rails-marker 0.1.2 → 0.2.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +5 -5
- data/README.md +13 -0
- data/Rakefile +3 -2
- data/app/assets/javascripts/marker/init.js +15 -0
- data/app/assets/javascripts/marker/map_google.js +146 -0
- data/app/views/marker/_google.html.erb +1 -1
- data/lib/marker/helpers/field_tag.rb +4 -0
- data/lib/marker/version.rb +1 -1
- metadata +22 -9
- data/app/assets/javascripts/marker/init.js.coffee +0 -8
- data/app/assets/javascripts/marker/map_google.js.coffee +0 -108
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
|
-
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: da6f80ba26d842eb913f298d647d3ae21588f5fe440c8aa2eda0538a8ae55fd0
|
4
|
+
data.tar.gz: 487b0f7160ce7cfd8ff789cfa8c1c77c1a321b79266431378d5526b02f383b8e
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 5cedf637c1472a4041282c371a5e1f8a57ec9b8fe8dd9ecef3c9c5a6a0daf9f2791b635cb3f5d6151e2d2feab67110f792da0864a3c341a43d9654b2e4bf9a0a
|
7
|
+
data.tar.gz: 5fccc7147dd2d35a1a73aea74467538f4413a818340da50ee553d06c171347ca877f1430e5933cb931d14b4ef0238752c7f908298b8de67e01adba96dc4d9291
|
data/README.md
CHANGED
@@ -71,6 +71,19 @@ It's all you need! Just move the marker, and zoom, latitude and longitude fields
|
|
71
71
|
|
72
72
|
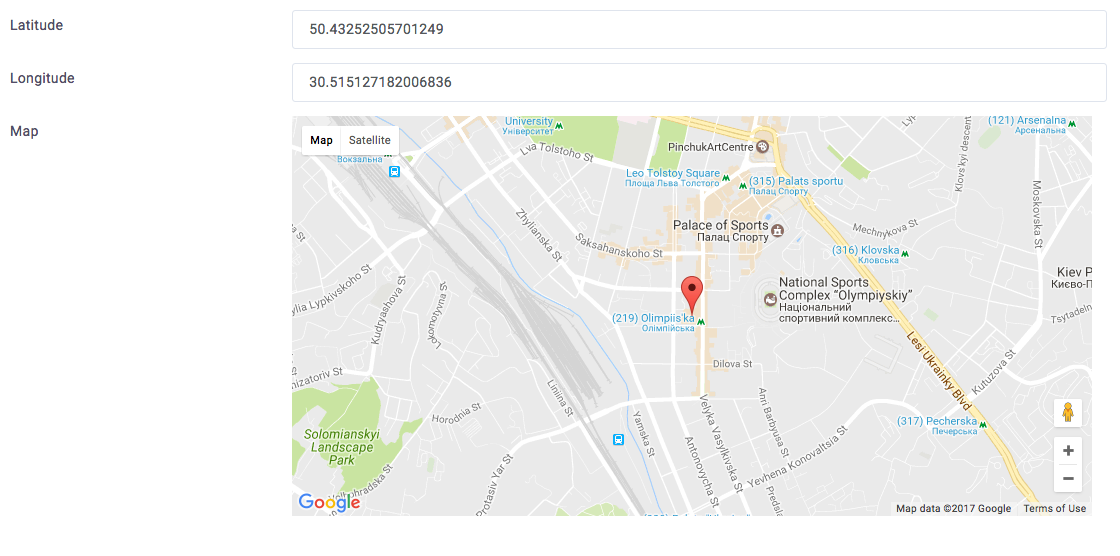
|
73
73
|
|
74
|
+
### ActiveAdmin
|
75
|
+
|
76
|
+
Formtastic integration
|
77
|
+
|
78
|
+
``` ruby
|
79
|
+
f.inputs name: 'Location', for: [:location, f.object.location || f.object.build_location] do |loc|
|
80
|
+
loc.input :latitude, input_html: { data: { map: 'lat' } }
|
81
|
+
loc.input :longitude, input_html: { data: { map: 'lng' } }
|
82
|
+
loc.input :content, input_html: { rows: 2 }
|
83
|
+
loc.input :map, as: :marker, google_api_key: 'your_google_api_key'
|
84
|
+
end
|
85
|
+
```
|
86
|
+
|
74
87
|
## Contributing
|
75
88
|
|
76
89
|
1. Fork it
|
data/Rakefile
CHANGED
@@ -1,2 +1,3 @@
|
|
1
|
-
|
2
|
-
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
require 'bundler/gem_tasks'
|
@@ -0,0 +1,15 @@
|
|
1
|
+
(function() {
|
2
|
+
var initMarkerMap;
|
3
|
+
|
4
|
+
initMarkerMap = function() {
|
5
|
+
var elements;
|
6
|
+
elements = document.querySelectorAll('[data-marker]');
|
7
|
+
return elements.forEach(function(el, index, array) {
|
8
|
+
var map;
|
9
|
+
map = new MapGoogle(el);
|
10
|
+
return $(el).data('map', map);
|
11
|
+
});
|
12
|
+
};
|
13
|
+
|
14
|
+
window['initMarkerMap'] = initMarkerMap;
|
15
|
+
}).call(this);
|
@@ -0,0 +1,146 @@
|
|
1
|
+
(function() {
|
2
|
+
var $, MapGoogle;
|
3
|
+
|
4
|
+
$ = jQuery;
|
5
|
+
|
6
|
+
MapGoogle = (function() {
|
7
|
+
function MapGoogle(dom, options) {
|
8
|
+
var defaults;
|
9
|
+
this.dom = dom;
|
10
|
+
if (options == null) {
|
11
|
+
options = {};
|
12
|
+
}
|
13
|
+
defaults = {
|
14
|
+
lat: 50.44067063154785,
|
15
|
+
lng: 30.52654266357422,
|
16
|
+
zoom: 6,
|
17
|
+
radius: 1000
|
18
|
+
};
|
19
|
+
this.element = $(this.dom);
|
20
|
+
this.options = $.extend(defaults, this.element.data(), options);
|
21
|
+
this.name = this.element.data('marker');
|
22
|
+
this.options.field_lat = "[data-" + this.name + "='lat']";
|
23
|
+
this.options.field_lng = "[data-" + this.name + "='lng']";
|
24
|
+
this.options.field_zoom = "[data-" + this.name + "='zoom']";
|
25
|
+
this.options.field_radius = "[data-" + this.name + "='radius']";
|
26
|
+
|
27
|
+
this._setup();
|
28
|
+
}
|
29
|
+
|
30
|
+
MapGoogle.prototype._setup = function() {
|
31
|
+
var number;
|
32
|
+
|
33
|
+
this.location = this._build_location();
|
34
|
+
this.map = this._build_map(this.element.get(0));
|
35
|
+
this.marker = this._build_marker();
|
36
|
+
this.field_lat = $(this.options.field_lat + ':eq(0)');
|
37
|
+
this.field_lng = $(this.options.field_lng + ':eq(0)');
|
38
|
+
this.field_zoom = $(this.options.field_zoom + ':eq(0)');
|
39
|
+
this.field_radius = $(this.options.field_radius + ':eq(0)');
|
40
|
+
|
41
|
+
google.maps.event.addListener(this.marker, 'dragend', (function(_this) {
|
42
|
+
return function(event) {
|
43
|
+
return _this._update_location(event)
|
44
|
+
};
|
45
|
+
})(this));
|
46
|
+
google.maps.event.addListener(this.map, 'zoom_changed', (function(_this) {
|
47
|
+
return function() {
|
48
|
+
return _this.field_zoom.val(_this.map.getZoom());
|
49
|
+
};
|
50
|
+
})(this));
|
51
|
+
if (this.field_radius.length > 0) {
|
52
|
+
number = this._parse_value(this.field_radius, this.options.radius);
|
53
|
+
this.circle = this._build_circle({
|
54
|
+
radius: number
|
55
|
+
});
|
56
|
+
return this.circle.bindTo('center', this.marker, 'position');
|
57
|
+
}
|
58
|
+
};
|
59
|
+
|
60
|
+
MapGoogle.prototype.placeMarker = function(location) {
|
61
|
+
var marker;
|
62
|
+
marker = this._build_marker({
|
63
|
+
position: location
|
64
|
+
});
|
65
|
+
this.map.setCenter(location);
|
66
|
+
return marker;
|
67
|
+
};
|
68
|
+
|
69
|
+
MapGoogle.prototype._build_location = function() {
|
70
|
+
var lat, lng;
|
71
|
+
lat = this._parse_value(this.options.field_lat, this.options.lat);
|
72
|
+
lng = this._parse_value(this.options.field_lng, this.options.lng);
|
73
|
+
return new google.maps.LatLng(lat, lng);
|
74
|
+
};
|
75
|
+
|
76
|
+
MapGoogle.prototype._build_map = function(element, options) {
|
77
|
+
var defaults, settings, zoom;
|
78
|
+
if (options == null) {
|
79
|
+
options = {};
|
80
|
+
}
|
81
|
+
zoom = this._parse_value(this.options.field_zoom, this.options.zoom);
|
82
|
+
defaults = {
|
83
|
+
zoom: zoom,
|
84
|
+
center: this.location,
|
85
|
+
mapTypeId: google.maps.MapTypeId.ROADMAP
|
86
|
+
};
|
87
|
+
settings = $.extend(defaults, options);
|
88
|
+
return new google.maps.Map(element, settings);
|
89
|
+
};
|
90
|
+
|
91
|
+
MapGoogle.prototype._build_marker = function(options) {
|
92
|
+
var defaults, settings;
|
93
|
+
if (options == null) {
|
94
|
+
options = {};
|
95
|
+
}
|
96
|
+
defaults = {
|
97
|
+
position: this.location,
|
98
|
+
map: this.map,
|
99
|
+
draggable: true
|
100
|
+
};
|
101
|
+
settings = $.extend(defaults, options);
|
102
|
+
return new google.maps.Marker(settings);
|
103
|
+
};
|
104
|
+
|
105
|
+
MapGoogle.prototype._build_circle = function(options) {
|
106
|
+
var defaults, settings;
|
107
|
+
if (options == null) {
|
108
|
+
options = {};
|
109
|
+
}
|
110
|
+
defaults = {
|
111
|
+
map: this.map,
|
112
|
+
strokeColor: '#FF0000',
|
113
|
+
strokeOpacity: 0.8,
|
114
|
+
strokeWeight: 2,
|
115
|
+
fillColor: '#FF0000',
|
116
|
+
fillOpacity: 0.35,
|
117
|
+
radius: 1000
|
118
|
+
};
|
119
|
+
settings = $.extend(defaults, options);
|
120
|
+
return new google.maps.Circle(settings);
|
121
|
+
};
|
122
|
+
|
123
|
+
MapGoogle.prototype._update_location = function(event) {
|
124
|
+
var pos = this.marker.getPosition();
|
125
|
+
|
126
|
+
this.field_lat.val(pos.lat());
|
127
|
+
this.field_lng.val(pos.lng());
|
128
|
+
|
129
|
+
return this.map.setCenter(event.latLng);
|
130
|
+
};
|
131
|
+
|
132
|
+
MapGoogle.prototype._parse_value = function(field, default_value) {
|
133
|
+
var value;
|
134
|
+
value = parseFloat($(field).val());
|
135
|
+
if (value) {
|
136
|
+
return value;
|
137
|
+
} else {
|
138
|
+
return parseFloat(default_value);
|
139
|
+
}
|
140
|
+
};
|
141
|
+
|
142
|
+
return MapGoogle;
|
143
|
+
})();
|
144
|
+
|
145
|
+
window.MapGoogle = MapGoogle;
|
146
|
+
}).call(this);
|
@@ -1,4 +1,4 @@
|
|
1
1
|
<%= javascript_include_tag 'marker/application' %>
|
2
|
-
<%= javascript_include_tag "https://maps.googleapis.com/maps/api/js?key=#{
|
2
|
+
<%= javascript_include_tag "https://maps.googleapis.com/maps/api/js?key=#{field.google_api_key}&callback=initMarkerMap", async: true, defer: true %>
|
3
3
|
|
4
4
|
<%= content_tag :div, nil, field.input_options %>
|
data/lib/marker/version.rb
CHANGED
metadata
CHANGED
@@ -1,16 +1,30 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: rails-marker
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.
|
4
|
+
version: 0.2.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Igor Galeta
|
8
8
|
- Pavlo Galeta
|
9
|
-
autorequire:
|
9
|
+
autorequire:
|
10
10
|
bindir: bin
|
11
11
|
cert_chain: []
|
12
|
-
date:
|
12
|
+
date: 2022-12-18 00:00:00.000000000 Z
|
13
13
|
dependencies:
|
14
|
+
- !ruby/object:Gem::Dependency
|
15
|
+
name: rake
|
16
|
+
requirement: !ruby/object:Gem::Requirement
|
17
|
+
requirements:
|
18
|
+
- - ">="
|
19
|
+
- !ruby/object:Gem::Version
|
20
|
+
version: '0'
|
21
|
+
type: :development
|
22
|
+
prerelease: false
|
23
|
+
version_requirements: !ruby/object:Gem::Requirement
|
24
|
+
requirements:
|
25
|
+
- - ">="
|
26
|
+
- !ruby/object:Gem::Version
|
27
|
+
version: '0'
|
14
28
|
- !ruby/object:Gem::Dependency
|
15
29
|
name: sqlite3
|
16
30
|
requirement: !ruby/object:Gem::Requirement
|
@@ -36,8 +50,8 @@ files:
|
|
36
50
|
- README.md
|
37
51
|
- Rakefile
|
38
52
|
- app/assets/javascripts/marker/application.js
|
39
|
-
- app/assets/javascripts/marker/init.js
|
40
|
-
- app/assets/javascripts/marker/map_google.js
|
53
|
+
- app/assets/javascripts/marker/init.js
|
54
|
+
- app/assets/javascripts/marker/map_google.js
|
41
55
|
- app/views/marker/_google.html.erb
|
42
56
|
- lib/marker.rb
|
43
57
|
- lib/marker/engine.rb
|
@@ -52,7 +66,7 @@ homepage: https://github.com/galetahub/rails-marker
|
|
52
66
|
licenses:
|
53
67
|
- MIT
|
54
68
|
metadata: {}
|
55
|
-
post_install_message:
|
69
|
+
post_install_message:
|
56
70
|
rdoc_options: []
|
57
71
|
require_paths:
|
58
72
|
- lib
|
@@ -67,9 +81,8 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
67
81
|
- !ruby/object:Gem::Version
|
68
82
|
version: '0'
|
69
83
|
requirements: []
|
70
|
-
|
71
|
-
|
72
|
-
signing_key:
|
84
|
+
rubygems_version: 3.3.7
|
85
|
+
signing_key:
|
73
86
|
specification_version: 4
|
74
87
|
summary: Easy way to edit zoom, longitude and latitude
|
75
88
|
test_files: []
|
@@ -1,108 +0,0 @@
|
|
1
|
-
$ = jQuery
|
2
|
-
|
3
|
-
class MapGoogle
|
4
|
-
constructor: (@dom, options = {}) ->
|
5
|
-
defaults =
|
6
|
-
lat: 50.44067063154785
|
7
|
-
lng: 30.52654266357422
|
8
|
-
zoom: 6
|
9
|
-
radius: 1000
|
10
|
-
|
11
|
-
@element = $(@dom)
|
12
|
-
@options = $.extend defaults, @element.data(), options
|
13
|
-
|
14
|
-
@name = @element.data('marker')
|
15
|
-
|
16
|
-
@options.field_lat = "[data-#{@name}='lat']"
|
17
|
-
@options.field_lng = "[data-#{@name}='lng']"
|
18
|
-
@options.field_zoom = "[data-#{@name}='zoom']"
|
19
|
-
@options.field_radius = "[data-#{@name}='radius']"
|
20
|
-
|
21
|
-
this._setup()
|
22
|
-
|
23
|
-
_setup: ->
|
24
|
-
@location = this._build_location()
|
25
|
-
@map = this._build_map(@element.get(0))
|
26
|
-
@marker = this._build_marker()
|
27
|
-
|
28
|
-
@field_lat = $(@options.field_lat + ':eq(0)')
|
29
|
-
@field_lng = $(@options.field_lng + ':eq(0)')
|
30
|
-
@field_zoom = $(@options.field_zoom + ':eq(0)')
|
31
|
-
@field_radius = $(@options.field_radius + ':eq(0)')
|
32
|
-
|
33
|
-
google.maps.event.addListener(@marker, 'dragend', (event) =>
|
34
|
-
pos = @marker.getPosition()
|
35
|
-
|
36
|
-
@field_lat.val pos.lat()
|
37
|
-
@field_lng.val pos.lng()
|
38
|
-
|
39
|
-
@map.setCenter(event.latLng)
|
40
|
-
)
|
41
|
-
|
42
|
-
google.maps.event.addListener(@map, 'zoom_changed', () =>
|
43
|
-
@field_zoom.val @map.getZoom()
|
44
|
-
)
|
45
|
-
|
46
|
-
if @field_radius.length > 0
|
47
|
-
number = this._parse_value(@field_radius, @options.radius)
|
48
|
-
@circle = this._build_circle(radius: number)
|
49
|
-
@circle.bindTo('center', @marker, 'position')
|
50
|
-
|
51
|
-
placeMarker: (location) ->
|
52
|
-
marker = this._build_marker {position: location}
|
53
|
-
|
54
|
-
@map.setCenter(location);
|
55
|
-
|
56
|
-
return marker
|
57
|
-
|
58
|
-
_build_location: ->
|
59
|
-
lat = this._parse_value @options.field_lat, @options.lat
|
60
|
-
lng = this._parse_value @options.field_lng, @options.lng
|
61
|
-
|
62
|
-
new google.maps.LatLng(lat, lng)
|
63
|
-
|
64
|
-
_build_map: (element, options = {}) ->
|
65
|
-
zoom = this._parse_value @options.field_zoom, @options.zoom
|
66
|
-
|
67
|
-
defaults =
|
68
|
-
zoom: zoom
|
69
|
-
center: @location
|
70
|
-
mapTypeId: google.maps.MapTypeId.ROADMAP
|
71
|
-
|
72
|
-
settings = $.extend defaults, options
|
73
|
-
|
74
|
-
new google.maps.Map(element, settings)
|
75
|
-
|
76
|
-
_build_marker: (options = {}) ->
|
77
|
-
defaults =
|
78
|
-
position: @location
|
79
|
-
map: @map
|
80
|
-
draggable: true
|
81
|
-
|
82
|
-
settings = $.extend defaults, options
|
83
|
-
|
84
|
-
new google.maps.Marker(settings)
|
85
|
-
|
86
|
-
_build_circle: (options = {}) ->
|
87
|
-
defaults =
|
88
|
-
map: @map
|
89
|
-
strokeColor: '#FF0000',
|
90
|
-
strokeOpacity: 0.8,
|
91
|
-
strokeWeight: 2,
|
92
|
-
fillColor: '#FF0000',
|
93
|
-
fillOpacity: 0.35,
|
94
|
-
radius: 1000 # 1 kilometr
|
95
|
-
|
96
|
-
settings = $.extend defaults, options
|
97
|
-
|
98
|
-
new google.maps.Circle(settings)
|
99
|
-
|
100
|
-
_parse_value: (field, default_value) ->
|
101
|
-
value = parseFloat $(field).val()
|
102
|
-
|
103
|
-
if value
|
104
|
-
return value
|
105
|
-
else
|
106
|
-
return parseFloat(default_value)
|
107
|
-
|
108
|
-
window.MapGoogle = MapGoogle
|