multirb 0.0.1
Sign up to get free protection for your applications and to get access to all the features.
- data/.gitignore +17 -0
- data/Gemfile +4 -0
- data/LICENSE.txt +22 -0
- data/README.md +51 -0
- data/Rakefile +6 -0
- data/bin/multirb +30 -0
- data/lib/multirb.rb +66 -0
- data/lib/multirb/version.rb +3 -0
- data/multirb.gemspec +19 -0
- data/test/test_multirb.rb +29 -0
- metadata +59 -0
data/.gitignore
ADDED
data/Gemfile
ADDED
data/LICENSE.txt
ADDED
@@ -0,0 +1,22 @@
|
|
1
|
+
Copyright (c) 2013 Peter Cooper
|
2
|
+
|
3
|
+
MIT License
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
6
|
+
a copy of this software and associated documentation files (the
|
7
|
+
"Software"), to deal in the Software without restriction, including
|
8
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
9
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
10
|
+
permit persons to whom the Software is furnished to do so, subject to
|
11
|
+
the following conditions:
|
12
|
+
|
13
|
+
The above copyright notice and this permission notice shall be
|
14
|
+
included in all copies or substantial portions of the Software.
|
15
|
+
|
16
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
17
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
18
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
19
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
20
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
21
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
22
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,51 @@
|
|
1
|
+
# Multirb
|
2
|
+
|
3
|
+
Runs an IRB-esque prompt (but it's NOT really IRB!) over multiple Rubies using RVM.
|
4
|
+
|
5
|
+
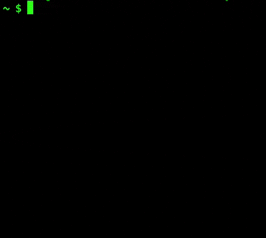
|
6
|
+
|
7
|
+
## Why is this useful?
|
8
|
+
|
9
|
+
If you're a regular developer, it's probably not very useful. But if you're researching or training people in the differences between versions and implementations of Ruby, it could be very helpful indeed.
|
10
|
+
|
11
|
+
This system was used in a different form in the [Ruby 1.9 Walkthrough](https://cooperpress.com/19walkthrough) and is now being used to develop the Ruby 2.0 Walkthrough. Multirb allows me to quickly demonstrate the ways in which different versions of Ruby handle or support various features.
|
12
|
+
|
13
|
+
## Installation
|
14
|
+
|
15
|
+
Install with:
|
16
|
+
|
17
|
+
$ gem install multirb
|
18
|
+
|
19
|
+
You also need a complete, working [RVM](https://rvm.io/) installation. Feel free to submit pull requests to make this auto detect other Ruby version managers, such as rbenv.
|
20
|
+
|
21
|
+
## Usage
|
22
|
+
|
23
|
+
Run with:
|
24
|
+
|
25
|
+
$ multirb
|
26
|
+
|
27
|
+
Or if you want to specify some different 'default' versions of Ruby to run:
|
28
|
+
|
29
|
+
$ multirb 1.9.2 1.9.3 [etc..]
|
30
|
+
|
31
|
+
Then:
|
32
|
+
|
33
|
+
1. Type in expressions and press Enter.
|
34
|
+
2. Leave whitespace on the end of lines to enter more lines.
|
35
|
+
3. Add `# all` to run all versions, nothing for default.
|
36
|
+
4. Add `# version,version,version` to run specific versions (e.g. `# 1.9.2, 1.9.3`.)
|
37
|
+
5. Type `exit` on its own to exit (or use Ctrl+D.)
|
38
|
+
|
39
|
+
Currently specifies jruby, 1.8.7, 1.9.2, 1.9.3, and 2.0.0 as 'all versions'; 1.8.7, 1.9.3, and 2.0.0 as 'default versions'.
|
40
|
+
|
41
|
+
## Versions
|
42
|
+
|
43
|
+
0.0.1: Initial version
|
44
|
+
|
45
|
+
## Contributing
|
46
|
+
|
47
|
+
1. Fork it
|
48
|
+
2. Create your feature branch (`git checkout -b my-new-feature`)
|
49
|
+
3. Commit your changes (`git commit -am 'Add some feature'`)
|
50
|
+
4. Push to the branch (`git push origin my-new-feature`)
|
51
|
+
5. Create new Pull Request
|
data/Rakefile
ADDED
data/bin/multirb
ADDED
@@ -0,0 +1,30 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
# encoding: utf-8
|
3
|
+
|
4
|
+
# multirb - Runs an IRB-esque prompt (but it's NOT really IRB!) over multiple
|
5
|
+
# versions of Ruby at once (using RVM)
|
6
|
+
#
|
7
|
+
# By Peter Cooper, BSD licensed
|
8
|
+
#
|
9
|
+
# 1. Type in expressions and press enter.
|
10
|
+
# 2. Leave whitespace on the end of lines to enter more lines.
|
11
|
+
# 3. Add # all to run all versions, nothing for default
|
12
|
+
# 4. Add # version,version,version to run specific versions.
|
13
|
+
# 5. exit on its own to exit (or Ctrl+D)
|
14
|
+
|
15
|
+
require_relative '../lib/multirb' # not strictly necessary, but laziness in development ;-)
|
16
|
+
|
17
|
+
include Multirb
|
18
|
+
|
19
|
+
loop do
|
20
|
+
lines = read_lines
|
21
|
+
filename = create_and_save_code(lines)
|
22
|
+
|
23
|
+
versions = determine_versions(lines, ARGV)
|
24
|
+
|
25
|
+
versions.each do |version|
|
26
|
+
run_code(filename, version)
|
27
|
+
end
|
28
|
+
|
29
|
+
puts
|
30
|
+
end
|
data/lib/multirb.rb
ADDED
@@ -0,0 +1,66 @@
|
|
1
|
+
# encoding: utf-8
|
2
|
+
|
3
|
+
require_relative 'multirb/version' # not strictly necessary, but laziness in development ;-)
|
4
|
+
require 'readline'
|
5
|
+
require 'tempfile'
|
6
|
+
|
7
|
+
module Multirb
|
8
|
+
ALL_VERSIONS = %w{jruby 1.8.7 1.9.2 1.9.3 2.0.0}
|
9
|
+
DEFAULT_VERSIONS = %w{1.8.7 1.9.3 2.0.0}
|
10
|
+
|
11
|
+
def read_lines
|
12
|
+
lines = []
|
13
|
+
begin
|
14
|
+
print "\e[0m"
|
15
|
+
line = Readline::readline("> ")
|
16
|
+
Readline::HISTORY << line
|
17
|
+
line.chomp!
|
18
|
+
lines << line
|
19
|
+
exit if line =~ /^exit$/i
|
20
|
+
end while line =~ /\s+$/
|
21
|
+
lines
|
22
|
+
end
|
23
|
+
|
24
|
+
def determine_versions(lines, defaults = DEFAULT_VERSIONS)
|
25
|
+
defaults = DEFAULT_VERSIONS if defaults.empty?
|
26
|
+
case lines.last
|
27
|
+
when /\#\s?all$/
|
28
|
+
ALL_VERSIONS
|
29
|
+
when /\#\s?(.+)$/
|
30
|
+
[*$1.strip.split(',').map(&:strip)]
|
31
|
+
else
|
32
|
+
defaults
|
33
|
+
end
|
34
|
+
end
|
35
|
+
|
36
|
+
def create_code(lines)
|
37
|
+
%{# encoding: utf-8
|
38
|
+
ARRAY = ['a', 'b', 'c']
|
39
|
+
HASH = { :name => "Jenny", :age => 40, :gender => :female, :hobbies => %w{chess cycling baking} }
|
40
|
+
ARRAY2 = [1,2,3]
|
41
|
+
STRING = "Hello"
|
42
|
+
STRING2 = "çé"
|
43
|
+
STRING3 = "ウabcé"
|
44
|
+
o = begin
|
45
|
+
"\e[32m" + eval(<<-'CODEHERE'
|
46
|
+
#{lines.join("\n")}
|
47
|
+
CODEHERE
|
48
|
+
).inspect + "\e[0m"
|
49
|
+
rescue Exception => e
|
50
|
+
"\e[31m!! \#{e.class}: \#{e.message}\e[0m"
|
51
|
+
end
|
52
|
+
print o}
|
53
|
+
end
|
54
|
+
|
55
|
+
def create_and_save_code(lines)
|
56
|
+
f = Tempfile.new('multirb')
|
57
|
+
f.puts create_code(lines)
|
58
|
+
f.close
|
59
|
+
f.path
|
60
|
+
end
|
61
|
+
|
62
|
+
def run_code(filename, version, out = STDOUT)
|
63
|
+
out.puts " #{version} => #{`rvm #{version} exec ruby #{filename}`}"
|
64
|
+
end
|
65
|
+
|
66
|
+
end
|
data/multirb.gemspec
ADDED
@@ -0,0 +1,19 @@
|
|
1
|
+
# -*- encoding: utf-8 -*-
|
2
|
+
lib = File.expand_path('../lib', __FILE__)
|
3
|
+
$LOAD_PATH.unshift(lib) unless $LOAD_PATH.include?(lib)
|
4
|
+
require 'multirb/version'
|
5
|
+
|
6
|
+
Gem::Specification.new do |gem|
|
7
|
+
gem.name = "multirb"
|
8
|
+
gem.version = Multirb::VERSION
|
9
|
+
gem.authors = ["Peter Cooper"]
|
10
|
+
gem.email = ["git@peterc.org"]
|
11
|
+
gem.description = %{Run Ruby code over multiple implementations/versions using RVM from a IRB-esque prompt}
|
12
|
+
gem.summary = %{Run Ruby code over multiple implementations/versions using RVM from a IRB-esque prompt}
|
13
|
+
gem.homepage = "https://github.com/peterc/multirb"
|
14
|
+
|
15
|
+
gem.files = `git ls-files`.split($/)
|
16
|
+
gem.executables = gem.files.grep(%r{^bin/}).map{ |f| File.basename(f) }
|
17
|
+
gem.test_files = gem.files.grep(%r{^(test|spec|features)/})
|
18
|
+
gem.require_paths = ["lib"]
|
19
|
+
end
|
@@ -0,0 +1,29 @@
|
|
1
|
+
$: << File.expand_path(__FILE__, "../../lib")
|
2
|
+
|
3
|
+
require 'minitest/autorun'
|
4
|
+
require 'multirb'
|
5
|
+
|
6
|
+
include Multirb
|
7
|
+
|
8
|
+
# Some merely cursory tests, in this case!
|
9
|
+
|
10
|
+
describe Multirb do
|
11
|
+
it "should have versions defined" do
|
12
|
+
ALL_VERSIONS.must_be_instance_of Array
|
13
|
+
DEFAULT_VERSIONS.must_be_instance_of Array
|
14
|
+
|
15
|
+
ALL_VERSIONS.length.must_be :>, 2
|
16
|
+
DEFAULT_VERSIONS.length.must_be :>, 2
|
17
|
+
end
|
18
|
+
|
19
|
+
it "should be able to determine versions wanted by the user" do
|
20
|
+
determine_versions(['2 + 2']).must_equal DEFAULT_VERSIONS
|
21
|
+
determine_versions(['2 + 2 #all']).must_equal ALL_VERSIONS
|
22
|
+
determine_versions(['2 + 2 # all']).must_equal ALL_VERSIONS
|
23
|
+
determine_versions(['2 + 2 #all', '5 + 5']).must_equal DEFAULT_VERSIONS
|
24
|
+
determine_versions(['2 + 2 #all', '5 + 5'], []).must_equal DEFAULT_VERSIONS
|
25
|
+
determine_versions(['2 + 2 #all', '5 + 5'], %w{1.9.2}).must_equal %w{1.9.2}
|
26
|
+
determine_versions(['2 + 2 # 1.9.2,1.9.3,2.0.0']).must_equal %w{1.9.2 1.9.3 2.0.0}
|
27
|
+
determine_versions(['2 + 2 # 1.9.2, 1.9.3, 2.0.0']).must_equal %w{1.9.2 1.9.3 2.0.0}
|
28
|
+
end
|
29
|
+
end
|
metadata
ADDED
@@ -0,0 +1,59 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: multirb
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.1
|
5
|
+
prerelease:
|
6
|
+
platform: ruby
|
7
|
+
authors:
|
8
|
+
- Peter Cooper
|
9
|
+
autorequire:
|
10
|
+
bindir: bin
|
11
|
+
cert_chain: []
|
12
|
+
date: 2013-01-27 00:00:00.000000000 Z
|
13
|
+
dependencies: []
|
14
|
+
description: Run Ruby code over multiple implementations/versions using RVM from a
|
15
|
+
IRB-esque prompt
|
16
|
+
email:
|
17
|
+
- git@peterc.org
|
18
|
+
executables:
|
19
|
+
- multirb
|
20
|
+
extensions: []
|
21
|
+
extra_rdoc_files: []
|
22
|
+
files:
|
23
|
+
- .gitignore
|
24
|
+
- Gemfile
|
25
|
+
- LICENSE.txt
|
26
|
+
- README.md
|
27
|
+
- Rakefile
|
28
|
+
- bin/multirb
|
29
|
+
- lib/multirb.rb
|
30
|
+
- lib/multirb/version.rb
|
31
|
+
- multirb.gemspec
|
32
|
+
- test/test_multirb.rb
|
33
|
+
homepage: https://github.com/peterc/multirb
|
34
|
+
licenses: []
|
35
|
+
post_install_message:
|
36
|
+
rdoc_options: []
|
37
|
+
require_paths:
|
38
|
+
- lib
|
39
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
40
|
+
none: false
|
41
|
+
requirements:
|
42
|
+
- - ! '>='
|
43
|
+
- !ruby/object:Gem::Version
|
44
|
+
version: '0'
|
45
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
46
|
+
none: false
|
47
|
+
requirements:
|
48
|
+
- - ! '>='
|
49
|
+
- !ruby/object:Gem::Version
|
50
|
+
version: '0'
|
51
|
+
requirements: []
|
52
|
+
rubyforge_project:
|
53
|
+
rubygems_version: 1.8.24
|
54
|
+
signing_key:
|
55
|
+
specification_version: 3
|
56
|
+
summary: Run Ruby code over multiple implementations/versions using RVM from a IRB-esque
|
57
|
+
prompt
|
58
|
+
test_files:
|
59
|
+
- test/test_multirb.rb
|