r5_fog 1.10.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/README.md +156 -0
- data/bin/fog +56 -0
- metadata +315 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: 1d75badb99d17d8b7f16c1fa17462ed82a7fed5f
|
4
|
+
data.tar.gz: 90adce36eda9fe60bd7569a05a65005ab62e79ae
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 6e163ce6009be3051bf4a7bd6a974c39affe5d0ab11d8ddfbd540c7df937c59a1bc721756fd37423ae02161396641de7896aafb684d829ceaf2a247e320cd16a
|
7
|
+
data.tar.gz: a1bc0f366f947bc3c31f17ea0264fb9e4a639761ac6f084d2623b18798a12d01ba044521f0faadf1415da65b39e85a804daa27201e34b420e33f07022d8460ce
|
data/README.md
ADDED
@@ -0,0 +1,156 @@
|
|
1
|
+
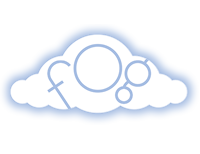
|
2
|
+
|
3
|
+
fog is the Ruby cloud services library, top to bottom:
|
4
|
+
|
5
|
+
* Collections provide a simplified interface, making clouds easier to work with and switch between.
|
6
|
+
* Requests allow power users to get the most out of the features of each individual cloud.
|
7
|
+
* Mocks make testing and integrating a breeze.
|
8
|
+
|
9
|
+
[](http://travis-ci.org/fog/fog)
|
10
|
+
[](http://badge.fury.io/rb/fog)
|
11
|
+
[](https://gemnasium.com/fog/fog)
|
12
|
+
[](https://codeclimate.com/github/fog/fog)
|
13
|
+
|
14
|
+
## Getting Started
|
15
|
+
|
16
|
+
sudo gem install fog
|
17
|
+
|
18
|
+
Now type `fog` to try stuff, confident that fog will let you know what to do.
|
19
|
+
Here is an example of wading through server creation for Amazon Elastic Compute Cloud:
|
20
|
+
|
21
|
+
>> server = Compute[:aws].servers.create
|
22
|
+
ArgumentError: image_id is required for this operation
|
23
|
+
|
24
|
+
>> server = Compute[:aws].servers.create(:image_id => 'ami-5ee70037')
|
25
|
+
<Fog::AWS::EC2::Server [...]>
|
26
|
+
|
27
|
+
>> server.destroy # cleanup after yourself or regret it, trust me
|
28
|
+
true
|
29
|
+
|
30
|
+
## Collections
|
31
|
+
|
32
|
+
A high level interface to each cloud is provided through collections, such as `images` and `servers`.
|
33
|
+
You can see a list of available collections by calling `collections` on the connection object.
|
34
|
+
You can try it out using the `fog` command:
|
35
|
+
|
36
|
+
>> Compute[:aws].collections
|
37
|
+
[:addresses, :directories, ..., :volumes, :zones]
|
38
|
+
|
39
|
+
Some collections are available across multiple providers:
|
40
|
+
|
41
|
+
* compute providers have `flavors`, `images` and `servers`
|
42
|
+
* dns providers have `zones` and `records`
|
43
|
+
* storage providers have `directories` and `files`
|
44
|
+
|
45
|
+
Collections share basic CRUD type operations, such as:
|
46
|
+
|
47
|
+
* `all` - fetch every object of that type from the provider.
|
48
|
+
* `create` - initialize a new record locally and a remote resource with the provider.
|
49
|
+
* `get` - fetch a single object by it's identity from the provider.
|
50
|
+
* `new` - initialize a new record locally, but do not create a remote resource with the provider.
|
51
|
+
|
52
|
+
As an example, we'll try initializing and persisting a Rackspace Cloud server:
|
53
|
+
|
54
|
+
require 'fog'
|
55
|
+
|
56
|
+
compute = Fog::Compute.new(
|
57
|
+
:provider => 'Rackspace',
|
58
|
+
:rackspace_api_key => key,
|
59
|
+
:rackspace_username => username
|
60
|
+
)
|
61
|
+
|
62
|
+
# boot a gentoo server (flavor 1 = 256, image 3 = gentoo 2008.0)
|
63
|
+
server = compute.servers.create(:flavor_id => 1, :image_id => 3, :name => 'my_server')
|
64
|
+
server.wait_for { ready? } # give server time to boot
|
65
|
+
|
66
|
+
# DO STUFF
|
67
|
+
|
68
|
+
server.destroy # cleanup after yourself or regret it, trust me
|
69
|
+
|
70
|
+
## Models
|
71
|
+
|
72
|
+
Many of the collection methods return individual objects, which also provide common methods:
|
73
|
+
|
74
|
+
* `destroy` - will destroy the persisted object from the provider
|
75
|
+
* `save` - persist the object to the provider
|
76
|
+
* `wait_for` - takes a block and waits for either the block to return true for the object or for a timeout (defaults to 10 minutes)
|
77
|
+
|
78
|
+
## Mocks
|
79
|
+
|
80
|
+
As you might imagine, testing code using Fog can be slow and expensive, constantly turning on and and shutting down instances.
|
81
|
+
Mocking allows skipping this overhead by providing an in memory representation resources as you make requests.
|
82
|
+
Enabling mocking easy to use, before you run other commands, simply run:
|
83
|
+
|
84
|
+
Fog.mock!
|
85
|
+
|
86
|
+
Then proceed as usual, if you run into unimplemented mocks, fog will raise an error and as always contributions are welcome!
|
87
|
+
|
88
|
+
## Requests
|
89
|
+
|
90
|
+
Requests allow you to dive deeper when the models just can't cut it.
|
91
|
+
You can see a list of available requests by calling `#requests` on the connection object.
|
92
|
+
|
93
|
+
For instance, ec2 provides methods related to reserved instances that don't have any models (yet). Here is how you can lookup your reserved instances:
|
94
|
+
|
95
|
+
$ fog
|
96
|
+
>> Compute[:aws].describe_reserved_instances
|
97
|
+
#<Excon::Response [...]>
|
98
|
+
|
99
|
+
It will return an [excon](http://github.com/geemus/excon) response, which has `body`, `headers` and `status`. Both return nice hashes.
|
100
|
+
|
101
|
+
## Go forth and conquer
|
102
|
+
|
103
|
+
Play around and use the console to explore or check out [fog.io](http://fog.io) for more details and examples.
|
104
|
+
Once you are ready to start scripting fog, here is a quick hint on how to make connections without the command line thing to help you.
|
105
|
+
|
106
|
+
# create a compute connection
|
107
|
+
compute = Fog::Compute.new(:provider => 'AWS', :aws_access_key_id => ACCESS_KEY_ID, :aws_secret_access_key => SECRET_ACCESS_KEY)
|
108
|
+
# compute operations go here
|
109
|
+
|
110
|
+
# create a storage connection
|
111
|
+
storage = Fog::Storage.new(:provider => 'AWS', :aws_access_key_id => ACCESS_KEY_ID, :aws_secret_access_key => SECRET_ACCESS_KEY)
|
112
|
+
# storage operations go here
|
113
|
+
|
114
|
+
geemus says: "That should give you everything you need to get started, but let me know if there is anything I can do to help!"
|
115
|
+
|
116
|
+
## Contributing
|
117
|
+
|
118
|
+
* Find something you would like to work on.
|
119
|
+
* Look for anything you can help with in the [issue tracker](https://github.com/fog/fog/issues).
|
120
|
+
* Look at the [code quality metrics](https://codeclimate.com/github/fog/fog) for anything you can help clean up.
|
121
|
+
* Or anything else!
|
122
|
+
* Fork the project and do your work in a topic branch.
|
123
|
+
* Make sure your changes will work on both Ruby 1.8.7 and Ruby 1.9
|
124
|
+
* Add a config at `tests/.fog` for the component you want to test.
|
125
|
+
* Add shindo tests to prove your code works and run all the tests using `bundle exec rake`.
|
126
|
+
* Rebase your branch against `fog/fog` to make sure everything is up to date.
|
127
|
+
* Commit your changes and send a pull request.
|
128
|
+
|
129
|
+
## Additional Resources
|
130
|
+
|
131
|
+
[fog.io](http://fog.io)
|
132
|
+
|
133
|
+
## Copyright
|
134
|
+
|
135
|
+
(The MIT License)
|
136
|
+
|
137
|
+
Copyright (c) 2013 [geemus (Wesley Beary)](http://github.com/geemus)
|
138
|
+
|
139
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
140
|
+
a copy of this software and associated documentation files (the
|
141
|
+
"Software"), to deal in the Software without restriction, including
|
142
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
143
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
144
|
+
permit persons to whom the Software is furnished to do so, subject to
|
145
|
+
the following conditions:
|
146
|
+
|
147
|
+
The above copyright notice and this permission notice shall be
|
148
|
+
included in all copies or substantial portions of the Software.
|
149
|
+
|
150
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
151
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
152
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
153
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
154
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
155
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
156
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/bin/fog
ADDED
@@ -0,0 +1,56 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
require File.expand_path(File.join(File.dirname(__FILE__), '..', 'lib', 'fog'))
|
3
|
+
require 'irb'
|
4
|
+
require 'yaml'
|
5
|
+
Fog.credential = ARGV.first ? ARGV.first.to_sym : nil
|
6
|
+
Fog.mock! if ENV['FOG_MOCK']
|
7
|
+
if Fog.credentials.empty?
|
8
|
+
begin
|
9
|
+
Fog::Errors.missing_credentials
|
10
|
+
rescue Fog::Errors::LoadError => error
|
11
|
+
abort error.message
|
12
|
+
end
|
13
|
+
end
|
14
|
+
|
15
|
+
require 'fog/bin'
|
16
|
+
|
17
|
+
providers = Fog.available_providers
|
18
|
+
providers = if providers.length > 1
|
19
|
+
providers[0...-1].join(', ') << ' and ' << providers[-1]
|
20
|
+
else
|
21
|
+
providers.first
|
22
|
+
end
|
23
|
+
|
24
|
+
if ARGV.length > 1
|
25
|
+
|
26
|
+
result = instance_eval(ARGV[1..-1].join(' '))
|
27
|
+
puts(Fog::JSON.encode(result))
|
28
|
+
|
29
|
+
else
|
30
|
+
|
31
|
+
ARGV.clear # Avoid passing args to IRB
|
32
|
+
IRB.setup(nil)
|
33
|
+
@irb = IRB::Irb.new(nil)
|
34
|
+
IRB.conf[:MAIN_CONTEXT] = @irb.context
|
35
|
+
IRB.conf[:PROMPT][:FOG] = IRB.conf[:PROMPT][:SIMPLE].dup
|
36
|
+
IRB.conf[:PROMPT][:FOG][:RETURN] = "%s\n"
|
37
|
+
@irb.context.prompt_mode = :FOG
|
38
|
+
@irb.context.workspace = IRB::WorkSpace.new(binding)
|
39
|
+
|
40
|
+
trap 'INT' do
|
41
|
+
@irb.signal_handle
|
42
|
+
end
|
43
|
+
|
44
|
+
Formatador.display_line('Welcome to fog interactive!')
|
45
|
+
Formatador.display_line(":#{Fog.credential} provides #{providers}")
|
46
|
+
providers = Fog.providers
|
47
|
+
|
48
|
+
# FIXME: hacks until we can `include Fog` in bin
|
49
|
+
CDN = Fog::CDN
|
50
|
+
Compute = Fog::Compute
|
51
|
+
DNS = Fog::DNS
|
52
|
+
Storage = Fog::Storage
|
53
|
+
|
54
|
+
catch(:IRB_EXIT) { @irb.eval_input }
|
55
|
+
|
56
|
+
end
|
metadata
ADDED
@@ -0,0 +1,315 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: r5_fog
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 1.10.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- geemus (Wesley Beary)
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2013-03-05 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: builder
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - ">="
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '0'
|
20
|
+
type: :runtime
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - ">="
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '0'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: excon
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - "~>"
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '0.14'
|
34
|
+
type: :runtime
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - "~>"
|
39
|
+
- !ruby/object:Gem::Version
|
40
|
+
version: '0.14'
|
41
|
+
- !ruby/object:Gem::Dependency
|
42
|
+
name: formatador
|
43
|
+
requirement: !ruby/object:Gem::Requirement
|
44
|
+
requirements:
|
45
|
+
- - "~>"
|
46
|
+
- !ruby/object:Gem::Version
|
47
|
+
version: 0.2.0
|
48
|
+
type: :runtime
|
49
|
+
prerelease: false
|
50
|
+
version_requirements: !ruby/object:Gem::Requirement
|
51
|
+
requirements:
|
52
|
+
- - "~>"
|
53
|
+
- !ruby/object:Gem::Version
|
54
|
+
version: 0.2.0
|
55
|
+
- !ruby/object:Gem::Dependency
|
56
|
+
name: multi_json
|
57
|
+
requirement: !ruby/object:Gem::Requirement
|
58
|
+
requirements:
|
59
|
+
- - "~>"
|
60
|
+
- !ruby/object:Gem::Version
|
61
|
+
version: '1.0'
|
62
|
+
type: :runtime
|
63
|
+
prerelease: false
|
64
|
+
version_requirements: !ruby/object:Gem::Requirement
|
65
|
+
requirements:
|
66
|
+
- - "~>"
|
67
|
+
- !ruby/object:Gem::Version
|
68
|
+
version: '1.0'
|
69
|
+
- !ruby/object:Gem::Dependency
|
70
|
+
name: mime-types
|
71
|
+
requirement: !ruby/object:Gem::Requirement
|
72
|
+
requirements:
|
73
|
+
- - "~>"
|
74
|
+
- !ruby/object:Gem::Version
|
75
|
+
version: '2.99'
|
76
|
+
type: :runtime
|
77
|
+
prerelease: false
|
78
|
+
version_requirements: !ruby/object:Gem::Requirement
|
79
|
+
requirements:
|
80
|
+
- - "~>"
|
81
|
+
- !ruby/object:Gem::Version
|
82
|
+
version: '2.99'
|
83
|
+
- !ruby/object:Gem::Dependency
|
84
|
+
name: net-scp
|
85
|
+
requirement: !ruby/object:Gem::Requirement
|
86
|
+
requirements:
|
87
|
+
- - "~>"
|
88
|
+
- !ruby/object:Gem::Version
|
89
|
+
version: '1.1'
|
90
|
+
type: :runtime
|
91
|
+
prerelease: false
|
92
|
+
version_requirements: !ruby/object:Gem::Requirement
|
93
|
+
requirements:
|
94
|
+
- - "~>"
|
95
|
+
- !ruby/object:Gem::Version
|
96
|
+
version: '1.1'
|
97
|
+
- !ruby/object:Gem::Dependency
|
98
|
+
name: net-ssh
|
99
|
+
requirement: !ruby/object:Gem::Requirement
|
100
|
+
requirements:
|
101
|
+
- - ">="
|
102
|
+
- !ruby/object:Gem::Version
|
103
|
+
version: 2.1.3
|
104
|
+
type: :runtime
|
105
|
+
prerelease: false
|
106
|
+
version_requirements: !ruby/object:Gem::Requirement
|
107
|
+
requirements:
|
108
|
+
- - ">="
|
109
|
+
- !ruby/object:Gem::Version
|
110
|
+
version: 2.1.3
|
111
|
+
- !ruby/object:Gem::Dependency
|
112
|
+
name: nokogiri
|
113
|
+
requirement: !ruby/object:Gem::Requirement
|
114
|
+
requirements:
|
115
|
+
- - "~>"
|
116
|
+
- !ruby/object:Gem::Version
|
117
|
+
version: 1.5.0
|
118
|
+
type: :runtime
|
119
|
+
prerelease: false
|
120
|
+
version_requirements: !ruby/object:Gem::Requirement
|
121
|
+
requirements:
|
122
|
+
- - "~>"
|
123
|
+
- !ruby/object:Gem::Version
|
124
|
+
version: 1.5.0
|
125
|
+
- !ruby/object:Gem::Dependency
|
126
|
+
name: ruby-hmac
|
127
|
+
requirement: !ruby/object:Gem::Requirement
|
128
|
+
requirements:
|
129
|
+
- - ">="
|
130
|
+
- !ruby/object:Gem::Version
|
131
|
+
version: '0'
|
132
|
+
type: :runtime
|
133
|
+
prerelease: false
|
134
|
+
version_requirements: !ruby/object:Gem::Requirement
|
135
|
+
requirements:
|
136
|
+
- - ">="
|
137
|
+
- !ruby/object:Gem::Version
|
138
|
+
version: '0'
|
139
|
+
- !ruby/object:Gem::Dependency
|
140
|
+
name: jekyll
|
141
|
+
requirement: !ruby/object:Gem::Requirement
|
142
|
+
requirements:
|
143
|
+
- - ">="
|
144
|
+
- !ruby/object:Gem::Version
|
145
|
+
version: '0'
|
146
|
+
type: :development
|
147
|
+
prerelease: false
|
148
|
+
version_requirements: !ruby/object:Gem::Requirement
|
149
|
+
requirements:
|
150
|
+
- - ">="
|
151
|
+
- !ruby/object:Gem::Version
|
152
|
+
version: '0'
|
153
|
+
- !ruby/object:Gem::Dependency
|
154
|
+
name: rake
|
155
|
+
requirement: !ruby/object:Gem::Requirement
|
156
|
+
requirements:
|
157
|
+
- - ">="
|
158
|
+
- !ruby/object:Gem::Version
|
159
|
+
version: '0'
|
160
|
+
type: :development
|
161
|
+
prerelease: false
|
162
|
+
version_requirements: !ruby/object:Gem::Requirement
|
163
|
+
requirements:
|
164
|
+
- - ">="
|
165
|
+
- !ruby/object:Gem::Version
|
166
|
+
version: '0'
|
167
|
+
- !ruby/object:Gem::Dependency
|
168
|
+
name: rbvmomi
|
169
|
+
requirement: !ruby/object:Gem::Requirement
|
170
|
+
requirements:
|
171
|
+
- - ">="
|
172
|
+
- !ruby/object:Gem::Version
|
173
|
+
version: '0'
|
174
|
+
type: :development
|
175
|
+
prerelease: false
|
176
|
+
version_requirements: !ruby/object:Gem::Requirement
|
177
|
+
requirements:
|
178
|
+
- - ">="
|
179
|
+
- !ruby/object:Gem::Version
|
180
|
+
version: '0'
|
181
|
+
- !ruby/object:Gem::Dependency
|
182
|
+
name: yard
|
183
|
+
requirement: !ruby/object:Gem::Requirement
|
184
|
+
requirements:
|
185
|
+
- - ">="
|
186
|
+
- !ruby/object:Gem::Version
|
187
|
+
version: '0'
|
188
|
+
type: :development
|
189
|
+
prerelease: false
|
190
|
+
version_requirements: !ruby/object:Gem::Requirement
|
191
|
+
requirements:
|
192
|
+
- - ">="
|
193
|
+
- !ruby/object:Gem::Version
|
194
|
+
version: '0'
|
195
|
+
- !ruby/object:Gem::Dependency
|
196
|
+
name: thor
|
197
|
+
requirement: !ruby/object:Gem::Requirement
|
198
|
+
requirements:
|
199
|
+
- - ">="
|
200
|
+
- !ruby/object:Gem::Version
|
201
|
+
version: '0'
|
202
|
+
type: :development
|
203
|
+
prerelease: false
|
204
|
+
version_requirements: !ruby/object:Gem::Requirement
|
205
|
+
requirements:
|
206
|
+
- - ">="
|
207
|
+
- !ruby/object:Gem::Version
|
208
|
+
version: '0'
|
209
|
+
- !ruby/object:Gem::Dependency
|
210
|
+
name: rspec
|
211
|
+
requirement: !ruby/object:Gem::Requirement
|
212
|
+
requirements:
|
213
|
+
- - "~>"
|
214
|
+
- !ruby/object:Gem::Version
|
215
|
+
version: 1.3.1
|
216
|
+
type: :development
|
217
|
+
prerelease: false
|
218
|
+
version_requirements: !ruby/object:Gem::Requirement
|
219
|
+
requirements:
|
220
|
+
- - "~>"
|
221
|
+
- !ruby/object:Gem::Version
|
222
|
+
version: 1.3.1
|
223
|
+
- !ruby/object:Gem::Dependency
|
224
|
+
name: rbovirt
|
225
|
+
requirement: !ruby/object:Gem::Requirement
|
226
|
+
requirements:
|
227
|
+
- - ">="
|
228
|
+
- !ruby/object:Gem::Version
|
229
|
+
version: 0.0.11
|
230
|
+
type: :development
|
231
|
+
prerelease: false
|
232
|
+
version_requirements: !ruby/object:Gem::Requirement
|
233
|
+
requirements:
|
234
|
+
- - ">="
|
235
|
+
- !ruby/object:Gem::Version
|
236
|
+
version: 0.0.11
|
237
|
+
- !ruby/object:Gem::Dependency
|
238
|
+
name: shindo
|
239
|
+
requirement: !ruby/object:Gem::Requirement
|
240
|
+
requirements:
|
241
|
+
- - "~>"
|
242
|
+
- !ruby/object:Gem::Version
|
243
|
+
version: 0.3.4
|
244
|
+
type: :development
|
245
|
+
prerelease: false
|
246
|
+
version_requirements: !ruby/object:Gem::Requirement
|
247
|
+
requirements:
|
248
|
+
- - "~>"
|
249
|
+
- !ruby/object:Gem::Version
|
250
|
+
version: 0.3.4
|
251
|
+
- !ruby/object:Gem::Dependency
|
252
|
+
name: fission
|
253
|
+
requirement: !ruby/object:Gem::Requirement
|
254
|
+
requirements:
|
255
|
+
- - ">="
|
256
|
+
- !ruby/object:Gem::Version
|
257
|
+
version: '0'
|
258
|
+
type: :development
|
259
|
+
prerelease: false
|
260
|
+
version_requirements: !ruby/object:Gem::Requirement
|
261
|
+
requirements:
|
262
|
+
- - ">="
|
263
|
+
- !ruby/object:Gem::Version
|
264
|
+
version: '0'
|
265
|
+
- !ruby/object:Gem::Dependency
|
266
|
+
name: pry
|
267
|
+
requirement: !ruby/object:Gem::Requirement
|
268
|
+
requirements:
|
269
|
+
- - ">="
|
270
|
+
- !ruby/object:Gem::Version
|
271
|
+
version: '0'
|
272
|
+
type: :development
|
273
|
+
prerelease: false
|
274
|
+
version_requirements: !ruby/object:Gem::Requirement
|
275
|
+
requirements:
|
276
|
+
- - ">="
|
277
|
+
- !ruby/object:Gem::Version
|
278
|
+
version: '0'
|
279
|
+
description: The Ruby cloud services library. Supports all major cloud providers including
|
280
|
+
AWS, Rackspace, Linode, Blue Box, StormOnDemand, and many others. Full support for
|
281
|
+
most AWS services including EC2, S3, CloudWatch, SimpleDB, ELB, and RDS.
|
282
|
+
email: geemus@gmail.com
|
283
|
+
executables:
|
284
|
+
- fog
|
285
|
+
extensions: []
|
286
|
+
extra_rdoc_files:
|
287
|
+
- README.md
|
288
|
+
files:
|
289
|
+
- README.md
|
290
|
+
- bin/fog
|
291
|
+
homepage: http://github.com/fog/fog
|
292
|
+
licenses: []
|
293
|
+
metadata: {}
|
294
|
+
post_install_message:
|
295
|
+
rdoc_options:
|
296
|
+
- "--charset=UTF-8"
|
297
|
+
require_paths:
|
298
|
+
- lib
|
299
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
300
|
+
requirements:
|
301
|
+
- - ">="
|
302
|
+
- !ruby/object:Gem::Version
|
303
|
+
version: '0'
|
304
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
305
|
+
requirements:
|
306
|
+
- - ">="
|
307
|
+
- !ruby/object:Gem::Version
|
308
|
+
version: '0'
|
309
|
+
requirements: []
|
310
|
+
rubyforge_project: r5_fog
|
311
|
+
rubygems_version: 2.4.5
|
312
|
+
signing_key:
|
313
|
+
specification_version: 2
|
314
|
+
summary: brings clouds to you
|
315
|
+
test_files: []
|