qiflib 0.0.1alpha
Sign up to get free protection for your applications and to get access to all the features.
- data/README.md +78 -0
- data/lib/qiflib.rb +6 -0
- data/lib/qiflib_date.rb +38 -0
- data/lib/qiflib_money.rb +21 -0
- data/lib/qiflib_transaction.rb +78 -0
- data/lib/qiflib_util.rb +43 -0
- data/lib/qiflib_version.rb +6 -0
- metadata +64 -0
data/README.md
ADDED
@@ -0,0 +1,78 @@
|
|
1
|
+
# factory_girl [](http://travis-ci.org/thoughtbot/factory_girl?branch=master) [](https://gemnasium.com/thoughtbot/factory_girl)
|
2
|
+
|
3
|
+
factory_girl is a fixtures replacement with a straightforward definition syntax, support for multiple build strategies (saved instances, unsaved instances, attribute hashes, and stubbed objects), and support for multiple factories for the same class (user, admin_user, and so on), including factory inheritance.
|
4
|
+
|
5
|
+
If you want to use factory_girl with Rails, see
|
6
|
+
[factory_girl_rails](https://github.com/thoughtbot/factory_girl_rails).
|
7
|
+
|
8
|
+
Documentation
|
9
|
+
-------------
|
10
|
+
|
11
|
+
You should find the documentation for your version of factory_girl on [Rubygems](https://rubygems.org/gems/factory_girl).
|
12
|
+
|
13
|
+
See [GETTING_STARTED](https://github.com/thoughtbot/factory_girl/blob/master/GETTING_STARTED.md) for information on defining and using factories.
|
14
|
+
|
15
|
+
Install
|
16
|
+
--------
|
17
|
+
|
18
|
+
```shell
|
19
|
+
gem install factory_girl
|
20
|
+
```
|
21
|
+
or add the following line to Gemfile:
|
22
|
+
|
23
|
+
```ruby
|
24
|
+
gem 'factory_girl'
|
25
|
+
```
|
26
|
+
and run `bundle install` from your shell.
|
27
|
+
|
28
|
+
Supported Ruby versions
|
29
|
+
-----------------------
|
30
|
+
|
31
|
+
The Factory Girl 3.x series supports Ruby 1.9.x.
|
32
|
+
|
33
|
+
For older versions of Ruby, please use the Factory Girl 2.x series.
|
34
|
+
|
35
|
+
More Information
|
36
|
+
----------------
|
37
|
+
|
38
|
+
* [Rubygems](https://rubygems.org/gems/factory_girl)
|
39
|
+
* [Mailing list](http://groups.google.com/group/factory_girl)
|
40
|
+
* [Issues](https://github.com/thoughtbot/factory_girl/issues)
|
41
|
+
* [GIANT ROBOTS SMASHING INTO OTHER GIANT ROBOTS](http://robots.thoughtbot.com/)
|
42
|
+
|
43
|
+
Contributing
|
44
|
+
------------
|
45
|
+
|
46
|
+
Please see the [contribution guidelines](https://github.com/thoughtbot/factory_girl/blob/master/CONTRIBUTION_GUIDELINES.md).
|
47
|
+
|
48
|
+
Credits
|
49
|
+
-------
|
50
|
+
|
51
|
+
factory_girl was written by Joe Ferris with contributions from several authors, including:
|
52
|
+
|
53
|
+
* Alex Sharp
|
54
|
+
* Eugene Bolshakov
|
55
|
+
* Jon Yurek
|
56
|
+
* Josh Nichols
|
57
|
+
* Josh Owens
|
58
|
+
* Nate Sutton
|
59
|
+
* Josh Clayton
|
60
|
+
* Thomas Walpole
|
61
|
+
|
62
|
+
The syntax layers are derived from software written by the following authors:
|
63
|
+
|
64
|
+
* Pete Yandell
|
65
|
+
* Rick Bradley
|
66
|
+
* Yossef Mendelssohn
|
67
|
+
|
68
|
+
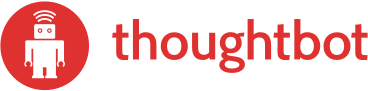
|
69
|
+
|
70
|
+
factory_girl is maintained and funded by [thoughtbot, inc](http://thoughtbot.com/community)
|
71
|
+
|
72
|
+
The names and logos for thoughtbot are trademarks of thoughtbot, inc.
|
73
|
+
|
74
|
+
License
|
75
|
+
-------
|
76
|
+
|
77
|
+
factory_girl is Copyright © 2008-2011 Joe Ferris and thoughtbot. It is free software, and may be redistributed under the terms specified in the LICENSE file.
|
78
|
+
|
data/lib/qiflib.rb
ADDED
data/lib/qiflib_date.rb
ADDED
@@ -0,0 +1,38 @@
|
|
1
|
+
=begin rdoc
|
2
|
+
|
3
|
+
Instances of this class represent a date from within a qif file,
|
4
|
+
such as 'D5/24/94'.
|
5
|
+
|
6
|
+
=end
|
7
|
+
|
8
|
+
module Qiflib
|
9
|
+
|
10
|
+
class Date
|
11
|
+
|
12
|
+
attr_accessor :string_value, :ccyymmdd, :year, :year_mm, :yy, :mm, :dd
|
13
|
+
|
14
|
+
def initialize(string_value='')
|
15
|
+
@cc, @yy, @mm, @dd = '00', '00', '00', '00'
|
16
|
+
@string_value = "#{string_value}".tr('D','')
|
17
|
+
tokens = @string_value.split('/')
|
18
|
+
if (tokens && tokens.size > 2)
|
19
|
+
m = tokens[0].to_i
|
20
|
+
d = tokens[1].to_i
|
21
|
+
y = tokens[2].to_i
|
22
|
+
@yy = tokens[2]
|
23
|
+
y < 50 ? @cc = "20" : @cc = "19"
|
24
|
+
m < 10 ? @mm = "0#{m}" : @mm = "#{m}"
|
25
|
+
d < 10 ? @dd = "0#{d}" : @dd = "#{d}"
|
26
|
+
end
|
27
|
+
@ccyymmdd = "#{@cc}#{@yy}-#{@mm}-#{@dd}"
|
28
|
+
@year = "#{@cc}#{@yy}"
|
29
|
+
@year_mm = "#{@cc}#{@yy}-#{@mm}"
|
30
|
+
end
|
31
|
+
|
32
|
+
def to_s
|
33
|
+
@ccyymmdd
|
34
|
+
end
|
35
|
+
|
36
|
+
end
|
37
|
+
|
38
|
+
end
|
data/lib/qiflib_money.rb
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
|
2
|
+
module Qiflib
|
3
|
+
|
4
|
+
class Money
|
5
|
+
|
6
|
+
def initialize(s='0.00')
|
7
|
+
s ? @string_value = s.strip.tr('TBL, ' , '') : @string_value = '0.00'
|
8
|
+
@string_value = '0.00' if @string_value.size < 1
|
9
|
+
end
|
10
|
+
|
11
|
+
def to_s
|
12
|
+
@string_value
|
13
|
+
end
|
14
|
+
|
15
|
+
def cents
|
16
|
+
@string_value.tr(".","").to_i
|
17
|
+
end
|
18
|
+
|
19
|
+
end
|
20
|
+
|
21
|
+
end
|
@@ -0,0 +1,78 @@
|
|
1
|
+
|
2
|
+
module Qiflib
|
3
|
+
|
4
|
+
class Transaction
|
5
|
+
|
6
|
+
attr_accessor :raw_lines, :id, :acct_owner, :acct_name, :acct_type, :date, :amount
|
7
|
+
attr_accessor :valid, :balance, :cleared, :category, :number, :payee, :memo, :address
|
8
|
+
|
9
|
+
def initialize(acct_owner=nil, acct_name=nil, acct_type=nil)
|
10
|
+
if acct_owner
|
11
|
+
@acct_owner = "#{acct_owner}".downcase
|
12
|
+
@acct_name = "#{acct_name}".downcase
|
13
|
+
@acct_type = "#{acct_type}".downcase
|
14
|
+
@cleared, @number, @memo, @raw_lines, @splits, @address = '', '', '', [], [], []
|
15
|
+
end
|
16
|
+
end
|
17
|
+
|
18
|
+
def add_line(line)
|
19
|
+
if line
|
20
|
+
stripped = line.strip
|
21
|
+
puts "Transaction.add_line: #{stripped}"
|
22
|
+
if stripped.size > 0
|
23
|
+
# Field Indicator Explanations:
|
24
|
+
# D Date
|
25
|
+
# T Amount
|
26
|
+
# C Cleared status
|
27
|
+
# N Num (check or reference number)
|
28
|
+
# P Payee
|
29
|
+
# M Memo
|
30
|
+
# A Address (up to five lines; the sixth line is an optional message)
|
31
|
+
# L Category (Category/Subcategory/Transfer/Class)
|
32
|
+
# S Category in split (Category/Transfer/Class)
|
33
|
+
# E Memo in split
|
34
|
+
# $ Dollar amount of split
|
35
|
+
# ^ End of the entry
|
36
|
+
if (stripped.match(/^D/))
|
37
|
+
@date = Qiflib::Date.new(line_value(stripped))
|
38
|
+
elsif (stripped.match(/^T/))
|
39
|
+
@amount = Qiflib::Money.new(line_value(stripped))
|
40
|
+
elsif (stripped.match(/^P/))
|
41
|
+
@payee = line_value(stripped)
|
42
|
+
elsif (stripped.match(/^C/))
|
43
|
+
@cleared = line_value(stripped)
|
44
|
+
elsif (stripped.match(/^N/))
|
45
|
+
@number = line_value(stripped)
|
46
|
+
elsif (stripped.match(/^M/))
|
47
|
+
@memo = line_value(stripped)
|
48
|
+
elsif (stripped.match(/^L/))
|
49
|
+
@category = line_value(stripped)
|
50
|
+
elsif (stripped.match(/^S/))
|
51
|
+
@split_category = line_value(stripped)
|
52
|
+
elsif (stripped.match(/^E/))
|
53
|
+
@split_memo = line_value(stripped)
|
54
|
+
elsif (stripped.match(/^A/))
|
55
|
+
@address << stripped
|
56
|
+
elsif (stripped.match(/^$/))
|
57
|
+
# ignore
|
58
|
+
end
|
59
|
+
end
|
60
|
+
end
|
61
|
+
end
|
62
|
+
|
63
|
+
def valid?
|
64
|
+
(date.nil?) ? false : true
|
65
|
+
end
|
66
|
+
|
67
|
+
def to_csv
|
68
|
+
"x,y,z"
|
69
|
+
end
|
70
|
+
|
71
|
+
def line_value(s)
|
72
|
+
return '' if s.nil? || s.size < 1
|
73
|
+
s[1, s.size]
|
74
|
+
end
|
75
|
+
|
76
|
+
end
|
77
|
+
|
78
|
+
end
|
data/lib/qiflib_util.rb
ADDED
@@ -0,0 +1,43 @@
|
|
1
|
+
|
2
|
+
module Qiflib
|
3
|
+
|
4
|
+
class Util
|
5
|
+
|
6
|
+
def self.qiftocsv(files=[])
|
7
|
+
transactions, csv_lines = [], []
|
8
|
+
if files
|
9
|
+
files.each { | filename |
|
10
|
+
process_qif_file(filename, transactions)
|
11
|
+
}
|
12
|
+
transactions.each { | tran |
|
13
|
+
csv_lines << tran.to_csv
|
14
|
+
}
|
15
|
+
end
|
16
|
+
csv_lines
|
17
|
+
end
|
18
|
+
|
19
|
+
def self.process_qif_file(filename, transactions)
|
20
|
+
begin
|
21
|
+
file = File.new(filename, 'r')
|
22
|
+
current_tran = Qiflib::Transaction.new
|
23
|
+
while (line = file.gets)
|
24
|
+
stripped = line.strip
|
25
|
+
if stripped == '^'
|
26
|
+
if current_tran.valid?
|
27
|
+
transactions << current_tran
|
28
|
+
end
|
29
|
+
current_tran = Qiflib::Transaction.new
|
30
|
+
else
|
31
|
+
current_tran.add_line(stripped)
|
32
|
+
end
|
33
|
+
end
|
34
|
+
file.close
|
35
|
+
rescue => err
|
36
|
+
puts "Exception: #{err.inspect}"
|
37
|
+
end
|
38
|
+
end
|
39
|
+
|
40
|
+
end
|
41
|
+
|
42
|
+
end
|
43
|
+
|
metadata
ADDED
@@ -0,0 +1,64 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: qiflib
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.1alpha
|
5
|
+
prerelease: 5
|
6
|
+
platform: ruby
|
7
|
+
authors:
|
8
|
+
- Chris Joakim
|
9
|
+
autorequire:
|
10
|
+
bindir: bin
|
11
|
+
cert_chain: []
|
12
|
+
date: 2012-03-31 00:00:00.000000000Z
|
13
|
+
dependencies:
|
14
|
+
- !ruby/object:Gem::Dependency
|
15
|
+
name: rspec
|
16
|
+
requirement: &70364218596520 !ruby/object:Gem::Requirement
|
17
|
+
none: false
|
18
|
+
requirements:
|
19
|
+
- - ! '>='
|
20
|
+
- !ruby/object:Gem::Version
|
21
|
+
version: 2.9.0
|
22
|
+
type: :development
|
23
|
+
prerelease: false
|
24
|
+
version_requirements: *70364218596520
|
25
|
+
description: A ruby library for qif file processing.
|
26
|
+
email: cjoakim@bellsouth.net
|
27
|
+
executables: []
|
28
|
+
extensions: []
|
29
|
+
extra_rdoc_files: []
|
30
|
+
files:
|
31
|
+
- lib/qiflib.rb
|
32
|
+
- lib/qiflib_date.rb
|
33
|
+
- lib/qiflib_money.rb
|
34
|
+
- lib/qiflib_transaction.rb
|
35
|
+
- lib/qiflib_util.rb
|
36
|
+
- lib/qiflib_version.rb
|
37
|
+
- README.md
|
38
|
+
homepage: http://rubygems.org/gems/qiflib
|
39
|
+
licenses:
|
40
|
+
- MIT
|
41
|
+
- GPL-2
|
42
|
+
post_install_message:
|
43
|
+
rdoc_options: []
|
44
|
+
require_paths:
|
45
|
+
- lib
|
46
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
47
|
+
none: false
|
48
|
+
requirements:
|
49
|
+
- - ! '>='
|
50
|
+
- !ruby/object:Gem::Version
|
51
|
+
version: 1.9.2
|
52
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
53
|
+
none: false
|
54
|
+
requirements:
|
55
|
+
- - ! '>'
|
56
|
+
- !ruby/object:Gem::Version
|
57
|
+
version: 1.3.1
|
58
|
+
requirements: []
|
59
|
+
rubyforge_project:
|
60
|
+
rubygems_version: 1.8.17
|
61
|
+
signing_key:
|
62
|
+
specification_version: 3
|
63
|
+
summary: qiflib
|
64
|
+
test_files: []
|