merit 0.2.4 → 0.2.5
Sign up to get free protection for your applications and to get access to all the features.
- data/README.markdown +154 -0
- data/lib/merit/controller_extensions.rb +1 -1
- data/lib/merit/rules_points.rb +4 -1
- metadata +3 -3
- data/README.rdoc +0 -136
data/README.markdown
ADDED
@@ -0,0 +1,154 @@
|
|
1
|
+
# Merit Gem: Reputation rules (badges, points and rankings) for Rails applications
|
2
|
+
|
3
|
+
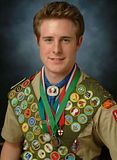
|
4
|
+
|
5
|
+
|
6
|
+
# Installation
|
7
|
+
|
8
|
+
1. Add 'merit' to your Gemfile
|
9
|
+
2. Run <tt>rails g merit:install</tt>
|
10
|
+
3. Run <tt>rails g merit MODEL_NAME</tt>
|
11
|
+
4. Run <tt>rake db:migrate</tt>
|
12
|
+
5. Configure reputation rules for your application
|
13
|
+
|
14
|
+
---
|
15
|
+
|
16
|
+
# Defining badge rules
|
17
|
+
|
18
|
+
You may give badges to any resource on your application if some condition
|
19
|
+
holds. Badges may have levels, and may be temporary. Define rules on
|
20
|
+
<tt>app/models/merit_badge_rules.rb</tt>:
|
21
|
+
|
22
|
+
<tt>grant_on</tt> accepts:
|
23
|
+
|
24
|
+
* <tt>'controller#action'</tt> string (similar to Rails routes)
|
25
|
+
* <tt>:badge</tt> for badge name
|
26
|
+
* <tt>:level</tt> for badge level
|
27
|
+
* <tt>:to</tt> method name over target_object which obtains object to badge
|
28
|
+
* <tt>:model_name</tt> (string) define controller's name if it differs from
|
29
|
+
the model (like <tt>RegistrationsController</tt> for <tt>User</tt> model).
|
30
|
+
* <tt>:temporary</tt> (boolean) if the receiver had the badge but the
|
31
|
+
condition doesn't hold anymore, remove it. <tt>false</tt> by default (badges
|
32
|
+
are kept forever).
|
33
|
+
* <tt>&block</tt>
|
34
|
+
* empty (always grants)
|
35
|
+
* a block which evaluates to boolean (recieves target object as parameter)
|
36
|
+
* a block with a hash composed of methods to run on the target object with
|
37
|
+
expected values
|
38
|
+
|
39
|
+
## Examples
|
40
|
+
|
41
|
+
grant_on 'comments#vote', :badge => 'relevant-commenter', :to => :user do
|
42
|
+
{ :votes => 5 }
|
43
|
+
end
|
44
|
+
|
45
|
+
grant_on ['users#create', 'users#update'], :badge => 'autobiographer', :temporary => true do |user|
|
46
|
+
user.name.present? && user.address.present?
|
47
|
+
end
|
48
|
+
|
49
|
+
---
|
50
|
+
|
51
|
+
# Defining point rules
|
52
|
+
|
53
|
+
Points are a simple integer value which are given to "meritable" resources.
|
54
|
+
They are given on actions-triggered, either to the action user or to the
|
55
|
+
method(s) defined in the <tt>:to</tt> option. Define rules on
|
56
|
+
<tt>app/models/merit_point_rules.rb</tt>:
|
57
|
+
|
58
|
+
## Examples
|
59
|
+
|
60
|
+
score 10, :on => [
|
61
|
+
'users#update'
|
62
|
+
]
|
63
|
+
|
64
|
+
score 15, :on => 'reviews#create', :to => [:reviewer, :reviewed]
|
65
|
+
|
66
|
+
score 20, :on => [
|
67
|
+
'comments#create',
|
68
|
+
'photos#create'
|
69
|
+
]
|
70
|
+
|
71
|
+
---
|
72
|
+
|
73
|
+
# Defining rank rules
|
74
|
+
|
75
|
+
Rankings are very similar to badges. They give "badges" which have a hierarchy
|
76
|
+
defined by <tt>level</tt>'s lexicografical order (greater is better). If a
|
77
|
+
rank is granted, lower level ranks are taken off. 5 stars is a common ranking
|
78
|
+
use case.
|
79
|
+
|
80
|
+
They are not given at specified actions like badges, you should define a cron
|
81
|
+
job to test if ranks are to be granted.
|
82
|
+
|
83
|
+
Define rules on <tt>app/models/merit_rank_rules.rb</tt>:
|
84
|
+
|
85
|
+
<tt>set_rank</tt> accepts:
|
86
|
+
|
87
|
+
* <tt>badge_name</tt> name of this ranking
|
88
|
+
* <tt>:level</tt> ranking level (greater is better)
|
89
|
+
* <tt>:to</tt> model or scope to check if new rankings apply
|
90
|
+
|
91
|
+
Check for rules on a rake task executed in background like:
|
92
|
+
|
93
|
+
task :cron => :environment do
|
94
|
+
MeritRankRules.new.check_rank_rules
|
95
|
+
end
|
96
|
+
|
97
|
+
|
98
|
+
## Examples
|
99
|
+
|
100
|
+
set_rank :stars, :level => 2, :to => Commiter.active do |commiter|
|
101
|
+
commiter.branches > 1 && commiter.followers >= 10
|
102
|
+
end
|
103
|
+
|
104
|
+
set_rank :stars, :level => 3, :to => Commiter.active do |commiter|
|
105
|
+
commiter.branches > 2 && commiter.followers >= 20
|
106
|
+
end
|
107
|
+
|
108
|
+
---
|
109
|
+
|
110
|
+
# Grant manually
|
111
|
+
|
112
|
+
You may also add badges/rank "by hand" from controller actions:
|
113
|
+
|
114
|
+
Badge.find(3).grant_to(current_user)
|
115
|
+
|
116
|
+
---
|
117
|
+
|
118
|
+
# Upgrade to 0.2.0
|
119
|
+
|
120
|
+
Added <tt>had_errors</tt> boolean attribute to <tt>merit_actions</tt> table.
|
121
|
+
|
122
|
+
---
|
123
|
+
|
124
|
+
# Test application
|
125
|
+
|
126
|
+
To run tests follow:
|
127
|
+
|
128
|
+
cd test/dummy
|
129
|
+
rails g merit:install # Next n's for not overriding already defined rules
|
130
|
+
n
|
131
|
+
n
|
132
|
+
n
|
133
|
+
rails g merit user
|
134
|
+
rake db:migrate ; rake db:seed
|
135
|
+
cd ../.. ; rake test
|
136
|
+
|
137
|
+
---
|
138
|
+
|
139
|
+
# To-do list
|
140
|
+
|
141
|
+
* Ranking should not be badges, so .badges doesn't return them (2-stars
|
142
|
+
shouldn't be badge).
|
143
|
+
* <tt>grep -r 'FIXME\|TODO' .</tt>
|
144
|
+
* :value parameter (for star voting for example) should be configurable
|
145
|
+
(depends on params[:value] on the controller).
|
146
|
+
* Make fixtures for integration testing (now creating objects on test file!).
|
147
|
+
|
148
|
+
---
|
149
|
+
|
150
|
+
# Contributors
|
151
|
+
|
152
|
+
* [A4bandas media](https://github.com/a4bandas)
|
153
|
+
* [Juan Schwindt](https://github.com/jschwindt)
|
154
|
+
* [Eric Knudtson](https://github.com/ek)
|
@@ -10,7 +10,7 @@ module Merit
|
|
10
10
|
point_rules = ::MeritPointRules.new
|
11
11
|
if badge_rules.defined_rules[action].present? || point_rules.actions_to_point[action].present?
|
12
12
|
target_id = params[:id]
|
13
|
-
# TODO: target_object should be configurable (now it's singularized controller name)
|
13
|
+
# TODO: target_object should be configurable (now it's singularized controller name)
|
14
14
|
target_object = instance_variable_get(:"@#{controller_name.singularize}")
|
15
15
|
unless target_id =~ /^[0-9]+$/ # id nil, or string (friendly_id)?
|
16
16
|
target_id = target_object.try(:id)
|
data/lib/merit/rules_points.rb
CHANGED
@@ -11,7 +11,10 @@ module Merit
|
|
11
11
|
options[:to] ||= [:action_user]
|
12
12
|
targets = options[:to].kind_of?(Array) ? options[:to] : [options[:to]]
|
13
13
|
actions.each do |action|
|
14
|
-
actions_to_point[action] = {
|
14
|
+
actions_to_point[action] = {
|
15
|
+
:to => targets,
|
16
|
+
:score => points
|
17
|
+
}
|
15
18
|
end
|
16
19
|
end
|
17
20
|
|
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: merit
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.2.
|
4
|
+
version: 0.2.5
|
5
5
|
prerelease:
|
6
6
|
platform: ruby
|
7
7
|
authors:
|
@@ -9,7 +9,7 @@ authors:
|
|
9
9
|
autorequire:
|
10
10
|
bindir: bin
|
11
11
|
cert_chain: []
|
12
|
-
date: 2012-02-
|
12
|
+
date: 2012-02-29 00:00:00.000000000 Z
|
13
13
|
dependencies: []
|
14
14
|
description: General reputation Rails engine.
|
15
15
|
email: tutecosta@gmail.com
|
@@ -45,7 +45,7 @@ files:
|
|
45
45
|
- MIT-LICENSE
|
46
46
|
- Rakefile
|
47
47
|
- Gemfile
|
48
|
-
- README.
|
48
|
+
- README.markdown
|
49
49
|
homepage:
|
50
50
|
licenses: []
|
51
51
|
post_install_message:
|
data/README.rdoc
DELETED
@@ -1,136 +0,0 @@
|
|
1
|
-
= Merit Rails Gem
|
2
|
-
|
3
|
-
Define reputation for users and data on your application.
|
4
|
-
|
5
|
-
http://i567.photobucket.com/albums/ss118/DeuceBigglebags/th_nspot26_300.jpg
|
6
|
-
|
7
|
-
|
8
|
-
= Installation
|
9
|
-
|
10
|
-
1. Add 'merit' to your Gemfile
|
11
|
-
2. Run +rails+ +g+ +merit+:+install+
|
12
|
-
3. Run +rails+ +g+ +merit+ +MODEL_NAME+
|
13
|
-
4. Run +rake+ +db+:+migrate+
|
14
|
-
5. Configure reputation rules for your application
|
15
|
-
|
16
|
-
|
17
|
-
= Defining badge rules
|
18
|
-
|
19
|
-
You may give badges to any resource on your application if some condition
|
20
|
-
holds. Badges may have levels, and may be temporary.
|
21
|
-
|
22
|
-
Define rules on +app/models/merit_badge_rules.rb+:
|
23
|
-
|
24
|
-
+grant_on+ accepts:
|
25
|
-
* +controller+#+action+ string (similar to Rails routes)
|
26
|
-
* :+badge+ for badge name
|
27
|
-
* :+level+ for badge level
|
28
|
-
* :+to+: method name over target_object which obtains user to badge.
|
29
|
-
* :+model_name+ (string): when controller's name differs from the model being
|
30
|
-
worked (like RegistrationsController for User model).
|
31
|
-
* :+temporary+ (boolean): if the condition doesn't hold and the receiver had
|
32
|
-
the badge, it gets removed. +false+ by default (badges are kept forever).
|
33
|
-
* &+block+
|
34
|
-
* empty (always grants)
|
35
|
-
* a block which evaluates to boolean (recieves target object as parameter)
|
36
|
-
* a block with a hash composed of methods to run on the target object with
|
37
|
-
expected values
|
38
|
-
|
39
|
-
== Examples
|
40
|
-
|
41
|
-
grant_on 'comments#vote', :badge => 'relevant-commenter', :to => :user do
|
42
|
-
{ :votes => 5 }
|
43
|
-
end
|
44
|
-
|
45
|
-
grant_on ['users#create', 'users#update'], :badge => 'autobiographer', :temporary => true do |user|
|
46
|
-
user.name.present? && user.address.present?
|
47
|
-
end
|
48
|
-
|
49
|
-
|
50
|
-
= Defining point rules
|
51
|
-
|
52
|
-
Points are a simple integer value which are given to "meritable" resources.
|
53
|
-
They are given on actions-triggered, either to the action user or to the
|
54
|
-
method (or array of methods) defined in the +:to+ option.
|
55
|
-
|
56
|
-
Define rules on +app/models/merit_point_rules.rb+:
|
57
|
-
|
58
|
-
== Examples
|
59
|
-
|
60
|
-
score 10, :on => [
|
61
|
-
'users#update'
|
62
|
-
]
|
63
|
-
|
64
|
-
score 15, :on => 'reviews#create', :to => [:reviewer, :reviewed]
|
65
|
-
|
66
|
-
score 20, :on => [
|
67
|
-
'comments#create',
|
68
|
-
'photos#create'
|
69
|
-
]
|
70
|
-
|
71
|
-
|
72
|
-
= Defining rank rules
|
73
|
-
|
74
|
-
Rankings are very similar to badges. They give "badges" which have a hierarchy
|
75
|
-
defined by +level+'s lexicografical order (greater is better). If a rank is
|
76
|
-
granted, lower level ranks are taken off. 5 stars is a common ranking use
|
77
|
-
case.
|
78
|
-
|
79
|
-
They are not given at specified actions like badges, you should define a cron
|
80
|
-
job to test if ranks are to be granted.
|
81
|
-
|
82
|
-
Define rules on +app/models/merit_rank_rules.rb+:
|
83
|
-
|
84
|
-
+set_rank+ accepts:
|
85
|
-
* +badge_name+ name of this ranking
|
86
|
-
* :+level+ ranking level (greater is better)
|
87
|
-
* :+to+ model or scope to check if new rankings apply
|
88
|
-
|
89
|
-
Check for rules on a rake task executed in background like:
|
90
|
-
task :cron => :environment do
|
91
|
-
MeritRankRules.new.check_rank_rules
|
92
|
-
end
|
93
|
-
|
94
|
-
|
95
|
-
== Examples
|
96
|
-
|
97
|
-
set_rank :stars, :level => 2, :to => Commiter.active do |commiter|
|
98
|
-
commiter.branches > 1 && commiter.followers >= 10
|
99
|
-
end
|
100
|
-
|
101
|
-
set_rank :stars, :level => 3, :to => Commiter.active do |commiter|
|
102
|
-
commiter.branches > 2 && commiter.followers >= 20
|
103
|
-
end
|
104
|
-
|
105
|
-
|
106
|
-
= Grant manually
|
107
|
-
|
108
|
-
You may also add badges/rank "by hand" from controller actions:
|
109
|
-
Badge.find(3).grant_to(current_user)
|
110
|
-
|
111
|
-
|
112
|
-
= Upgrade to 0.2.0
|
113
|
-
|
114
|
-
Added +had_errors+ boolean attribute to +merit_actions+ table.
|
115
|
-
|
116
|
-
|
117
|
-
= Test application
|
118
|
-
|
119
|
-
To run the test application inside this gem follow:
|
120
|
-
cd test/dummy
|
121
|
-
rails g merit:install
|
122
|
-
rails g merit user
|
123
|
-
rake db:migrate ; rake db:seed
|
124
|
-
rails s
|
125
|
-
|
126
|
-
|
127
|
-
= To-do list
|
128
|
-
|
129
|
-
* Test points granting with different options.
|
130
|
-
* Test model_name attribute for badge_rules.
|
131
|
-
* Add model_name option on rank and point rules.
|
132
|
-
* Ranking should not be badges, so .badges doesn't return them (2-stars shouldn't be badge).
|
133
|
-
* grep -r 'FIXME\|TODO' .
|
134
|
-
* :value parameter (for star voting for example) should be configurable (depends
|
135
|
-
on params[:value] on the controller).
|
136
|
-
* Make fixtures for integration testing (now creating objects on test file!).
|