mc_duck 0.0.1
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/MIT-LICENSE +20 -0
- data/README.md +43 -0
- data/Rakefile +9 -0
- data/bin/mc_duck +5 -0
- data/lib/mc_duck.rb +3 -0
- data/lib/mc_duck/backup.rb +25 -0
- data/lib/mc_duck/command_line.rb +51 -0
- data/lib/mc_duck/s3_manager.rb +54 -0
- data/lib/mc_duck/version.rb +3 -0
- metadata +109 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: 2e4c9b22dafed37d818d289001e72357f0a9b550
|
4
|
+
data.tar.gz: ac5773ef61a12e730acd982b95e9906f4143d192
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: cc36914c2ab2a43ba75c5b91ba91e912b0859a3066d37958dfb346fb872e12e2b54988f8b5ced8612ff4c291e48958b473bc756dd097ca31094048618748e37c
|
7
|
+
data.tar.gz: b5357e5b377be9d0543a473bed279edf20496a18c36e91ea4d0b3750c62b1770aca980dd2b4dd569c3df576d2b90263ecdd40458cd726cd04038a2d0bf04b2e3
|
data/MIT-LICENSE
ADDED
@@ -0,0 +1,20 @@
|
|
1
|
+
Copyright 2012 Tiago Scolari
|
2
|
+
|
3
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
4
|
+
a copy of this software and associated documentation files (the
|
5
|
+
"Software"), to deal in the Software without restriction, including
|
6
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
7
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
8
|
+
permit persons to whom the Software is furnished to do so, subject to
|
9
|
+
the following conditions:
|
10
|
+
|
11
|
+
The above copyright notice and this permission notice shall be
|
12
|
+
included in all copies or substantial portions of the Software.
|
13
|
+
|
14
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
15
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
16
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
17
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
18
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
19
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
20
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,43 @@
|
|
1
|
+
[](https://codeclimate.com/github/tscolari/mc_duck)
|
2
|
+
|
3
|
+
McDuck
|
4
|
+
====
|
5
|
+
|
6
|
+
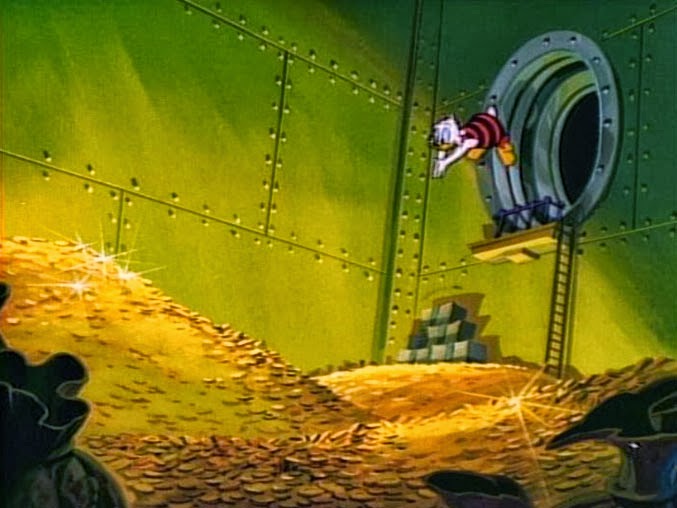
|
7
|
+
|
8
|
+
Single "file" backup tool with versions to S3.
|
9
|
+
|
10
|
+
The objective is to backup individual files with versions on S3,
|
11
|
+
for example keeping database backups.
|
12
|
+
|
13
|
+
```
|
14
|
+
Usage: mc_duck OPTIONS
|
15
|
+
-k, --aws-key-id KEY_ID AWS key id
|
16
|
+
-a, --aws-access-key ACCESS_KEY AWS access key
|
17
|
+
-b, --s3-bucket BUCKET_NAME S3 bucket name
|
18
|
+
-v, --verbose Verbose
|
19
|
+
-f, --file_name REMOTE_FILENAME Target folder in s3, e.g. 'production'
|
20
|
+
--keep [10] Number of versions to keep
|
21
|
+
```
|
22
|
+
|
23
|
+
Example:
|
24
|
+
|
25
|
+
```
|
26
|
+
pg_dump ... | gzip | mc_duck -k $AWS_KEY -a $AWS_SECRET -b my_bucket -f backups/postgres.gz
|
27
|
+
```
|
28
|
+
|
29
|
+
this will create inside the bucket:
|
30
|
+
|
31
|
+
```
|
32
|
+
/backups/postgres.gz/TIMESTAMP
|
33
|
+
```
|
34
|
+
|
35
|
+
the `--keep` option controls how many versions do you want to keep, by default it keeps 10.
|
36
|
+
|
37
|
+
Theoretically it can backup anything you can pipe through the command line.
|
38
|
+
|
39
|
+
Other examples:
|
40
|
+
|
41
|
+
* `cat big_backup.gz | mc_duck -k $AWS_KEY -a $AWS_SECRET -b my_bucket -f backups/postgres.gz`
|
42
|
+
* `tar cz | mc_duck -k $AWS_KEY -a $AWS_SECRET -b my_bucket -f backups/postgres.gz`
|
43
|
+
|
data/Rakefile
ADDED
data/bin/mc_duck
ADDED
data/lib/mc_duck.rb
ADDED
@@ -0,0 +1,25 @@
|
|
1
|
+
module McDuck
|
2
|
+
class Backup
|
3
|
+
|
4
|
+
def initialize(key_id, access_key, logger = Logger.new)
|
5
|
+
@logger = logger
|
6
|
+
@s3_manager = S3Mananger.new(key_id, access_key, @logger)
|
7
|
+
end
|
8
|
+
|
9
|
+
def run(bucket_name, file_name, file_content, keep)
|
10
|
+
backup(bucket_name, file_name, file_content)
|
11
|
+
cleanup(bucket_name, file_name, keep || 10)
|
12
|
+
end
|
13
|
+
|
14
|
+
private
|
15
|
+
|
16
|
+
def backup(bucket, backup_file_name, content)
|
17
|
+
@s3_manager.upload(bucket, backup_file_name, content)
|
18
|
+
end
|
19
|
+
|
20
|
+
def cleanup(bucket_name, file_name, keep)
|
21
|
+
@s3_manager.cleanup(bucket_name, file_name, keep)
|
22
|
+
end
|
23
|
+
|
24
|
+
end
|
25
|
+
end
|
@@ -0,0 +1,51 @@
|
|
1
|
+
module McDuck
|
2
|
+
class CommandLine
|
3
|
+
require 'optparse'
|
4
|
+
require 'logger'
|
5
|
+
|
6
|
+
def run(args, file)
|
7
|
+
logger = Logger.new($stdout)
|
8
|
+
logger.level = Logger::WARN
|
9
|
+
|
10
|
+
options = fetch_options(args, logger)
|
11
|
+
Backup.new(options.delete(:key_id), options.delete(:access_key), logger).
|
12
|
+
run(options.delete(:bucket), options.delete(:file_name), file.read, options.delete(:keep))
|
13
|
+
end
|
14
|
+
|
15
|
+
private
|
16
|
+
|
17
|
+
def fetch_options(args, logger)
|
18
|
+
Hash.new.tap do |options|
|
19
|
+
OptionParser.new do |opts|
|
20
|
+
opts.banner = 'Usage: s3ckup OPTIONS'
|
21
|
+
|
22
|
+
opts.on('-k', '--aws-key-id KEY_ID', 'AWS key id') do |key_id|
|
23
|
+
options[:key_id] = key_id
|
24
|
+
end
|
25
|
+
|
26
|
+
opts.on('-a', '--aws-access-key ACCESS_KEY', 'AWS access key') do |access_key|
|
27
|
+
options[:access_key] = access_key
|
28
|
+
end
|
29
|
+
|
30
|
+
opts.on('-b', '--s3-bucket BUCKET_NAME', 'S3 bucket name') do |bucket_name|
|
31
|
+
options[:bucket] = bucket_name
|
32
|
+
end
|
33
|
+
|
34
|
+
opts.on('-v', '--verbose', 'Verbose') do
|
35
|
+
logger.level = Logger::INFO
|
36
|
+
end
|
37
|
+
|
38
|
+
opts.on('--keep [10]', 'Backups to keep') do |keep|
|
39
|
+
options[:keep] = keep
|
40
|
+
end
|
41
|
+
|
42
|
+
opts.on('-f', '--file_name REMOTE_FILE_NAME', 'Backups filename in S3') do |file_name|
|
43
|
+
options[:file_name] = file_name
|
44
|
+
end
|
45
|
+
|
46
|
+
end.parse!(args)
|
47
|
+
end
|
48
|
+
end
|
49
|
+
|
50
|
+
end
|
51
|
+
end
|
@@ -0,0 +1,54 @@
|
|
1
|
+
require 'aws/s3'
|
2
|
+
|
3
|
+
module McDuck
|
4
|
+
class S3Mananger
|
5
|
+
|
6
|
+
def initialize(key_id, access_key, logger = Logger.new)
|
7
|
+
connect!(key_id, access_key)
|
8
|
+
@logger = logger
|
9
|
+
end
|
10
|
+
|
11
|
+
def upload(bucket, file_name, file_content)
|
12
|
+
target_name = create_target_name(file_name)
|
13
|
+
@logger.info("Uploading file: #{target_name}...")
|
14
|
+
AWS::S3::S3Object.store(target_name, file_content, bucket)
|
15
|
+
end
|
16
|
+
|
17
|
+
def backups_list(bucket_name, file_name)
|
18
|
+
bucket = AWS::S3::Bucket.find(bucket_name)
|
19
|
+
backups = bucket.map(&:key).sort
|
20
|
+
backups.select { |file| file.include?(file_name) }
|
21
|
+
end
|
22
|
+
|
23
|
+
def cleanup(bucket_name, file_name, backups_to_keep = 10)
|
24
|
+
expired_backups = backups_to_delete(bucket_name, file_name, backups_to_keep)
|
25
|
+
expired_backups.each do |backup|
|
26
|
+
@logger.info("Deleting old backup: #{backup}...")
|
27
|
+
AWS::S3::S3Object.delete backup, bucket_name
|
28
|
+
end
|
29
|
+
end
|
30
|
+
|
31
|
+
private
|
32
|
+
|
33
|
+
def backups_to_delete(bucket, file_name, backups_to_keep)
|
34
|
+
backups = backups_list(bucket, file_name)
|
35
|
+
backups - backups.last(backups_to_keep.to_i)
|
36
|
+
end
|
37
|
+
|
38
|
+
def create_target_name(file_name)
|
39
|
+
"#{file_name}/#{timestamp}"
|
40
|
+
end
|
41
|
+
|
42
|
+
def timestamp
|
43
|
+
@timestamp ||= Time.now.utc.strftime("%Y%m%d%H%M%S")
|
44
|
+
end
|
45
|
+
|
46
|
+
def connect!(key_id, access_key)
|
47
|
+
AWS::S3::Base.establish_connection!(
|
48
|
+
:access_key_id => key_id,
|
49
|
+
:secret_access_key => access_key
|
50
|
+
)
|
51
|
+
end
|
52
|
+
|
53
|
+
end
|
54
|
+
end
|
metadata
ADDED
@@ -0,0 +1,109 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: mc_duck
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.1
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Tiago Scolari
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2014-08-23 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: aws-s3
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - ">="
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '0'
|
20
|
+
type: :runtime
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - ">="
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '0'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: pry
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - ">="
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '0'
|
34
|
+
type: :development
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - ">="
|
39
|
+
- !ruby/object:Gem::Version
|
40
|
+
version: '0'
|
41
|
+
- !ruby/object:Gem::Dependency
|
42
|
+
name: rspec
|
43
|
+
requirement: !ruby/object:Gem::Requirement
|
44
|
+
requirements:
|
45
|
+
- - ">="
|
46
|
+
- !ruby/object:Gem::Version
|
47
|
+
version: '0'
|
48
|
+
type: :development
|
49
|
+
prerelease: false
|
50
|
+
version_requirements: !ruby/object:Gem::Requirement
|
51
|
+
requirements:
|
52
|
+
- - ">="
|
53
|
+
- !ruby/object:Gem::Version
|
54
|
+
version: '0'
|
55
|
+
- !ruby/object:Gem::Dependency
|
56
|
+
name: pry-nav
|
57
|
+
requirement: !ruby/object:Gem::Requirement
|
58
|
+
requirements:
|
59
|
+
- - ">="
|
60
|
+
- !ruby/object:Gem::Version
|
61
|
+
version: '0'
|
62
|
+
type: :development
|
63
|
+
prerelease: false
|
64
|
+
version_requirements: !ruby/object:Gem::Requirement
|
65
|
+
requirements:
|
66
|
+
- - ">="
|
67
|
+
- !ruby/object:Gem::Version
|
68
|
+
version: '0'
|
69
|
+
description: Uploads command outputs to s3.
|
70
|
+
email:
|
71
|
+
- tscolari@gmail.com
|
72
|
+
executables:
|
73
|
+
- mc_duck
|
74
|
+
extensions: []
|
75
|
+
extra_rdoc_files: []
|
76
|
+
files:
|
77
|
+
- MIT-LICENSE
|
78
|
+
- README.md
|
79
|
+
- Rakefile
|
80
|
+
- bin/mc_duck
|
81
|
+
- lib/mc_duck.rb
|
82
|
+
- lib/mc_duck/backup.rb
|
83
|
+
- lib/mc_duck/command_line.rb
|
84
|
+
- lib/mc_duck/s3_manager.rb
|
85
|
+
- lib/mc_duck/version.rb
|
86
|
+
homepage: https://github.com/tscolari/s3ckup
|
87
|
+
licenses: []
|
88
|
+
metadata: {}
|
89
|
+
post_install_message:
|
90
|
+
rdoc_options: []
|
91
|
+
require_paths:
|
92
|
+
- lib
|
93
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
94
|
+
requirements:
|
95
|
+
- - ">="
|
96
|
+
- !ruby/object:Gem::Version
|
97
|
+
version: '0'
|
98
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
99
|
+
requirements:
|
100
|
+
- - ">="
|
101
|
+
- !ruby/object:Gem::Version
|
102
|
+
version: '0'
|
103
|
+
requirements: []
|
104
|
+
rubyforge_project:
|
105
|
+
rubygems_version: 2.2.2
|
106
|
+
signing_key:
|
107
|
+
specification_version: 4
|
108
|
+
summary: Versioned file backup tool to S3.
|
109
|
+
test_files: []
|