rails_sortable 0.0.3 → 0.0.4
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +77 -2
- data/app/controllers/sortable_controller.rb +1 -1
- data/app/models/rails_sortable/model.rb +62 -0
- data/lib/rails_sortable/version.rb +1 -1
- data/spec/controllers/sortable_controller_spec.rb +3 -3
- data/spec/dummy/app/models/item.rb +3 -0
- data/spec/dummy/log/test.log +1863 -0
- data/spec/helpers/{enumerable_spec.rb → sortable_helper_spec.rb} +0 -0
- data/spec/models/rails_sortable/model_spec.rb +46 -0
- data/spec/spec_helper.rb +1 -0
- metadata +35 -4
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: f17638e379aacbde21fa9143d10eae85a20ba780
|
4
|
+
data.tar.gz: e12425497f4000922f75408ab1612261d36471c0
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 0500c8372108a65c6bd9dd87968094262c0b6185a78d3c4ef0b726760efbcaecd55e0a69cf5c0e08ad5d2feb5d2cdf624ba50d2f1191da6c48782a63cb0d5b50
|
7
|
+
data.tar.gz: 4649c612052168c34c3041eed4e148fead36c750dcc22feeb393824b9b5f5e58526bf8d95c918f4011e80737deb0560e8c0b47a9f18154b86e59d4887f4d291a
|
data/README.md
CHANGED
@@ -1,3 +1,78 @@
|
|
1
|
-
|
1
|
+
# RailsSortable
|
2
2
|
|
3
|
-
|
3
|
+
RailsSortable is a simple Rails gem that allows you to create a listing view with drag & drop sorting.
|
4
|
+
|
5
|
+
see the video @YouTube
|
6
|
+
|
7
|
+
[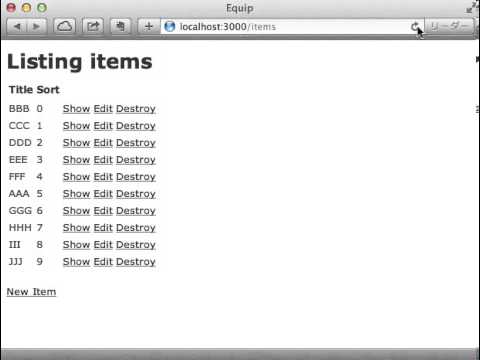](http://www.youtube.com/watch?v=MpT3hiNVmsc)
|
8
|
+
|
9
|
+
## Setup
|
10
|
+
|
11
|
+
Add it to your Gemfile then run bundle to install it.
|
12
|
+
```
|
13
|
+
gem 'jquery-ui-rails'
|
14
|
+
gem 'rails_sortable'
|
15
|
+
```
|
16
|
+
* rails_sortable depends on jquery-ui-rails (~> 4.0)
|
17
|
+
|
18
|
+
And then add it to the Asset Pipeline in the application.js file:
|
19
|
+
```
|
20
|
+
//= require jquery.ui.sortable
|
21
|
+
//= require rails_sortable
|
22
|
+
```
|
23
|
+
|
24
|
+
## Usage
|
25
|
+
|
26
|
+
RailsSortable requires specific column on ActiveRecord Model for own implementation.
|
27
|
+
|
28
|
+
For instance, the following migration indicates the case that you are attemtting to make Item model sortable.
|
29
|
+
|
30
|
+
```ruby
|
31
|
+
class CreateItems < ActiveRecord::Migration
|
32
|
+
def change
|
33
|
+
create_table :items do |t|
|
34
|
+
t.string :title
|
35
|
+
t.integer :sort # for RailsSortable
|
36
|
+
|
37
|
+
t.timestamps
|
38
|
+
end
|
39
|
+
end
|
40
|
+
end
|
41
|
+
```
|
42
|
+
and Item model is
|
43
|
+
```ruby
|
44
|
+
class Item < ActiveRecord::Base
|
45
|
+
include RailsSortable::Model
|
46
|
+
set_sortable :sort # indicate sort column
|
47
|
+
end
|
48
|
+
```
|
49
|
+
|
50
|
+
and its listing view (typically - index.html.erb) is
|
51
|
+
```erb
|
52
|
+
...
|
53
|
+
<tbody class="sortable"> <!-- sortable target -->
|
54
|
+
<% sortable_fetch(@items) do |item, id_tag| %> <!-- RailsSortable helper -->
|
55
|
+
<tr id="<%= id_tag %>"> <!-- you must write it -->
|
56
|
+
<td><%= item.title %></td>
|
57
|
+
<td><%= item.sort %></td>
|
58
|
+
<td><%= link_to 'Show', item %></td>
|
59
|
+
<td><%= link_to 'Edit', edit_item_path(item) %></td>
|
60
|
+
<td><%= link_to 'Destroy', item, method: :delete, data: { confirm: 'Are you sure?' } %></td>
|
61
|
+
</tr>
|
62
|
+
<% end %>
|
63
|
+
</tbody>
|
64
|
+
</table>
|
65
|
+
...
|
66
|
+
```
|
67
|
+
|
68
|
+
finally, you apply sortable with Javascript.
|
69
|
+
|
70
|
+
```coffeescript
|
71
|
+
jQuery ->
|
72
|
+
$(".sortable").railsSortable()
|
73
|
+
```
|
74
|
+
|
75
|
+
## Javascript options
|
76
|
+
jquery plugin `railsSortable` is just a wrapper `jquery.ui.sortable`. therefore it accepts all of `sortbale` options.
|
77
|
+
|
78
|
+
see the [http://api.jqueryui.com/sortable/](http://api.jqueryui.com/sortable/) to get detail.
|
@@ -7,7 +7,7 @@ class SortableController < ApplicationController
|
|
7
7
|
models = klass.order(:sort).to_a
|
8
8
|
ids.each_with_index do |id, new_sort|
|
9
9
|
model = models.find {|m| m.id == id }
|
10
|
-
model.
|
10
|
+
model.update_sort!(new_sort) if model.sort != new_sort
|
11
11
|
end
|
12
12
|
render nothing: true
|
13
13
|
end
|
@@ -0,0 +1,62 @@
|
|
1
|
+
module RailsSortable
|
2
|
+
|
3
|
+
#
|
4
|
+
# Include this module to your ActiveRecord model.
|
5
|
+
# And you must call `set_sortable` method for using sortable model.
|
6
|
+
#
|
7
|
+
# ex)
|
8
|
+
# class SampleModel < ActiveRecord::Base
|
9
|
+
# include RailsSortable::Model
|
10
|
+
# set_sortable :sort, silence_recording_timestamps: true
|
11
|
+
# end
|
12
|
+
#
|
13
|
+
module Model
|
14
|
+
def self.included(base)
|
15
|
+
base.class_eval do
|
16
|
+
before_create :maximize_sort
|
17
|
+
end
|
18
|
+
base.extend ClassMethods
|
19
|
+
end
|
20
|
+
|
21
|
+
def update_sort!(new_value)
|
22
|
+
write_attribute sort_attribute, new_value
|
23
|
+
if sortable_options[:silence_recording_timestamps]
|
24
|
+
silence_recording_timestamps { save! }
|
25
|
+
else
|
26
|
+
save!
|
27
|
+
end
|
28
|
+
end
|
29
|
+
|
30
|
+
protected
|
31
|
+
|
32
|
+
def maximize_sort
|
33
|
+
return if read_attribute(sort_attribute)
|
34
|
+
write_attribute sort_attribute, max_sort
|
35
|
+
end
|
36
|
+
|
37
|
+
def silence_recording_timestamps
|
38
|
+
raise ArgumentError unless block_given?
|
39
|
+
original_record_timestamps = self.class.record_timestamps
|
40
|
+
self.class.record_timestamps = false
|
41
|
+
yield
|
42
|
+
self.class.record_timestamps = original_record_timestamps
|
43
|
+
end
|
44
|
+
|
45
|
+
def max_sort
|
46
|
+
(self.class.maximum(sort_attribute) || 0) + 1
|
47
|
+
end
|
48
|
+
|
49
|
+
module ClassMethods
|
50
|
+
#
|
51
|
+
# allowed options
|
52
|
+
# - silence_recording_timestamps
|
53
|
+
# When it is true, timestamp(updated_at) will be NOT modified with reordering.
|
54
|
+
#
|
55
|
+
def set_sortable(attribute, options = {})
|
56
|
+
define_method("sort_attribute") { attribute }
|
57
|
+
define_method("sortable_options") { options }
|
58
|
+
end
|
59
|
+
end
|
60
|
+
end
|
61
|
+
|
62
|
+
end
|
@@ -3,9 +3,9 @@ require 'spec_helper'
|
|
3
3
|
describe SortableController do
|
4
4
|
describe "POST reorder" do
|
5
5
|
before do
|
6
|
-
@item1 = Item.create!
|
7
|
-
@item2 = Item.create!
|
8
|
-
@item3 = Item.create!
|
6
|
+
@item1 = Item.create!
|
7
|
+
@item2 = Item.create!
|
8
|
+
@item3 = Item.create!
|
9
9
|
end
|
10
10
|
it "should reorder models" do
|
11
11
|
post :reorder, Item: [@item1.id, @item3.id, @item2.id]
|
data/spec/dummy/log/test.log
CHANGED
@@ -357,3 +357,1866 @@ Completed 200 OK in 13ms (Views: 6.9ms | ActiveRecord: 0.9ms)
|
|
357
357
|
[1m[35m (0.1ms)[0m rollback transaction
|
358
358
|
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
359
359
|
[1m[35m (0.0ms)[0m rollback transaction
|
360
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
361
|
+
[1m[35m (0.1ms)[0m begin transaction
|
362
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
363
|
+
[1m[35m (0.1ms)[0m begin transaction
|
364
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
365
|
+
[1m[35m (0.1ms)[0m begin transaction
|
366
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
367
|
+
[1m[35mSQL (8.0ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00], ["sort", 0], ["updated_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00]]
|
368
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
369
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
370
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00]]
|
371
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
372
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
373
|
+
[1m[35mSQL (0.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00]]
|
374
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
375
|
+
Processing by SortableController#reorder as HTML
|
376
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
377
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" ORDER BY "items"."sort" ASC
|
378
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
379
|
+
[1m[35mSQL (0.2ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 3 [["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00]]
|
380
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
381
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
382
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 2[0m [["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:36:34 UTC +00:00]]
|
383
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
384
|
+
Completed 200 OK in 19ms (Views: 14.5ms | ActiveRecord: 0.8ms)
|
385
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 1]]
|
386
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 2]]
|
387
|
+
[1m[36mItem Load (0.0ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 3]]
|
388
|
+
[1m[35m (0.5ms)[0m rollback transaction
|
389
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
390
|
+
[1m[35m (0.1ms)[0m begin transaction
|
391
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
392
|
+
[1m[35m (0.1ms)[0m begin transaction
|
393
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
394
|
+
[1m[35m (0.1ms)[0m begin transaction
|
395
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
396
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
397
|
+
[1m[35m (0.1ms)[0m begin transaction
|
398
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
399
|
+
[1m[35m (0.1ms)[0m begin transaction
|
400
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
401
|
+
[1m[35m (0.1ms)[0m begin transaction
|
402
|
+
[1m[36m (0.0ms)[0m [1mrollback transaction[0m
|
403
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
404
|
+
[1m[35m (0.1ms)[0m begin transaction
|
405
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
406
|
+
[1m[35m (0.1ms)[0m begin transaction
|
407
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
408
|
+
[1m[35m (0.0ms)[0m begin transaction
|
409
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
410
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
411
|
+
[1m[35m (0.1ms)[0m begin transaction
|
412
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
413
|
+
[1m[35m (0.1ms)[0m begin transaction
|
414
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
415
|
+
[1m[35m (0.1ms)[0m begin transaction
|
416
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
417
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
418
|
+
[1m[35m (0.1ms)[0m begin transaction
|
419
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
420
|
+
[1m[35m (0.1ms)[0m begin transaction
|
421
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
422
|
+
[1m[35m (0.1ms)[0m begin transaction
|
423
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
424
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
425
|
+
[1m[35m (0.1ms)[0m begin transaction
|
426
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
427
|
+
[1m[35m (0.0ms)[0m begin transaction
|
428
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
429
|
+
[1m[35m (0.1ms)[0m begin transaction
|
430
|
+
[1m[36m (0.0ms)[0m [1mrollback transaction[0m
|
431
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
432
|
+
[1m[35m (0.1ms)[0m begin transaction
|
433
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
434
|
+
[1m[35m (0.0ms)[0m begin transaction
|
435
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
436
|
+
[1m[35m (0.0ms)[0m begin transaction
|
437
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
438
|
+
[1m[35mSQL (3.5ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00], ["sort", 0], ["updated_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00]]
|
439
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
440
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
441
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00]]
|
442
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
443
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
444
|
+
[1m[35mSQL (0.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00]]
|
445
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
446
|
+
Processing by SortableController#reorder as HTML
|
447
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
448
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" ORDER BY "items"."sort" ASC
|
449
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
450
|
+
[1m[35mSQL (0.2ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 3 [["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00]]
|
451
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
452
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
453
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 2[0m [["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:47:11 UTC +00:00]]
|
454
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
455
|
+
Completed 200 OK in 14ms (Views: 4.5ms | ActiveRecord: 0.8ms)
|
456
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 1]]
|
457
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 2]]
|
458
|
+
[1m[36mItem Load (0.0ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 3]]
|
459
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
460
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
461
|
+
[1m[35m (0.1ms)[0m begin transaction
|
462
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
463
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:50:38 UTC +00:00], ["sort", 0], ["updated_at", Wed, 15 Jan 2014 09:50:38 UTC +00:00]]
|
464
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
465
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
466
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:50:38 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:50:38 UTC +00:00]]
|
467
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
468
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
469
|
+
[1m[35mSQL (0.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:50:38 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:50:38 UTC +00:00]]
|
470
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
471
|
+
Processing by SortableController#reorder as HTML
|
472
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
473
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" ORDER BY "items"."sort" ASC
|
474
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
475
|
+
[1m[35mSQL (0.2ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", nil]]
|
476
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
477
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
478
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 2[0m [["sort", nil]]
|
479
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
480
|
+
Rendered text template (0.0ms)
|
481
|
+
Completed 200 OK in 16ms (Views: 10.5ms | ActiveRecord: 0.7ms)
|
482
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 1]]
|
483
|
+
[1m[35mItem Load (0.0ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 2]]
|
484
|
+
[1m[36m (1.7ms)[0m [1mrollback transaction[0m
|
485
|
+
[1m[35m (0.1ms)[0m begin transaction
|
486
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
487
|
+
[1m[35m (0.0ms)[0m begin transaction
|
488
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
489
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
490
|
+
[1m[35m (0.1ms)[0m begin transaction
|
491
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
492
|
+
[1m[35m (0.1ms)[0m begin transaction
|
493
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
494
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
495
|
+
[1m[35m (0.1ms)[0m begin transaction
|
496
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
497
|
+
[1m[35mSQL (3.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:52:09 UTC +00:00], ["sort", 0], ["updated_at", Wed, 15 Jan 2014 09:52:09 UTC +00:00]]
|
498
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
499
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
500
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:52:09 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:52:09 UTC +00:00]]
|
501
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
502
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
503
|
+
[1m[35mSQL (0.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:52:09 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:52:09 UTC +00:00]]
|
504
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
505
|
+
Processing by SortableController#reorder as HTML
|
506
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
507
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" ORDER BY "items"."sort" ASC
|
508
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
509
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", nil]]
|
510
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
511
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
512
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 2[0m [["sort", nil]]
|
513
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
514
|
+
Rendered text template (0.0ms)
|
515
|
+
Completed 200 OK in 10ms (Views: 5.6ms | ActiveRecord: 0.6ms)
|
516
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 1]]
|
517
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 2]]
|
518
|
+
[1m[36m (1.9ms)[0m [1mrollback transaction[0m
|
519
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
520
|
+
[1m[35m (0.1ms)[0m begin transaction
|
521
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
522
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:57:16 UTC +00:00], ["sort", 0], ["updated_at", Wed, 15 Jan 2014 09:57:16 UTC +00:00]]
|
523
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
524
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
525
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:57:16 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:57:16 UTC +00:00]]
|
526
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
527
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
528
|
+
[1m[35mSQL (0.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 09:57:16 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:57:16 UTC +00:00]]
|
529
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
530
|
+
[1m[35m (1.6ms)[0m rollback transaction
|
531
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
532
|
+
[1m[35m (0.1ms)[0m rollback transaction
|
533
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
534
|
+
[1m[35m (0.1ms)[0m rollback transaction
|
535
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
536
|
+
[1m[35m (0.1ms)[0m begin transaction
|
537
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
538
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
539
|
+
[1m[36mSQL (3.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:57:33 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:57:33 UTC +00:00]]
|
540
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
541
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
542
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
543
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:57:33 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:57:33 UTC +00:00]]
|
544
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
545
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
546
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
547
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:57:33 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 09:57:33 UTC +00:00]]
|
548
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
549
|
+
Processing by SortableController#reorder as HTML
|
550
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
551
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
552
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
553
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", nil]]
|
554
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
555
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
556
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", nil]]
|
557
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
558
|
+
Rendered text template (0.0ms)
|
559
|
+
Completed 200 OK in 11ms (Views: 5.9ms | ActiveRecord: 0.7ms)
|
560
|
+
[1m[35mItem Load (0.2ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
561
|
+
[1m[36m (1.9ms)[0m [1mrollback transaction[0m
|
562
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
563
|
+
[1m[35m (0.1ms)[0m begin transaction
|
564
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
565
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
566
|
+
[1m[36mSQL (3.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:58:16 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:58:16 UTC +00:00]]
|
567
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
568
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
569
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
570
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:58:16 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:58:16 UTC +00:00]]
|
571
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
572
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
573
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
574
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:58:16 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 09:58:16 UTC +00:00]]
|
575
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
576
|
+
Processing by SortableController#reorder as HTML
|
577
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
578
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
579
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
580
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", nil]]
|
581
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
582
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
583
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", nil]]
|
584
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
585
|
+
Rendered text template (0.0ms)
|
586
|
+
Completed 200 OK in 10ms (Views: 5.2ms | ActiveRecord: 0.7ms)
|
587
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
588
|
+
[1m[36m (1.4ms)[0m [1mrollback transaction[0m
|
589
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
590
|
+
[1m[35m (0.1ms)[0m begin transaction
|
591
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
592
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
593
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:58:25 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:58:25 UTC +00:00]]
|
594
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
595
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
596
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
597
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:58:25 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:58:25 UTC +00:00]]
|
598
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
599
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
600
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
601
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:58:25 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 09:58:25 UTC +00:00]]
|
602
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
603
|
+
Processing by SortableController#reorder as HTML
|
604
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
605
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
606
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
607
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", nil]]
|
608
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
609
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
610
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", nil]]
|
611
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
612
|
+
Rendered text template (0.0ms)
|
613
|
+
Completed 200 OK in 11ms (Views: 6.0ms | ActiveRecord: 0.6ms)
|
614
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
615
|
+
[1m[36m (1.7ms)[0m [1mrollback transaction[0m
|
616
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
617
|
+
[1m[35m (0.1ms)[0m begin transaction
|
618
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
619
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
620
|
+
[1m[36mSQL (3.6ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:59:36 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 09:59:36 UTC +00:00]]
|
621
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
622
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
623
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
624
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:59:36 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 09:59:36 UTC +00:00]]
|
625
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
626
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
627
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
628
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 09:59:36 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 09:59:36 UTC +00:00]]
|
629
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
630
|
+
Processing by SortableController#reorder as HTML
|
631
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
632
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
633
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
634
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", nil]]
|
635
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
636
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
637
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", nil]]
|
638
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
639
|
+
Rendered text template (0.0ms)
|
640
|
+
Completed 200 OK in 10ms (Views: 5.9ms | ActiveRecord: 0.5ms)
|
641
|
+
[1m[35m (0.1ms)[0m SELECT "items"."sort" FROM "items" ORDER BY "items"."sort" ASC
|
642
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 1]]
|
643
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
644
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
645
|
+
[1m[35m (0.1ms)[0m begin transaction
|
646
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
647
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
648
|
+
[1m[36mSQL (3.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:12 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:00:12 UTC +00:00]]
|
649
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
650
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
651
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
652
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:12 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 10:00:12 UTC +00:00]]
|
653
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
654
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
655
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
656
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:12 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 10:00:12 UTC +00:00]]
|
657
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
658
|
+
[1m[36m (0.1ms)[0m [1mSELECT "items"."sort" FROM "items" ORDER BY "items"."sort" ASC[0m
|
659
|
+
Processing by SortableController#reorder as HTML
|
660
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
661
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" ORDER BY "items"."sort" ASC
|
662
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
663
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", nil]]
|
664
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
665
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
666
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 3[0m [["sort", nil]]
|
667
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
668
|
+
Rendered text template (0.0ms)
|
669
|
+
Completed 200 OK in 9ms (Views: 5.1ms | ActiveRecord: 0.5ms)
|
670
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 1]]
|
671
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
672
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
673
|
+
[1m[35m (0.1ms)[0m begin transaction
|
674
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
675
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
676
|
+
[1m[36mSQL (3.7ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:42 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:00:42 UTC +00:00]]
|
677
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
678
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
679
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
680
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:42 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 10:00:42 UTC +00:00]]
|
681
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
682
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
683
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
684
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:42 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 10:00:42 UTC +00:00]]
|
685
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
686
|
+
Processing by SortableController#reorder as HTML
|
687
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
688
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
689
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
690
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 0]]
|
691
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
692
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
693
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", 1]]
|
694
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
695
|
+
Rendered text template (0.1ms)
|
696
|
+
Completed 200 OK in 13ms (Views: 8.6ms | ActiveRecord: 0.5ms)
|
697
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
698
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 2]]
|
699
|
+
[1m[35mItem Load (0.0ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 3]]
|
700
|
+
[1m[36m (1.7ms)[0m [1mrollback transaction[0m
|
701
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
702
|
+
[1m[35m (0.1ms)[0m begin transaction
|
703
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
704
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
705
|
+
[1m[36mSQL (3.8ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:52 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:00:52 UTC +00:00]]
|
706
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
707
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
708
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
709
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:52 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 10:00:52 UTC +00:00]]
|
710
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
711
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
712
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
713
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:00:52 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 10:00:52 UTC +00:00]]
|
714
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
715
|
+
Processing by SortableController#reorder as HTML
|
716
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
717
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
718
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
719
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 0]]
|
720
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
721
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
722
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 3 [["sort", 1]]
|
723
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
724
|
+
Rendered text template (0.0ms)
|
725
|
+
Completed 200 OK in 16ms (Views: 11.6ms | ActiveRecord: 0.5ms)
|
726
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
727
|
+
[1m[36mItem Load (0.0ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 2]]
|
728
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 3]]
|
729
|
+
[1m[36m (2.4ms)[0m [1mrollback transaction[0m
|
730
|
+
[1m[35m (0.1ms)[0m begin transaction
|
731
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
732
|
+
[1m[35m (0.1ms)[0m begin transaction
|
733
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
734
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
735
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
736
|
+
[1m[35m (0.1ms)[0m begin transaction
|
737
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
738
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:06:22 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:06:22 UTC +00:00]]
|
739
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
740
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
741
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
742
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:06:22 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:06:22 UTC +00:00]]
|
743
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
744
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
745
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
746
|
+
[1m[35m (0.1ms)[0m begin transaction
|
747
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
748
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:09:39 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:09:39 UTC +00:00]]
|
749
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
750
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
751
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
752
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:09:39 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:09:39 UTC +00:00]]
|
753
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
754
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
755
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
756
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
757
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:09:39 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:09:39 UTC +00:00]]
|
758
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
759
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
760
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
761
|
+
[1m[35m (0.1ms)[0m begin transaction
|
762
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
763
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
764
|
+
[1m[36mSQL (3.8ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:12:13 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:12:13 UTC +00:00]]
|
765
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
766
|
+
[1m[36m (8.0ms)[0m [1mrollback transaction[0m
|
767
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
768
|
+
[1m[35m (0.1ms)[0m begin transaction
|
769
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
770
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
771
|
+
[1m[36mSQL (3.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:12:27 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:12:27 UTC +00:00]]
|
772
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
773
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
774
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
775
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
776
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
777
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
778
|
+
[1m[35m (0.1ms)[0m begin transaction
|
779
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
780
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
781
|
+
[1m[36mSQL (3.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:12:40 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:12:40 UTC +00:00]]
|
782
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
783
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
784
|
+
[1m[35mSQL (0.4ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1 [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:12:40 UTC +00:00]]
|
785
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
786
|
+
[1m[35m (1.6ms)[0m rollback transaction
|
787
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
788
|
+
[1m[35m (0.1ms)[0m begin transaction
|
789
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
790
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
791
|
+
[1m[36mSQL (3.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:12:50 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:12:50 UTC +00:00]]
|
792
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
793
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
794
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
795
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
796
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
797
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
798
|
+
[1m[35m (0.1ms)[0m begin transaction
|
799
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
800
|
+
[1m[35m (0.0ms)[0m begin transaction
|
801
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
802
|
+
[1m[35mSQL (4.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:15:09 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:15:09 UTC +00:00]]
|
803
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
804
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
805
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
806
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
807
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
808
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:15:09 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:15:09 UTC +00:00]]
|
809
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
810
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
811
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 1000]]
|
812
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
813
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
814
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
815
|
+
[1m[35m (0.1ms)[0m begin transaction
|
816
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
817
|
+
[1m[35m (0.0ms)[0m begin transaction
|
818
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
819
|
+
[1m[35mSQL (2.9ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:15:23 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:15:23 UTC +00:00]]
|
820
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
821
|
+
[1m[35m (1.6ms)[0m rollback transaction
|
822
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
823
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
824
|
+
[1m[36m (0.2ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
825
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:15:23 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:15:23 UTC +00:00]]
|
826
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
827
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
828
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 1000]]
|
829
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
830
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
831
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
832
|
+
[1m[35m (0.1ms)[0m begin transaction
|
833
|
+
[1m[36m (0.0ms)[0m [1mrollback transaction[0m
|
834
|
+
[1m[35m (0.1ms)[0m begin transaction
|
835
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
836
|
+
[1m[35mSQL (3.0ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:15:37 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:15:37 UTC +00:00]]
|
837
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
838
|
+
[1m[35m (1.6ms)[0m rollback transaction
|
839
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
840
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
841
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:15:37 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:15:37 UTC +00:00]]
|
842
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
843
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
844
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
845
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:15:37 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:15:37 UTC +00:00]]
|
846
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
847
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
848
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
849
|
+
[1m[35m (0.1ms)[0m begin transaction
|
850
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
851
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00]]
|
852
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
853
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
854
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
855
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00]]
|
856
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
857
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
858
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
859
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
860
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00]]
|
861
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
862
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
863
|
+
[1m[35m (0.0ms)[0m begin transaction
|
864
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
865
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
866
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:15:53 UTC +00:00]]
|
867
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
868
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
869
|
+
[1m[35mSQL (0.4ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
870
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
871
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
872
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
873
|
+
[1m[35m (0.1ms)[0m begin transaction
|
874
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
875
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
876
|
+
[1m[36mSQL (3.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00]]
|
877
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
878
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
879
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
880
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
881
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
882
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
883
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
884
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00]]
|
885
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
886
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
887
|
+
[1m[35m (0.1ms)[0m begin transaction
|
888
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
889
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00]]
|
890
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
891
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
892
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
893
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:16:47 UTC +00:00]]
|
894
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
895
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
896
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
897
|
+
[1m[35m (0.1ms)[0m begin transaction
|
898
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
899
|
+
[1m[35mSQL (3.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00]]
|
900
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
901
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
902
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
903
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00]]
|
904
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
905
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
906
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
907
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
908
|
+
[1m[36mSQL (0.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00]]
|
909
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
910
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
911
|
+
[1m[35m (0.0ms)[0m begin transaction
|
912
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
913
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
914
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00]]
|
915
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
916
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
917
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
918
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
919
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
920
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
921
|
+
[1m[35m (0.1ms)[0m rollback transaction
|
922
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
923
|
+
[1m[35m (0.1ms)[0m rollback transaction
|
924
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
925
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
926
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
927
|
+
[1m[35mSQL (0.5ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00]]
|
928
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
929
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
930
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
931
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00]]
|
932
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
933
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
934
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
935
|
+
[1m[35mSQL (0.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 10:17:09 UTC +00:00]]
|
936
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
937
|
+
Processing by SortableController#reorder as HTML
|
938
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
939
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" ORDER BY "items"."sort" ASC
|
940
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
941
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 0]]
|
942
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
943
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
944
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 3[0m [["sort", 1]]
|
945
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
946
|
+
Completed 200 OK in 8ms (Views: 4.1ms | ActiveRecord: 0.5ms)
|
947
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 1]]
|
948
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 2]]
|
949
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 3]]
|
950
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
951
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
952
|
+
[1m[35m (0.1ms)[0m begin transaction
|
953
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
954
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00]]
|
955
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
956
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
957
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
958
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00]]
|
959
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
960
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
961
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
962
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
963
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00]]
|
964
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
965
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
966
|
+
[1m[35m (0.1ms)[0m begin transaction
|
967
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
968
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
969
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:18:46 UTC +00:00]]
|
970
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
971
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
972
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
973
|
+
[1m[35m (0.1ms)[0m begin transaction
|
974
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
975
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
976
|
+
[1m[36mSQL (4.9ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:18:59 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:18:59 UTC +00:00]]
|
977
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
978
|
+
[1m[36m (1.8ms)[0m [1mrollback transaction[0m
|
979
|
+
[1m[35m (0.1ms)[0m begin transaction
|
980
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
981
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("sort") VALUES (?) [["sort", 1000]]
|
982
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
983
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
984
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
985
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
986
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1000]]
|
987
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
988
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
989
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
990
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1001]]
|
991
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
992
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
993
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
994
|
+
[1m[35m (0.1ms)[0m begin transaction
|
995
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
996
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00]]
|
997
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
998
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
999
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1000
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00]]
|
1001
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1002
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
1003
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1004
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1005
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00]]
|
1006
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1007
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1008
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1009
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1010
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1011
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:19:11 UTC +00:00]]
|
1012
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1013
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1014
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1015
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1016
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1017
|
+
[1m[35mSQL (3.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00]]
|
1018
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1019
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1020
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1021
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00]]
|
1022
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1023
|
+
[1m[35m (1.8ms)[0m rollback transaction
|
1024
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1025
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1026
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00]]
|
1027
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1028
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1029
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1030
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1031
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1032
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:19:31 UTC +00:00]]
|
1033
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1034
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1035
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1036
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1037
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1038
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1039
|
+
[1m[36mSQL (3.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:19:51 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:19:51 UTC +00:00]]
|
1040
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1041
|
+
[1m[36m (1.9ms)[0m [1mrollback transaction[0m
|
1042
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1043
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1044
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("sort") VALUES (?) [["sort", 1000]]
|
1045
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1046
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1047
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1048
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1049
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1000]]
|
1050
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1051
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1052
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1053
|
+
[1m[36mSQL (0.2ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1001]]
|
1054
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1055
|
+
[1m[36m (0.5ms)[0m [1mrollback transaction[0m
|
1056
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1057
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1058
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1059
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1060
|
+
[1m[36mSQL (3.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:22:27 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:22:27 UTC +00:00]]
|
1061
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1062
|
+
[1m[36m (1.9ms)[0m [1mrollback transaction[0m
|
1063
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1064
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1065
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("sort") VALUES (?) [["sort", 1000]]
|
1066
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1067
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1068
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1069
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1070
|
+
[1m[36mSQL (0.2ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1000]]
|
1071
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1072
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1073
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1074
|
+
[1m[36mSQL (0.2ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1001]]
|
1075
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1076
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1077
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1078
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1079
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1080
|
+
[1m[35mSQL (4.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00]]
|
1081
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1082
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1083
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1084
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00]]
|
1085
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1086
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
1087
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1088
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1089
|
+
[1m[36mSQL (0.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00]]
|
1090
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1091
|
+
[1m[36m (0.6ms)[0m [1mrollback transaction[0m
|
1092
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1093
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1094
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1095
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:23:04 UTC +00:00]]
|
1096
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1097
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1098
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1099
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1100
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1101
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1102
|
+
[1m[36mSQL (3.8ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:24:11 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:24:11 UTC +00:00]]
|
1103
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1104
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1105
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1106
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1107
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("sort") VALUES (?) [["sort", 1000]]
|
1108
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1109
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1110
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1111
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1112
|
+
[1m[36mSQL (0.2ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1000]]
|
1113
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1114
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1115
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1116
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1001]]
|
1117
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1118
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1119
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1120
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1121
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1122
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1123
|
+
[1m[36mSQL (3.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:24:30 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:24:30 UTC +00:00]]
|
1124
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1125
|
+
[1m[36m (1.7ms)[0m [1mrollback transaction[0m
|
1126
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1127
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1128
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("sort") VALUES (?) [["sort", 1000]]
|
1129
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1130
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1131
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1132
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1133
|
+
[1m[36mSQL (0.2ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1000]]
|
1134
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1135
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1136
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1137
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1001]]
|
1138
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1139
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1140
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1141
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1142
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1143
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00]]
|
1144
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1145
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1146
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1147
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00]]
|
1148
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1149
|
+
[1m[35m (1.8ms)[0m rollback transaction
|
1150
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1151
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1152
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00]]
|
1153
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1154
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1155
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1156
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1157
|
+
[1m[35m (0.3ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1158
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:24:34 UTC +00:00]]
|
1159
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1160
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1161
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1162
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1163
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1164
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1165
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00]]
|
1166
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1167
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1168
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1169
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00]]
|
1170
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1171
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1172
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1173
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1174
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00]]
|
1175
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1176
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1177
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1178
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1179
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1180
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:25:12 UTC +00:00]]
|
1181
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1182
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1183
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1184
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1185
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1186
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1187
|
+
[1m[36mSQL (7.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:32:21 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:32:21 UTC +00:00]]
|
1188
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1189
|
+
[1m[36m (1.5ms)[0m [1mrollback transaction[0m
|
1190
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1191
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1192
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1193
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1194
|
+
[1m[36mSQL (3.7ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00]]
|
1195
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1196
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1197
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1198
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00]]
|
1199
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1200
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1201
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1202
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00]]
|
1203
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1204
|
+
Processing by SortableController#reorder as HTML
|
1205
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
1206
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
1207
|
+
Completed 500 Internal Server Error in 1ms
|
1208
|
+
[1m[35m (1.6ms)[0m rollback transaction
|
1209
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1210
|
+
[1m[35m (0.1ms)[0m rollback transaction
|
1211
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1212
|
+
[1m[35m (0.1ms)[0m rollback transaction
|
1213
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1214
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1215
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00]]
|
1216
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1217
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1218
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1219
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00]]
|
1220
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1221
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1222
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1223
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1224
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00]]
|
1225
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1226
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1227
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1228
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1229
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1230
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:32:48 UTC +00:00]]
|
1231
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1232
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1233
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1234
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1235
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1236
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1237
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:33:57 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:33:57 UTC +00:00]]
|
1238
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1239
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1240
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1241
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1242
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1243
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1244
|
+
[1m[36mSQL (3.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:34:27 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:34:27 UTC +00:00]]
|
1245
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1246
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1247
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1248
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1249
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("sort") VALUES (?) [["sort", 1000]]
|
1250
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1251
|
+
[1m[35m (0.5ms)[0m rollback transaction
|
1252
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1253
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1254
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1000]]
|
1255
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1256
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1257
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1258
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("sort") VALUES (?)[0m [["sort", 1001]]
|
1259
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1260
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1261
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1262
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1263
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1264
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1265
|
+
[1m[36mSQL (5.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:39:47 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:39:47 UTC +00:00]]
|
1266
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1267
|
+
[1m[36m (1.4ms)[0m [1mrollback transaction[0m
|
1268
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1269
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1270
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1271
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1272
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1273
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1274
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1275
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:43:43 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:43:43 UTC +00:00]]
|
1276
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1277
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1278
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1279
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1280
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
1281
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1282
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1283
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1284
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1285
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1286
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:44:28 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:44:28 UTC +00:00]]
|
1287
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1288
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1289
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1290
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1291
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1292
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1293
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1294
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1295
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1296
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:44:44 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:44:44 UTC +00:00]]
|
1297
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1298
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1299
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1300
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1301
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1302
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1303
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1304
|
+
[1m[35m (0.2ms)[0m begin transaction
|
1305
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1306
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00]]
|
1307
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1308
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1309
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1310
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00]]
|
1311
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1312
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1313
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1314
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1315
|
+
[1m[36mSQL (0.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00]]
|
1316
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1317
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1318
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1319
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1320
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1321
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:45:52 UTC +00:00]]
|
1322
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1323
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1324
|
+
[1m[35mSQL (0.5ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1325
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1326
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1327
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1328
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1329
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1330
|
+
[1m[35mSQL (3.0ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00]]
|
1331
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1332
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1333
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1334
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00]]
|
1335
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1336
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
1337
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1338
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1339
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00]]
|
1340
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1341
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1342
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1343
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1344
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1345
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:46:11 UTC +00:00]]
|
1346
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1347
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1348
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1349
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1350
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1351
|
+
[1m[35mSQL (3.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00]]
|
1352
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1353
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1354
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1355
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00]]
|
1356
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1357
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1358
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1359
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1360
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00]]
|
1361
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1362
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1363
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1364
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1365
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1366
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:46:20 UTC +00:00]]
|
1367
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1368
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1369
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1370
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1371
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1372
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1373
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1374
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1375
|
+
[1m[35mSQL (3.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00]]
|
1376
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1377
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1378
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1379
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00]]
|
1380
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1381
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
1382
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1383
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1384
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00]]
|
1385
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1386
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1387
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1388
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1389
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1390
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:47:21 UTC +00:00]]
|
1391
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1392
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1393
|
+
[1m[35mSQL (0.5ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1394
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1395
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1396
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1397
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1398
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1399
|
+
[1m[35mSQL (2.9ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00]]
|
1400
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1401
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1402
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "updated_at") VALUES (?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00], ["updated_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00]]
|
1403
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1404
|
+
[1m[36m (1.7ms)[0m [1mrollback transaction[0m
|
1405
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1406
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1407
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00]]
|
1408
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1409
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1410
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1411
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1412
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "updated_at") VALUES (?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00], ["updated_at", Wed, 15 Jan 2014 10:48:28 UTC +00:00]]
|
1413
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1414
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1415
|
+
[1m[35mSQL (0.4ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1416
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1417
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1418
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1419
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1420
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1421
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00]]
|
1422
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1423
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1424
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1425
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00]]
|
1426
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1427
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1428
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1429
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1430
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00]]
|
1431
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1432
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1433
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1434
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1435
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1436
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:48:52 UTC +00:00]]
|
1437
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1438
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1439
|
+
[1m[35mSQL (0.5ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1440
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1441
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1442
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1443
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1444
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1445
|
+
[1m[35mSQL (2.9ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00]]
|
1446
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1447
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1448
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1449
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00]]
|
1450
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1451
|
+
[1m[35m (2.1ms)[0m rollback transaction
|
1452
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1453
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1454
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00]]
|
1455
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1456
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1457
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1458
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1459
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1460
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:50:17 UTC +00:00]]
|
1461
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1462
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1463
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1464
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1465
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1466
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1467
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1468
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1469
|
+
[1m[35mSQL (5.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00]]
|
1470
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1471
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1472
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1473
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00]]
|
1474
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1475
|
+
[1m[35m (2.0ms)[0m rollback transaction
|
1476
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1477
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1478
|
+
[1m[36mSQL (0.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00]]
|
1479
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1480
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1481
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1482
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1483
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1484
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:50:39 UTC +00:00]]
|
1485
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1486
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1487
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1488
|
+
[1m[36m (6.7ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1489
|
+
[1m[35m (0.5ms)[0m rollback transaction
|
1490
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1491
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1492
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1493
|
+
[1m[35mSQL (3.7ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00]]
|
1494
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1495
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1496
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1497
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00]]
|
1498
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1499
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1500
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1501
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1502
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00]]
|
1503
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1504
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1505
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1506
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1507
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1508
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:51:13 UTC +00:00]]
|
1509
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1510
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1511
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1512
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1513
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1514
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1515
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1516
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
1517
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1518
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1519
|
+
[1m[35m (0.1ms)[0m ROLLBACK TO SAVEPOINT active_record_1
|
1520
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
1521
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1522
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1523
|
+
[1m[35m (0.1ms)[0m ROLLBACK TO SAVEPOINT active_record_1
|
1524
|
+
[1m[36m (0.0ms)[0m [1mrollback transaction[0m
|
1525
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1526
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1527
|
+
[1m[35m (0.0ms)[0m ROLLBACK TO SAVEPOINT active_record_1
|
1528
|
+
[1m[36m (0.0ms)[0m [1mrollback transaction[0m
|
1529
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1530
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1531
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1532
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00]]
|
1533
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1534
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1535
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1536
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00]]
|
1537
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1538
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1539
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1540
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1541
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00]]
|
1542
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1543
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1544
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1545
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1546
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1547
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00]]
|
1548
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1549
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1550
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1551
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1552
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1553
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1554
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1555
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1556
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:52:53 UTC +00:00]]
|
1557
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1558
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1559
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 1000]]
|
1560
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1561
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1562
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1563
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1564
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1565
|
+
[1m[35mSQL (3.5ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00]]
|
1566
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1567
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1568
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1569
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00]]
|
1570
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1571
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1572
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1573
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1574
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00]]
|
1575
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1576
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1577
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1578
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1579
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1580
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00]]
|
1581
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1582
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1583
|
+
[1m[35mSQL (0.4ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1584
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1585
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1586
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1587
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1588
|
+
[1m[36m (0.2ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1589
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:53:28 UTC +00:00]]
|
1590
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1591
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1592
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 1000]]
|
1593
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1594
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1595
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1596
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1597
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1598
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1599
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:54:20 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:54:20 UTC +00:00]]
|
1600
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1601
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1602
|
+
[1m[35mSQL (0.4ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1603
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1604
|
+
[1m[35m (1.5ms)[0m rollback transaction
|
1605
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1606
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1607
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1608
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1609
|
+
[1m[36mSQL (3.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:54:51 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:54:51 UTC +00:00]]
|
1610
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1611
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1612
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1613
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1614
|
+
[1m[35m (1.8ms)[0m rollback transaction
|
1615
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1616
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1617
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1618
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00]]
|
1619
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1620
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1621
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1622
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00]]
|
1623
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1624
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1625
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1626
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1627
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00]]
|
1628
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1629
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1630
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1631
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1632
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1633
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00]]
|
1634
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1635
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1636
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1637
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1638
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1639
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1640
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1641
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1642
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:54:55 UTC +00:00]]
|
1643
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1644
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1645
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 1000]]
|
1646
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1647
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1648
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1649
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1650
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1651
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00]]
|
1652
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1653
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1654
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1655
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00]]
|
1656
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1657
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1658
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1659
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1660
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00]]
|
1661
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1662
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1663
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1664
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1665
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1666
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00]]
|
1667
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1668
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1669
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1670
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1671
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1672
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:55:27 UTC +00:00]]
|
1673
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1674
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1675
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1676
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1677
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1678
|
+
[1m[35mSQL (2.9ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00]]
|
1679
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1680
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1681
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1682
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00]]
|
1683
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1684
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1685
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1686
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1687
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00]]
|
1688
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1689
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1690
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1691
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1692
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1693
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00]]
|
1694
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1695
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1696
|
+
[1m[35mSQL (0.4ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1697
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1698
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1699
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
1700
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1701
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1702
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 10:55:47 UTC +00:00]]
|
1703
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1704
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1705
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1706
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1707
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1708
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1709
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1710
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1711
|
+
[1m[36mSQL (3.6ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00]]
|
1712
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1713
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1714
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1715
|
+
[1m[36m (1.5ms)[0m [1mrollback transaction[0m
|
1716
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1717
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1718
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1719
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00]]
|
1720
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1721
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1722
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1723
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1724
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1725
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1726
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1727
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00]]
|
1728
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1729
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1730
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1731
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1732
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00]]
|
1733
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1734
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1735
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1736
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:00:21 UTC +00:00]]
|
1737
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1738
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1739
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1740
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1741
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1742
|
+
[1m[35mSQL (3.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00]]
|
1743
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1744
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1745
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1746
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00]]
|
1747
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1748
|
+
[1m[35m (1.5ms)[0m rollback transaction
|
1749
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1750
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1751
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00]]
|
1752
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1753
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1754
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1755
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1756
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1757
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00]]
|
1758
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1759
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1760
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1761
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1762
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
1763
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1764
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1765
|
+
[1m[36m (0.2ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1766
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:00:34 UTC +00:00]]
|
1767
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1768
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1769
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1770
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1771
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1772
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1773
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1774
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1775
|
+
[1m[36mSQL (3.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:01:14 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:01:14 UTC +00:00]]
|
1776
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1777
|
+
[1m[36m (0.2ms)[0m [1mSAVEPOINT active_record_1[0m
|
1778
|
+
[1m[35m (0.2ms)[0m RELEASE SAVEPOINT active_record_1
|
1779
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.3ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1780
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1781
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1782
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1783
|
+
[1m[36mSQL (3.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:01:41 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:01:41 UTC +00:00]]
|
1784
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1785
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1786
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1787
|
+
[1m[36m (1.6ms)[0m [1mrollback transaction[0m
|
1788
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1789
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1790
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1791
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1792
|
+
[1m[36mSQL (3.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:01:46 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:01:46 UTC +00:00]]
|
1793
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1794
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1795
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1796
|
+
[1m[36m (1.7ms)[0m [1mrollback transaction[0m
|
1797
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1798
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1799
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1800
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1801
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:01:51 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:01:51 UTC +00:00]]
|
1802
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1803
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1804
|
+
[1m[35mSQL (1.2ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1805
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1806
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
1807
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1808
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1809
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1810
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1811
|
+
[1m[36mSQL (3.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:02:04 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:02:04 UTC +00:00]]
|
1812
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1813
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1814
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1815
|
+
[1m[36m (1.6ms)[0m [1mrollback transaction[0m
|
1816
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1817
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1818
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1819
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1820
|
+
[1m[36mSQL (2.9ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:02:15 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:02:15 UTC +00:00]]
|
1821
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1822
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1823
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1824
|
+
[1m[36m (1.8ms)[0m [1mrollback transaction[0m
|
1825
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1826
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1827
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1828
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1829
|
+
[1m[36mSQL (3.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:02:53 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:02:53 UTC +00:00]]
|
1830
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1831
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1832
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1833
|
+
[1m[36m (0.8ms)[0m [1mrollback transaction[0m
|
1834
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1835
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1836
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1837
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1838
|
+
[1m[36mSQL (3.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:03:09 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:03:09 UTC +00:00]]
|
1839
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1840
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1841
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1842
|
+
[1m[36m (1.8ms)[0m [1mrollback transaction[0m
|
1843
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1844
|
+
[1m[35m (0.2ms)[0m begin transaction
|
1845
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1846
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1847
|
+
[1m[36mSQL (4.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:03:45 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:03:45 UTC +00:00]]
|
1848
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1849
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1850
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1851
|
+
[1m[36m (0.5ms)[0m [1mrollback transaction[0m
|
1852
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1853
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1854
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1855
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1856
|
+
[1m[36mSQL (3.9ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:03:51 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:03:51 UTC +00:00]]
|
1857
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1858
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1859
|
+
[1m[35mSQL (0.4ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1860
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1861
|
+
[1m[35m (1.4ms)[0m rollback transaction
|
1862
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1863
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1864
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1865
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1866
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:05:21 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:05:21 UTC +00:00]]
|
1867
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1868
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1869
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1870
|
+
[1m[36m (1.6ms)[0m [1mrollback transaction[0m
|
1871
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1872
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1873
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1874
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1875
|
+
[1m[36mSQL (3.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:05:40 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:05:40 UTC +00:00]]
|
1876
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1877
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1878
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1879
|
+
[1m[36m (1.6ms)[0m [1mrollback transaction[0m
|
1880
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1881
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1882
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1883
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1884
|
+
[1m[36mSQL (4.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:05:57 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:05:57 UTC +00:00]]
|
1885
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1886
|
+
[1m[36m (0.2ms)[0m [1mSAVEPOINT active_record_1[0m
|
1887
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1888
|
+
[1m[36m (1.8ms)[0m [1mrollback transaction[0m
|
1889
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1890
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1891
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1892
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1893
|
+
[1m[36mSQL (3.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:06:28 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:06:28 UTC +00:00]]
|
1894
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1895
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1896
|
+
[1m[35mSQL (0.6ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1 [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:06:28 UTC +00:00]]
|
1897
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1898
|
+
[1m[35m (1.6ms)[0m rollback transaction
|
1899
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1900
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1901
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1902
|
+
[1m[35mSQL (3.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00]]
|
1903
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1904
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1905
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1906
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00]]
|
1907
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1908
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1909
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1910
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1911
|
+
[1m[36mSQL (0.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00]]
|
1912
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1913
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1914
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1915
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1916
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1917
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00]]
|
1918
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1919
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1920
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1921
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1922
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1923
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1924
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1925
|
+
[1m[36m (0.2ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1926
|
+
[1m[35mSQL (0.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00]]
|
1927
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1928
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1929
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:06:32 UTC +00:00]]
|
1930
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1931
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1932
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1933
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1934
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
1935
|
+
[1m[35mSQL (3.2ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00]]
|
1936
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1937
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1938
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1939
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00]]
|
1940
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1941
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
1942
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1943
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1944
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00]]
|
1945
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1946
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1947
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1948
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1949
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1950
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00]]
|
1951
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1952
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1953
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
1954
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1955
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
1956
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1957
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1958
|
+
[1m[36m (0.2ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1959
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00]]
|
1960
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1961
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1962
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:01 UTC +00:00]]
|
1963
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1964
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
1965
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1966
|
+
[1m[35m (0.1ms)[0m begin transaction
|
1967
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1968
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1969
|
+
[1m[36mSQL (3.5ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00]]
|
1970
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1971
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1972
|
+
[1m[35mSQL (0.5ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1 [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00]]
|
1973
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1974
|
+
[1m[35m (1.9ms)[0m rollback transaction
|
1975
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
1976
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1977
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
1978
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00]]
|
1979
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1980
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
1981
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ? WHERE "items"."id" = 1[0m [["sort", 1000]]
|
1982
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1983
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1984
|
+
[1m[35m (0.0ms)[0m begin transaction
|
1985
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1986
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00]]
|
1987
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
1988
|
+
[1m[35m (0.2ms)[0m rollback transaction
|
1989
|
+
[1m[36m (0.2ms)[0m [1mbegin transaction[0m
|
1990
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
1991
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:05 UTC +00:00]]
|
1992
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
1993
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
1994
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
1995
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:08:06 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:08:06 UTC +00:00]]
|
1996
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
1997
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
1998
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
1999
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2000
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2001
|
+
[1m[35mSQL (3.4ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00]]
|
2002
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2003
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2004
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2005
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00]]
|
2006
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2007
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
2008
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
2009
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
2010
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00]]
|
2011
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2012
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
2013
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2014
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2015
|
+
[1m[35m (0.2ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2016
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00]]
|
2017
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2018
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2019
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
2020
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2021
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
2022
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
2023
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
2024
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2025
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00]]
|
2026
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2027
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2028
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:08:56 UTC +00:00]]
|
2029
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2030
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
2031
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.2ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
2032
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2033
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2034
|
+
[1m[35mSQL (3.1ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2035
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2036
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2037
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2038
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2039
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2040
|
+
[1m[35m (0.7ms)[0m rollback transaction
|
2041
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
2042
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2043
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2044
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2045
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
2046
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2047
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2048
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2049
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2050
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2051
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2052
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
2053
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2054
|
+
[1m[35m (0.3ms)[0m rollback transaction
|
2055
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
2056
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2057
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2058
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2059
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2060
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2061
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2062
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2063
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
2064
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2065
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
2066
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2067
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
2068
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2069
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2070
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2071
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2072
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2073
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2074
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2075
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2076
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2077
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2078
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2079
|
+
[1m[36mSQL (0.2ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2080
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2081
|
+
Processing by SortableController#reorder as HTML
|
2082
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
2083
|
+
[1m[36mItem Load (0.2ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
2084
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
2085
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 0], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2086
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2087
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2088
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 3 [["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:13:53 UTC +00:00]]
|
2089
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2090
|
+
Completed 200 OK in 12ms (Views: 7.8ms | ActiveRecord: 0.7ms)
|
2091
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
2092
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 2]]
|
2093
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 3]]
|
2094
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
2095
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
2096
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2097
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2098
|
+
[1m[35mSQL (3.5ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00]]
|
2099
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2100
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2101
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2102
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00]]
|
2103
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2104
|
+
[1m[35m (1.7ms)[0m rollback transaction
|
2105
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
2106
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
2107
|
+
[1m[36mSQL (0.4ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00]]
|
2108
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2109
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
2110
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2111
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2112
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2113
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00]]
|
2114
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2115
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2116
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
2117
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2118
|
+
[1m[35m (0.5ms)[0m rollback transaction
|
2119
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
2120
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
2121
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2122
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00]]
|
2123
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2124
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2125
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 11:18:18 UTC +00:00]]
|
2126
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2127
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
2128
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2129
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
2130
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2131
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
2132
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2133
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2134
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2135
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00]]
|
2136
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2137
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2138
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2139
|
+
[1m[36mSQL (0.2ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00]]
|
2140
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2141
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2142
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2143
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00]]
|
2144
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2145
|
+
Processing by SortableController#reorder as HTML
|
2146
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
2147
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
2148
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
2149
|
+
[1m[36mSQL (0.1ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 0], ["updated_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00]]
|
2150
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2151
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2152
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 3 [["sort", 1], ["updated_at", Wed, 15 Jan 2014 11:18:19 UTC +00:00]]
|
2153
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2154
|
+
Completed 200 OK in 9ms (Views: 5.6ms | ActiveRecord: 0.6ms)
|
2155
|
+
[1m[35mItem Load (0.2ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
2156
|
+
[1m[36mItem Load (0.0ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 2]]
|
2157
|
+
[1m[35mItem Load (0.1ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 3]]
|
2158
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|
2159
|
+
[1m[36mActiveRecord::SchemaMigration Load (0.1ms)[0m [1mSELECT "schema_migrations".* FROM "schema_migrations"[0m
|
2160
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2161
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
2162
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2163
|
+
[1m[36m (0.1ms)[0m [1mrollback transaction[0m
|
2164
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2165
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2166
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2167
|
+
[1m[36mSQL (8.0ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2168
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2169
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2170
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2171
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 2], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2172
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2173
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2174
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2175
|
+
[1m[36mSQL (0.1ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 3], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2176
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2177
|
+
Processing by SortableController#reorder as HTML
|
2178
|
+
Parameters: {"Item"=>["1", "3", "2"]}
|
2179
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" ORDER BY "items"."sort" ASC[0m
|
2180
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2181
|
+
[1m[36mSQL (0.2ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 0], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2182
|
+
[1m[35m (0.1ms)[0m RELEASE SAVEPOINT active_record_1
|
2183
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2184
|
+
[1m[35mSQL (0.1ms)[0m UPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 3 [["sort", 1], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2185
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2186
|
+
Completed 200 OK in 15ms (Views: 10.3ms | ActiveRecord: 0.7ms)
|
2187
|
+
[1m[35mItem Load (0.2ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 1]]
|
2188
|
+
[1m[36mItem Load (0.1ms)[0m [1mSELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1[0m [["id", 2]]
|
2189
|
+
[1m[35mItem Load (0.0ms)[0m SELECT "items".* FROM "items" WHERE "items"."id" = ? ORDER BY "items"."sort" ASC LIMIT 1 [["id", 3]]
|
2190
|
+
[1m[36m (0.5ms)[0m [1mrollback transaction[0m
|
2191
|
+
[1m[35m (0.1ms)[0m begin transaction
|
2192
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2193
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2194
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2195
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2196
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2197
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 1001], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2198
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2199
|
+
[1m[35m (0.5ms)[0m rollback transaction
|
2200
|
+
[1m[36m (0.1ms)[0m [1mbegin transaction[0m
|
2201
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2202
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 1000], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2203
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2204
|
+
[1m[36m (0.3ms)[0m [1mrollback transaction[0m
|
2205
|
+
[1m[35m (0.0ms)[0m begin transaction
|
2206
|
+
[1m[36m (0.0ms)[0m [1mSAVEPOINT active_record_1[0m
|
2207
|
+
[1m[35m (0.1ms)[0m SELECT MAX("items"."sort") AS max_id FROM "items"
|
2208
|
+
[1m[36mSQL (0.3ms)[0m [1mINSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?)[0m [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2209
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2210
|
+
[1m[36m (0.1ms)[0m [1mSAVEPOINT active_record_1[0m
|
2211
|
+
[1m[35mSQL (0.3ms)[0m UPDATE "items" SET "sort" = ? WHERE "items"."id" = 1 [["sort", 1000]]
|
2212
|
+
[1m[36m (0.1ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2213
|
+
[1m[35m (0.4ms)[0m rollback transaction
|
2214
|
+
[1m[36m (0.0ms)[0m [1mbegin transaction[0m
|
2215
|
+
[1m[35m (0.1ms)[0m SAVEPOINT active_record_1
|
2216
|
+
[1m[36m (0.1ms)[0m [1mSELECT MAX("items"."sort") AS max_id FROM "items"[0m
|
2217
|
+
[1m[35mSQL (0.3ms)[0m INSERT INTO "items" ("created_at", "sort", "updated_at") VALUES (?, ?, ?) [["created_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00], ["sort", 1], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2218
|
+
[1m[36m (0.0ms)[0m [1mRELEASE SAVEPOINT active_record_1[0m
|
2219
|
+
[1m[35m (0.0ms)[0m SAVEPOINT active_record_1
|
2220
|
+
[1m[36mSQL (0.3ms)[0m [1mUPDATE "items" SET "sort" = ?, "updated_at" = ? WHERE "items"."id" = 1[0m [["sort", 1000], ["updated_at", Wed, 15 Jan 2014 13:53:17 UTC +00:00]]
|
2221
|
+
[1m[35m (0.0ms)[0m RELEASE SAVEPOINT active_record_1
|
2222
|
+
[1m[36m (0.4ms)[0m [1mrollback transaction[0m
|