knifeswitch 1.1.0 → 1.1.1
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +3 -0
- data/lib/knifeswitch/circuit.rb +60 -31
- data/lib/knifeswitch/version.rb +1 -1
- metadata +3 -3
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA256:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 0a4ccbfd70b65a64a5dd7ca2b4415776a2edbf69ecff735d264410f52e872c9c
|
4
|
+
data.tar.gz: e05145c272a86defde56a34123049f1e05995c456291a8f3b08c9e8b46728890
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 333b47a38d2c6cfb4a71f9a2fd44a7502e9ca294d93310d31d9279fcce504136ec1b1a5fcbf734a4bb45e9bf4c05479e3687fc2f1b5684bd774f7cb3291ed651
|
7
|
+
data.tar.gz: fdfbfe1d54427f06991127547f6f1a700dd0caba0dffe7de0514e9970eed1a769cc594ac5d53b684f263f88a7b104db3a1460a9925b959c52ca59336c6434f29
|
data/README.md
CHANGED
@@ -1,4 +1,7 @@
|
|
1
1
|
# Knifeswitch
|
2
|
+
|
3
|
+
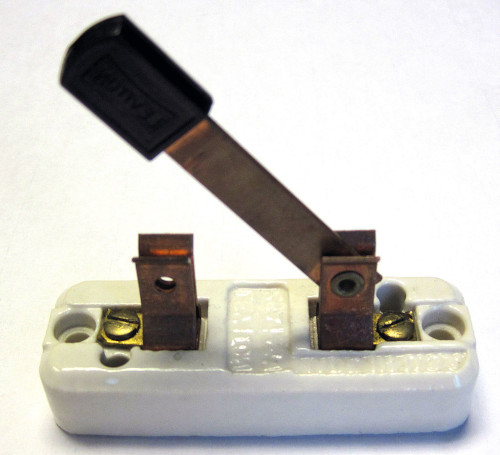
|
4
|
+
|
2
5
|
Yet another circuit breaker gem. This one strives to be as small and simple as possible. In the effort to remain simple, it currently only supports Rails with MySQL.
|
3
6
|
|
4
7
|
## Usage
|
data/lib/knifeswitch/circuit.rb
CHANGED
@@ -49,23 +49,38 @@ module Knifeswitch
|
|
49
49
|
#
|
50
50
|
# Raises Knifeswitch::CircuitOpen when called while the circuit is open.
|
51
51
|
def run
|
52
|
-
|
53
|
-
|
54
|
-
|
55
|
-
|
52
|
+
with_connection do
|
53
|
+
if open?
|
54
|
+
callback.try(:call, CircuitOpen.new)
|
55
|
+
raise CircuitOpen
|
56
|
+
end
|
57
|
+
|
58
|
+
begin
|
59
|
+
result = yield
|
60
|
+
rescue Exception => error
|
61
|
+
if exceptions.any? { |watched| error.is_a?(watched) }
|
62
|
+
increment_counter!
|
63
|
+
callback.try(:call, error)
|
64
|
+
else
|
65
|
+
reset_counter!
|
66
|
+
end
|
67
|
+
|
68
|
+
raise error
|
69
|
+
end
|
56
70
|
|
57
|
-
result = yield
|
58
|
-
reset_counter!
|
59
|
-
result
|
60
|
-
rescue Exception => error
|
61
|
-
if exceptions.any? { |watched| error.is_a?(watched) }
|
62
|
-
increment_counter!
|
63
|
-
callback.try(:call, error)
|
64
|
-
else
|
65
71
|
reset_counter!
|
72
|
+
result
|
66
73
|
end
|
74
|
+
ensure
|
75
|
+
reset_record
|
76
|
+
end
|
77
|
+
|
78
|
+
def closetime
|
79
|
+
record&.dig("closetime")
|
80
|
+
end
|
67
81
|
|
68
|
-
|
82
|
+
def counter
|
83
|
+
record&.dig("counter") || 0
|
69
84
|
end
|
70
85
|
|
71
86
|
# Queries the database to see if the circuit is open.
|
@@ -74,22 +89,7 @@ module Knifeswitch
|
|
74
89
|
# When the circuit is open, calls to `run` will raise CircuitOpen
|
75
90
|
# instead of yielding.
|
76
91
|
def open?
|
77
|
-
|
78
|
-
SELECT COUNT(*) c FROM knifeswitch_counters
|
79
|
-
WHERE name = ? AND closetime > ?
|
80
|
-
), namespace, DateTime.now)
|
81
|
-
|
82
|
-
result > 0
|
83
|
-
end
|
84
|
-
|
85
|
-
# Retrieves the current counter value.
|
86
|
-
def counter
|
87
|
-
result = sql(:select_value, %(
|
88
|
-
SELECT counter FROM knifeswitch_counters
|
89
|
-
WHERE name = ?
|
90
|
-
), namespace)
|
91
|
-
|
92
|
-
result || 0
|
92
|
+
return closetime && closetime > DateTime.now
|
93
93
|
end
|
94
94
|
|
95
95
|
# Increments counter and opens the circuit if it went
|
@@ -117,6 +117,7 @@ module Knifeswitch
|
|
117
117
|
|
118
118
|
# Sets the counter to zero
|
119
119
|
def reset_counter!
|
120
|
+
return if counter == 0
|
120
121
|
sql(:execute, %(
|
121
122
|
INSERT INTO knifeswitch_counters (name,counter)
|
122
123
|
VALUES (?, 0)
|
@@ -126,14 +127,42 @@ module Knifeswitch
|
|
126
127
|
|
127
128
|
private
|
128
129
|
|
130
|
+
def load_record
|
131
|
+
sql(:select_one, %(
|
132
|
+
SELECT counter, closetime FROM knifeswitch_counters
|
133
|
+
WHERE name = ?
|
134
|
+
), @namespace)
|
135
|
+
end
|
136
|
+
|
137
|
+
def reset_record
|
138
|
+
@record = nil
|
139
|
+
end
|
140
|
+
|
141
|
+
def record
|
142
|
+
@record ||= load_record
|
143
|
+
end
|
144
|
+
|
129
145
|
# Executes a SQL query with the given Connection method
|
130
146
|
# (i.e. :execute, or :select_values)
|
131
147
|
def sql(method, query, *args)
|
132
148
|
query = ActiveRecord::Base.send(:sanitize_sql_array, [query] + args)
|
133
|
-
|
134
|
-
ActiveRecord::Base.connection_pool.with_connection do |conn|
|
149
|
+
with_connection do |conn|
|
135
150
|
conn.send(method, query)
|
136
151
|
end
|
137
152
|
end
|
153
|
+
|
154
|
+
def with_connection
|
155
|
+
if @conn
|
156
|
+
yield(@conn)
|
157
|
+
else
|
158
|
+
begin
|
159
|
+
@conn = ActiveRecord::Base.connection_pool.checkout
|
160
|
+
yield(@conn)
|
161
|
+
ensure
|
162
|
+
ActiveRecord::Base.connection_pool.checkin(@conn)
|
163
|
+
@conn = nil
|
164
|
+
end
|
165
|
+
end
|
166
|
+
end
|
138
167
|
end
|
139
168
|
end
|
data/lib/knifeswitch/version.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: knifeswitch
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 1.1.
|
4
|
+
version: 1.1.1
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Nigel Baillie
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2019-
|
11
|
+
date: 2019-10-28 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: rails
|
@@ -80,7 +80,7 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
80
80
|
- !ruby/object:Gem::Version
|
81
81
|
version: '0'
|
82
82
|
requirements: []
|
83
|
-
rubygems_version: 3.0.
|
83
|
+
rubygems_version: 3.0.6
|
84
84
|
signing_key:
|
85
85
|
specification_version: 4
|
86
86
|
summary: Simple implementation of the circuit breaker pattern.
|