neat 0.4.2
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- data/.gitignore +14 -0
- data/Gemfile +4 -0
- data/LICENSE +23 -0
- data/README.md +283 -0
- data/app/assets/stylesheets/_neat.scss +18 -0
- data/app/assets/stylesheets/functions/_grid.scss +15 -0
- data/app/assets/stylesheets/functions/_line-height.scss +0 -0
- data/app/assets/stylesheets/functions/_px-to-em.scss +3 -0
- data/app/assets/stylesheets/grid/_grid.scss +152 -0
- data/app/assets/stylesheets/settings/_grid.scss +6 -0
- data/app/assets/stylesheets/settings/_typography.scss +3 -0
- data/app/assets/stylesheets/typography/_typography.scss +4 -0
- data/lib/neat/engine.rb +5 -0
- data/lib/neat/version.rb +3 -0
- data/lib/neat.rb +15 -0
- data/lib/tasks/install.rake +23 -0
- data/neat.gemspec +29 -0
- metadata +132 -0
data/.gitignore
ADDED
data/Gemfile
ADDED
data/LICENSE
ADDED
@@ -0,0 +1,23 @@
|
|
1
|
+
LICENSE
|
2
|
+
|
3
|
+
The MIT License
|
4
|
+
|
5
|
+
Copyright (c) 2011 thoughtbot, inc.
|
6
|
+
|
7
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
8
|
+
of this software and associated documentation files (the "Software"), to deal
|
9
|
+
in the Software without restriction, including without limitation the rights
|
10
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
11
|
+
copies of the Software, and to permit persons to whom the Software is
|
12
|
+
furnished to do so, subject to the following conditions:
|
13
|
+
|
14
|
+
The above copyright notice and this permission notice shall be included in
|
15
|
+
all copies or substantial portions of the Software.
|
16
|
+
|
17
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
18
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
19
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
20
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
21
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
22
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
23
|
+
THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,283 @@
|
|
1
|
+
[](http://thoughtbot.com/neat)
|
2
|
+
|
3
|
+
**Neat** is an open source fluid grid framework built on top of [Bourbon](http://thoughtbot.com/bourbon) with the aim of being easy enough to use out of the box and flexible enough to customize down the road.
|
4
|
+
|
5
|
+
Learn more about Neat and why we built it [here](http://thoughtbot.com/neat/).
|
6
|
+
|
7
|
+
|
8
|
+
Getting started
|
9
|
+
===
|
10
|
+
|
11
|
+
Requirements:
|
12
|
+
|
13
|
+
- Sass 3.2+
|
14
|
+
- Bourbon 2.1+
|
15
|
+
|
16
|
+
|
17
|
+
Gemfile contents:
|
18
|
+
|
19
|
+
gem 'bourbon'
|
20
|
+
gem 'neat'
|
21
|
+
|
22
|
+
After running `bundle install` you will be able to use bourbon and neat together. Within your application.css.scss file place the following:
|
23
|
+
|
24
|
+
@import "bourbon";
|
25
|
+
@import "neat";
|
26
|
+
|
27
|
+
|
28
|
+
Using the grid
|
29
|
+
===
|
30
|
+
The default grid uses 12 columns (a setting that can be easily overridden as detailed further down).
|
31
|
+
|
32
|
+
### Containers
|
33
|
+
Include the ```outer-container``` mixin in the topmost container (to which the ```max-width``` setting will be applied):
|
34
|
+
|
35
|
+
div.container {
|
36
|
+
@include outer-container;
|
37
|
+
}
|
38
|
+
|
39
|
+
|
40
|
+
You can include this mixin in more than one element in the same page.
|
41
|
+
|
42
|
+
### Columns
|
43
|
+
Use the ```span-columns``` mixin to specify the number of columns an element should span:
|
44
|
+
|
45
|
+
@include span-columns($span: $columns of $container-columns, $display: block)
|
46
|
+
|
47
|
+
* ```columns``` is the number of columns you wish this element to span.
|
48
|
+
* ```container-columns``` (optional) is the number of columns the container spans, defaults to the total number of columns in the grid.
|
49
|
+
* ```display``` (optional) changes the display type of the grid. Use ```block```—the default—for floated layout, ```table``` for table-cell layout, and ```inline-block``` for an inline block layout.
|
50
|
+
|
51
|
+
eg. Element that spans across 6 columns (out of the default 12):
|
52
|
+
|
53
|
+
div.element {
|
54
|
+
@include span-columns(6);
|
55
|
+
}
|
56
|
+
|
57
|
+
|
58
|
+
If the element's parent isn't the top-most container, you need to add the number of columns of the parent element to keep the right proportions:
|
59
|
+
|
60
|
+
div.container {
|
61
|
+
@include outer-container;
|
62
|
+
|
63
|
+
div.parent-element {
|
64
|
+
@include span-columns(8);
|
65
|
+
|
66
|
+
div.element {
|
67
|
+
@include span-columns(6 of 8);
|
68
|
+
}
|
69
|
+
}
|
70
|
+
}
|
71
|
+
|
72
|
+
To use a table-cell layout, add ```table``` as the ```display``` argument:
|
73
|
+
|
74
|
+
@include span-columns(6 of 8, table)
|
75
|
+
|
76
|
+
|
77
|
+
Likewise for inline-block:
|
78
|
+
|
79
|
+
@include span-columns(6 of 8, inline-block)
|
80
|
+
|
81
|
+
The following syntaxes would also work:
|
82
|
+
|
83
|
+
@include span-columns(6 / 8,inline-block);
|
84
|
+
@include span-columns(6 8,inline-block);
|
85
|
+
|
86
|
+
|
87
|
+
### Rows
|
88
|
+
In order to clear floated or table-cell columns, use the ```row``` mixin:
|
89
|
+
|
90
|
+
@include row($display);
|
91
|
+
|
92
|
+
* ```display``` takes either ```block```—the default—or ```table```.
|
93
|
+
|
94
|
+
|
95
|
+
### Shifting columns
|
96
|
+
|
97
|
+
|
98
|
+
To move an element to the left or right by a number of columns, use the ```shift``` mixin:
|
99
|
+
|
100
|
+
@include shift(2); // Move 2 columns to the right
|
101
|
+
@include shift(-3); // Move 3 columns to the left
|
102
|
+
|
103
|
+
Please note that the ```shift()``` mixin is incompatible with display ```table```.
|
104
|
+
|
105
|
+
|
106
|
+
### Padding columns
|
107
|
+
|
108
|
+
To add padding around the entire column use ```pad()```. By default it adds the same value as the grid's gutter but can take any unit value.
|
109
|
+
|
110
|
+
@include pad; // Adds a padding equivalent to the grid's gutter
|
111
|
+
@extend pad(20px); // Adds a padding of 20px
|
112
|
+
|
113
|
+
The ```pad()``` mixin works particularly well with ```span-columns(x, y, table)``` by adding the necessary padding without breaking your table-based grid layout.
|
114
|
+
|
115
|
+
### Removing gutter
|
116
|
+
|
117
|
+
Neat automatically removes the last columns's gutter. However, if you are queueing more than one row of columns within the same parent element, you need to specify which columns are considered last in their row to preserve the layout. Use the ```omega``` mixin to achieve this:
|
118
|
+
|
119
|
+
@include omega; // Removes right gutter
|
120
|
+
|
121
|
+
You can also use ```nth-omega``` to remove the gutter of a specific column or set of columns:
|
122
|
+
|
123
|
+
@include nth-omega(nth-child);
|
124
|
+
|
125
|
+
* ```nth-child``` takes any valid :nth-child value. See [https://developer.mozilla.org/en-US/docs/CSS/:nth-child](Mozilla's :nth-child documentation)
|
126
|
+
|
127
|
+
eg. Remove every 3rd gutter using:
|
128
|
+
|
129
|
+
@include nth-omega(3n);
|
130
|
+
|
131
|
+
### Filling parent elements
|
132
|
+
|
133
|
+
This makes sure that the child fills 100% of its parent:
|
134
|
+
|
135
|
+
@include fill-parent;
|
136
|
+
|
137
|
+
### Breakpoints
|
138
|
+
|
139
|
+
The ```breakpoint()``` mixin allows you to use media-queries to modify both the grid and the layout. It takes two arguments:
|
140
|
+
|
141
|
+
@include breakpoint($query:$feature $value, $total-columns: $grid-columns)
|
142
|
+
|
143
|
+
* ```query``` contains the media feature (min-width, max-width, etc.) and the value (481px, 30em, etc.). If you specify the value only, ```min-width``` will be used by default (ideal if you follow a mobile-first approach). You can also change the default feature in the settings (see section below).
|
144
|
+
* ```total-columns``` (optional) is the total number of columns to be used inside this media query. Changing the total number of columns will change the width, padding and margin percentages obtained using the ```span-column``` mixin.
|
145
|
+
|
146
|
+
##### Example 1
|
147
|
+
|
148
|
+
.my-class {
|
149
|
+
@include breakpoint(481px) {
|
150
|
+
font-size: 1.2em;
|
151
|
+
}
|
152
|
+
}
|
153
|
+
|
154
|
+
// Compiled CSS
|
155
|
+
|
156
|
+
@media screen and (min-width: 481px) {
|
157
|
+
.my-class {
|
158
|
+
font-size: 1.2em;
|
159
|
+
}
|
160
|
+
}
|
161
|
+
|
162
|
+
##### Example 2
|
163
|
+
|
164
|
+
.my-class {
|
165
|
+
@include breakpoint(max-width 769px) {
|
166
|
+
float: none;
|
167
|
+
}
|
168
|
+
}
|
169
|
+
|
170
|
+
// Compiled CSS
|
171
|
+
|
172
|
+
@media screen and (max-width: 769px) {
|
173
|
+
.my-class {
|
174
|
+
float: none;
|
175
|
+
}
|
176
|
+
}
|
177
|
+
|
178
|
+
##### Example 3
|
179
|
+
|
180
|
+
.my-class {
|
181
|
+
@include breakpoint(max-width 769px) {
|
182
|
+
@include span-columns(6);
|
183
|
+
}
|
184
|
+
}
|
185
|
+
|
186
|
+
// Compiled CSS
|
187
|
+
|
188
|
+
@media screen and (max-width: 769px) {
|
189
|
+
.my-class {
|
190
|
+
display: block;
|
191
|
+
float: left;
|
192
|
+
margin-right: 2.35765%;
|
193
|
+
width: 48.82117%; // That's 6 columns of the total 12
|
194
|
+
}
|
195
|
+
|
196
|
+
.my-class:last-child {
|
197
|
+
margin-right: 0;
|
198
|
+
}
|
199
|
+
}
|
200
|
+
|
201
|
+
##### Example 4
|
202
|
+
|
203
|
+
.my-class {
|
204
|
+
@include breakpoint(max-width 769px, 6) { // Use a 6 column grid (instead of the default 12)
|
205
|
+
@include span-columns(6);
|
206
|
+
}
|
207
|
+
}
|
208
|
+
|
209
|
+
// Compiled CSS
|
210
|
+
|
211
|
+
@media screen and (max-width: 769px) {
|
212
|
+
.my-class {
|
213
|
+
display: block;
|
214
|
+
float: left;
|
215
|
+
margin-right: 4.82916%;
|
216
|
+
width: 100%; // That's 6 columns of the total 6 specified for this media query
|
217
|
+
}
|
218
|
+
.my-class:last-child {
|
219
|
+
margin-right: 0;
|
220
|
+
}
|
221
|
+
}
|
222
|
+
|
223
|
+
For convenience, you can create a list variable to hold your media context (breakpoint/column-count) and use it throughout your code:
|
224
|
+
|
225
|
+
$mobile: max-width 480px 4; // Use a 4 column grid in mobile devices (requires all three arguments to work)
|
226
|
+
|
227
|
+
.my-class {
|
228
|
+
@include breakpoint($mobile) {
|
229
|
+
@include span-columns(2);
|
230
|
+
}
|
231
|
+
}
|
232
|
+
|
233
|
+
// Compiled CSS
|
234
|
+
|
235
|
+
@media screen and (max-width: 480px) {
|
236
|
+
.my-class {
|
237
|
+
display: block;
|
238
|
+
float: left;
|
239
|
+
margin-right: 7.42297%;
|
240
|
+
width: 46.28851%; // 2 columns of the total 4 in this media context
|
241
|
+
}
|
242
|
+
.my-class:last-child {
|
243
|
+
margin-right: 0;
|
244
|
+
}
|
245
|
+
}
|
246
|
+
|
247
|
+
Here is a summary of possible argument combinations:
|
248
|
+
|
249
|
+
// YAY
|
250
|
+
@include breakpoint(480px);
|
251
|
+
@include breakpoint(max-width 480px);
|
252
|
+
@include breakpoint(480px, 4);
|
253
|
+
@include breakpoint(max-width 480px, 4);
|
254
|
+
@include breakpoint(max-width 480px 4); // Shorthand syntax
|
255
|
+
|
256
|
+
// NAY
|
257
|
+
@include breakpoint(480px 4);
|
258
|
+
@include breakpoint(max-width 4);
|
259
|
+
@include breakpoint(max-width, 4);
|
260
|
+
|
261
|
+
|
262
|
+
### Changing the defaults
|
263
|
+
|
264
|
+
All the default settings can be overridden, including ```$grid-columns``` and ```$max-width```. The complete list of settings can be found in the ```/settings``` subfolder. In order to override any of these settings, redefine the variable in your site-wide ```_variables.scss``` and make sure to import it *before* Neat (failing to do so will cause Neat to use the default values):
|
265
|
+
|
266
|
+
@import "bourbon/bourbon";
|
267
|
+
@import "variables";
|
268
|
+
@import "neat/neat";
|
269
|
+
|
270
|
+
|
271
|
+
Credits
|
272
|
+
===
|
273
|
+
|
274
|
+
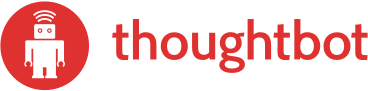
|
275
|
+
|
276
|
+
Bourbon is maintained and funded by [thoughtbot, inc](http://thoughtbot.com/community). Tweet your questions or suggestions at [Kyle](https://twitter.com/kylefiedler) / [Reda](https://twitter.com/kaishin).
|
277
|
+
|
278
|
+
License
|
279
|
+
===
|
280
|
+
|
281
|
+
Bourbon Neat is Copyright © 2012 thoughtbot. It is free software, and may be redistributed under the terms specified in the LICENSE file.
|
282
|
+
|
283
|
+
|
@@ -0,0 +1,18 @@
|
|
1
|
+
// Bourbon Neat 0.9 beta
|
2
|
+
// MIT Licensed
|
3
|
+
// Copyright (c) 2011 thoughtbot, inc.
|
4
|
+
|
5
|
+
// Custom Functions
|
6
|
+
@import "functions/line-height";
|
7
|
+
@import "functions/px-to-em";
|
8
|
+
@import "functions/grid";
|
9
|
+
|
10
|
+
// Default Settings
|
11
|
+
@import "settings/grid";
|
12
|
+
@import "settings/typography";
|
13
|
+
|
14
|
+
// The Grid
|
15
|
+
@import "grid/grid";
|
16
|
+
|
17
|
+
// Typography
|
18
|
+
@import "typography/typography";
|
@@ -0,0 +1,15 @@
|
|
1
|
+
@function container-span($span: $span) {
|
2
|
+
@if length($span) == 3 {
|
3
|
+
$container-columns: nth($span, 3);
|
4
|
+
@return $container-columns;
|
5
|
+
}
|
6
|
+
|
7
|
+
@else if length($span) == 2 {
|
8
|
+
$container-columns: nth($span, 2);
|
9
|
+
@return $container-columns;
|
10
|
+
}
|
11
|
+
|
12
|
+
@else {
|
13
|
+
@return $grid-columns;
|
14
|
+
}
|
15
|
+
}
|
File without changes
|
@@ -0,0 +1,152 @@
|
|
1
|
+
@if $border-box-sizing == true {
|
2
|
+
* {
|
3
|
+
@include box-sizing(border-box);
|
4
|
+
}
|
5
|
+
}
|
6
|
+
|
7
|
+
$fg-column: $column;
|
8
|
+
$fg-gutter: $gutter;
|
9
|
+
$fg-max-columns: $grid-columns;
|
10
|
+
$fg-max-width: $max-width;
|
11
|
+
|
12
|
+
// outer wrapper center container
|
13
|
+
@mixin outer-container() {
|
14
|
+
@include clearfix;
|
15
|
+
max-width: $fg-max-width;
|
16
|
+
text-align: left;
|
17
|
+
margin: {
|
18
|
+
left: auto;
|
19
|
+
right: auto;
|
20
|
+
}
|
21
|
+
}
|
22
|
+
|
23
|
+
// Grid span columns
|
24
|
+
@mixin span-columns($span: $columns of $container-columns, $display: block) {
|
25
|
+
|
26
|
+
$columns: nth($span, 1);
|
27
|
+
$container-columns: container-span($span);
|
28
|
+
|
29
|
+
@if $display == table {
|
30
|
+
display: table-cell;
|
31
|
+
padding-right: flex-gutter($container-columns);
|
32
|
+
width: flex-grid($columns, $container-columns) + flex-gutter($container-columns);
|
33
|
+
|
34
|
+
&:last-child {
|
35
|
+
padding-right: 0;
|
36
|
+
}
|
37
|
+
}
|
38
|
+
|
39
|
+
@else if $display == inline-block {
|
40
|
+
@include inline-block;
|
41
|
+
margin-right: flex-gutter($container-columns);
|
42
|
+
width: flex-grid($columns, $container-columns);
|
43
|
+
|
44
|
+
&:last-child {
|
45
|
+
margin-right: 0;
|
46
|
+
}
|
47
|
+
}
|
48
|
+
|
49
|
+
@else {
|
50
|
+
display: block;
|
51
|
+
float: left;
|
52
|
+
margin-right: flex-gutter($container-columns);
|
53
|
+
width: flex-grid($columns, $container-columns);
|
54
|
+
|
55
|
+
&:last-child {
|
56
|
+
margin-right: 0;
|
57
|
+
}
|
58
|
+
}
|
59
|
+
}
|
60
|
+
|
61
|
+
// Clearfix / row container
|
62
|
+
@mixin row($display: block) {
|
63
|
+
@include clearfix;
|
64
|
+
@if $display == table {
|
65
|
+
display: table;
|
66
|
+
}
|
67
|
+
|
68
|
+
@else {
|
69
|
+
display: block;
|
70
|
+
}
|
71
|
+
}
|
72
|
+
|
73
|
+
// Shift
|
74
|
+
@mixin shift($n-columns: 1) {
|
75
|
+
margin-left: $n-columns * flex-grid(1) + $n-columns * flex-gutter();
|
76
|
+
}
|
77
|
+
|
78
|
+
|
79
|
+
// Pad
|
80
|
+
@mixin pad($padding: flex-gutter()) {
|
81
|
+
padding: $padding;
|
82
|
+
}
|
83
|
+
|
84
|
+
// Remove element gutter
|
85
|
+
@mixin omega($display: block, $direction: right) {
|
86
|
+
@if $display == table {
|
87
|
+
padding-#{$direction}: 0;
|
88
|
+
}
|
89
|
+
|
90
|
+
@else {
|
91
|
+
margin-#{$direction}: 0;
|
92
|
+
}
|
93
|
+
}
|
94
|
+
|
95
|
+
@mixin nth-omega($nth, $display: block, $direction: right) {
|
96
|
+
@if $display == table {
|
97
|
+
&:nth-child(#{$nth}) {
|
98
|
+
padding-#{$direction}: 0;
|
99
|
+
}
|
100
|
+
}
|
101
|
+
|
102
|
+
@else {
|
103
|
+
&:nth-child(#{$nth}) {
|
104
|
+
margin-#{$direction}: 0;
|
105
|
+
}
|
106
|
+
}
|
107
|
+
}
|
108
|
+
|
109
|
+
// Fill 100% of parent
|
110
|
+
@mixin fill-parent() {
|
111
|
+
width: 100%;
|
112
|
+
|
113
|
+
@if $border-box-sizing == false {
|
114
|
+
@include box-sizing(border-box);
|
115
|
+
}
|
116
|
+
}
|
117
|
+
|
118
|
+
// Breakpoints
|
119
|
+
@mixin breakpoint($query:$feature $value $columns, $total-columns: $grid-columns) {
|
120
|
+
|
121
|
+
@if length($query) == 1 {
|
122
|
+
@media screen and ($default-feature: nth($query, 1)) {
|
123
|
+
$default-grid-columns: $grid-columns;
|
124
|
+
$grid-columns: $total-columns;
|
125
|
+
@content;
|
126
|
+
$grid-columns: $default-grid-columns;
|
127
|
+
}
|
128
|
+
}
|
129
|
+
|
130
|
+
@else if length($query) == 2 {
|
131
|
+
@media screen and (nth($query, 1): nth($query, 2)) {
|
132
|
+
$default-grid-columns: $grid-columns;
|
133
|
+
$grid-columns: $total-columns;
|
134
|
+
@content;
|
135
|
+
$grid-columns: $default-grid-columns;
|
136
|
+
}
|
137
|
+
}
|
138
|
+
|
139
|
+
@else if length($query) == 3 {
|
140
|
+
@media screen and (nth($query, 1): nth($query, 2)) {
|
141
|
+
$default-grid-columns: $grid-columns;
|
142
|
+
$grid-columns: nth($query, 3);
|
143
|
+
@content;
|
144
|
+
$grid-columns: $default-grid-columns;
|
145
|
+
}
|
146
|
+
}
|
147
|
+
|
148
|
+
@else {
|
149
|
+
@warn "Wrong number of arguments for breakpoint(). Read the documentation for more details.";
|
150
|
+
}
|
151
|
+
}
|
152
|
+
|
data/lib/neat/engine.rb
ADDED
data/lib/neat/version.rb
ADDED
data/lib/neat.rb
ADDED
@@ -0,0 +1,15 @@
|
|
1
|
+
module Neat
|
2
|
+
if defined?(Rails) && defined?(Rails::Engine)
|
3
|
+
class Engine < ::Rails::Engine
|
4
|
+
require 'neat/engine'
|
5
|
+
end
|
6
|
+
|
7
|
+
module Rails
|
8
|
+
class Railtie < ::Rails::Railtie
|
9
|
+
rake_tasks do
|
10
|
+
load "tasks/install.rake"
|
11
|
+
end
|
12
|
+
end
|
13
|
+
end
|
14
|
+
end
|
15
|
+
end
|
@@ -0,0 +1,23 @@
|
|
1
|
+
# Needed for pre-3.1.
|
2
|
+
|
3
|
+
require "fileutils"
|
4
|
+
require "find"
|
5
|
+
|
6
|
+
namespace :bourbon do
|
7
|
+
namespace :neat do
|
8
|
+
desc "Copy Neat's files to the Rails assets directory."
|
9
|
+
task :install, [:sass_path] do |t, args|
|
10
|
+
args.with_defaults(:sass_path => 'public/stylesheets/sass')
|
11
|
+
source_root = File.expand_path(File.join(File.dirname(__FILE__), '..', '..'))
|
12
|
+
FileUtils.mkdir_p("#{Rails.root}/#{args.sass_path}/neat")
|
13
|
+
FileUtils.cp_r("#{source_root}/app/assets/stylesheets/.", "#{Rails.root}/#{args.sass_path}/neat", { :preserve => true })
|
14
|
+
|
15
|
+
Find.find("#{Rails.root}/#{args.sass_path}/neat") do |path|
|
16
|
+
if path.end_with?(".css.scss")
|
17
|
+
path_without_css_extension = path.gsub(/\.css\.scss$/, ".scss")
|
18
|
+
FileUtils.mv(path, path_without_css_extension)
|
19
|
+
end
|
20
|
+
end
|
21
|
+
end
|
22
|
+
end
|
23
|
+
end
|
data/neat.gemspec
ADDED
@@ -0,0 +1,29 @@
|
|
1
|
+
# -*- encoding: utf-8 -*-
|
2
|
+
$:.push File.expand_path('../lib', __FILE__)
|
3
|
+
require 'neat/version'
|
4
|
+
|
5
|
+
Gem::Specification.new do |s|
|
6
|
+
s.name = "neat"
|
7
|
+
s.version = Neat::VERSION
|
8
|
+
s.platform = Gem::Platform::RUBY
|
9
|
+
s.authors = ["Kyle Fiedler", "Reda Lemeden", "Joel Oliveira"]
|
10
|
+
s.email = ["support@thoughtbot.com"]
|
11
|
+
s.homepage = "https://github.com/thoughtbot/neat"
|
12
|
+
s.summary = "A fluid grid framework on top of Bourbon"
|
13
|
+
s.description = <<-DESC
|
14
|
+
Neat is an open source fluid grid framework built on top of Bourbon with the aim of being easy enough to use out of the box and flexible enough to customize down the road.
|
15
|
+
DESC
|
16
|
+
|
17
|
+
s.rubyforge_project = "neat"
|
18
|
+
|
19
|
+
s.files = `git ls-files`.split("\n")
|
20
|
+
s.test_files = `git ls-files -- {test,spec,features}/*`.split("\n")
|
21
|
+
s.executables = `git ls-files -- bin/*`.split("\n").map{ |f| File.basename(f) }
|
22
|
+
s.require_paths = ["lib"]
|
23
|
+
|
24
|
+
s.add_dependency('sass', '>= 3.2')
|
25
|
+
s.add_dependency('bourbon', '>= 2.1')
|
26
|
+
|
27
|
+
s.add_development_dependency('aruba', '~> 0.4')
|
28
|
+
s.add_development_dependency('rake')
|
29
|
+
end
|
metadata
ADDED
@@ -0,0 +1,132 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: neat
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.4.2
|
5
|
+
prerelease:
|
6
|
+
platform: ruby
|
7
|
+
authors:
|
8
|
+
- Kyle Fiedler
|
9
|
+
- Reda Lemeden
|
10
|
+
- Joel Oliveira
|
11
|
+
autorequire:
|
12
|
+
bindir: bin
|
13
|
+
cert_chain: []
|
14
|
+
date: 2012-08-31 00:00:00.000000000 Z
|
15
|
+
dependencies:
|
16
|
+
- !ruby/object:Gem::Dependency
|
17
|
+
name: sass
|
18
|
+
requirement: !ruby/object:Gem::Requirement
|
19
|
+
none: false
|
20
|
+
requirements:
|
21
|
+
- - ! '>='
|
22
|
+
- !ruby/object:Gem::Version
|
23
|
+
version: '3.2'
|
24
|
+
type: :runtime
|
25
|
+
prerelease: false
|
26
|
+
version_requirements: !ruby/object:Gem::Requirement
|
27
|
+
none: false
|
28
|
+
requirements:
|
29
|
+
- - ! '>='
|
30
|
+
- !ruby/object:Gem::Version
|
31
|
+
version: '3.2'
|
32
|
+
- !ruby/object:Gem::Dependency
|
33
|
+
name: bourbon
|
34
|
+
requirement: !ruby/object:Gem::Requirement
|
35
|
+
none: false
|
36
|
+
requirements:
|
37
|
+
- - ! '>='
|
38
|
+
- !ruby/object:Gem::Version
|
39
|
+
version: '2.1'
|
40
|
+
type: :runtime
|
41
|
+
prerelease: false
|
42
|
+
version_requirements: !ruby/object:Gem::Requirement
|
43
|
+
none: false
|
44
|
+
requirements:
|
45
|
+
- - ! '>='
|
46
|
+
- !ruby/object:Gem::Version
|
47
|
+
version: '2.1'
|
48
|
+
- !ruby/object:Gem::Dependency
|
49
|
+
name: aruba
|
50
|
+
requirement: !ruby/object:Gem::Requirement
|
51
|
+
none: false
|
52
|
+
requirements:
|
53
|
+
- - ~>
|
54
|
+
- !ruby/object:Gem::Version
|
55
|
+
version: '0.4'
|
56
|
+
type: :development
|
57
|
+
prerelease: false
|
58
|
+
version_requirements: !ruby/object:Gem::Requirement
|
59
|
+
none: false
|
60
|
+
requirements:
|
61
|
+
- - ~>
|
62
|
+
- !ruby/object:Gem::Version
|
63
|
+
version: '0.4'
|
64
|
+
- !ruby/object:Gem::Dependency
|
65
|
+
name: rake
|
66
|
+
requirement: !ruby/object:Gem::Requirement
|
67
|
+
none: false
|
68
|
+
requirements:
|
69
|
+
- - ! '>='
|
70
|
+
- !ruby/object:Gem::Version
|
71
|
+
version: '0'
|
72
|
+
type: :development
|
73
|
+
prerelease: false
|
74
|
+
version_requirements: !ruby/object:Gem::Requirement
|
75
|
+
none: false
|
76
|
+
requirements:
|
77
|
+
- - ! '>='
|
78
|
+
- !ruby/object:Gem::Version
|
79
|
+
version: '0'
|
80
|
+
description: ! 'Neat is an open source fluid grid framework built on top of Bourbon
|
81
|
+
with the aim of being easy enough to use out of the box and flexible enough to customize
|
82
|
+
down the road.
|
83
|
+
|
84
|
+
'
|
85
|
+
email:
|
86
|
+
- support@thoughtbot.com
|
87
|
+
executables: []
|
88
|
+
extensions: []
|
89
|
+
extra_rdoc_files: []
|
90
|
+
files:
|
91
|
+
- .gitignore
|
92
|
+
- Gemfile
|
93
|
+
- LICENSE
|
94
|
+
- README.md
|
95
|
+
- app/assets/stylesheets/_neat.scss
|
96
|
+
- app/assets/stylesheets/functions/_grid.scss
|
97
|
+
- app/assets/stylesheets/functions/_line-height.scss
|
98
|
+
- app/assets/stylesheets/functions/_px-to-em.scss
|
99
|
+
- app/assets/stylesheets/grid/_grid.scss
|
100
|
+
- app/assets/stylesheets/settings/_grid.scss
|
101
|
+
- app/assets/stylesheets/settings/_typography.scss
|
102
|
+
- app/assets/stylesheets/typography/_typography.scss
|
103
|
+
- lib/neat.rb
|
104
|
+
- lib/neat/engine.rb
|
105
|
+
- lib/neat/version.rb
|
106
|
+
- lib/tasks/install.rake
|
107
|
+
- neat.gemspec
|
108
|
+
homepage: https://github.com/thoughtbot/neat
|
109
|
+
licenses: []
|
110
|
+
post_install_message:
|
111
|
+
rdoc_options: []
|
112
|
+
require_paths:
|
113
|
+
- lib
|
114
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
115
|
+
none: false
|
116
|
+
requirements:
|
117
|
+
- - ! '>='
|
118
|
+
- !ruby/object:Gem::Version
|
119
|
+
version: '0'
|
120
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
121
|
+
none: false
|
122
|
+
requirements:
|
123
|
+
- - ! '>='
|
124
|
+
- !ruby/object:Gem::Version
|
125
|
+
version: '0'
|
126
|
+
requirements: []
|
127
|
+
rubyforge_project: neat
|
128
|
+
rubygems_version: 1.8.22
|
129
|
+
signing_key:
|
130
|
+
specification_version: 3
|
131
|
+
summary: A fluid grid framework on top of Bourbon
|
132
|
+
test_files: []
|