konamio 0.0.3 → 0.1.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +11 -16
- data/lib/konamio/sequence/listener.rb +24 -10
- data/lib/konamio/sequence/requisition.rb +35 -16
- data/lib/konamio/version.rb +1 -1
- data/test/konamio/sequence/listener_test.rb +1 -1
- data/test/konamio/sequence/requisition_test.rb +3 -0
- metadata +2 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 22b984e3f6c89a0d7cda0f184d2ec96290a77ee3
|
4
|
+
data.tar.gz: 9eca8ee78b957a7f9dff172b7bd8f3f241040ee8
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 29ea0e389242c88c80b08383342ea42fce8d0be740d3185c7c2cf740ee22903c2cd3b8c2afaf08747a33158b743adfc160af2970e5adb04c19b57fd70f9de90d
|
7
|
+
data.tar.gz: c53abe1659fccb8c2d31f12edb9088177ca294c07206c55cad5f5a83707b6564dd0b15db3847bc1fa6a7325ea44e31fc28758a12d3d3983f6f43e230c6274d2d
|
data/README.md
CHANGED
@@ -3,37 +3,32 @@
|
|
3
3
|
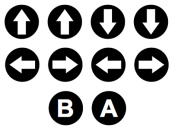
|
4
4
|
|
5
5
|
gem install konamio
|
6
|
-
|
6
|
+
|
7
7
|
and
|
8
8
|
|
9
9
|
require "konamio"
|
10
10
|
|
11
11
|
|
12
12
|
Default Usage:
|
13
|
-
```
|
14
|
-
> Konamio::Sequence::Requisition.new
|
13
|
+
```
|
14
|
+
> Konamio::Sequence::Requisition.new.execute!
|
15
15
|
Enter konami code (or hit escape)
|
16
16
|
Good job, you.
|
17
|
-
|
18
|
-
=>
|
19
|
-
|
20
|
-
@prompt="Enter konami code (or hit escape)",
|
21
|
-
@sequence="\e[A\e[A\e[B\e[B\e[D\e[C\e[D\e[CBA">
|
17
|
+
=> #<PayDirt::Result:0x937ddb4
|
18
|
+
@data={:data=>{:confirmation=>"Good job, you."}},
|
19
|
+
@success=true>
|
22
20
|
```
|
23
21
|
|
24
22
|
You can configure it to listen for any sequence you want:
|
25
|
-
```
|
23
|
+
```
|
26
24
|
> Konamio::Sequence::Requisition.new({
|
27
25
|
sequence: "a".upto("z").to_a.reverse,
|
28
26
|
prompt: "Say the alphabet backwards",
|
29
27
|
confirmation: "Okay, you can go"
|
30
|
-
})
|
28
|
+
}).execute!
|
31
29
|
Say the alphabet backwards
|
32
30
|
Okay, you can go
|
33
|
-
=> #<
|
34
|
-
|
35
|
-
|
36
|
-
@sequence=["z","y","x",...,"a"]>
|
31
|
+
=> #<PayDirt::Result:0x9265788
|
32
|
+
@data={:data=>{:confirmation=>"Okay, you can go"}},
|
33
|
+
@success=true>
|
37
34
|
```
|
38
|
-
|
39
|
-
Just something fun...
|
@@ -2,28 +2,42 @@ module Konamio
|
|
2
2
|
module Sequence
|
3
3
|
class Listener < PayDirt::Base
|
4
4
|
def initialize(options)
|
5
|
-
options = { input: $stdin }.merge(options)
|
5
|
+
options = { input: $stdin, debounce: 0.0001 }.merge(options)
|
6
6
|
load_options(:sequence, options)
|
7
7
|
end
|
8
8
|
|
9
9
|
def execute!
|
10
|
-
|
11
|
-
|
12
|
-
if result == true
|
10
|
+
case listen
|
11
|
+
when true
|
13
12
|
return PayDirt::Result.new(success: true, data: { sequence: @sequence[1..-1]})
|
14
|
-
|
15
|
-
return PayDirt::Result.new(success: false, data: {})
|
16
|
-
|
17
|
-
return PayDirt::Result.new(success: false, data: {
|
13
|
+
when false
|
14
|
+
return PayDirt::Result.new(success: false, data: { sequence: @sequence })
|
15
|
+
when :negative
|
16
|
+
return PayDirt::Result.new(success: false, data: { sequence: :negative })
|
17
|
+
else
|
18
|
+
return PayDirt::Result.new(success: false, data: { sequence: @sequence })
|
18
19
|
end
|
19
20
|
end
|
20
21
|
|
21
22
|
def listen
|
22
|
-
|
23
|
+
input = @input.getch
|
24
|
+
if(input == "\e")
|
25
|
+
extra_thread = Thread.new{
|
26
|
+
input << @input.getch
|
27
|
+
input << @input.getch
|
28
|
+
}
|
29
|
+
|
30
|
+
# wait just long enough for special keys to get swallowed
|
31
|
+
extra_thread.join(@debounce)
|
32
|
+
# kill thread so not-so-long special keys don't wait on getc
|
33
|
+
extra_thread.kill
|
34
|
+
end
|
35
|
+
|
36
|
+
case input
|
23
37
|
when @sequence[0]
|
24
38
|
true
|
25
39
|
when "\e"
|
26
|
-
|
40
|
+
:negative
|
27
41
|
else
|
28
42
|
false
|
29
43
|
end
|
@@ -5,42 +5,61 @@ module Konamio
|
|
5
5
|
options = {
|
6
6
|
speaker: Konamio::Prompt,
|
7
7
|
listener: Konamio::Sequence::Listener,
|
8
|
-
sequence: "\e[A\e[A\e[B\e[B\e[D\e[C\e[D\e[
|
8
|
+
sequence: ["\e[A","\e[A","\e[B","\e[B","\e[D","\e[C","\e[D","\e[C","B","A"],
|
9
9
|
prompt: "Enter konami code (or hit escape)",
|
10
10
|
confirmation: "Good job, you."
|
11
11
|
}.merge(options)
|
12
12
|
|
13
13
|
load_options(:sequence, options)
|
14
|
+
end
|
14
15
|
|
16
|
+
def execute!
|
15
17
|
prompt
|
16
|
-
listen(@sequence)
|
17
|
-
finish(false)
|
18
|
+
return listen(@sequence)
|
18
19
|
end
|
19
20
|
|
20
|
-
def prompt
|
21
|
-
@speaker.new(prompt:
|
21
|
+
def prompt(prompt = @prompt)
|
22
|
+
@speaker.new(prompt: prompt).execute!
|
22
23
|
end
|
23
24
|
|
24
25
|
def listen(sequence)
|
25
26
|
listener = @listener.new(sequence: sequence)
|
26
27
|
received = listener.execute!
|
28
|
+
signal = received.data[:sequence]
|
27
29
|
|
28
|
-
|
29
|
-
|
30
|
-
|
31
|
-
|
32
|
-
|
33
|
-
|
34
|
-
|
35
|
-
|
36
|
-
else
|
30
|
+
|
31
|
+
prompt(@confirmation) and return result(true, data: { confirmation: @confirmation }) if signal.empty?
|
32
|
+
|
33
|
+
case signal
|
34
|
+
when :negative
|
35
|
+
prompt("Goodbye!")
|
36
|
+
result(false, data: { confirmation: "User terminated." })
|
37
|
+
when sequence
|
37
38
|
prompt
|
38
39
|
listen(@sequence)
|
40
|
+
when sequence[1..-1]
|
41
|
+
listen received.data[:sequence]
|
42
|
+
else
|
43
|
+
result(false, data: { confirmation: "Unexpected termination" })
|
39
44
|
end
|
45
|
+
#if received.successful?
|
46
|
+
# if received.data[:sequence].empty?
|
47
|
+
# prompt(@confirmation)
|
48
|
+
# result(true, data: { confirmation: @confirmation })
|
49
|
+
# else
|
50
|
+
# listen(received.data[:sequence])
|
51
|
+
# end
|
52
|
+
#elsif received.data[:terminate]
|
53
|
+
# prompt("Goodbye!")
|
54
|
+
# result(false, data: { confirmation: :negative })
|
55
|
+
#else
|
56
|
+
# prompt
|
57
|
+
# listen(@sequence)
|
58
|
+
#end
|
40
59
|
end
|
41
60
|
|
42
|
-
def
|
43
|
-
|
61
|
+
def result(success, data={})
|
62
|
+
PayDirt::Result.new(success: success, data: data)
|
44
63
|
end
|
45
64
|
end
|
46
65
|
end
|
data/lib/konamio/version.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: konamio
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0
|
4
|
+
version: 0.1.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Tad Hosford
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2013-05-
|
11
|
+
date: 2013-05-04 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: pay_dirt
|