notorious 0.1.0
Sign up to get free protection for your applications and to get access to all the features.
- data/Gemfile +3 -0
- data/LICENSE +22 -0
- data/Makefile +5 -0
- data/README.md +98 -0
- data/Rakefile +2 -0
- data/bin/notorious +73 -0
- data/lib/notorious.rb +136 -0
- data/lib/notorious/version.rb +3 -0
- data/notorious.gemspec +21 -0
- data/spec/examples/example.png +0 -0
- data/spec/examples/example_notes.html +206 -0
- data/spec/examples/example_notes.md +84 -0
- data/spec/examples/notorious_spec.rb +93 -0
- data/spec/examples/spec_helper.rb +3 -0
- data/stylesheets/default.css +107 -0
- metadata +99 -0
data/Gemfile
ADDED
data/LICENSE
ADDED
@@ -0,0 +1,22 @@
|
|
1
|
+
Copyright (c) 2012 Zack Reneau-Wedeen
|
2
|
+
|
3
|
+
MIT License
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
6
|
+
a copy of this software and associated documentation files (the
|
7
|
+
"Software"), to deal in the Software without restriction, including
|
8
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
9
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
10
|
+
permit persons to whom the Software is furnished to do so, subject to
|
11
|
+
the following conditions:
|
12
|
+
|
13
|
+
The above copyright notice and this permission notice shall be
|
14
|
+
included in all copies or substantial portions of the Software.
|
15
|
+
|
16
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
17
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
18
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
19
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
20
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
21
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
22
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/Makefile
ADDED
data/README.md
ADDED
@@ -0,0 +1,98 @@
|
|
1
|
+
# Notorious
|
2
|
+
|
3
|
+
Notorious allows you to take notes in simple markdown, and then build them
|
4
|
+
into a stylish, easy-to-study outline in the browser.
|
5
|
+
|
6
|
+
## Installation
|
7
|
+
|
8
|
+
Add this line to your application's Gemfile:
|
9
|
+
|
10
|
+
gem 'notorious'
|
11
|
+
|
12
|
+
And then execute:
|
13
|
+
|
14
|
+
$ bundle
|
15
|
+
|
16
|
+
Or install it yourself as:
|
17
|
+
|
18
|
+
$ gem install notorious
|
19
|
+
|
20
|
+
## Usage
|
21
|
+
|
22
|
+
###Take notes in a file:
|
23
|
+
<!-- notes.md -->
|
24
|
+
|
25
|
+
# U.S. History Class
|
26
|
+
|
27
|
+
## George Washington
|
28
|
+
|
29
|
+
First prez; tall dude. George Washington (February 22, 1732 – December 14, 1799)
|
30
|
+
was one of the Founding Fathers of the United States, serving as the
|
31
|
+
commander-in-chief of the Continental Army during the American Revolutionary
|
32
|
+
War and later as the new republic's first President.
|
33
|
+

|
34
|
+
|
35
|
+
## Ben Franklin
|
36
|
+
|
37
|
+
Smart guy; efficient planner. Benjamin Franklin (January 17, 1706 - April 17, 1790)
|
38
|
+
was one of the Founding Fathers of the United States. A noted polymath,
|
39
|
+
Franklin was a leading author, printer, political theorist, politician, postmaster,
|
40
|
+
scientist, musician, inventor, satirist, civic activist, statesman, and
|
41
|
+
diplomat. As a scientist, he was a major figure in the American
|
42
|
+
Enlightenment and the history of physics for his discoveries and theories
|
43
|
+
regarding electricity. He invented the lightning rod, bifocals, the
|
44
|
+
Franklin stove, a carriage odometer, and the glass 'armonica'.[1] He
|
45
|
+
facilitated many civic organizations, including a fire department and a university.
|
46
|
+
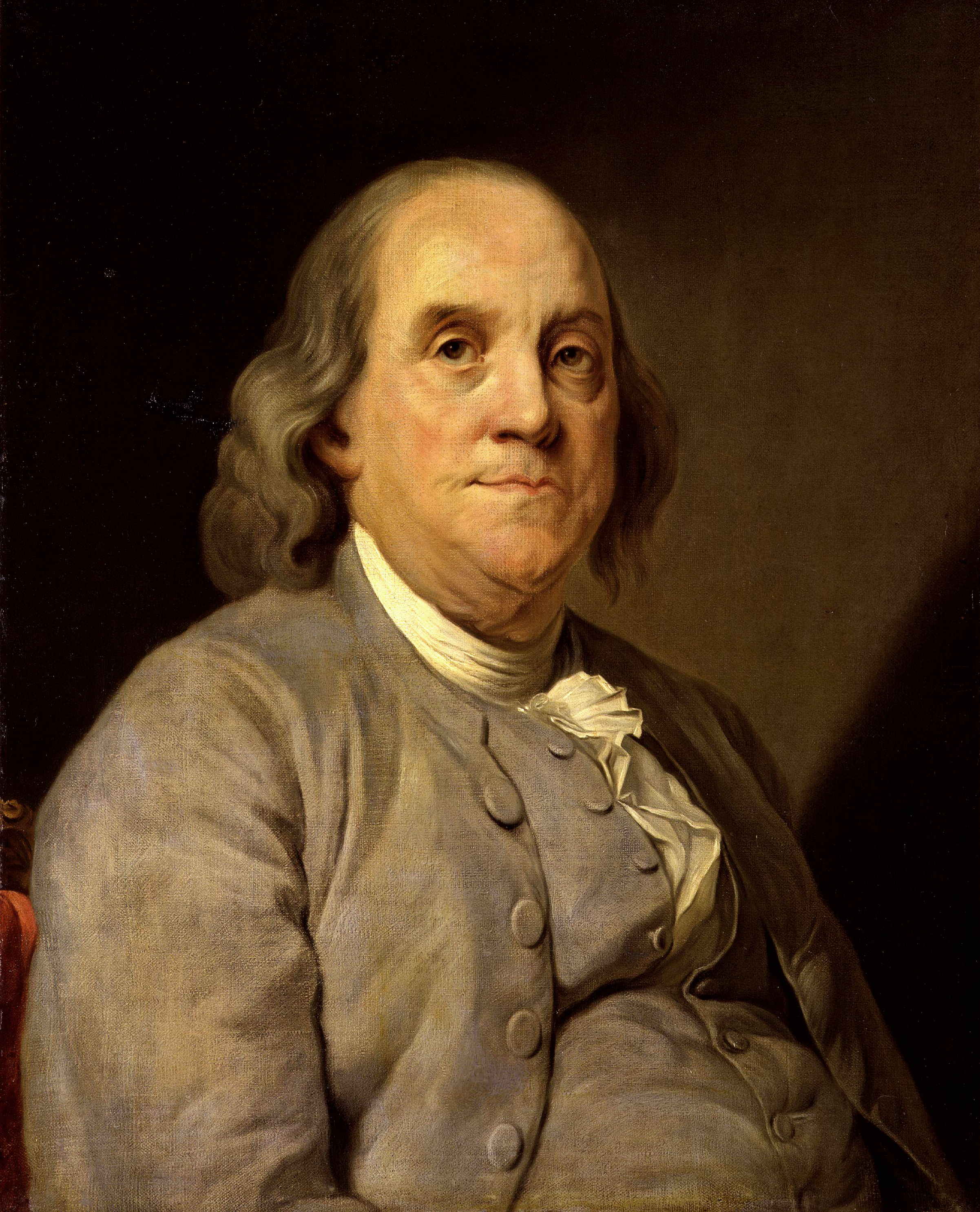
|
47
|
+
|
48
|
+
## U.S. States
|
49
|
+
### [Map](http://www.mapsofworld.com/usa/usa-maps/usa-state-capital-map.jpg)
|
50
|
+
|
51
|
+
* There were originally 13 colonies.
|
52
|
+
* Now there are 50 states
|
53
|
+
* Alabama - Montgomery
|
54
|
+
* Alaska - Juneau
|
55
|
+
* etc..
|
56
|
+
|
57
|
+
###Then call notorious
|
58
|
+
|
59
|
+
$ notorious build notes.md
|
60
|
+
|
61
|
+
###And see your fancy notes
|
62
|
+
|
63
|
+
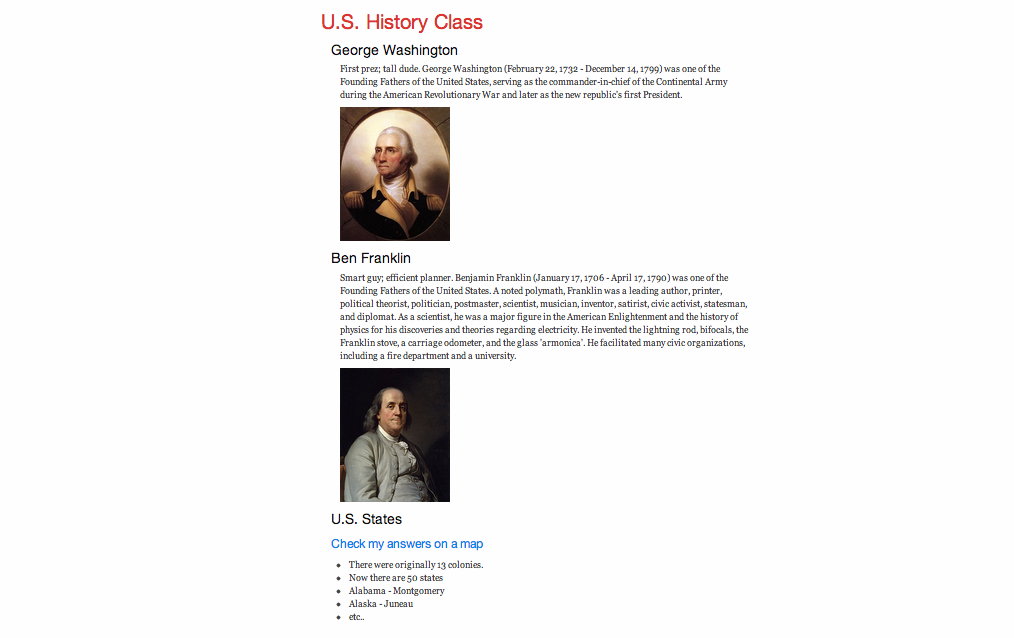
|
64
|
+
|
65
|
+
###To run this example
|
66
|
+
|
67
|
+
$ notorious example
|
68
|
+
|
69
|
+
###If notorious can't open your notes
|
70
|
+
When you call notorious build, notorious creates an html file (by default the same
|
71
|
+
directory as the markdown file). If notorious cannot open your newly made notes,
|
72
|
+
notorious will output their location, and you may open the file on your own in
|
73
|
+
your favorite browser.
|
74
|
+
|
75
|
+
|
76
|
+
###Options
|
77
|
+
|
78
|
+
$ notorious --help
|
79
|
+
Usage: notorious [options] [action] file
|
80
|
+
|
81
|
+
actions:
|
82
|
+
build [file] The markdown file to build.
|
83
|
+
|
84
|
+
options:
|
85
|
+
-o, --output [file] Specify output file name (defaults to input_file.html)
|
86
|
+
-s, --stylesheet [file] Specify a custom stylesheet
|
87
|
+
-v, --verbose Run verbosely
|
88
|
+
|
89
|
+
###Testing
|
90
|
+
$ make test
|
91
|
+
|
92
|
+
## Contributing
|
93
|
+
|
94
|
+
1. Fork it
|
95
|
+
2. Create your feature branch (`git checkout -b my-new-feature`)
|
96
|
+
3. Commit your changes (`git commit -am 'Added some feature'`)
|
97
|
+
4. Push to the branch (`git push origin my-new-feature`)
|
98
|
+
5. Create new Pull Request
|
data/Rakefile
ADDED
data/bin/notorious
ADDED
@@ -0,0 +1,73 @@
|
|
1
|
+
#! /usr/bin/env ruby
|
2
|
+
|
3
|
+
require 'notorious'
|
4
|
+
require 'optparse'
|
5
|
+
|
6
|
+
def parse
|
7
|
+
options = {}
|
8
|
+
|
9
|
+
opts = OptionParser.new do |opts|
|
10
|
+
opts.banner = "Usage: notorious [options] [action] file"
|
11
|
+
opts.separator ""
|
12
|
+
opts.separator "actions:"
|
13
|
+
opts.separator " build [file] The markdown file to build."
|
14
|
+
|
15
|
+
opts.separator ""
|
16
|
+
opts.separator "options:"
|
17
|
+
|
18
|
+
opts.on("-o", "--output [file]", String,
|
19
|
+
"Specify output file name (defaults to input_file.html)") do |o|
|
20
|
+
o = Notorious.ensure_extension(o, "html")
|
21
|
+
options[:output] = o
|
22
|
+
end
|
23
|
+
|
24
|
+
opts.on("-s", "--stylesheet [file]", String,
|
25
|
+
"Specify a custom stylesheet") do |s|
|
26
|
+
s = Notorious.ensure_extension(s, "css")
|
27
|
+
options[:stylesheet] = s
|
28
|
+
end
|
29
|
+
|
30
|
+
opts.on("-v", "--verbose", "Run verbosely") do |v|
|
31
|
+
options[:verbose] = v
|
32
|
+
end
|
33
|
+
|
34
|
+
end
|
35
|
+
opts.parse!
|
36
|
+
options
|
37
|
+
end
|
38
|
+
|
39
|
+
# Parse options
|
40
|
+
options = parse
|
41
|
+
|
42
|
+
# assign arguments
|
43
|
+
unless ARGV[0]
|
44
|
+
puts "Notorious. Run `notorious --help` for details"
|
45
|
+
end
|
46
|
+
|
47
|
+
if ARGV[0] == 'build' && !ARGV[1]
|
48
|
+
raise(ArgumentError, "you must specify a file to build")
|
49
|
+
end
|
50
|
+
|
51
|
+
case ARGV[0]
|
52
|
+
when 'build'
|
53
|
+
options[:file_name] = ARGV[1] || raise(ArgumentError, "you must specify a file to build")
|
54
|
+
when 'example'
|
55
|
+
options[:file_name] = File.expand_path("../../spec/examples/example_notes.md", __FILE__)
|
56
|
+
else
|
57
|
+
raise ArgumentError, "you have specified an invalid command"
|
58
|
+
end
|
59
|
+
|
60
|
+
# must be a markdown file
|
61
|
+
Notorious.validate_extension(options[:file_name], ["md", "mdown", "markdown", "Markdown"])
|
62
|
+
|
63
|
+
options[:stylesheet] ||= File.expand_path("../../stylesheets/default.css", __FILE__)
|
64
|
+
|
65
|
+
unless options[:output]
|
66
|
+
split = options[:file_name].split('.')
|
67
|
+
split.pop if split.length > 1
|
68
|
+
options[:output] = split.push('html').join('.')
|
69
|
+
end
|
70
|
+
|
71
|
+
|
72
|
+
# Decide what to call
|
73
|
+
Notorious.build(options)
|
data/lib/notorious.rb
ADDED
@@ -0,0 +1,136 @@
|
|
1
|
+
require "notorious/version"
|
2
|
+
require "optparse"
|
3
|
+
require "redcarpet"
|
4
|
+
|
5
|
+
|
6
|
+
|
7
|
+
module Notorious
|
8
|
+
|
9
|
+
def self.build(opts)
|
10
|
+
file_name = opts[:file_name]
|
11
|
+
stylesheet = opts[:stylesheet]
|
12
|
+
output = opts[:output]
|
13
|
+
verbose = opts[:verbose]
|
14
|
+
|
15
|
+
|
16
|
+
# make the html
|
17
|
+
html = self.render(file_name, stylesheet)
|
18
|
+
|
19
|
+
# write to the output file
|
20
|
+
outfile = File.new(File.expand_path(output), 'w')
|
21
|
+
outfile.write(html)
|
22
|
+
outfile.close
|
23
|
+
|
24
|
+
if verbose
|
25
|
+
puts "your notes are at #{output}. Attempting to open them with a web browser"
|
26
|
+
end
|
27
|
+
|
28
|
+
# try to open them
|
29
|
+
begin
|
30
|
+
`open #{output}`
|
31
|
+
rescue
|
32
|
+
puts "your notes are at #{output}. Please open them with a web browser"
|
33
|
+
end
|
34
|
+
end
|
35
|
+
|
36
|
+
def self.render(file_name, stylesheet)
|
37
|
+
begin
|
38
|
+
styles = File.new(stylesheet, 'r').read
|
39
|
+
rescue
|
40
|
+
raise RuntimeError, "Could not load the stylesheet. Make sure there is a file at #{stylesheet}"
|
41
|
+
end
|
42
|
+
|
43
|
+
# read in and convert the markdown file
|
44
|
+
begin
|
45
|
+
md_file = File.new(file_name, 'r')
|
46
|
+
rescue
|
47
|
+
raise ArgumentError, "Could not find the file you specified: #{file_name}"
|
48
|
+
end
|
49
|
+
|
50
|
+
# convert markdown to HTML
|
51
|
+
md_contents = md_file.read
|
52
|
+
md = Redcarpet::Markdown.new(Redcarpet::Render::HTML)
|
53
|
+
body = md.render(md_contents)
|
54
|
+
md_file.close
|
55
|
+
|
56
|
+
self.html(styles, body)
|
57
|
+
end
|
58
|
+
|
59
|
+
def self.html(styles, body)
|
60
|
+
|
61
|
+
<<HEREDOC
|
62
|
+
<!DOCTYPE html>
|
63
|
+
<html>
|
64
|
+
<head>
|
65
|
+
<style type='text/css'>
|
66
|
+
#{styles}
|
67
|
+
</style>
|
68
|
+
<title>Tech Notes</title>
|
69
|
+
</head>
|
70
|
+
<body>
|
71
|
+
#{body}
|
72
|
+
</body>
|
73
|
+
</html>
|
74
|
+
HEREDOC
|
75
|
+
|
76
|
+
end
|
77
|
+
|
78
|
+
def self.parse
|
79
|
+
|
80
|
+
options = {}
|
81
|
+
|
82
|
+
opts = OptionParser.new do |opts|
|
83
|
+
opts.banner = "Usage: notorious command file [options]"
|
84
|
+
opts.separator ""
|
85
|
+
opts.separator "Specific options:"
|
86
|
+
|
87
|
+
opts.on("-o", "--output [file_name]", String,
|
88
|
+
"Specify output file name (defaults to input_file_name.html") do |o|
|
89
|
+
o = self.ensure_extension(o, "html")
|
90
|
+
options[:output] = o
|
91
|
+
end
|
92
|
+
|
93
|
+
opts.on("-s", "--stylesheet [file_name]", String,
|
94
|
+
"Specify a custom stylesheet") do |s|
|
95
|
+
s = self.ensure_extension(s, "css")
|
96
|
+
options[:stylesheet] = s
|
97
|
+
end
|
98
|
+
|
99
|
+
opts.on("-v", "--verbose", "Run verbosely") do |v|
|
100
|
+
options[:verbose] = v
|
101
|
+
end
|
102
|
+
|
103
|
+
end
|
104
|
+
opts.parse!
|
105
|
+
|
106
|
+
options
|
107
|
+
end
|
108
|
+
|
109
|
+
def self.ensure_extension(file, ext)
|
110
|
+
split = file.split('.')
|
111
|
+
unless split.length > 1 && split[-1] == ext
|
112
|
+
file = "#{file}.#{ext}"
|
113
|
+
end
|
114
|
+
file
|
115
|
+
end
|
116
|
+
|
117
|
+
def self.validate_extension(file, ext)
|
118
|
+
split = file.split('.')
|
119
|
+
unless split.length > 1 && ext.include?(split[-1])
|
120
|
+
raise ArgumentError, "Input must have extension in #{ext}"
|
121
|
+
end
|
122
|
+
end
|
123
|
+
|
124
|
+
end
|
125
|
+
|
126
|
+
|
127
|
+
|
128
|
+
|
129
|
+
|
130
|
+
|
131
|
+
|
132
|
+
|
133
|
+
|
134
|
+
|
135
|
+
|
136
|
+
|
data/notorious.gemspec
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
# -*- encoding: utf-8 -*-
|
2
|
+
require File.expand_path('../lib/notorious/version', __FILE__)
|
3
|
+
|
4
|
+
Gem::Specification.new do |gem|
|
5
|
+
gem.authors = ["Zack Reneau-Wedeen"]
|
6
|
+
gem.email = ["z.reneau.wedeen@gmail.com"]
|
7
|
+
gem.description = %q{Notorious allows you to take notes in pure Markdown and review them in your browser.}
|
8
|
+
gem.summary = %q{Notorious, the Markdown note taker}
|
9
|
+
gem.homepage = ""
|
10
|
+
|
11
|
+
gem.files = `git ls-files`.split($\)
|
12
|
+
gem.executables = ["notorious"]
|
13
|
+
gem.test_files = gem.files.grep(%r{^(test|spec|features)/})
|
14
|
+
gem.name = "notorious"
|
15
|
+
gem.require_paths = ["lib"]
|
16
|
+
gem.version = Notorious::VERSION
|
17
|
+
|
18
|
+
gem.add_dependency("redcarpet", "~> 2.1.0")
|
19
|
+
|
20
|
+
gem.add_development_dependency 'rspec'
|
21
|
+
end
|
Binary file
|
@@ -0,0 +1,206 @@
|
|
1
|
+
<!DOCTYPE html>
|
2
|
+
<html>
|
3
|
+
<head>
|
4
|
+
<style type='text/css'>
|
5
|
+
html { font-size: 100%; overflow-y: scroll; -webkit-text-size-adjust: 100%; -ms-text-size-adjust: 100%; }
|
6
|
+
|
7
|
+
body{
|
8
|
+
color:#444;
|
9
|
+
font-family:Georgia, Palatino, 'Palatino Linotype', Times, 'Times New Roman', serif;
|
10
|
+
font-size:13px;
|
11
|
+
line-height:1.5em;
|
12
|
+
padding:1em;
|
13
|
+
margin:auto;
|
14
|
+
max-width:48em;
|
15
|
+
background:#fefefe;
|
16
|
+
}
|
17
|
+
|
18
|
+
a{ color: #08E; text-decoration:none;}
|
19
|
+
a:visited{ color: #08E; }
|
20
|
+
a:hover{ color: #C33; }
|
21
|
+
a:active{ color:#C33; }
|
22
|
+
a:focus{ outline: thin dotted; }
|
23
|
+
a:hover, a:active{ outline: 0; }
|
24
|
+
|
25
|
+
::-moz-selection{background:rgba(255,255,0,0.3);color:#000}
|
26
|
+
::selection{background:rgba(255,255,0,0.3);color:#000}
|
27
|
+
|
28
|
+
a::-moz-selection{background:rgba(255,255,0,0.3);color:#0645ad}
|
29
|
+
a::selection{background:rgba(255,255,0,0.3);color:#0645ad}
|
30
|
+
|
31
|
+
p{
|
32
|
+
margin:0.5em 1em;
|
33
|
+
}
|
34
|
+
|
35
|
+
img{
|
36
|
+
max-width:100%;
|
37
|
+
margin: 0 auto;
|
38
|
+
}
|
39
|
+
|
40
|
+
h1,h2,h3,h4,h5,h6{
|
41
|
+
font-weight:normal;
|
42
|
+
font-family:"Helvetica Neue", Helvetica, sans-serif;
|
43
|
+
color:#111;
|
44
|
+
line-height:1em;
|
45
|
+
margin-bottom: 0.5em;
|
46
|
+
}
|
47
|
+
|
48
|
+
|
49
|
+
h4,h5,h6{ font-weight: bold; }
|
50
|
+
h1{ font-size:2.5em; color: #DD3530; margin-left: -0.5em; }
|
51
|
+
h2{ font-size:1.7em; }
|
52
|
+
h3{ font-size:1.5em; }
|
53
|
+
h4{ font-size:1.2em; }
|
54
|
+
h5{ font-size:1em; }
|
55
|
+
h6{ font-size:0.9em; }
|
56
|
+
|
57
|
+
blockquote{
|
58
|
+
color:#666666;
|
59
|
+
margin:0;
|
60
|
+
padding-left: 3em;
|
61
|
+
border-left: 0.5em #EEE solid;
|
62
|
+
}
|
63
|
+
hr { display: block; height: 2px; border: 0; border-top: 1px solid #aaa;border-bottom: 1px solid #eee; margin: 1em 0; padding: 0; }
|
64
|
+
pre, code, kbd, samp { color: #000; font-family: monospace, monospace; _font-family: 'courier new', monospace; font-size: 0.98em; }
|
65
|
+
pre { white-space: pre; white-space: pre-wrap; word-wrap: break-word; }
|
66
|
+
|
67
|
+
b, strong { font-weight: bold; }
|
68
|
+
|
69
|
+
dfn { font-style: italic; }
|
70
|
+
|
71
|
+
ins { background: #ff9; color: #000; text-decoration: none; }
|
72
|
+
|
73
|
+
mark { background: #ff0; color: #000; font-style: italic; font-weight: bold; }
|
74
|
+
|
75
|
+
sub, sup { font-size: 75%; line-height: 0; position: relative; vertical-align: baseline; }
|
76
|
+
sup { top: -0.5em; }
|
77
|
+
sub { bottom: -0.25em; }
|
78
|
+
|
79
|
+
ul, ol { margin: 1em 0; padding: 0 0 0 2em; }
|
80
|
+
li p:last-child { margin:0 }
|
81
|
+
dd { margin: 0 0 0 2em; }
|
82
|
+
|
83
|
+
img { border: 0; -ms-interpolation-mode: bicubic; vertical-align: middle; }
|
84
|
+
|
85
|
+
table { border-collapse: collapse; border-spacing: 0; }
|
86
|
+
td { vertical-align: top; }
|
87
|
+
|
88
|
+
@media only screen and (min-width: 480px) {
|
89
|
+
body{font-size:14px;}
|
90
|
+
}
|
91
|
+
|
92
|
+
@media only screen and (min-width: 768px) {
|
93
|
+
body{font-size:16px;}
|
94
|
+
}
|
95
|
+
|
96
|
+
@media print {
|
97
|
+
* { background: transparent !important; color: black !important; filter:none !important; -ms-filter: none !important; }
|
98
|
+
body{font-size:12pt; max-width:100%;}
|
99
|
+
a, a:visited { text-decoration: underline; }
|
100
|
+
hr { height: 1px; border:0; border-bottom:1px solid black; }
|
101
|
+
a[href]:after { content: " (" attr(href) ")"; }
|
102
|
+
abbr[title]:after { content: " (" attr(title) ")"; }
|
103
|
+
.ir a:after, a[href^="javascript:"]:after, a[href^="#"]:after { content: ""; }
|
104
|
+
pre, blockquote { border: 1px solid #999; padding-right: 1em; page-break-inside: avoid; }
|
105
|
+
tr, img { page-break-inside: avoid; }
|
106
|
+
img { max-width: 100% !important; }
|
107
|
+
@page :left { margin: 15mm 20mm 15mm 10mm; }
|
108
|
+
@page :right { margin: 15mm 10mm 15mm 20mm; }
|
109
|
+
p, h2, h3 { orphans: 3; widows: 3; }
|
110
|
+
h2, h3 { page-break-after: avoid; }
|
111
|
+
}
|
112
|
+
|
113
|
+
</style>
|
114
|
+
<title>Tech Notes</title>
|
115
|
+
</head>
|
116
|
+
<body>
|
117
|
+
<!-- notes.md -->
|
118
|
+
|
119
|
+
<h1>U.S. History Class</h1>
|
120
|
+
|
121
|
+
<h2>George Washington</h2>
|
122
|
+
|
123
|
+
<p>First prez; tall dude. George Washington (February 22, 1732 - December 14, 1799)
|
124
|
+
was one of the Founding Fathers of the United States, serving as the
|
125
|
+
commander-in-chief of the Continental Army during the American Revolutionary
|
126
|
+
War and later as the new republic's first President.</p>
|
127
|
+
|
128
|
+
<p><img src="http://upload.wikimedia.org/wikipedia/commons/thumb/8/88/Portrait_of_George_Washington.jpeg/220px-Portrait_of_George_Washington.jpeg" alt="Pic of George"></p>
|
129
|
+
|
130
|
+
<h2>Ben Franklin</h2>
|
131
|
+
|
132
|
+
<p>Smart guy; efficient planner. Benjamin Franklin (January 17, 1706 - April 17, 1790)
|
133
|
+
was one of the Founding Fathers of the United States. A noted polymath,
|
134
|
+
Franklin was a leading author, printer, political theorist, politician, postmaster,
|
135
|
+
scientist, musician, inventor, satirist, civic activist, statesman, and
|
136
|
+
diplomat. As a scientist, he was a major figure in the American
|
137
|
+
Enlightenment and the history of physics for his discoveries and theories
|
138
|
+
regarding electricity. He invented the lightning rod, bifocals, the
|
139
|
+
Franklin stove, a carriage odometer, and the glass 'armonica'. He
|
140
|
+
facilitated many civic organizations, including a fire department and a university.</p>
|
141
|
+
|
142
|
+
<p><img src="http://upload.wikimedia.org/wikipedia/commons/thumb/c/cc/BenFranklinDuplessis.jpg/220px-BenFranklinDuplessis.jpg" alt="Pic of Ben"></p>
|
143
|
+
|
144
|
+
<h2>U.S. States</h2>
|
145
|
+
|
146
|
+
<h3><a href="http://www.mapsofworld.com/usa/usa-maps/usa-state-capital-map.jpg">Check my answers on a map</a></h3>
|
147
|
+
|
148
|
+
<ul>
|
149
|
+
<li>There were originally 13 colonies.</li>
|
150
|
+
<li>Now there are 50 states</li>
|
151
|
+
<li>Alabama - Montgomery</li>
|
152
|
+
<li>Alaska - Juneau</li>
|
153
|
+
<li>Arizona - Phoenix</li>
|
154
|
+
<li>Arkansas - Little Rock</li>
|
155
|
+
<li>California - Sacramento</li>
|
156
|
+
<li>Colorado - Denver</li>
|
157
|
+
<li>Connecticut - Hartford</li>
|
158
|
+
<li>Delaware - Dover</li>
|
159
|
+
<li>Florida - Tallahassee</li>
|
160
|
+
<li>Georgia - Atlanta</li>
|
161
|
+
<li>Hawaii - Honolulu</li>
|
162
|
+
<li>Idaho - Boise</li>
|
163
|
+
<li>Illinois - Springfield</li>
|
164
|
+
<li>Indiana - Indianapolis</li>
|
165
|
+
<li>Iowa - Des Moines</li>
|
166
|
+
<li>Kansas - Topeka</li>
|
167
|
+
<li>Kentucky - Frankfort</li>
|
168
|
+
<li>Louisiana - Baton Rouge</li>
|
169
|
+
<li>Maine - Augusta</li>
|
170
|
+
<li>Maryland - Annapolis</li>
|
171
|
+
<li>Massachusetts - Boston</li>
|
172
|
+
<li>Michigan - Lansing</li>
|
173
|
+
<li>Minnesota - St. Paul</li>
|
174
|
+
<li>Mississippi - Jackson</li>
|
175
|
+
<li>Missouri - Jefferson City</li>
|
176
|
+
<li>Montana - Helena</li>
|
177
|
+
<li>Nebraska - Lincoln</li>
|
178
|
+
<li>Nevada - Carson City</li>
|
179
|
+
<li>New Hampshire - Concord</li>
|
180
|
+
<li>New Jersey - Trenton</li>
|
181
|
+
<li>New Mexico - Santa Fe</li>
|
182
|
+
<li>New York - Albany</li>
|
183
|
+
<li>North Carolina - Raleigh</li>
|
184
|
+
<li>North Dakota - Bismarck</li>
|
185
|
+
<li>Ohio - Columbus</li>
|
186
|
+
<li>Oklahoma - Oklahoma City</li>
|
187
|
+
<li>Oregon - Salem</li>
|
188
|
+
<li>Pennsylvania - Harrisburg</li>
|
189
|
+
<li>Rhode Island - Providence</li>
|
190
|
+
<li>South Carolina - Columbia</li>
|
191
|
+
<li>South Dakota - Pierre</li>
|
192
|
+
<li>Tennessee - Nashville</li>
|
193
|
+
<li>Texas - Austin</li>
|
194
|
+
<li>Utah - Salt Lake City</li>
|
195
|
+
<li>Vermont - Montpelier</li>
|
196
|
+
<li>Virginia - Richmond</li>
|
197
|
+
<li>Washington - Olympia</li>
|
198
|
+
<li>West Virginia - Charleston</li>
|
199
|
+
<li>Wisconsin - Madison</li>
|
200
|
+
<li>Wyoming - Cheyenne</li>
|
201
|
+
</ul>
|
202
|
+
|
203
|
+
<p><strong>time for a nap..</strong> </p>
|
204
|
+
|
205
|
+
</body>
|
206
|
+
</html>
|
@@ -0,0 +1,84 @@
|
|
1
|
+
<!-- notes.md -->
|
2
|
+
|
3
|
+
# U.S. History Class
|
4
|
+
|
5
|
+
## George Washington
|
6
|
+
|
7
|
+
First prez; tall dude. George Washington (February 22, 1732 - December 14, 1799)
|
8
|
+
was one of the Founding Fathers of the United States, serving as the
|
9
|
+
commander-in-chief of the Continental Army during the American Revolutionary
|
10
|
+
War and later as the new republic's first President.
|
11
|
+
|
12
|
+

|
13
|
+
|
14
|
+
## Ben Franklin
|
15
|
+
|
16
|
+
Smart guy; efficient planner. Benjamin Franklin (January 17, 1706 - April 17, 1790)
|
17
|
+
was one of the Founding Fathers of the United States. A noted polymath,
|
18
|
+
Franklin was a leading author, printer, political theorist, politician, postmaster,
|
19
|
+
scientist, musician, inventor, satirist, civic activist, statesman, and
|
20
|
+
diplomat. As a scientist, he was a major figure in the American
|
21
|
+
Enlightenment and the history of physics for his discoveries and theories
|
22
|
+
regarding electricity. He invented the lightning rod, bifocals, the
|
23
|
+
Franklin stove, a carriage odometer, and the glass 'armonica'. He
|
24
|
+
facilitated many civic organizations, including a fire department and a university.
|
25
|
+
|
26
|
+

|
27
|
+
|
28
|
+
## U.S. States
|
29
|
+
### [Check my answers on a map](http://www.mapsofworld.com/usa/usa-maps/usa-state-capital-map.jpg)
|
30
|
+
|
31
|
+
* There were originally 13 colonies.
|
32
|
+
* Now there are 50 states
|
33
|
+
* Alabama - Montgomery
|
34
|
+
* Alaska - Juneau
|
35
|
+
* Arizona - Phoenix
|
36
|
+
* Arkansas - Little Rock
|
37
|
+
* California - Sacramento
|
38
|
+
* Colorado - Denver
|
39
|
+
* Connecticut - Hartford
|
40
|
+
* Delaware - Dover
|
41
|
+
* Florida - Tallahassee
|
42
|
+
* Georgia - Atlanta
|
43
|
+
* Hawaii - Honolulu
|
44
|
+
* Idaho - Boise
|
45
|
+
* Illinois - Springfield
|
46
|
+
* Indiana - Indianapolis
|
47
|
+
* Iowa - Des Moines
|
48
|
+
* Kansas - Topeka
|
49
|
+
* Kentucky - Frankfort
|
50
|
+
* Louisiana - Baton Rouge
|
51
|
+
* Maine - Augusta
|
52
|
+
* Maryland - Annapolis
|
53
|
+
* Massachusetts - Boston
|
54
|
+
* Michigan - Lansing
|
55
|
+
* Minnesota - St. Paul
|
56
|
+
* Mississippi - Jackson
|
57
|
+
* Missouri - Jefferson City
|
58
|
+
* Montana - Helena
|
59
|
+
* Nebraska - Lincoln
|
60
|
+
* Nevada - Carson City
|
61
|
+
* New Hampshire - Concord
|
62
|
+
* New Jersey - Trenton
|
63
|
+
* New Mexico - Santa Fe
|
64
|
+
* New York - Albany
|
65
|
+
* North Carolina - Raleigh
|
66
|
+
* North Dakota - Bismarck
|
67
|
+
* Ohio - Columbus
|
68
|
+
* Oklahoma - Oklahoma City
|
69
|
+
* Oregon - Salem
|
70
|
+
* Pennsylvania - Harrisburg
|
71
|
+
* Rhode Island - Providence
|
72
|
+
* South Carolina - Columbia
|
73
|
+
* South Dakota - Pierre
|
74
|
+
* Tennessee - Nashville
|
75
|
+
* Texas - Austin
|
76
|
+
* Utah - Salt Lake City
|
77
|
+
* Vermont - Montpelier
|
78
|
+
* Virginia - Richmond
|
79
|
+
* Washington - Olympia
|
80
|
+
* West Virginia - Charleston
|
81
|
+
* Wisconsin - Madison
|
82
|
+
* Wyoming - Cheyenne
|
83
|
+
|
84
|
+
**time for a nap..**
|
@@ -0,0 +1,93 @@
|
|
1
|
+
require File.expand_path('../spec_helper', __FILE__)
|
2
|
+
|
3
|
+
describe 'Notorious' do
|
4
|
+
|
5
|
+
context 'running the program' do
|
6
|
+
end
|
7
|
+
|
8
|
+
context '#ensure_extension' do
|
9
|
+
it 'should not change correctly extended files' do
|
10
|
+
Notorious.ensure_extension('correct.html', 'html')
|
11
|
+
.should == 'correct.html'
|
12
|
+
end
|
13
|
+
|
14
|
+
it 'should abide multiple extensions' do
|
15
|
+
Notorious.ensure_extension('correct.other.html', 'html')
|
16
|
+
.should == 'correct.other.html'
|
17
|
+
end
|
18
|
+
|
19
|
+
it 'should add the extension if there is none' do
|
20
|
+
Notorious.ensure_extension('wrong', 'txt')
|
21
|
+
.should == 'wrong.txt'
|
22
|
+
end
|
23
|
+
|
24
|
+
it 'should add the extension if it is wrong' do
|
25
|
+
Notorious.ensure_extension('wrong.html', 'erb')
|
26
|
+
.should == 'wrong.html.erb'
|
27
|
+
end
|
28
|
+
|
29
|
+
end
|
30
|
+
|
31
|
+
context '#validate_extension' do
|
32
|
+
|
33
|
+
it 'should accept correctly extended files' do
|
34
|
+
Notorious.validate_extension('correct.html', ['html']).should be_nil
|
35
|
+
end
|
36
|
+
|
37
|
+
it 'should abide multiple extensions' do
|
38
|
+
Notorious.validate_extension('correct.other.html', ['html']).should be_nil
|
39
|
+
end
|
40
|
+
|
41
|
+
it 'should abide multiple extensions' do
|
42
|
+
Notorious.validate_extension('correct.other.html', ['html', 'htm']).should be_nil
|
43
|
+
Notorious.validate_extension('correct.other.htm', ['html', 'htm']).should be_nil
|
44
|
+
end
|
45
|
+
|
46
|
+
it 'should reject no extension' do
|
47
|
+
expect {
|
48
|
+
Notorious.validate_extension('wrong', ['txt'])
|
49
|
+
}.to raise_error
|
50
|
+
end
|
51
|
+
|
52
|
+
it 'should reject a wrong extension' do
|
53
|
+
expect {
|
54
|
+
Notorious.validate_extension('wrong.tx', ['txt'])
|
55
|
+
}.to raise_error
|
56
|
+
end
|
57
|
+
|
58
|
+
it 'should only care about the last extension' do
|
59
|
+
expect {
|
60
|
+
Notorious.validate_extension('wrong.html.erb', ['html'])
|
61
|
+
}.to raise_error
|
62
|
+
end
|
63
|
+
|
64
|
+
it 'should work on multiple extensions' do
|
65
|
+
expect {
|
66
|
+
Notorious.validate_extension('wrong.html.erb', ['html', 'htm'])
|
67
|
+
}.to raise_error
|
68
|
+
end
|
69
|
+
end
|
70
|
+
|
71
|
+
context 'render' do
|
72
|
+
context 'bad input' do
|
73
|
+
|
74
|
+
end
|
75
|
+
end
|
76
|
+
|
77
|
+
|
78
|
+
|
79
|
+
end
|
80
|
+
|
81
|
+
|
82
|
+
|
83
|
+
|
84
|
+
|
85
|
+
|
86
|
+
|
87
|
+
|
88
|
+
|
89
|
+
|
90
|
+
|
91
|
+
|
92
|
+
|
93
|
+
|
@@ -0,0 +1,107 @@
|
|
1
|
+
html { font-size: 100%; overflow-y: scroll; -webkit-text-size-adjust: 100%; -ms-text-size-adjust: 100%; }
|
2
|
+
|
3
|
+
body{
|
4
|
+
color:#444;
|
5
|
+
font-family:Georgia, Palatino, 'Palatino Linotype', Times, 'Times New Roman', serif;
|
6
|
+
font-size:13px;
|
7
|
+
line-height:1.5em;
|
8
|
+
padding:1em;
|
9
|
+
margin:auto;
|
10
|
+
max-width:48em;
|
11
|
+
background:#fefefe;
|
12
|
+
}
|
13
|
+
|
14
|
+
a{ color: #08E; text-decoration:none;}
|
15
|
+
a:visited{ color: #08E; }
|
16
|
+
a:hover{ color: #C33; }
|
17
|
+
a:active{ color:#C33; }
|
18
|
+
a:focus{ outline: thin dotted; }
|
19
|
+
a:hover, a:active{ outline: 0; }
|
20
|
+
|
21
|
+
::-moz-selection{background:rgba(255,255,0,0.3);color:#000}
|
22
|
+
::selection{background:rgba(255,255,0,0.3);color:#000}
|
23
|
+
|
24
|
+
a::-moz-selection{background:rgba(255,255,0,0.3);color:#0645ad}
|
25
|
+
a::selection{background:rgba(255,255,0,0.3);color:#0645ad}
|
26
|
+
|
27
|
+
p{
|
28
|
+
margin:0.5em 1em;
|
29
|
+
}
|
30
|
+
|
31
|
+
img{
|
32
|
+
max-width:100%;
|
33
|
+
margin: 0 auto;
|
34
|
+
}
|
35
|
+
|
36
|
+
h1,h2,h3,h4,h5,h6{
|
37
|
+
font-weight:normal;
|
38
|
+
font-family:"Helvetica Neue", Helvetica, sans-serif;
|
39
|
+
color:#111;
|
40
|
+
line-height:1em;
|
41
|
+
margin-bottom: 0.5em;
|
42
|
+
}
|
43
|
+
|
44
|
+
|
45
|
+
h4,h5,h6{ font-weight: bold; }
|
46
|
+
h1{ font-size:2.5em; color: #DD3530; margin-left: -0.5em; }
|
47
|
+
h2{ font-size:1.7em; }
|
48
|
+
h3{ font-size:1.5em; }
|
49
|
+
h4{ font-size:1.2em; }
|
50
|
+
h5{ font-size:1em; }
|
51
|
+
h6{ font-size:0.9em; }
|
52
|
+
|
53
|
+
blockquote{
|
54
|
+
color:#666666;
|
55
|
+
margin:0;
|
56
|
+
padding-left: 3em;
|
57
|
+
border-left: 0.5em #EEE solid;
|
58
|
+
}
|
59
|
+
hr { display: block; height: 2px; border: 0; border-top: 1px solid #aaa;border-bottom: 1px solid #eee; margin: 1em 0; padding: 0; }
|
60
|
+
pre, code, kbd, samp { color: #000; font-family: monospace, monospace; _font-family: 'courier new', monospace; font-size: 0.98em; }
|
61
|
+
pre { white-space: pre; white-space: pre-wrap; word-wrap: break-word; }
|
62
|
+
|
63
|
+
b, strong { font-weight: bold; }
|
64
|
+
|
65
|
+
dfn { font-style: italic; }
|
66
|
+
|
67
|
+
ins { background: #ff9; color: #000; text-decoration: none; }
|
68
|
+
|
69
|
+
mark { background: #ff0; color: #000; font-style: italic; font-weight: bold; }
|
70
|
+
|
71
|
+
sub, sup { font-size: 75%; line-height: 0; position: relative; vertical-align: baseline; }
|
72
|
+
sup { top: -0.5em; }
|
73
|
+
sub { bottom: -0.25em; }
|
74
|
+
|
75
|
+
ul, ol { margin: 1em 0; padding: 0 0 0 2em; }
|
76
|
+
li p:last-child { margin:0 }
|
77
|
+
dd { margin: 0 0 0 2em; }
|
78
|
+
|
79
|
+
img { border: 0; -ms-interpolation-mode: bicubic; vertical-align: middle; }
|
80
|
+
|
81
|
+
table { border-collapse: collapse; border-spacing: 0; }
|
82
|
+
td { vertical-align: top; }
|
83
|
+
|
84
|
+
@media only screen and (min-width: 480px) {
|
85
|
+
body{font-size:14px;}
|
86
|
+
}
|
87
|
+
|
88
|
+
@media only screen and (min-width: 768px) {
|
89
|
+
body{font-size:16px;}
|
90
|
+
}
|
91
|
+
|
92
|
+
@media print {
|
93
|
+
* { background: transparent !important; color: black !important; filter:none !important; -ms-filter: none !important; }
|
94
|
+
body{font-size:12pt; max-width:100%;}
|
95
|
+
a, a:visited { text-decoration: underline; }
|
96
|
+
hr { height: 1px; border:0; border-bottom:1px solid black; }
|
97
|
+
a[href]:after { content: " (" attr(href) ")"; }
|
98
|
+
abbr[title]:after { content: " (" attr(title) ")"; }
|
99
|
+
.ir a:after, a[href^="javascript:"]:after, a[href^="#"]:after { content: ""; }
|
100
|
+
pre, blockquote { border: 1px solid #999; padding-right: 1em; page-break-inside: avoid; }
|
101
|
+
tr, img { page-break-inside: avoid; }
|
102
|
+
img { max-width: 100% !important; }
|
103
|
+
@page :left { margin: 15mm 20mm 15mm 10mm; }
|
104
|
+
@page :right { margin: 15mm 10mm 15mm 20mm; }
|
105
|
+
p, h2, h3 { orphans: 3; widows: 3; }
|
106
|
+
h2, h3 { page-break-after: avoid; }
|
107
|
+
}
|
metadata
ADDED
@@ -0,0 +1,99 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: notorious
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.1.0
|
5
|
+
prerelease:
|
6
|
+
platform: ruby
|
7
|
+
authors:
|
8
|
+
- Zack Reneau-Wedeen
|
9
|
+
autorequire:
|
10
|
+
bindir: bin
|
11
|
+
cert_chain: []
|
12
|
+
date: 2012-10-15 00:00:00.000000000 Z
|
13
|
+
dependencies:
|
14
|
+
- !ruby/object:Gem::Dependency
|
15
|
+
name: redcarpet
|
16
|
+
requirement: !ruby/object:Gem::Requirement
|
17
|
+
none: false
|
18
|
+
requirements:
|
19
|
+
- - ~>
|
20
|
+
- !ruby/object:Gem::Version
|
21
|
+
version: 2.1.0
|
22
|
+
type: :runtime
|
23
|
+
prerelease: false
|
24
|
+
version_requirements: !ruby/object:Gem::Requirement
|
25
|
+
none: false
|
26
|
+
requirements:
|
27
|
+
- - ~>
|
28
|
+
- !ruby/object:Gem::Version
|
29
|
+
version: 2.1.0
|
30
|
+
- !ruby/object:Gem::Dependency
|
31
|
+
name: rspec
|
32
|
+
requirement: !ruby/object:Gem::Requirement
|
33
|
+
none: false
|
34
|
+
requirements:
|
35
|
+
- - ! '>='
|
36
|
+
- !ruby/object:Gem::Version
|
37
|
+
version: '0'
|
38
|
+
type: :development
|
39
|
+
prerelease: false
|
40
|
+
version_requirements: !ruby/object:Gem::Requirement
|
41
|
+
none: false
|
42
|
+
requirements:
|
43
|
+
- - ! '>='
|
44
|
+
- !ruby/object:Gem::Version
|
45
|
+
version: '0'
|
46
|
+
description: Notorious allows you to take notes in pure Markdown and review them in
|
47
|
+
your browser.
|
48
|
+
email:
|
49
|
+
- z.reneau.wedeen@gmail.com
|
50
|
+
executables:
|
51
|
+
- notorious
|
52
|
+
extensions: []
|
53
|
+
extra_rdoc_files: []
|
54
|
+
files:
|
55
|
+
- Gemfile
|
56
|
+
- LICENSE
|
57
|
+
- Makefile
|
58
|
+
- README.md
|
59
|
+
- Rakefile
|
60
|
+
- bin/notorious
|
61
|
+
- lib/notorious.rb
|
62
|
+
- lib/notorious/version.rb
|
63
|
+
- notorious.gemspec
|
64
|
+
- spec/examples/example.png
|
65
|
+
- spec/examples/example_notes.html
|
66
|
+
- spec/examples/example_notes.md
|
67
|
+
- spec/examples/notorious_spec.rb
|
68
|
+
- spec/examples/spec_helper.rb
|
69
|
+
- stylesheets/default.css
|
70
|
+
homepage: ''
|
71
|
+
licenses: []
|
72
|
+
post_install_message:
|
73
|
+
rdoc_options: []
|
74
|
+
require_paths:
|
75
|
+
- lib
|
76
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
77
|
+
none: false
|
78
|
+
requirements:
|
79
|
+
- - ! '>='
|
80
|
+
- !ruby/object:Gem::Version
|
81
|
+
version: '0'
|
82
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
83
|
+
none: false
|
84
|
+
requirements:
|
85
|
+
- - ! '>='
|
86
|
+
- !ruby/object:Gem::Version
|
87
|
+
version: '0'
|
88
|
+
requirements: []
|
89
|
+
rubyforge_project:
|
90
|
+
rubygems_version: 1.8.24
|
91
|
+
signing_key:
|
92
|
+
specification_version: 3
|
93
|
+
summary: Notorious, the Markdown note taker
|
94
|
+
test_files:
|
95
|
+
- spec/examples/example.png
|
96
|
+
- spec/examples/example_notes.html
|
97
|
+
- spec/examples/example_notes.md
|
98
|
+
- spec/examples/notorious_spec.rb
|
99
|
+
- spec/examples/spec_helper.rb
|