qiflib 0.0.1alpha → 0.0.2alpha
Sign up to get free protection for your applications and to get access to all the features.
- data/README.md +1 -78
- data/lib/qiflib.rb +6 -0
- data/lib/qiflib_money.rb +10 -3
- data/lib/qiflib_transaction.rb +31 -11
- data/lib/qiflib_util.rb +1 -1
- data/lib/qiflib_version.rb +2 -2
- metadata +4 -4
data/README.md
CHANGED
@@ -1,78 +1 @@
|
|
1
|
-
|
2
|
-
|
3
|
-
factory_girl is a fixtures replacement with a straightforward definition syntax, support for multiple build strategies (saved instances, unsaved instances, attribute hashes, and stubbed objects), and support for multiple factories for the same class (user, admin_user, and so on), including factory inheritance.
|
4
|
-
|
5
|
-
If you want to use factory_girl with Rails, see
|
6
|
-
[factory_girl_rails](https://github.com/thoughtbot/factory_girl_rails).
|
7
|
-
|
8
|
-
Documentation
|
9
|
-
-------------
|
10
|
-
|
11
|
-
You should find the documentation for your version of factory_girl on [Rubygems](https://rubygems.org/gems/factory_girl).
|
12
|
-
|
13
|
-
See [GETTING_STARTED](https://github.com/thoughtbot/factory_girl/blob/master/GETTING_STARTED.md) for information on defining and using factories.
|
14
|
-
|
15
|
-
Install
|
16
|
-
--------
|
17
|
-
|
18
|
-
```shell
|
19
|
-
gem install factory_girl
|
20
|
-
```
|
21
|
-
or add the following line to Gemfile:
|
22
|
-
|
23
|
-
```ruby
|
24
|
-
gem 'factory_girl'
|
25
|
-
```
|
26
|
-
and run `bundle install` from your shell.
|
27
|
-
|
28
|
-
Supported Ruby versions
|
29
|
-
-----------------------
|
30
|
-
|
31
|
-
The Factory Girl 3.x series supports Ruby 1.9.x.
|
32
|
-
|
33
|
-
For older versions of Ruby, please use the Factory Girl 2.x series.
|
34
|
-
|
35
|
-
More Information
|
36
|
-
----------------
|
37
|
-
|
38
|
-
* [Rubygems](https://rubygems.org/gems/factory_girl)
|
39
|
-
* [Mailing list](http://groups.google.com/group/factory_girl)
|
40
|
-
* [Issues](https://github.com/thoughtbot/factory_girl/issues)
|
41
|
-
* [GIANT ROBOTS SMASHING INTO OTHER GIANT ROBOTS](http://robots.thoughtbot.com/)
|
42
|
-
|
43
|
-
Contributing
|
44
|
-
------------
|
45
|
-
|
46
|
-
Please see the [contribution guidelines](https://github.com/thoughtbot/factory_girl/blob/master/CONTRIBUTION_GUIDELINES.md).
|
47
|
-
|
48
|
-
Credits
|
49
|
-
-------
|
50
|
-
|
51
|
-
factory_girl was written by Joe Ferris with contributions from several authors, including:
|
52
|
-
|
53
|
-
* Alex Sharp
|
54
|
-
* Eugene Bolshakov
|
55
|
-
* Jon Yurek
|
56
|
-
* Josh Nichols
|
57
|
-
* Josh Owens
|
58
|
-
* Nate Sutton
|
59
|
-
* Josh Clayton
|
60
|
-
* Thomas Walpole
|
61
|
-
|
62
|
-
The syntax layers are derived from software written by the following authors:
|
63
|
-
|
64
|
-
* Pete Yandell
|
65
|
-
* Rick Bradley
|
66
|
-
* Yossef Mendelssohn
|
67
|
-
|
68
|
-
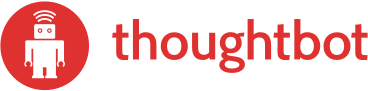
|
69
|
-
|
70
|
-
factory_girl is maintained and funded by [thoughtbot, inc](http://thoughtbot.com/community)
|
71
|
-
|
72
|
-
The names and logos for thoughtbot are trademarks of thoughtbot, inc.
|
73
|
-
|
74
|
-
License
|
75
|
-
-------
|
76
|
-
|
77
|
-
factory_girl is Copyright © 2008-2011 Joe Ferris and thoughtbot. It is free software, and may be redistributed under the terms specified in the LICENSE file.
|
78
|
-
|
1
|
+
README for qiflib
|
data/lib/qiflib.rb
CHANGED
data/lib/qiflib_money.rb
CHANGED
@@ -3,9 +3,16 @@ module Qiflib
|
|
3
3
|
|
4
4
|
class Money
|
5
5
|
|
6
|
+
attr_reader :string_value
|
7
|
+
|
6
8
|
def initialize(s='0.00')
|
7
|
-
|
8
|
-
|
9
|
+
@string_value = "#{s}".tr('TBL, ' , '')
|
10
|
+
if @string_value.size < 1
|
11
|
+
@string_value = '0.00'
|
12
|
+
end
|
13
|
+
if @string_value.end_with?('.')
|
14
|
+
@string_value = "#{string_value}00"
|
15
|
+
end
|
9
16
|
end
|
10
17
|
|
11
18
|
def to_s
|
@@ -13,7 +20,7 @@ module Qiflib
|
|
13
20
|
end
|
14
21
|
|
15
22
|
def cents
|
16
|
-
|
23
|
+
string_value.tr(".","").to_f.to_i
|
17
24
|
end
|
18
25
|
|
19
26
|
end
|
data/lib/qiflib_transaction.rb
CHANGED
@@ -3,15 +3,19 @@ module Qiflib
|
|
3
3
|
|
4
4
|
class Transaction
|
5
5
|
|
6
|
-
|
7
|
-
|
6
|
+
attr_reader :id # computed field
|
7
|
+
attr_reader :acct_owner, :acct_name, :acct_type # constructor arg fields
|
8
|
+
attr_reader :date, :amount, :cleared, :category, :number, :payee, :memo # data fields
|
9
|
+
attr_reader :address
|
10
|
+
|
8
11
|
|
9
12
|
def initialize(acct_owner=nil, acct_name=nil, acct_type=nil)
|
10
13
|
if acct_owner
|
11
14
|
@acct_owner = "#{acct_owner}".downcase
|
12
15
|
@acct_name = "#{acct_name}".downcase
|
13
16
|
@acct_type = "#{acct_type}".downcase
|
14
|
-
@
|
17
|
+
@id, @date, @amount, @cleared, @category, @number, @memo, @payee = 0, nil, nil, '', '', '', '', ''
|
18
|
+
@splits, @curr_split, @address = [], {}, []
|
15
19
|
end
|
16
20
|
end
|
17
21
|
|
@@ -48,29 +52,45 @@ module Qiflib
|
|
48
52
|
elsif (stripped.match(/^L/))
|
49
53
|
@category = line_value(stripped)
|
50
54
|
elsif (stripped.match(/^S/))
|
51
|
-
|
55
|
+
current_split['category'] = line_value(stripped)
|
52
56
|
elsif (stripped.match(/^E/))
|
53
|
-
|
57
|
+
puts "E lv: #{line_value(stripped)}"
|
58
|
+
current_split['memo'] = line_value(stripped)
|
54
59
|
elsif (stripped.match(/^A/))
|
55
|
-
@address << stripped
|
56
|
-
elsif (stripped.match(
|
57
|
-
|
60
|
+
@address << line_value(stripped)
|
61
|
+
elsif (stripped.match(/^\$/))
|
62
|
+
current_split['amount'] = Qiflib::Money.new(line_value(stripped))
|
63
|
+
splits << current_split
|
64
|
+
@curr_split = {}
|
58
65
|
end
|
59
66
|
end
|
60
67
|
end
|
61
68
|
end
|
62
69
|
|
63
70
|
def valid?
|
64
|
-
|
71
|
+
return false if @date.nil?
|
72
|
+
true
|
73
|
+
end
|
74
|
+
|
75
|
+
def current_split
|
76
|
+
@curr_split = {} if @curr_split.nil?
|
77
|
+
@curr_split
|
78
|
+
end
|
79
|
+
|
80
|
+
def splits
|
81
|
+
@splits = [] if @splits.nil?
|
82
|
+
@splits
|
65
83
|
end
|
66
84
|
|
67
85
|
def to_csv
|
68
|
-
|
86
|
+
CSV.generate do | csv |
|
87
|
+
csv << [ acct_owner, acct_name, acct_type, date, amount, cleared, category, number, payee, memo ]
|
88
|
+
end
|
69
89
|
end
|
70
90
|
|
71
91
|
def line_value(s)
|
72
92
|
return '' if s.nil? || s.size < 1
|
73
|
-
s[1, s.size]
|
93
|
+
s[1, s.size].strip
|
74
94
|
end
|
75
95
|
|
76
96
|
end
|
data/lib/qiflib_util.rb
CHANGED
data/lib/qiflib_version.rb
CHANGED
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: qiflib
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
4
|
+
version: 0.0.2alpha
|
5
5
|
prerelease: 5
|
6
6
|
platform: ruby
|
7
7
|
authors:
|
@@ -9,11 +9,11 @@ authors:
|
|
9
9
|
autorequire:
|
10
10
|
bindir: bin
|
11
11
|
cert_chain: []
|
12
|
-
date: 2012-03
|
12
|
+
date: 2012-04-03 00:00:00.000000000Z
|
13
13
|
dependencies:
|
14
14
|
- !ruby/object:Gem::Dependency
|
15
15
|
name: rspec
|
16
|
-
requirement: &
|
16
|
+
requirement: &70134028206920 !ruby/object:Gem::Requirement
|
17
17
|
none: false
|
18
18
|
requirements:
|
19
19
|
- - ! '>='
|
@@ -21,7 +21,7 @@ dependencies:
|
|
21
21
|
version: 2.9.0
|
22
22
|
type: :development
|
23
23
|
prerelease: false
|
24
|
-
version_requirements: *
|
24
|
+
version_requirements: *70134028206920
|
25
25
|
description: A ruby library for qif file processing.
|
26
26
|
email: cjoakim@bellsouth.net
|
27
27
|
executables: []
|