raes 0.1.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +7 -0
- data/MIT-LICENSE +20 -0
- data/README.md +97 -0
- data/Rakefile +24 -0
- data/app/assets/config/raes_manifest.js +1 -0
- data/app/assets/stylesheets/raes/application.css +15 -0
- data/app/controllers/raes/application_controller.rb +7 -0
- data/app/helpers/async_event_store/application_helper.rb +6 -0
- data/app/jobs/raes/application_job.rb +6 -0
- data/app/jobs/raes/subscribe_job.rb +9 -0
- data/app/mailers/raes/application_mailer.rb +8 -0
- data/app/models/raes/action.rb +9 -0
- data/app/models/raes/application_record.rb +7 -0
- data/app/models/raes/storage.rb +10 -0
- data/app/views/layouts/raes/application.html.erb +15 -0
- data/config/routes.rb +4 -0
- data/db/migrate/20200628202001_create_raes_actions.rb +15 -0
- data/db/migrate/20200628202002_create_raes_storages.rb +14 -0
- data/lib/raes.rb +17 -0
- data/lib/raes/dispatch.rb +27 -0
- data/lib/raes/engine.rb +18 -0
- data/lib/raes/reducer.rb +33 -0
- data/lib/raes/use_caller.rb +29 -0
- data/lib/raes/use_storage.rb +21 -0
- data/lib/raes/version.rb +5 -0
- data/lib/tasks/raes_tasks.rake +5 -0
- metadata +131 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: b6043ba9c36590180a011fbf26a25f7c84e20c6f9817bc580b7c0f50fd9b1e44
|
4
|
+
data.tar.gz: 259f6da4d4546da83eb11a874e2ce08d5db951065e372b22270d0e3ae6923386
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 0e7e932f79f460e372e505f86e39446736536050d7dd9e637815e945147f42eab26866321c39701143b5ad8e0959421dac6b2ed32f340696eeef54652ebb517d
|
7
|
+
data.tar.gz: f2568c2639857b90b49df2a52e3f1e557126de3ab256bc9e923b9c3867fba0b396966d603b2600791f1788e57f2ef277e149802ad220d10ea82d6e6773ae9b70
|
data/MIT-LICENSE
ADDED
@@ -0,0 +1,20 @@
|
|
1
|
+
Copyright 2020 ogom
|
2
|
+
|
3
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
4
|
+
a copy of this software and associated documentation files (the
|
5
|
+
"Software"), to deal in the Software without restriction, including
|
6
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
7
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
8
|
+
permit persons to whom the Software is furnished to do so, subject to
|
9
|
+
the following conditions:
|
10
|
+
|
11
|
+
The above copyright notice and this permission notice shall be
|
12
|
+
included in all copies or substantial portions of the Software.
|
13
|
+
|
14
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
15
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
16
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
17
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
18
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
19
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
20
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,97 @@
|
|
1
|
+
# Raes
|
2
|
+
|
3
|
+
Raes is Rails Async Event Store. Executes a class method call asynchronously.
|
4
|
+
Others use Rails' [Active Job](https://edgeguides.rubyonrails.org/active_job_basics.html) and [Active Storage](https://edgeguides.rubyonrails.org/active_storage_overview.html) services to store files.
|
5
|
+
|
6
|
+
- Raes (※)
|
7
|
+
|
8
|
+
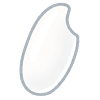
|
9
|
+
|
10
|
+
## Usage
|
11
|
+
|
12
|
+
Dispatch a class name that has a call method.
|
13
|
+
|
14
|
+
### Simple Echo
|
15
|
+
|
16
|
+
Same echo call method.
|
17
|
+
|
18
|
+
```ruby
|
19
|
+
class SimpleEcho
|
20
|
+
include Raes::UseCaller
|
21
|
+
|
22
|
+
def initialize(params)
|
23
|
+
@params = params
|
24
|
+
end
|
25
|
+
|
26
|
+
def call
|
27
|
+
@params
|
28
|
+
end
|
29
|
+
end
|
30
|
+
```
|
31
|
+
|
32
|
+
When executed, `{ message:'Hi' }` is saved in state.
|
33
|
+
|
34
|
+
```ruby
|
35
|
+
Raes.dispatch(SimpleEcho, { message: 'Hi' })
|
36
|
+
Raes.search(SimpleEcho).last.state # "{\"message\":\"Hi\"}"
|
37
|
+
```
|
38
|
+
|
39
|
+
### File Maker
|
40
|
+
|
41
|
+
Rewrite old file newly.
|
42
|
+
|
43
|
+
```ruby
|
44
|
+
class FileMaker
|
45
|
+
include Raes::UseCaller
|
46
|
+
include Raes::UseStorage
|
47
|
+
|
48
|
+
def initialize(params)
|
49
|
+
@raes_storage_id = params[:raes_storage_id]
|
50
|
+
end
|
51
|
+
|
52
|
+
def call
|
53
|
+
old_file = raes_storage_content
|
54
|
+
new_file = old_file + 'bar'
|
55
|
+
|
56
|
+
raes_storage_attach({ io: StringIO.new(new_file), filename: 'new_file.txt', content_type: 'text/plain' })
|
57
|
+
end
|
58
|
+
end
|
59
|
+
```
|
60
|
+
|
61
|
+
Content will be changed to the new file.
|
62
|
+
|
63
|
+
```ruby
|
64
|
+
Raes.dispatch(FileMaker, raes_storage: { io: StringIO.new('foo'), filename: 'old_file.txt', content_type: 'text/plain' })
|
65
|
+
Raes.search(FileMaker).last.storages.first.content.download # foobar
|
66
|
+
```
|
67
|
+
|
68
|
+
## Installation
|
69
|
+
|
70
|
+
Add this line to your application's Gemfile:
|
71
|
+
|
72
|
+
```ruby
|
73
|
+
gem 'raes'
|
74
|
+
```
|
75
|
+
|
76
|
+
And then execute:
|
77
|
+
|
78
|
+
```bash
|
79
|
+
$ bundle
|
80
|
+
$ rails raes:install:migrations
|
81
|
+
```
|
82
|
+
|
83
|
+
If use storage, then execute:
|
84
|
+
|
85
|
+
```bash
|
86
|
+
$ rails active_storage:install
|
87
|
+
```
|
88
|
+
|
89
|
+
## Test
|
90
|
+
|
91
|
+
```bash
|
92
|
+
$ rails app:spec
|
93
|
+
```
|
94
|
+
|
95
|
+
## License
|
96
|
+
|
97
|
+
The gem is available as open source under the terms of the [MIT License](https://opensource.org/licenses/MIT).
|
data/Rakefile
ADDED
@@ -0,0 +1,24 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
begin
|
4
|
+
require 'bundler/setup'
|
5
|
+
rescue LoadError
|
6
|
+
puts 'You must `gem install bundler` and `bundle install` to run rake tasks'
|
7
|
+
end
|
8
|
+
|
9
|
+
require 'rdoc/task'
|
10
|
+
|
11
|
+
RDoc::Task.new(:rdoc) do |rdoc|
|
12
|
+
rdoc.rdoc_dir = 'rdoc'
|
13
|
+
rdoc.title = 'Raes'
|
14
|
+
rdoc.options << '--line-numbers'
|
15
|
+
rdoc.rdoc_files.include('README.md')
|
16
|
+
rdoc.rdoc_files.include('lib/**/*.rb')
|
17
|
+
end
|
18
|
+
|
19
|
+
APP_RAKEFILE = File.expand_path('spec/dummy/Rakefile', __dir__)
|
20
|
+
load 'rails/tasks/engine.rake'
|
21
|
+
|
22
|
+
load 'rails/tasks/statistics.rake'
|
23
|
+
|
24
|
+
require 'bundler/gem_tasks'
|
@@ -0,0 +1 @@
|
|
1
|
+
//= link_directory ../stylesheets/raes .css
|
@@ -0,0 +1,15 @@
|
|
1
|
+
/*
|
2
|
+
* This is a manifest file that'll be compiled into application.css, which will include all the files
|
3
|
+
* listed below.
|
4
|
+
*
|
5
|
+
* Any CSS and SCSS file within this directory, lib/assets/stylesheets, vendor/assets/stylesheets,
|
6
|
+
* or any plugin's vendor/assets/stylesheets directory can be referenced here using a relative path.
|
7
|
+
*
|
8
|
+
* You're free to add application-wide styles to this file and they'll appear at the bottom of the
|
9
|
+
* compiled file so the styles you add here take precedence over styles defined in any other CSS/SCSS
|
10
|
+
* files in this directory. Styles in this file should be added after the last require_* statement.
|
11
|
+
* It is generally better to create a new file per style scope.
|
12
|
+
*
|
13
|
+
*= require_tree .
|
14
|
+
*= require_self
|
15
|
+
*/
|
data/config/routes.rb
ADDED
@@ -0,0 +1,15 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
class CreateRaesActions < ActiveRecord::Migration[6.0]
|
4
|
+
def change
|
5
|
+
create_table :raes_actions do |t|
|
6
|
+
t.integer :status, null: false, default: 0, index: true
|
7
|
+
t.string :name, null: false, default: '', index: true
|
8
|
+
t.json :payload, null: false, default: {}
|
9
|
+
t.json :state, null: false, default: {}
|
10
|
+
|
11
|
+
t.timestamps
|
12
|
+
end
|
13
|
+
add_index :raes_actions, %i[status name]
|
14
|
+
end
|
15
|
+
end
|
@@ -0,0 +1,14 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
class CreateRaesStorages < ActiveRecord::Migration[6.0]
|
4
|
+
def change
|
5
|
+
create_table :raes_storages do |t|
|
6
|
+
t.integer :status, null: false, default: 0, index: true
|
7
|
+
t.string :name, null: false, default: '', index: true
|
8
|
+
t.references :record, null: false, polymorphic: true
|
9
|
+
|
10
|
+
t.timestamps
|
11
|
+
end
|
12
|
+
add_index :raes_storages, %i[status name]
|
13
|
+
end
|
14
|
+
end
|
data/lib/raes.rb
ADDED
@@ -0,0 +1,17 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
require 'raes/engine'
|
4
|
+
require 'raes/use_caller'
|
5
|
+
require 'raes/use_storage'
|
6
|
+
require 'raes/dispatch'
|
7
|
+
require 'raes/reducer'
|
8
|
+
|
9
|
+
module Raes
|
10
|
+
def self.dispatch(name, payload = {})
|
11
|
+
Dispatch.call(name, payload)
|
12
|
+
end
|
13
|
+
|
14
|
+
def self.search(name)
|
15
|
+
Action.search(name)
|
16
|
+
end
|
17
|
+
end
|
@@ -0,0 +1,27 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Raes
|
4
|
+
class Dispatch
|
5
|
+
include UseCaller
|
6
|
+
|
7
|
+
def initialize(name, payload = {})
|
8
|
+
@action = Action.create(name: name)
|
9
|
+
@payload = payload
|
10
|
+
end
|
11
|
+
|
12
|
+
def call
|
13
|
+
@action.payload = create_payload.to_json
|
14
|
+
SubscribeJob.perform_later(@action) if @action.save
|
15
|
+
@action
|
16
|
+
end
|
17
|
+
|
18
|
+
def create_payload
|
19
|
+
if @payload[:raes_storage].present?
|
20
|
+
storage = @action.storages.create(name: @action.name, content: @payload[:raes_storage])
|
21
|
+
@payload.delete(:raes_storage)
|
22
|
+
@payload[:raes_storage_id] = storage.id
|
23
|
+
end
|
24
|
+
@payload
|
25
|
+
end
|
26
|
+
end
|
27
|
+
end
|
data/lib/raes/engine.rb
ADDED
@@ -0,0 +1,18 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Raes
|
4
|
+
class Engine < ::Rails::Engine
|
5
|
+
isolate_namespace Raes
|
6
|
+
|
7
|
+
config.generators do |g|
|
8
|
+
g.test_framework :rspec,
|
9
|
+
controller_specs: false,
|
10
|
+
helper_specs: false,
|
11
|
+
request_specs: false,
|
12
|
+
routing_specs: false,
|
13
|
+
view_specs: false
|
14
|
+
g.fixture_replacement :factory_bot
|
15
|
+
g.factory_bot dir: 'spec/factories'
|
16
|
+
end
|
17
|
+
end
|
18
|
+
end
|
data/lib/raes/reducer.rb
ADDED
@@ -0,0 +1,33 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Raes
|
4
|
+
class Reducer
|
5
|
+
include UseCaller
|
6
|
+
|
7
|
+
def initialize(action)
|
8
|
+
@action = action
|
9
|
+
end
|
10
|
+
|
11
|
+
def call
|
12
|
+
return @action unless @action.queued!
|
13
|
+
|
14
|
+
@action.working!
|
15
|
+
@action.state = create_state.to_json
|
16
|
+
@action.status = :completed
|
17
|
+
rescue StandardError => e
|
18
|
+
@action.state = { message: e }
|
19
|
+
@action.status = :failed
|
20
|
+
ensure
|
21
|
+
@action.save!
|
22
|
+
@action
|
23
|
+
end
|
24
|
+
|
25
|
+
def create_state
|
26
|
+
if @action.name.constantize.method(:call).arity.zero?
|
27
|
+
@action.name.constantize.call
|
28
|
+
else
|
29
|
+
@action.name.constantize.call(JSON.parse(@action.payload).symbolize_keys)
|
30
|
+
end
|
31
|
+
end
|
32
|
+
end
|
33
|
+
end
|
@@ -0,0 +1,29 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Raes
|
4
|
+
module UseCaller
|
5
|
+
extend ActiveSupport::Concern
|
6
|
+
|
7
|
+
included do
|
8
|
+
private_class_method :new
|
9
|
+
end
|
10
|
+
|
11
|
+
class_methods do
|
12
|
+
def call(*params)
|
13
|
+
new(*params).send(:call)
|
14
|
+
end
|
15
|
+
end
|
16
|
+
|
17
|
+
private
|
18
|
+
|
19
|
+
def initialize(*params)
|
20
|
+
return if params.empty?
|
21
|
+
|
22
|
+
raise NotImplementedError, "You must implement #{self.class}##{__method__}"
|
23
|
+
end
|
24
|
+
|
25
|
+
def call
|
26
|
+
raise NotImplementedError, "You must implement #{self.class}##{__method__}"
|
27
|
+
end
|
28
|
+
end
|
29
|
+
end
|
@@ -0,0 +1,21 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
module Raes
|
4
|
+
module UseStorage
|
5
|
+
extend ActiveSupport::Concern
|
6
|
+
|
7
|
+
included do
|
8
|
+
attr_accessor :raes_storage_id
|
9
|
+
end
|
10
|
+
|
11
|
+
private
|
12
|
+
|
13
|
+
def raes_storage_content
|
14
|
+
Storage.find(raes_storage_id).content.download
|
15
|
+
end
|
16
|
+
|
17
|
+
def raes_storage_attach(content)
|
18
|
+
Storage.find(raes_storage_id).content.attach(content)
|
19
|
+
end
|
20
|
+
end
|
21
|
+
end
|
data/lib/raes/version.rb
ADDED
metadata
ADDED
@@ -0,0 +1,131 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: raes
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.1.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- ogom
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2020-06-30 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: rails
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - "~>"
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: 6.0.3
|
20
|
+
- - ">="
|
21
|
+
- !ruby/object:Gem::Version
|
22
|
+
version: 6.0.3.2
|
23
|
+
type: :runtime
|
24
|
+
prerelease: false
|
25
|
+
version_requirements: !ruby/object:Gem::Requirement
|
26
|
+
requirements:
|
27
|
+
- - "~>"
|
28
|
+
- !ruby/object:Gem::Version
|
29
|
+
version: 6.0.3
|
30
|
+
- - ">="
|
31
|
+
- !ruby/object:Gem::Version
|
32
|
+
version: 6.0.3.2
|
33
|
+
- !ruby/object:Gem::Dependency
|
34
|
+
name: factory_bot_rails
|
35
|
+
requirement: !ruby/object:Gem::Requirement
|
36
|
+
requirements:
|
37
|
+
- - ">="
|
38
|
+
- !ruby/object:Gem::Version
|
39
|
+
version: '0'
|
40
|
+
type: :development
|
41
|
+
prerelease: false
|
42
|
+
version_requirements: !ruby/object:Gem::Requirement
|
43
|
+
requirements:
|
44
|
+
- - ">="
|
45
|
+
- !ruby/object:Gem::Version
|
46
|
+
version: '0'
|
47
|
+
- !ruby/object:Gem::Dependency
|
48
|
+
name: rspec-rails
|
49
|
+
requirement: !ruby/object:Gem::Requirement
|
50
|
+
requirements:
|
51
|
+
- - ">="
|
52
|
+
- !ruby/object:Gem::Version
|
53
|
+
version: '0'
|
54
|
+
type: :development
|
55
|
+
prerelease: false
|
56
|
+
version_requirements: !ruby/object:Gem::Requirement
|
57
|
+
requirements:
|
58
|
+
- - ">="
|
59
|
+
- !ruby/object:Gem::Version
|
60
|
+
version: '0'
|
61
|
+
- !ruby/object:Gem::Dependency
|
62
|
+
name: sqlite3
|
63
|
+
requirement: !ruby/object:Gem::Requirement
|
64
|
+
requirements:
|
65
|
+
- - ">="
|
66
|
+
- !ruby/object:Gem::Version
|
67
|
+
version: '0'
|
68
|
+
type: :development
|
69
|
+
prerelease: false
|
70
|
+
version_requirements: !ruby/object:Gem::Requirement
|
71
|
+
requirements:
|
72
|
+
- - ">="
|
73
|
+
- !ruby/object:Gem::Version
|
74
|
+
version: '0'
|
75
|
+
description: Description of Rails Async Event Store.
|
76
|
+
email:
|
77
|
+
- ogom@outlook.com
|
78
|
+
executables: []
|
79
|
+
extensions: []
|
80
|
+
extra_rdoc_files: []
|
81
|
+
files:
|
82
|
+
- MIT-LICENSE
|
83
|
+
- README.md
|
84
|
+
- Rakefile
|
85
|
+
- app/assets/config/raes_manifest.js
|
86
|
+
- app/assets/stylesheets/raes/application.css
|
87
|
+
- app/controllers/raes/application_controller.rb
|
88
|
+
- app/helpers/async_event_store/application_helper.rb
|
89
|
+
- app/jobs/raes/application_job.rb
|
90
|
+
- app/jobs/raes/subscribe_job.rb
|
91
|
+
- app/mailers/raes/application_mailer.rb
|
92
|
+
- app/models/raes/action.rb
|
93
|
+
- app/models/raes/application_record.rb
|
94
|
+
- app/models/raes/storage.rb
|
95
|
+
- app/views/layouts/raes/application.html.erb
|
96
|
+
- config/routes.rb
|
97
|
+
- db/migrate/20200628202001_create_raes_actions.rb
|
98
|
+
- db/migrate/20200628202002_create_raes_storages.rb
|
99
|
+
- lib/raes.rb
|
100
|
+
- lib/raes/dispatch.rb
|
101
|
+
- lib/raes/engine.rb
|
102
|
+
- lib/raes/reducer.rb
|
103
|
+
- lib/raes/use_caller.rb
|
104
|
+
- lib/raes/use_storage.rb
|
105
|
+
- lib/raes/version.rb
|
106
|
+
- lib/tasks/raes_tasks.rake
|
107
|
+
homepage: https://github.com/ogom/raes
|
108
|
+
licenses:
|
109
|
+
- MIT
|
110
|
+
metadata:
|
111
|
+
allowed_push_host: https://rubygems.org/
|
112
|
+
post_install_message:
|
113
|
+
rdoc_options: []
|
114
|
+
require_paths:
|
115
|
+
- lib
|
116
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
117
|
+
requirements:
|
118
|
+
- - ">="
|
119
|
+
- !ruby/object:Gem::Version
|
120
|
+
version: '0'
|
121
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
122
|
+
requirements:
|
123
|
+
- - ">="
|
124
|
+
- !ruby/object:Gem::Version
|
125
|
+
version: '0'
|
126
|
+
requirements: []
|
127
|
+
rubygems_version: 3.0.3
|
128
|
+
signing_key:
|
129
|
+
specification_version: 4
|
130
|
+
summary: Summary of Rails Async Event Store.
|
131
|
+
test_files: []
|