motion-giphy 1.2.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +7 -0
- data/README.md +77 -0
- data/lib/motion-giphy.rb +10 -0
- data/lib/motion-giphy/client.rb +30 -0
- data/lib/motion-giphy/configuration.rb +16 -0
- data/lib/motion-giphy/error.rb +10 -0
- data/lib/motion-giphy/gif.rb +59 -0
- data/lib/motion-giphy/image.rb +13 -0
- data/lib/motion-giphy/meta.rb +10 -0
- data/lib/motion-giphy/pagination.rb +11 -0
- data/lib/motion-giphy/request.rb +28 -0
- data/lib/motion-giphy/response.rb +45 -0
- metadata +97 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: d1183020c545e3dbe18e10a8ca8b48d465720e07
|
4
|
+
data.tar.gz: 63860430c1c747d8cc0c76be51e41785aa4884ff
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 5decaab9279ad5439a945b30a90cecc61578a7c35a029dab514860429e7bd2cb3bd4c391c637471c5b32439b0f335d62ae2a8ba206821790312d3d196b04518b
|
7
|
+
data.tar.gz: 31ee0f84d28ce025dafe1e84d6a53aa35691e9eaaff7fb257b44dfaac2aec06a86a988bb9371eb4620bd00282c9cfd8d1acd11eca6bb05531c88d91a278c033a
|
data/README.md
ADDED
@@ -0,0 +1,77 @@
|
|
1
|
+
# Motion Giphy
|
2
|
+
> A wrapper around http://giphy.com
|
3
|
+
|
4
|
+
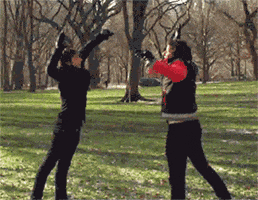
|
5
|
+
|
6
|
+
## Installation
|
7
|
+
|
8
|
+
Add this to your Gemfile:
|
9
|
+
```ruby
|
10
|
+
gem "motion-giphy"
|
11
|
+
```
|
12
|
+
|
13
|
+
Then execute:
|
14
|
+
|
15
|
+
```
|
16
|
+
$ bundle
|
17
|
+
```
|
18
|
+
|
19
|
+
MotionGiphy relies on [AFMotion](https://github.com/usepropeller/afmotion) to run so you'll need to install the neccessary pods.
|
20
|
+
|
21
|
+
```
|
22
|
+
# First get Cocoapods up and running.
|
23
|
+
$ pod setup
|
24
|
+
|
25
|
+
# Now install the needed pods.
|
26
|
+
$ rake pod:install
|
27
|
+
```
|
28
|
+
|
29
|
+
## Usage
|
30
|
+
|
31
|
+
First you'll need to set your API. You can do this in the `app_delegate.rb` file.
|
32
|
+
|
33
|
+
```ruby
|
34
|
+
MotionGiphy::Configuration.configure do |config|
|
35
|
+
config.api_key = "API_KEY"
|
36
|
+
end
|
37
|
+
```
|
38
|
+
|
39
|
+
[Giphy](http://giphy.com/api) provide a development API key that you can use. If you're moving to production you'll need to contact them.
|
40
|
+
|
41
|
+
With the gem you can retrieve the Top 100 Gifs, search and get a specific Gif via it's ID.
|
42
|
+
|
43
|
+
```ruby
|
44
|
+
MotionGiphy::Client.trending do |response|
|
45
|
+
end
|
46
|
+
```
|
47
|
+
|
48
|
+
```ruby
|
49
|
+
MotionGiphy::Client.search("dancing") do |response|
|
50
|
+
end
|
51
|
+
```
|
52
|
+
|
53
|
+
```ruby
|
54
|
+
MotionGiphy::Client.gif(gif_id) do |response|
|
55
|
+
end
|
56
|
+
```
|
57
|
+
|
58
|
+
All three methods will return a `MotionGiphy::Response` object. From here you can determine the state of the request, pagination details and either an array of Gifs (`#search`, `#trending`) or a single Gif (`#id`).
|
59
|
+
|
60
|
+
```ruby
|
61
|
+
MotionGiphy::Client.search("dancing") do |response|
|
62
|
+
if response.success?
|
63
|
+
gif = response.data.first
|
64
|
+
|
65
|
+
puts gif.id
|
66
|
+
puts gif.giphy_url
|
67
|
+
|
68
|
+
# Returns a MotionGiphy::Image
|
69
|
+
puts gif.fixed_width.url
|
70
|
+
else
|
71
|
+
puts response.error.message
|
72
|
+
end
|
73
|
+
end
|
74
|
+
```
|
75
|
+
|
76
|
+
To see all the methods you have access to I would recommend having a read through the `MotionGiphy::Gif` class and the `MotionGiphy::Image` class.
|
77
|
+
|
data/lib/motion-giphy.rb
ADDED
@@ -0,0 +1,10 @@
|
|
1
|
+
require "afmotion"
|
2
|
+
|
3
|
+
unless defined?(Motion::Project::Config)
|
4
|
+
raise "This file must be required within a RubyMotion project Rakefile."
|
5
|
+
end
|
6
|
+
|
7
|
+
lib_dir_path = File.dirname(File.expand_path(__FILE__))
|
8
|
+
Motion::Project::App.setup do |app|
|
9
|
+
app.files.unshift(Dir.glob(File.join(lib_dir_path, "motion-giphy/*.rb")))
|
10
|
+
end
|
@@ -0,0 +1,30 @@
|
|
1
|
+
module MotionGiphy
|
2
|
+
class Client
|
3
|
+
def self.search(query, options = {}, &block)
|
4
|
+
options = { q: query }.merge(options)
|
5
|
+
|
6
|
+
request.get("search", options) do |response|
|
7
|
+
response.process_data_as_batch if response.success?
|
8
|
+
block.call response
|
9
|
+
end
|
10
|
+
end
|
11
|
+
|
12
|
+
def self.gif(id, &block)
|
13
|
+
request.get("#{id}") do |response|
|
14
|
+
response.process_data_as_singular if response.success?
|
15
|
+
block.call response
|
16
|
+
end
|
17
|
+
end
|
18
|
+
|
19
|
+
def self.trending(options = {}, &block)
|
20
|
+
request.get("trending", options) do |response|
|
21
|
+
response.process_data_as_batch if response.success?
|
22
|
+
block.call response
|
23
|
+
end
|
24
|
+
end
|
25
|
+
|
26
|
+
def self.request
|
27
|
+
MotionGiphy::Request.new
|
28
|
+
end
|
29
|
+
end
|
30
|
+
end
|
@@ -0,0 +1,59 @@
|
|
1
|
+
module MotionGiphy
|
2
|
+
class Gif
|
3
|
+
def self.process_batch(data)
|
4
|
+
data.map { |gif_hash| new(gif_hash) }
|
5
|
+
end
|
6
|
+
|
7
|
+
attr_reader :hash
|
8
|
+
|
9
|
+
def initialize(hash)
|
10
|
+
@hash = hash
|
11
|
+
end
|
12
|
+
|
13
|
+
def id
|
14
|
+
hash["id"]
|
15
|
+
end
|
16
|
+
|
17
|
+
def giphy_url
|
18
|
+
hash["url"]
|
19
|
+
end
|
20
|
+
|
21
|
+
def original
|
22
|
+
image.new(images["original"])
|
23
|
+
end
|
24
|
+
|
25
|
+
def fixed_width
|
26
|
+
image.new(images["fixed_width"])
|
27
|
+
end
|
28
|
+
|
29
|
+
def fixed_width_downsampled
|
30
|
+
image.new(images["fixed_width_downsampled"])
|
31
|
+
end
|
32
|
+
|
33
|
+
def fixed_height
|
34
|
+
image.new(images["fixed_height"])
|
35
|
+
end
|
36
|
+
|
37
|
+
def fixed_height_downsampled
|
38
|
+
image.new(images["fixed_height_downsampled"])
|
39
|
+
end
|
40
|
+
|
41
|
+
def fixed_width_still
|
42
|
+
image.new(images["fixed_width_still"])
|
43
|
+
end
|
44
|
+
|
45
|
+
def fixed_height_still
|
46
|
+
image.new(images["fixed_height_still"])
|
47
|
+
end
|
48
|
+
|
49
|
+
private
|
50
|
+
|
51
|
+
def images
|
52
|
+
hash["images"]
|
53
|
+
end
|
54
|
+
|
55
|
+
def image
|
56
|
+
MotionGiphy::Image
|
57
|
+
end
|
58
|
+
end
|
59
|
+
end
|
@@ -0,0 +1,13 @@
|
|
1
|
+
module MotionGiphy
|
2
|
+
class Image
|
3
|
+
attr_reader :url, :width, :height, :frames, :size
|
4
|
+
|
5
|
+
def initialize(image_hash)
|
6
|
+
@url = image_hash["url"]
|
7
|
+
@width = image_hash["width"]
|
8
|
+
@height = image_hash["height"]
|
9
|
+
@frames = image_hash["frames"]
|
10
|
+
@size = image_hash["size"]
|
11
|
+
end
|
12
|
+
end
|
13
|
+
end
|
@@ -0,0 +1,28 @@
|
|
1
|
+
module MotionGiphy
|
2
|
+
class Request
|
3
|
+
def get(path, options = {}, &block)
|
4
|
+
client.get(path, create_options(options)) do |result|
|
5
|
+
block.call response.build_with_result(result)
|
6
|
+
end
|
7
|
+
end
|
8
|
+
|
9
|
+
private
|
10
|
+
|
11
|
+
def client
|
12
|
+
AFMotion::SessionClient.build("http://api.giphy.com/v1/gifs/") do
|
13
|
+
session_configuration :default
|
14
|
+
header "Accept", "application/json"
|
15
|
+
response_serializer :json
|
16
|
+
end
|
17
|
+
end
|
18
|
+
|
19
|
+
def response
|
20
|
+
MotionGiphy::Response
|
21
|
+
end
|
22
|
+
|
23
|
+
def create_options(options)
|
24
|
+
api_key = MotionGiphy::Configuration.api_key
|
25
|
+
{ api_key: api_key }.merge(options)
|
26
|
+
end
|
27
|
+
end
|
28
|
+
end
|
@@ -0,0 +1,45 @@
|
|
1
|
+
module MotionGiphy
|
2
|
+
class Response
|
3
|
+
def self.build_with_result(result)
|
4
|
+
response = self.new
|
5
|
+
|
6
|
+
if result.success?
|
7
|
+
response.success = true
|
8
|
+
response.json = result.object
|
9
|
+
else
|
10
|
+
response.success = false
|
11
|
+
response.error = MotionGiphy::Error.new(result.error)
|
12
|
+
end
|
13
|
+
|
14
|
+
response
|
15
|
+
end
|
16
|
+
|
17
|
+
attr_accessor :data, :json, :success, :error
|
18
|
+
|
19
|
+
def process_data_as_singular
|
20
|
+
self.data = gif.new(self.json["data"])
|
21
|
+
end
|
22
|
+
|
23
|
+
def process_data_as_batch
|
24
|
+
self.data = gif.process_batch(self.json["data"])
|
25
|
+
end
|
26
|
+
|
27
|
+
def pagination
|
28
|
+
@pages ||= MotionGiphy::Pagination.new(json["pagination"])
|
29
|
+
end
|
30
|
+
|
31
|
+
def meta
|
32
|
+
@meta ||= MoptionGiphy::Meta.new(json["meta"])
|
33
|
+
end
|
34
|
+
|
35
|
+
def success?
|
36
|
+
@success
|
37
|
+
end
|
38
|
+
|
39
|
+
private
|
40
|
+
|
41
|
+
def gif
|
42
|
+
MotionGiphy::Gif
|
43
|
+
end
|
44
|
+
end
|
45
|
+
end
|
metadata
ADDED
@@ -0,0 +1,97 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: motion-giphy
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 1.2.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Will Raxworthy
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2014-01-24 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: motion-cocoapods
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - '>='
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '0'
|
20
|
+
type: :runtime
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - '>='
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '0'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: afmotion
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - '>='
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '0'
|
34
|
+
type: :runtime
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - '>='
|
39
|
+
- !ruby/object:Gem::Version
|
40
|
+
version: '0'
|
41
|
+
- !ruby/object:Gem::Dependency
|
42
|
+
name: rake
|
43
|
+
requirement: !ruby/object:Gem::Requirement
|
44
|
+
requirements:
|
45
|
+
- - '>='
|
46
|
+
- !ruby/object:Gem::Version
|
47
|
+
version: '0'
|
48
|
+
type: :development
|
49
|
+
prerelease: false
|
50
|
+
version_requirements: !ruby/object:Gem::Requirement
|
51
|
+
requirements:
|
52
|
+
- - '>='
|
53
|
+
- !ruby/object:Gem::Version
|
54
|
+
version: '0'
|
55
|
+
description: Giphy API wrapper for RubyMotion
|
56
|
+
email:
|
57
|
+
- git@willrax.com
|
58
|
+
executables: []
|
59
|
+
extensions: []
|
60
|
+
extra_rdoc_files: []
|
61
|
+
files:
|
62
|
+
- README.md
|
63
|
+
- lib/motion-giphy/client.rb
|
64
|
+
- lib/motion-giphy/configuration.rb
|
65
|
+
- lib/motion-giphy/error.rb
|
66
|
+
- lib/motion-giphy/gif.rb
|
67
|
+
- lib/motion-giphy/image.rb
|
68
|
+
- lib/motion-giphy/meta.rb
|
69
|
+
- lib/motion-giphy/pagination.rb
|
70
|
+
- lib/motion-giphy/request.rb
|
71
|
+
- lib/motion-giphy/response.rb
|
72
|
+
- lib/motion-giphy.rb
|
73
|
+
homepage: ''
|
74
|
+
licenses:
|
75
|
+
- ''
|
76
|
+
metadata: {}
|
77
|
+
post_install_message:
|
78
|
+
rdoc_options: []
|
79
|
+
require_paths:
|
80
|
+
- lib
|
81
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
82
|
+
requirements:
|
83
|
+
- - '>='
|
84
|
+
- !ruby/object:Gem::Version
|
85
|
+
version: '0'
|
86
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
87
|
+
requirements:
|
88
|
+
- - '>='
|
89
|
+
- !ruby/object:Gem::Version
|
90
|
+
version: '0'
|
91
|
+
requirements: []
|
92
|
+
rubyforge_project:
|
93
|
+
rubygems_version: 2.0.14
|
94
|
+
signing_key:
|
95
|
+
specification_version: 4
|
96
|
+
summary: Giphy API wrapper for RubyMotion
|
97
|
+
test_files: []
|