pronto-annotations_formatter 0.1.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +7 -0
- data/CHANGELOG.md +10 -0
- data/LICENSE +21 -0
- data/README.md +70 -0
- data/lib/pronto/annotations_formatter/version.rb +7 -0
- data/lib/pronto/annotations_formatter.rb +73 -0
- metadata +72 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: d47d54a34a7ae4c0581de64e31dd7ee51d953ae6d6f8a7e2d87c3b90c0d5c977
|
4
|
+
data.tar.gz: a62ecd054dc312d9b5cbafb407e5ab9ceabc993e96aa0974338017ddd6130765
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: bea6670b76b06244805e08acfbd70919e7753ba3526c0d9ed5f7d8d72384578512e6aaae239e34722fb9182d6c4987a7320d6fb5823fc204c771d48e452e0bbd
|
7
|
+
data.tar.gz: c56169b1c22a30ce947055fc89216144ac2e9bd394287feb6de4330f63a7432c1504a5c717d0830f362f4a9dec49075baeb9041ab13c3054b8283f2b36d9e85a
|
data/CHANGELOG.md
ADDED
@@ -0,0 +1,10 @@
|
|
1
|
+
# Changelog
|
2
|
+
|
3
|
+
## 0.1.0
|
4
|
+
|
5
|
+
This initial version uses a monkeypatch to change `FORMATTERS` const, adding the new formatter.
|
6
|
+
Also, keeps compatibility with pronto versions prior to 0.11.2.
|
7
|
+
|
8
|
+
### New features
|
9
|
+
|
10
|
+
- Create AnnotationsFormatter class and change FORMATTERS const to include the new format
|
data/LICENSE
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
MIT License
|
2
|
+
|
3
|
+
Copyright (c) 2023 Emílio
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in all
|
13
|
+
copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
21
|
+
SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,70 @@
|
|
1
|
+
# Pronto::AnnotationsFormatter
|
2
|
+
|
3
|
+
Welcome!
|
4
|
+
|
5
|
+
[prontolabs/pronto](https://github.com/prontolabs/pronto) is a great tool for code review, but it post the warnings as comments,
|
6
|
+
which can be a bit of a mess and create many comments on the PR causing a huge noise for developers discussion.
|
7
|
+
|
8
|
+
In a combination with [pronto-annotate-action](https://github.com/emilio2hd/pronto-annotate-action), it's possible to write annotations to the code, like this:
|
9
|
+
|
10
|
+
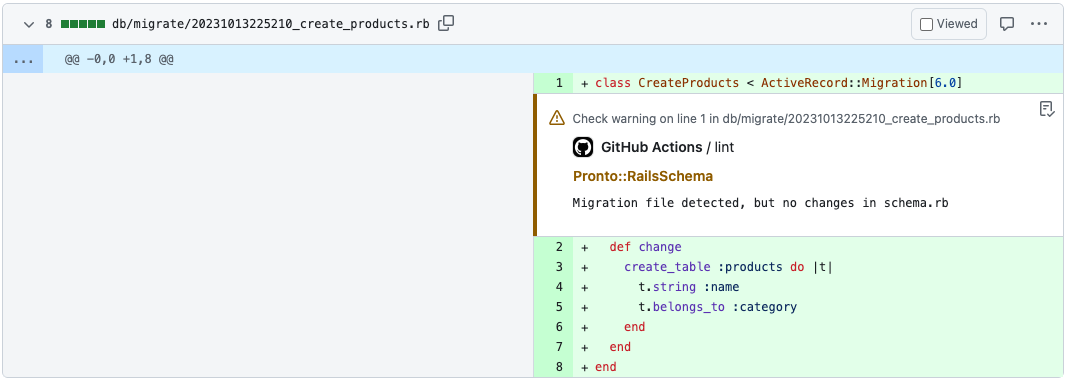
|
11
|
+
Leaving the comments just for the developers.
|
12
|
+
|
13
|
+
Check the [.github/workflows/pr_linter.yml](.github/workflows/pr_linter.yml) and [this pull-request](https://github.com/emilio2hd/pronto-annotations_formatter/pull/1/files).
|
14
|
+
|
15
|
+
## Installation
|
16
|
+
|
17
|
+
Add this line to your application's Gemfile:
|
18
|
+
|
19
|
+
```ruby
|
20
|
+
gem 'pronto-annotations_formatter'
|
21
|
+
```
|
22
|
+
|
23
|
+
And then execute:
|
24
|
+
|
25
|
+
```shell
|
26
|
+
bundle install
|
27
|
+
```
|
28
|
+
|
29
|
+
Or install it yourself as:
|
30
|
+
|
31
|
+
```shell
|
32
|
+
gem install pronto-annotations_formatter
|
33
|
+
```
|
34
|
+
|
35
|
+
## Usage
|
36
|
+
|
37
|
+
```shell
|
38
|
+
bundle exec pronto run -f annotations -c origin/main
|
39
|
+
```
|
40
|
+
|
41
|
+
The command above, will print some json like this:
|
42
|
+
|
43
|
+
```json
|
44
|
+
[
|
45
|
+
{
|
46
|
+
"message": "Migration file detected, but no changes in schema.rb",
|
47
|
+
"level": "warning",
|
48
|
+
"file": "db/migrate/20231013225210_create_products.rb",
|
49
|
+
"line": {
|
50
|
+
"start": 1,
|
51
|
+
"end": 1
|
52
|
+
},
|
53
|
+
"title": "Pronto::RailsSchema"
|
54
|
+
}
|
55
|
+
]
|
56
|
+
```
|
57
|
+
|
58
|
+
## Development
|
59
|
+
|
60
|
+
Then, run `bundle exec rspec` to run the tests. You can also run `bin/console` for an interactive prompt that will allow you to experiment.
|
61
|
+
|
62
|
+
To install this gem onto your local machine, run `bundle exec rake install`. To release a new version, update the version number in `version.rb`, and then run `bundle exec rake release`, which will create a git tag for the version, push git commits and the created tag, and push the `.gem` file to [rubygems.org](https://rubygems.org).
|
63
|
+
|
64
|
+
## Contributing
|
65
|
+
|
66
|
+
Bug reports and pull requests are welcome on GitHub at <https://github.com/emilio2hd/pronto-annotations_formatter>.
|
67
|
+
|
68
|
+
## License
|
69
|
+
|
70
|
+
The gem is available as open source under the terms of the [MIT License](https://opensource.org/licenses/MIT).
|
@@ -0,0 +1,73 @@
|
|
1
|
+
# frozen_string_literal: true
|
2
|
+
|
3
|
+
require "pronto/formatter/base"
|
4
|
+
|
5
|
+
require_relative "annotations_formatter/version"
|
6
|
+
|
7
|
+
module Pronto
|
8
|
+
module Formatter
|
9
|
+
class AnnotationsFormatter < Pronto::Formatter::Base
|
10
|
+
# {
|
11
|
+
# "message": "Some message",
|
12
|
+
# "level": "warning",
|
13
|
+
# "file": "some_file.rb",
|
14
|
+
# "line": { "start": 1, "end": 1 },
|
15
|
+
# "title": "The warning issuer, the runner"
|
16
|
+
# }
|
17
|
+
def format(messages, _repo, _patches)
|
18
|
+
messages.map { |m| build_report_line(m) }.to_json
|
19
|
+
end
|
20
|
+
|
21
|
+
private
|
22
|
+
|
23
|
+
def build_report_line(message)
|
24
|
+
lineno = message.line.new_lineno if message.line
|
25
|
+
|
26
|
+
result = {
|
27
|
+
message: message.msg,
|
28
|
+
level: get_github_level(message.level)
|
29
|
+
}
|
30
|
+
result[:file] = message.path if message.path
|
31
|
+
result[:line] = { start: lineno, end: lineno } if lineno
|
32
|
+
result[:title] = message.runner if message.runner
|
33
|
+
|
34
|
+
result
|
35
|
+
end
|
36
|
+
|
37
|
+
def get_github_level(pronto_level)
|
38
|
+
case pronto_level
|
39
|
+
when :error, :fatal
|
40
|
+
"error"
|
41
|
+
when :warning
|
42
|
+
"warning"
|
43
|
+
else
|
44
|
+
"notice"
|
45
|
+
end
|
46
|
+
end
|
47
|
+
end
|
48
|
+
end
|
49
|
+
|
50
|
+
module AnnotationsFormatterMonkeypatch
|
51
|
+
ANNOTATION_OPTION = "annotations"
|
52
|
+
ORIGINAL_FORMATTERS = {}.freeze
|
53
|
+
|
54
|
+
# Get Pronto::Formatter::FORMATTERS constant and set a new one, adding the annotations leading to new formatter.
|
55
|
+
# As FORMATTERS is a frozen hash, it's required remove it and set a new one with the new option.
|
56
|
+
def self.apply_patch
|
57
|
+
return unless Pronto::Formatter.const_defined?(:FORMATTERS)
|
58
|
+
return if Pronto::Formatter::FORMATTERS.keys.include?(ANNOTATION_OPTION)
|
59
|
+
|
60
|
+
formatters_const = Pronto::Formatter.const_get(:FORMATTERS)
|
61
|
+
Pronto::Formatter.send(:remove_const, :FORMATTERS)
|
62
|
+
|
63
|
+
send(:remove_const, :ORIGINAL_FORMATTERS)
|
64
|
+
const_set(:ORIGINAL_FORMATTERS, formatters_const)
|
65
|
+
Pronto::Formatter.const_set(
|
66
|
+
:FORMATTERS,
|
67
|
+
formatters_const.merge(ANNOTATION_OPTION => Pronto::Formatter::AnnotationsFormatter).freeze
|
68
|
+
)
|
69
|
+
end
|
70
|
+
|
71
|
+
apply_patch
|
72
|
+
end
|
73
|
+
end
|
metadata
ADDED
@@ -0,0 +1,72 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: pronto-annotations_formatter
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.1.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Emilio
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2023-10-31 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: pronto
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - ">="
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: 0.5.0
|
20
|
+
- - "<"
|
21
|
+
- !ruby/object:Gem::Version
|
22
|
+
version: 0.11.2
|
23
|
+
type: :runtime
|
24
|
+
prerelease: false
|
25
|
+
version_requirements: !ruby/object:Gem::Requirement
|
26
|
+
requirements:
|
27
|
+
- - ">="
|
28
|
+
- !ruby/object:Gem::Version
|
29
|
+
version: 0.5.0
|
30
|
+
- - "<"
|
31
|
+
- !ruby/object:Gem::Version
|
32
|
+
version: 0.11.2
|
33
|
+
description: |2
|
34
|
+
This pronto formatter will print out the warnings in a json format to be consumed by
|
35
|
+
https://github.com/emilio2hd/pronto-annotate-action.
|
36
|
+
email:
|
37
|
+
- emilio2hd@gmail.com
|
38
|
+
executables: []
|
39
|
+
extensions: []
|
40
|
+
extra_rdoc_files: []
|
41
|
+
files:
|
42
|
+
- CHANGELOG.md
|
43
|
+
- LICENSE
|
44
|
+
- README.md
|
45
|
+
- lib/pronto/annotations_formatter.rb
|
46
|
+
- lib/pronto/annotations_formatter/version.rb
|
47
|
+
homepage: https://github.com/emilio2hd/pronto-annotations_formatter
|
48
|
+
licenses:
|
49
|
+
- MIT
|
50
|
+
metadata:
|
51
|
+
changelog_uri: https://github.com/emilio2hd/pronto-annotations_formatter/blob/main/CHANGELOG.md
|
52
|
+
rubygems_mfa_required: 'true'
|
53
|
+
post_install_message:
|
54
|
+
rdoc_options: []
|
55
|
+
require_paths:
|
56
|
+
- lib
|
57
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
58
|
+
requirements:
|
59
|
+
- - ">="
|
60
|
+
- !ruby/object:Gem::Version
|
61
|
+
version: '2.7'
|
62
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
63
|
+
requirements:
|
64
|
+
- - ">="
|
65
|
+
- !ruby/object:Gem::Version
|
66
|
+
version: '0'
|
67
|
+
requirements: []
|
68
|
+
rubygems_version: 3.2.33
|
69
|
+
signing_key:
|
70
|
+
specification_version: 4
|
71
|
+
summary: A gem to print out the warnings in a json format to be consumed by pronto-annotate-action.
|
72
|
+
test_files: []
|