|
8
|
+
a2 = []; n.times { a2 << rand(999_999) }
|
9
|
+
|
10
|
+
start = Time.now
|
11
|
+
tree = RBTree.new
|
12
|
+
a1.each {|e| tree[e] = e }
|
13
|
+
a2.each {|e| tree[e] }
|
14
|
+
n.times do
|
15
|
+
tree.delete_if{|k, v| v == rand(999_999)}
|
16
|
+
end
|
17
|
+
|
18
|
+
return Time.now - start
|
19
|
+
end
|
20
|
+
|
21
|
+
N = (ARGV[0] || 5).to_i
|
22
|
+
|
23
|
+
N.times do
|
24
|
+
puts rbt_bm.to_f
|
25
|
+
puts "GC.count = #{GC.count}" if GC.respond_to?(:count)
|
26
|
+
end
|
data/benchmark/bm1.rb
ADDED
@@ -0,0 +1,30 @@
|
|
1
|
+
#$:.unshift(File.join(File.dirname(__FILE__), "..", "lib"))
|
2
|
+
require 'rbtree'
|
3
|
+
require "benchmark"
|
4
|
+
|
5
|
+
def rbt_bm
|
6
|
+
n = 100_000
|
7
|
+
a1 = []; n.times { a1 << rand(999_999) }
|
8
|
+
a2 = []; n.times { a2 << rand(999_999) }
|
9
|
+
|
10
|
+
start = Time.now
|
11
|
+
|
12
|
+
tree = RBTree.new
|
13
|
+
|
14
|
+
n.times {|i| tree[i] = i }
|
15
|
+
n.times {|i| tree.delete(i) }
|
16
|
+
|
17
|
+
tree = RBTree.new
|
18
|
+
a1.each {|e| tree[e] = e }
|
19
|
+
a2.each {|e| tree[e] }
|
20
|
+
tree.each {|key, value| value + 1 }
|
21
|
+
tree.reverse_each {|key, value| value + 1 }
|
22
|
+
return Time.now - start
|
23
|
+
end
|
24
|
+
|
25
|
+
N = (ARGV[0] || 5).to_i
|
26
|
+
|
27
|
+
N.times do
|
28
|
+
puts rbt_bm.to_f
|
29
|
+
#puts "GC.count = #{GC.count}" if GC.respond_to?(:count)
|
30
|
+
end
|
Binary file
|
@@ -0,0 +1,114 @@
|
|
1
|
+
$ ruby -v && ruby bm1.rb 20
|
2
|
+
ruby 1.9.3p327 (2012-11-10 revision 37606) [x86_64-linux]
|
3
|
+
4.194673558
|
4
|
+
4.134828053
|
5
|
+
4.206916244
|
6
|
+
4.1981704
|
7
|
+
4.239772576
|
8
|
+
4.126600891
|
9
|
+
4.197231759
|
10
|
+
4.182520993
|
11
|
+
4.264266545
|
12
|
+
4.175277231
|
13
|
+
4.281924272
|
14
|
+
4.180804353
|
15
|
+
4.288204194
|
16
|
+
4.219386288
|
17
|
+
4.288127756
|
18
|
+
4.174234309
|
19
|
+
4.289359396
|
20
|
+
4.186658554
|
21
|
+
4.281877193
|
22
|
+
4.17202375
|
23
|
+
|
24
|
+
$ ruby -v && ruby --server bm1.rb 20
|
25
|
+
jruby 1.7.0 (1.9.3p203) 2012-10-22 ff1ebbe on Java HotSpot(TM) 64-Bit Server VM 1.7.0_09-b05 [linux-amd64]
|
26
|
+
3.936
|
27
|
+
1.743
|
28
|
+
1.854
|
29
|
+
1.697
|
30
|
+
1.678
|
31
|
+
1.759
|
32
|
+
1.772
|
33
|
+
1.719
|
34
|
+
1.697
|
35
|
+
1.681
|
36
|
+
1.646
|
37
|
+
1.755
|
38
|
+
1.72
|
39
|
+
1.669
|
40
|
+
1.719
|
41
|
+
1.647
|
42
|
+
1.771
|
43
|
+
1.714
|
44
|
+
1.658
|
45
|
+
1.706
|
46
|
+
|
47
|
+
$ ruby -v && ruby -Xcompile.invokedynamic=true bm1.rb 20
|
48
|
+
jruby 1.7.0 (1.9.3p203) 2012-10-22 ff1ebbe on Java HotSpot(TM) 64-Bit Server VM 1.7.0_09-b05 [linux-amd64]
|
49
|
+
4.489
|
50
|
+
2.282
|
51
|
+
1.76
|
52
|
+
0.984
|
53
|
+
0.928
|
54
|
+
1.123
|
55
|
+
0.988
|
56
|
+
0.998
|
57
|
+
0.915
|
58
|
+
0.973
|
59
|
+
0.981
|
60
|
+
0.955
|
61
|
+
0.95
|
62
|
+
0.946
|
63
|
+
0.979
|
64
|
+
0.964
|
65
|
+
0.955
|
66
|
+
1.151
|
67
|
+
1.142
|
68
|
+
1.092
|
69
|
+
|
70
|
+
$ ruby -v && ruby bm1.rb 20
|
71
|
+
ruby 1.9.3p327 (2012-11-10 revision 37606) [x86_64-linux]
|
72
|
+
0.719245967
|
73
|
+
0.764002064
|
74
|
+
0.721334569
|
75
|
+
0.724404531
|
76
|
+
0.759026423
|
77
|
+
0.762418384
|
78
|
+
0.862859985
|
79
|
+
0.7481005
|
80
|
+
0.733698733
|
81
|
+
0.768279468
|
82
|
+
0.740369856
|
83
|
+
0.735618093
|
84
|
+
0.752477701
|
85
|
+
0.845850977
|
86
|
+
0.742914898
|
87
|
+
0.762281986
|
88
|
+
0.816316566
|
89
|
+
0.739624534
|
90
|
+
0.79819584
|
91
|
+
0.749143339
|
92
|
+
|
93
|
+
$ ruby -v && ruby --server bm1.rb 20
|
94
|
+
jruby 1.7.0 (1.9.3p203) 2012-10-22 ff1ebbe on Java HotSpot(TM) 64-Bit Server VM 1.7.0_09-b05 [linux-amd64]
|
95
|
+
1.044
|
96
|
+
0.37
|
97
|
+
0.406
|
98
|
+
0.349
|
99
|
+
0.293
|
100
|
+
0.332
|
101
|
+
0.305
|
102
|
+
0.424
|
103
|
+
0.332
|
104
|
+
0.314
|
105
|
+
0.332
|
106
|
+
0.335
|
107
|
+
0.334
|
108
|
+
0.347
|
109
|
+
0.335
|
110
|
+
0.304
|
111
|
+
0.333
|
112
|
+
0.302
|
113
|
+
0.335
|
114
|
+
0.315
|