motion-form 0.0.7 → 0.0.8
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +46 -7
- data/lib/project/form/base.rb +4 -0
- data/lib/project/rows/text_field_row.rb +35 -0
- metadata +3 -3
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 93259a7e0e32f3c910f42236b224ac39f740e3a5
|
4
|
+
data.tar.gz: a406a91fe8a71febc9e362cbce1676ad57b9dd78
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 042d1cabbe16fcca39b1ae14fdba95aca48137bce45d32439385894e1689d857b1e1c50ac6f7ebe8e3debc2fe52163e791970e4bbdd36d0aaa8678018a068f8e
|
7
|
+
data.tar.gz: a87072db409e61c051e153871ed90f9536d6f1f9800d97b9348e0b515feb9e781d327f83de90c12a1f27bfc001dfa4dfd2a44bf5cfe351e369f0d9ba04e7aa02
|
data/README.md
CHANGED
@@ -6,13 +6,22 @@ motion-form is heavily inspired by the gem simple_form for Rails.
|
|
6
6
|
|
7
7
|
It aims to bring a simple, yet flexible DSL to the tedious task of creating iOS forms.
|
8
8
|
|
9
|
+
[](http://badge.fury.io/rb/motion-form)
|
10
|
+
[](https://codeclimate.com/github/dblandin/motion-form)
|
11
|
+
[](https://travis-ci.org/dblandin/motion-form)
|
12
|
+
|
13
|
+
motion-form's initial development was sponsored by [dscout](https://dscout.com). Many thanks to them!
|
14
|
+
|
9
15
|
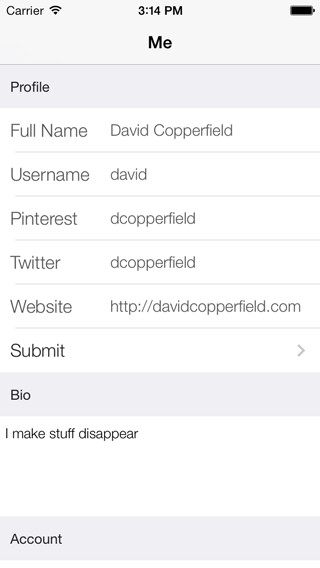
|
10
16
|
|
11
17
|
## Usage
|
12
18
|
|
13
|
-
```ruby
|
14
|
-
form = MotionForm.form_for(view) do |
|
15
|
-
|
19
|
+
``` ruby
|
20
|
+
form = MotionForm.form_for(view) do |form|
|
21
|
+
# If you don't want section header views, leave the section name blank
|
22
|
+
# Ex: form.section do |section|
|
23
|
+
|
24
|
+
form.section 'Profile' do |section|
|
16
25
|
section.input :name, icon: :contact, value: 'David Copperfield'
|
17
26
|
section.input :username, icon: :user
|
18
27
|
section.input :pinterest, icon: :pinterest
|
@@ -21,24 +30,50 @@ form = MotionForm.form_for(view) do |f|
|
|
21
30
|
section.input :bio, icon: :info
|
22
31
|
end
|
23
32
|
|
24
|
-
|
33
|
+
form.section 'Account' do |section|
|
25
34
|
section.button :change_email, icon: :email, action: push_email_controller
|
26
35
|
section.button :change_password, icon: :lock, action: push_password_controller
|
27
36
|
end
|
28
37
|
end
|
29
38
|
|
30
39
|
def push_email_controller
|
31
|
-
|
40
|
+
lambda do
|
41
|
+
# push controller
|
42
|
+
end
|
32
43
|
end
|
33
44
|
|
34
45
|
def push_password_controller
|
35
|
-
|
46
|
+
lambda do
|
47
|
+
# push controller
|
48
|
+
end
|
49
|
+
end
|
50
|
+
```
|
51
|
+
|
52
|
+
### Validation
|
53
|
+
|
54
|
+
You can add validation rules to input fields.
|
55
|
+
|
56
|
+
The following syntax is supported:
|
57
|
+
|
58
|
+
``` ruby
|
59
|
+
awesomeness_validator = lambda do |value|
|
60
|
+
# validate awesomeness
|
61
|
+
end
|
62
|
+
|
63
|
+
MotionForm.form_for(view) do |form|
|
64
|
+
form.section do |section
|
65
|
+
section.input :name, required: true # present and not blank
|
66
|
+
section.input :email, email: true # valid email address
|
67
|
+
section.input :website, url: true # valid url
|
68
|
+
section.input :age, format: /^\d+$/ # matches regex
|
69
|
+
section.input :awesomeness, validate_with: awesomeness_validator # custom validator
|
70
|
+
end
|
36
71
|
end
|
37
72
|
```
|
38
73
|
|
39
74
|
### Configuration
|
40
75
|
|
41
|
-
```ruby
|
76
|
+
``` ruby
|
42
77
|
MotionForm.config do |config|
|
43
78
|
config.icon_font = UIFont.fontWithName('dscovr', size: 14.0)
|
44
79
|
config.section_header_color = UIColor.blueColor
|
@@ -68,3 +103,7 @@ Or install it yourself as:
|
|
68
103
|
3. Commit your changes (`git commit -am 'Add some feature'`)
|
69
104
|
4. Push to the branch (`git push origin my-new-feature`)
|
70
105
|
5. Create new Pull Request
|
106
|
+
|
107
|
+
## Thanks
|
108
|
+
|
109
|
+
[dscout](https://dscout.com) - for their sponsorship
|
data/lib/project/form/base.rb
CHANGED
@@ -102,6 +102,10 @@ module MotionForm
|
|
102
102
|
sections.map(&:rows).flatten
|
103
103
|
end
|
104
104
|
|
105
|
+
def valid?
|
106
|
+
rows.select { |row| row.is_a? TextFieldRow }.all? { |row| row.valid? }
|
107
|
+
end
|
108
|
+
|
105
109
|
def tableView(table_view, cellForRowAtIndexPath: index_path)
|
106
110
|
section = sections[index_path.section]
|
107
111
|
row = section.rows[index_path.row]
|
@@ -2,14 +2,49 @@ motion_require './base_row'
|
|
2
2
|
motion_require '../cells/text_field_cell'
|
3
3
|
|
4
4
|
class TextFieldRow < BaseRow
|
5
|
+
EMAIL_REGEX = /^[A-Z0-9a-z._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,4}$/
|
6
|
+
URL_REGEX = /https?:\/\/[\S]+/
|
7
|
+
|
5
8
|
attr_accessor :value
|
6
9
|
|
7
10
|
def initialize(key, options)
|
8
11
|
super
|
9
12
|
|
13
|
+
setup_validation
|
14
|
+
|
10
15
|
listen
|
11
16
|
end
|
12
17
|
|
18
|
+
def setup_validation
|
19
|
+
if options[:required]
|
20
|
+
validation_rules << lambda { |value| value && value != '' }
|
21
|
+
end
|
22
|
+
|
23
|
+
if options[:email]
|
24
|
+
validation_rules << lambda { |value| value && value[EMAIL_REGEX] }
|
25
|
+
end
|
26
|
+
|
27
|
+
if options[:url]
|
28
|
+
validation_rules << lambda { |value| value && value[URL_REGEX] }
|
29
|
+
end
|
30
|
+
|
31
|
+
if options[:format] && options[:format].is_a?(Regexp)
|
32
|
+
validation_rules << lambda { |value| value && value[options[:format]] }
|
33
|
+
end
|
34
|
+
|
35
|
+
if options[:validate_with] && options[:validate_with].is_a?(Proc)
|
36
|
+
validation_rules << options[:validate_with]
|
37
|
+
end
|
38
|
+
end
|
39
|
+
|
40
|
+
def valid?
|
41
|
+
validation_rules.all? { |rule| rule.call(value) }
|
42
|
+
end
|
43
|
+
|
44
|
+
def validation_rules
|
45
|
+
@validation_rules ||= []
|
46
|
+
end
|
47
|
+
|
13
48
|
def listen
|
14
49
|
observe('FormCellDidEndEditing', 'did_end_editing:')
|
15
50
|
end
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: motion-form
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
4
|
+
version: 0.0.8
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Devon Blandin
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2013-
|
11
|
+
date: 2013-09-17 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: motion-keyboard-avoiding
|
@@ -95,7 +95,7 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
95
95
|
version: '0'
|
96
96
|
requirements: []
|
97
97
|
rubyforge_project:
|
98
|
-
rubygems_version: 2.
|
98
|
+
rubygems_version: 2.1.1
|
99
99
|
signing_key:
|
100
100
|
specification_version: 4
|
101
101
|
summary: Simple DSL for RubyMotion form creation
|