missy_elliott 1.0.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/Gemfile +6 -0
- data/Gemfile.lock +19 -0
- data/LICENSE.txt +22 -0
- data/README.md +51 -0
- data/Rakefile +8 -0
- data/lib/missy_elliott.rb +25 -0
- data/lib/missy_elliott/version.rb +3 -0
- data/missy_elliott.gemspec +23 -0
- data/spec/missy_elliott_spec.rb +12 -0
- metadata +83 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: b68e4b9d6ff725c2dbbf06ff04cc350cdb940c53
|
4
|
+
data.tar.gz: 7df32050e8dd66ec61bea7491bdcd4a0037cf7bb
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 82f46937fb487a6f91d5b4223c92a30f421cc5f7af7ab717ea51ba38d35efdc3a1444e3ba88bedec308f991a822774e3c3ffea86b168f9bc946c1ebf0395fe75
|
7
|
+
data.tar.gz: fd3e24e157c9222cfd5133303d71b04dd96a326508e22d34e69c095125efa18d801a848982f1fc5d0c3bd0ec1381cf15d11339351b1c3508f2b560d6d2d2646f
|
data/Gemfile
ADDED
data/Gemfile.lock
ADDED
@@ -0,0 +1,19 @@
|
|
1
|
+
PATH
|
2
|
+
remote: .
|
3
|
+
specs:
|
4
|
+
missy_elliott (1.0.0)
|
5
|
+
|
6
|
+
GEM
|
7
|
+
remote: https://rubygems.org/
|
8
|
+
specs:
|
9
|
+
minitest (5.5.1)
|
10
|
+
rake (10.4.2)
|
11
|
+
|
12
|
+
PLATFORMS
|
13
|
+
ruby
|
14
|
+
|
15
|
+
DEPENDENCIES
|
16
|
+
bundler (~> 1.7)
|
17
|
+
minitest
|
18
|
+
missy_elliott!
|
19
|
+
rake (~> 10.0)
|
data/LICENSE.txt
ADDED
@@ -0,0 +1,22 @@
|
|
1
|
+
Copyright (c) 2015 Tom Lord
|
2
|
+
|
3
|
+
MIT License
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
6
|
+
a copy of this software and associated documentation files (the
|
7
|
+
"Software"), to deal in the Software without restriction, including
|
8
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
9
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
10
|
+
permit persons to whom the Software is furnished to do so, subject to
|
11
|
+
the following conditions:
|
12
|
+
|
13
|
+
The above copyright notice and this permission notice shall be
|
14
|
+
included in all copies or substantial portions of the Software.
|
15
|
+
|
16
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
17
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
18
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
19
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
20
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
21
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
22
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,51 @@
|
|
1
|
+
# MissyElliott
|
2
|
+
|
3
|
+

|
4
|
+
|
5
|
+
```ruby
|
6
|
+
MissyElliott.encode("Example") # => "\xAE\xF0\xBC\xA4\xF8\xE4\xAC"
|
7
|
+
|
8
|
+
#"Example"
|
9
|
+
#--> ["E", "x", "a", "m", "p", "l", "e"]
|
10
|
+
#--> [69, 120, 97, 109, 112, 108, 101]
|
11
|
+
#--> ["01000101", "01111000", "01100001", "01101101", "01110000", "01101100", "01100101"]
|
12
|
+
# Shift yo bits down
|
13
|
+
#--> ["10001010", "11110000", "11000010", "11011010", "11100000", "11011000", "11001010"]
|
14
|
+
# Flip it
|
15
|
+
#--> ["01110101", "00001111", "00111101", "00100101", "00011111", "00100111", "00110101"]
|
16
|
+
# And reverse it
|
17
|
+
#--> ["10101110", "11110000", "10111100", "10100100", "11111000", "11100100", "10101100"]
|
18
|
+
#--> [174, 240, 188, 164, 248, 228, 172]
|
19
|
+
#--> ["\xAE", "\xF0", "\xBC", "\xA4", "\xF8", "\xE4", "\xAC"]
|
20
|
+
#--> ["\xAE\xF0\xBC\xA4\xF8\xE4\xAC"]
|
21
|
+
|
22
|
+
MissyElliott.decode("\xAE\xF0\xBC\xA4\xF8\xE4\xAC") # => "Example"
|
23
|
+
```
|
24
|
+
|
25
|
+
This gem is a blatant rip-off of [an old XKCD comic](http://xkcd.com/153/):
|
26
|
+
|
27
|
+
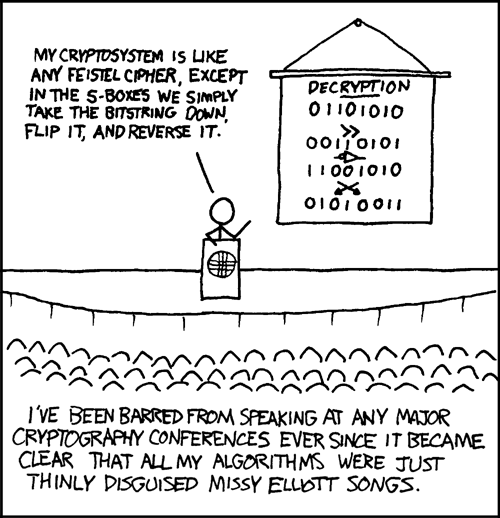
|
28
|
+
|
29
|
+
## Installation
|
30
|
+
|
31
|
+
Add this line to your application's Gemfile:
|
32
|
+
|
33
|
+
```ruby
|
34
|
+
gem 'missy_elliott'
|
35
|
+
```
|
36
|
+
|
37
|
+
And then execute:
|
38
|
+
|
39
|
+
$ bundle
|
40
|
+
|
41
|
+
Or install it yourself as:
|
42
|
+
|
43
|
+
$ gem install missy_elliott
|
44
|
+
|
45
|
+
## Contributing
|
46
|
+
|
47
|
+
1. Got a bug fix?
|
48
|
+
2. You'd better work on it ( https://github.com/tom-lord/missy_elliott/fork )
|
49
|
+
3. Create your feature branch (`git checkout -b my-new-feature`)
|
50
|
+
4. Commit it (`git commit -am 'Add some feature'`)
|
51
|
+
5. And converse it (`git push origin my-new-feature`)
|
data/Rakefile
ADDED
@@ -0,0 +1,25 @@
|
|
1
|
+
module MissyElliott
|
2
|
+
def self.encode(input)
|
3
|
+
input.bytes.map do |byte|
|
4
|
+
bits = byte.to_s(2).rjust(8, "0").split("")
|
5
|
+
bits << bits.shift # Shift yo bits down
|
6
|
+
flip_it(bits).reverse.join.to_i(2).chr # Flip it and reverse it
|
7
|
+
end.join
|
8
|
+
end
|
9
|
+
|
10
|
+
def self.decode(input)
|
11
|
+
input.bytes.map do |byte|
|
12
|
+
bits = byte.to_s(2).rjust(8, "0").split("")
|
13
|
+
bits = flip_it(bits.reverse) # Reverse it and flip it
|
14
|
+
bits.unshift(bits.pop).join.to_i(2).chr # Shift yo bits up
|
15
|
+
end.join
|
16
|
+
end
|
17
|
+
|
18
|
+
private
|
19
|
+
def self.flip_it(bits)
|
20
|
+
bits.map do |bit|
|
21
|
+
bit == "1" ? "0" : "1"
|
22
|
+
end
|
23
|
+
end
|
24
|
+
end
|
25
|
+
|
@@ -0,0 +1,23 @@
|
|
1
|
+
# coding: utf-8
|
2
|
+
lib = File.expand_path('../lib', __FILE__)
|
3
|
+
$LOAD_PATH.unshift(lib) unless $LOAD_PATH.include?(lib)
|
4
|
+
require 'missy_elliott/version'
|
5
|
+
|
6
|
+
Gem::Specification.new do |spec|
|
7
|
+
spec.name = "missy_elliott"
|
8
|
+
spec.version = MissyElliott::VERSION
|
9
|
+
spec.authors = ["Tom Lord"]
|
10
|
+
spec.email = ["tom.lord@gmail.com"]
|
11
|
+
spec.summary = %q{Applies the Missy Elliott encryption algorithm on a string}
|
12
|
+
spec.description = %q{Got a big string? Let me search it. Shift yo bits down, flip it and reverse it.}
|
13
|
+
spec.homepage = ""
|
14
|
+
spec.license = "MIT"
|
15
|
+
|
16
|
+
spec.files = `git ls-files -z`.split("\x0")
|
17
|
+
spec.executables = spec.files.grep(%r{^bin/}) { |f| File.basename(f) }
|
18
|
+
spec.test_files = spec.files.grep(%r{^(test|spec|features)/})
|
19
|
+
spec.require_paths = ["lib"]
|
20
|
+
|
21
|
+
spec.add_development_dependency "bundler", "~> 1.7"
|
22
|
+
spec.add_development_dependency "rake", "~> 10.0"
|
23
|
+
end
|
@@ -0,0 +1,12 @@
|
|
1
|
+
require 'minitest/autorun'
|
2
|
+
require './lib/missy_elliott.rb'
|
3
|
+
|
4
|
+
describe "MissyElliott" do
|
5
|
+
it "Must be worth it" do
|
6
|
+
original = "Is it worth it?"
|
7
|
+
encoded = MissyElliott.encode(original)
|
8
|
+
decoded = MissyElliott.decode(encoded)
|
9
|
+
original.must_equal decoded
|
10
|
+
end
|
11
|
+
end
|
12
|
+
|
metadata
ADDED
@@ -0,0 +1,83 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: missy_elliott
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 1.0.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Tom Lord
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2015-03-21 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: bundler
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - "~>"
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '1.7'
|
20
|
+
type: :development
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - "~>"
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '1.7'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: rake
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - "~>"
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '10.0'
|
34
|
+
type: :development
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - "~>"
|
39
|
+
- !ruby/object:Gem::Version
|
40
|
+
version: '10.0'
|
41
|
+
description: Got a big string? Let me search it. Shift yo bits down, flip it and reverse
|
42
|
+
it.
|
43
|
+
email:
|
44
|
+
- tom.lord@gmail.com
|
45
|
+
executables: []
|
46
|
+
extensions: []
|
47
|
+
extra_rdoc_files: []
|
48
|
+
files:
|
49
|
+
- Gemfile
|
50
|
+
- Gemfile.lock
|
51
|
+
- LICENSE.txt
|
52
|
+
- README.md
|
53
|
+
- Rakefile
|
54
|
+
- lib/missy_elliott.rb
|
55
|
+
- lib/missy_elliott/version.rb
|
56
|
+
- missy_elliott.gemspec
|
57
|
+
- spec/missy_elliott_spec.rb
|
58
|
+
homepage: ''
|
59
|
+
licenses:
|
60
|
+
- MIT
|
61
|
+
metadata: {}
|
62
|
+
post_install_message:
|
63
|
+
rdoc_options: []
|
64
|
+
require_paths:
|
65
|
+
- lib
|
66
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
67
|
+
requirements:
|
68
|
+
- - ">="
|
69
|
+
- !ruby/object:Gem::Version
|
70
|
+
version: '0'
|
71
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
72
|
+
requirements:
|
73
|
+
- - ">="
|
74
|
+
- !ruby/object:Gem::Version
|
75
|
+
version: '0'
|
76
|
+
requirements: []
|
77
|
+
rubyforge_project:
|
78
|
+
rubygems_version: 2.4.5
|
79
|
+
signing_key:
|
80
|
+
specification_version: 4
|
81
|
+
summary: Applies the Missy Elliott encryption algorithm on a string
|
82
|
+
test_files:
|
83
|
+
- spec/missy_elliott_spec.rb
|