motion-gradle 1.0.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/LICENSE +21 -0
- data/README.md +94 -0
- data/lib/motion-gradle.rb +2 -0
- data/lib/motion/project/gradle.erb +27 -0
- data/lib/motion/project/gradle.rb +121 -0
- data/lib/motion/project/version.rb +5 -0
- metadata +50 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: ed5e4e00917e92a5f230a2a05d30c42a3452f976
|
4
|
+
data.tar.gz: fba75781e58386f1a71971434b1df69254591882
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: c5327a2442668aa9297f034dbeca76c6976f26c0c77580559f149163d2940be74ad166afe0b0c6fd2141c2b5c5affb20f3a5949e64ecc78afed681726981b109
|
7
|
+
data.tar.gz: 7f6cff7ed624d511523af66f8e725b0481c315aeb9c6ea55ec533b83493c92107a6f859f27262f0dd080d76fd9f10134964b0a7dad36b27bdcca0b8992c5c206
|
data/LICENSE
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
The MIT License (MIT)
|
2
|
+
|
3
|
+
Copyright (c) 2015 Joffrey Jaffeux
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in
|
13
|
+
all copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN
|
21
|
+
THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,94 @@
|
|
1
|
+
# motion-gradle
|
2
|
+
|
3
|
+
motion-gradle allows RubyMotion projects to integrate with the
|
4
|
+
[Gradle](http://gradle.apache.org/) dependency manager.
|
5
|
+
|
6
|
+
|
7
|
+
## Installation
|
8
|
+
|
9
|
+
You need to have gradle installed:
|
10
|
+
|
11
|
+
```
|
12
|
+
$ brew install gradle
|
13
|
+
```
|
14
|
+
|
15
|
+
And the gem installed:
|
16
|
+
|
17
|
+
```
|
18
|
+
$ [sudo] gem install motion-gradle
|
19
|
+
```
|
20
|
+
|
21
|
+
Or if you use Bundler:
|
22
|
+
|
23
|
+
```ruby
|
24
|
+
gem 'motion-gradle'
|
25
|
+
```
|
26
|
+
|
27
|
+
You also need to install `Extras/Android Support Repository` the Android SDK Manager gui.
|
28
|
+
|
29
|
+
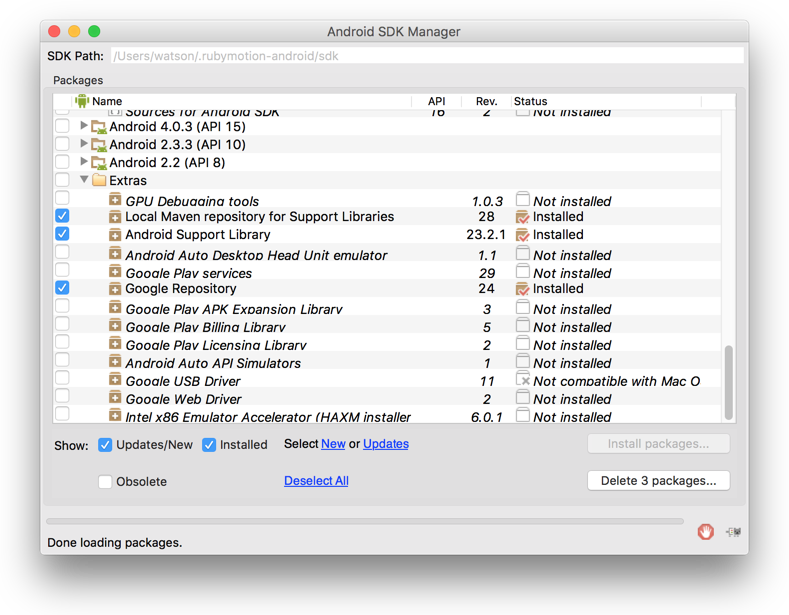
|
30
|
+
|
31
|
+
|
32
|
+
## Setup
|
33
|
+
|
34
|
+
1. Edit the `Rakefile` of your RubyMotion project and add the following require
|
35
|
+
lines:
|
36
|
+
|
37
|
+
```ruby
|
38
|
+
require 'rubygems'
|
39
|
+
require 'motion-gradle'
|
40
|
+
```
|
41
|
+
|
42
|
+
2. Still in the `Rakefile`, set your dependencies
|
43
|
+
|
44
|
+
```ruby
|
45
|
+
Motion::Project::App.setup do |app|
|
46
|
+
# ...
|
47
|
+
app.gradle do
|
48
|
+
dependency 'com.mcxiaoke.volley', :artifact => 'library', :version => '1.0.10'
|
49
|
+
dependency 'commons-cli'
|
50
|
+
dependency 'ehcache', :version => '1.2.3'
|
51
|
+
end
|
52
|
+
end
|
53
|
+
```
|
54
|
+
|
55
|
+
* :version will default to latest: +
|
56
|
+
|
57
|
+
* :artifact will default to dependency name
|
58
|
+
|
59
|
+
|
60
|
+
## Configuration
|
61
|
+
|
62
|
+
If the `gradle` command is not in your path, you can configure it:
|
63
|
+
|
64
|
+
```ruby
|
65
|
+
Motion::Project::App.setup do |app|
|
66
|
+
# ...
|
67
|
+
app.gradle.path = '/some/path/gradle'
|
68
|
+
end
|
69
|
+
```
|
70
|
+
|
71
|
+
You can also add other repositories :
|
72
|
+
|
73
|
+
```ruby
|
74
|
+
Motion::Project::App.setup do |app|
|
75
|
+
# ...
|
76
|
+
app.gradle do
|
77
|
+
repository 'https://bintray.com/bintray/jcenter'
|
78
|
+
repository 'http://dl.bintray.com/austintaylor/gradle'
|
79
|
+
end
|
80
|
+
end
|
81
|
+
```
|
82
|
+
|
83
|
+
## Tasks
|
84
|
+
|
85
|
+
To tell motion-gradle to download your dependencies, run the following rake
|
86
|
+
task:
|
87
|
+
|
88
|
+
```
|
89
|
+
$ [bundle exec] rake gradle:install
|
90
|
+
```
|
91
|
+
|
92
|
+
After a `rake:clean:all` you will need to run the install task agin.
|
93
|
+
|
94
|
+
That’s all.
|
@@ -0,0 +1,27 @@
|
|
1
|
+
apply plugin: 'java'
|
2
|
+
apply plugin: 'eclipse'
|
3
|
+
|
4
|
+
task generateDependencies(type: Jar) {
|
5
|
+
baseName = 'dependencies'
|
6
|
+
from { configurations.compile.collect { it.isDirectory() ? it : zipTree(it) } }
|
7
|
+
with jar
|
8
|
+
}
|
9
|
+
|
10
|
+
repositories {
|
11
|
+
def androidHome = System.getenv("ANDROID_HOME")
|
12
|
+
maven {
|
13
|
+
url "$androidHome/extras/android/m2repository/"
|
14
|
+
}
|
15
|
+
<% @repositories.each do |url| %>
|
16
|
+
maven {
|
17
|
+
url "<%= url %>"
|
18
|
+
}
|
19
|
+
<% end %>
|
20
|
+
mavenCentral()
|
21
|
+
}
|
22
|
+
|
23
|
+
dependencies {
|
24
|
+
<% @dependencies.each do |dependency| %>
|
25
|
+
compile '<%= dependency[:name] %>:<%= dependency[:artifact] %>:<%= dependency[:version] %>'
|
26
|
+
<% end %>
|
27
|
+
}
|
@@ -0,0 +1,121 @@
|
|
1
|
+
unless defined?(Motion::Project::Config)
|
2
|
+
raise "This file must be required within a RubyMotion project Rakefile."
|
3
|
+
end
|
4
|
+
|
5
|
+
if Motion::Project::App.template != :android
|
6
|
+
raise "This file must be required within a RubyMotion Android project."
|
7
|
+
end
|
8
|
+
|
9
|
+
module Motion::Project
|
10
|
+
class Config
|
11
|
+
variable :gradle
|
12
|
+
|
13
|
+
def gradle(&block)
|
14
|
+
@gradle ||= Motion::Project::Gradle.new(self)
|
15
|
+
if block
|
16
|
+
@gradle.instance_eval(&block)
|
17
|
+
end
|
18
|
+
@gradle
|
19
|
+
end
|
20
|
+
end
|
21
|
+
|
22
|
+
class Gradle
|
23
|
+
GRADLE_ROOT = 'vendor/Gradle'
|
24
|
+
|
25
|
+
def initialize(config)
|
26
|
+
@gradle_path = '/usr/local/bin/gradle'
|
27
|
+
@config = config
|
28
|
+
@dependencies = []
|
29
|
+
@repositories = []
|
30
|
+
configure_project
|
31
|
+
end
|
32
|
+
|
33
|
+
def configure_project
|
34
|
+
@config.vendor_project(:jar => "#{GRADLE_ROOT}/build/libs/dependencies.jar")
|
35
|
+
end
|
36
|
+
|
37
|
+
def path=(path)
|
38
|
+
@gradle_path = path
|
39
|
+
end
|
40
|
+
|
41
|
+
def dependency(name, options = {})
|
42
|
+
@dependencies << normalized_dependency(name, options)
|
43
|
+
end
|
44
|
+
|
45
|
+
def repository(url, options = {})
|
46
|
+
@repositories << url
|
47
|
+
end
|
48
|
+
|
49
|
+
def install!(update)
|
50
|
+
generate_gradle_build_file
|
51
|
+
support_repository = File.join(ENV['RUBYMOTION_ANDROID_SDK'], 'extras', 'android', 'm2repository')
|
52
|
+
|
53
|
+
unless File.exist?(support_repository)
|
54
|
+
gui_path = File.join(ENV['RUBYMOTION_ANDROID_SDK'], 'tools', 'android')
|
55
|
+
$stderr.puts("[!] To use motion-gradle you need to install `Extras/Android Support Repository`. Open the gui to install it : #{gui_path}")
|
56
|
+
exit 1
|
57
|
+
end
|
58
|
+
|
59
|
+
system "#{gradle_command} --build-file #{gradle_build_file} generateDependencies"
|
60
|
+
end
|
61
|
+
|
62
|
+
# Helpers
|
63
|
+
def generate_gradle_build_file
|
64
|
+
template_path = File.expand_path("../gradle.erb", __FILE__)
|
65
|
+
template = ERB.new(File.new(template_path).read, nil, "%")
|
66
|
+
File.open(gradle_build_file, 'w') do |io|
|
67
|
+
io.puts template.result(binding)
|
68
|
+
end
|
69
|
+
end
|
70
|
+
|
71
|
+
def gradle_build_file
|
72
|
+
File.join(GRADLE_ROOT, 'build.gradle')
|
73
|
+
end
|
74
|
+
|
75
|
+
def gradle_command
|
76
|
+
unless system("command -v #{@gradle_path} >/dev/null")
|
77
|
+
$stderr.puts "[!] #{@gradle_path} command doesn’t exist. Verify your gradle installation. Or set a different one with `app.gradle.path=(path)`"
|
78
|
+
exit 1
|
79
|
+
end
|
80
|
+
|
81
|
+
if ENV['GRADLE_DEBUG']
|
82
|
+
"#{@gradle_path} --info"
|
83
|
+
else
|
84
|
+
"#{@gradle_path}"
|
85
|
+
end
|
86
|
+
end
|
87
|
+
|
88
|
+
def normalized_dependency(name, options)
|
89
|
+
{
|
90
|
+
name: name,
|
91
|
+
version: options.fetch(:version, '+'),
|
92
|
+
artifact: options.fetch(:artifact, name)
|
93
|
+
}
|
94
|
+
end
|
95
|
+
|
96
|
+
def inspect
|
97
|
+
@dependencies.map do |dependency|
|
98
|
+
"#{dependency[:name]} - #{dependency[:artifact]} (#{dependency[:version]})"
|
99
|
+
end.inspect
|
100
|
+
end
|
101
|
+
end
|
102
|
+
end
|
103
|
+
|
104
|
+
namespace :gradle do
|
105
|
+
desc "Download and build dependencies"
|
106
|
+
task :install do
|
107
|
+
FileUtils.mkdir_p Motion::Project::Gradle::GRADLE_ROOT
|
108
|
+
dependencies = App.config.gradle
|
109
|
+
dependencies.install!(true)
|
110
|
+
end
|
111
|
+
end
|
112
|
+
|
113
|
+
namespace :clean do
|
114
|
+
task :all do
|
115
|
+
dir = Motion::Project::Gradle::GRADLE_ROOT
|
116
|
+
if File.exist?(dir)
|
117
|
+
App.info 'Delete', dir
|
118
|
+
rm_rf dir
|
119
|
+
end
|
120
|
+
end
|
121
|
+
end
|
metadata
ADDED
@@ -0,0 +1,50 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: motion-gradle
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 1.0.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Joffrey Jaffeux
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2015-04-06 00:00:00.000000000 Z
|
12
|
+
dependencies: []
|
13
|
+
description: motion-gradle allows RubyMotion Android projects to have access to the
|
14
|
+
Gradle dependency manager.
|
15
|
+
email: j.jaffeux@gmail.com
|
16
|
+
executables: []
|
17
|
+
extensions: []
|
18
|
+
extra_rdoc_files: []
|
19
|
+
files:
|
20
|
+
- LICENSE
|
21
|
+
- README.md
|
22
|
+
- lib/motion-gradle.rb
|
23
|
+
- lib/motion/project/gradle.erb
|
24
|
+
- lib/motion/project/gradle.rb
|
25
|
+
- lib/motion/project/version.rb
|
26
|
+
homepage: http://www.rubymotion.com
|
27
|
+
licenses:
|
28
|
+
- MIT
|
29
|
+
metadata: {}
|
30
|
+
post_install_message:
|
31
|
+
rdoc_options: []
|
32
|
+
require_paths:
|
33
|
+
- lib
|
34
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
35
|
+
requirements:
|
36
|
+
- - ">="
|
37
|
+
- !ruby/object:Gem::Version
|
38
|
+
version: '0'
|
39
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
40
|
+
requirements:
|
41
|
+
- - ">="
|
42
|
+
- !ruby/object:Gem::Version
|
43
|
+
version: '0'
|
44
|
+
requirements: []
|
45
|
+
rubyforge_project:
|
46
|
+
rubygems_version: 2.2.2
|
47
|
+
signing_key:
|
48
|
+
specification_version: 4
|
49
|
+
summary: Gradle integration for RubyMotion Android projects
|
50
|
+
test_files: []
|