journey_planner 0.0.7 → 0.0.8
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +109 -2
- data/lib/journey.rb +9 -3
- data/lib/journey_planner/version.rb +1 -1
- data/lib/time_helpers.rb +11 -0
- data/screenshots/jp_gmaps_ex.jpg +0 -0
- data/spec/journey_spec.rb +2 -6
- metadata +4 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: aa804b245593378c53df6576d5b6b4b8c157ae2b
|
4
|
+
data.tar.gz: 5f1df6f9c52b9abbb26221c2b86b8e2ef97b7a6d
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 4464c10376d4848348d8316640b1faa563b579aeca9c14a8559142203e0a308fb2174add2efc8191ba78070366df157d219c712c7fc71faa7f7cb310122be618
|
7
|
+
data.tar.gz: 95208206d05bb3bb6561057201943dd25420c772e068dbdeac1f44b278d0158d6b5bfc46d4935fb1ef66a0e2857d728ededc517180c4faa7dba1d0c8bb3eee95
|
data/README.md
CHANGED
@@ -34,14 +34,121 @@ journeys = client.get_journeys from: "old street underground station", to: "oxfo
|
|
34
34
|
|
35
35
|
```
|
36
36
|
|
37
|
-
Methods you can play with:
|
37
|
+
### Methods you can play with:
|
38
|
+
|
39
|
+
The `instructions` method returns a hash of instructions, with the keys as departure and arrival times, and the values as arrays of verbal instructions.
|
38
40
|
|
39
41
|
```ruby
|
40
42
|
journeys.first.instructions
|
41
|
-
# =>
|
43
|
+
# {"Sep 5 2014 16:21 - Sep 5 2014 16:27"=>["Northern line to Euston / Northern line towards Edgware, Mill Hill East, or High Barnet"],
|
44
|
+
# "Sep 5 2014 16:32 - Sep 5 2014 16:35"=>["Victoria line to Oxford Circus / Victoria line towards Brixton"]}
|
45
|
+
```
|
46
|
+
|
47
|
+
### Integrating with Google Maps
|
48
|
+
|
49
|
+
Setting up a simple Sinatra app:
|
50
|
+
|
51
|
+
```ruby
|
52
|
+
|
53
|
+
require 'sinatra'
|
54
|
+
require 'sinatra/json'
|
55
|
+
require 'journey_planner'
|
56
|
+
|
57
|
+
get '/' do
|
58
|
+
erb :index
|
59
|
+
end
|
60
|
+
|
61
|
+
get '/maps' do
|
62
|
+
client = TFLJourneyPlanner::Client.new(app_id: ENV["TFL_ID"], app_key: ENV["TFL_KEY"])
|
63
|
+
journeys = client.get_journeys from: "old street underground station", to: "oxford circus underground station"
|
64
|
+
json journeys.first.map_path
|
65
|
+
end
|
66
|
+
|
67
|
+
```
|
68
|
+
|
69
|
+
Your HTML and Javascript (assuming JQuery, the Google Maps API, and GMapsJS have already been linked)
|
70
|
+
|
71
|
+
```html
|
72
|
+
<div id="map" style="height: 500px; width: 500px"></div>
|
73
|
+
```
|
74
|
+
|
75
|
+
```javascript
|
76
|
+
$(document).ready(function(){
|
77
|
+
|
78
|
+
$.get('/maps', function(coordinates){
|
79
|
+
|
80
|
+
var map = new GMaps({
|
81
|
+
div: '#map',
|
82
|
+
lat: coordinates[0][0],
|
83
|
+
lng: coordinates[0][1]
|
84
|
+
|
85
|
+
});
|
86
|
+
|
87
|
+
map.drawPolyline({
|
88
|
+
path: coordinates,
|
89
|
+
strokeColor: '#131540',
|
90
|
+
strokeOpacity: 0.6,
|
91
|
+
strokeWeight: 6
|
92
|
+
});
|
93
|
+
})
|
94
|
+
|
95
|
+
})
|
42
96
|
```
|
43
97
|
|
98
|
+
On launching your app, you should find that GMaps has created a polyline from the TFL Journey Planner path coordinates.
|
99
|
+
|
100
|
+
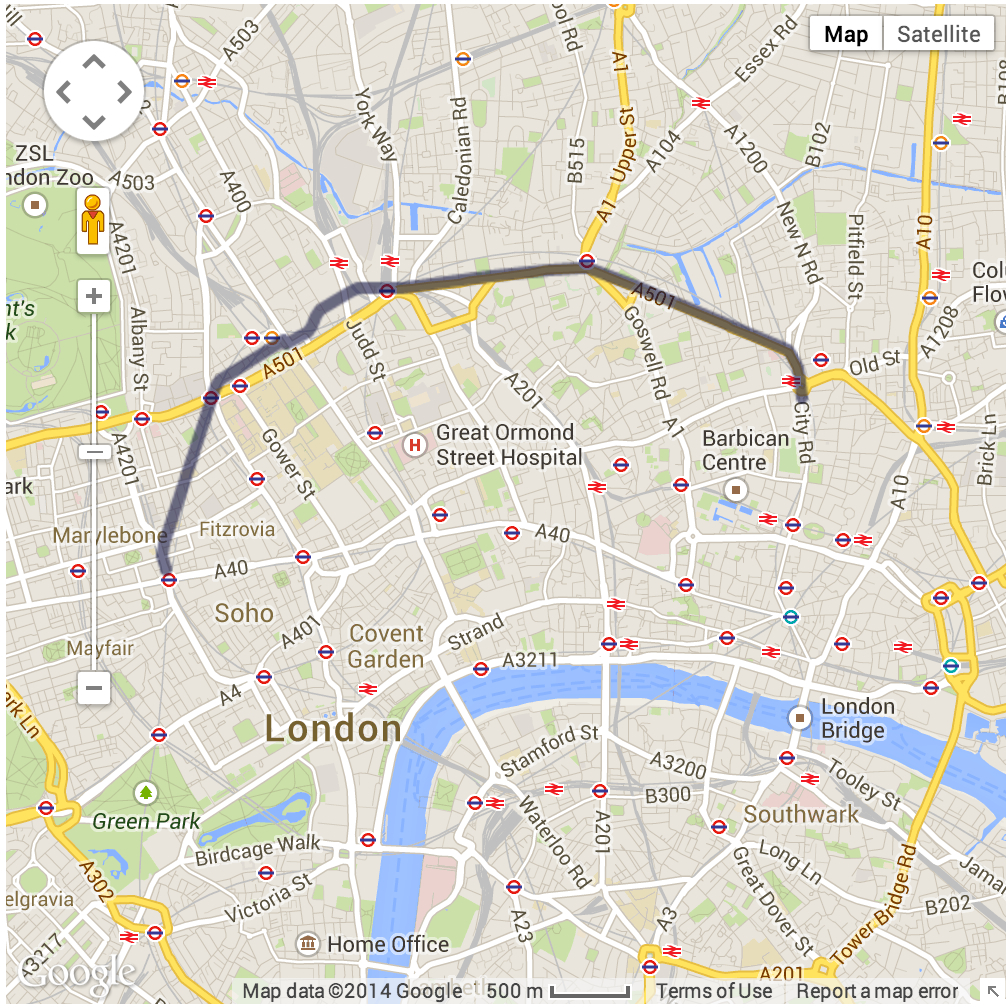
|
101
|
+
|
102
|
+
###Search Options
|
103
|
+
|
104
|
+
```ruby
|
105
|
+
client.get_journeys
|
106
|
+
|
107
|
+
from: #=> Origin of the journey (if in coordinate format then must be "longitude,latitude")
|
108
|
+
|
109
|
+
to: #=> Destination of the journey (if in coordinate format then must be "longitude,latitude")
|
110
|
+
|
111
|
+
via: #=> Travel through (if in coordinate format then must be "longitude,latidude")
|
112
|
+
|
113
|
+
national_search: #=> Does the journey cover stops outside London? eg. "nationalSearch=true". Set to false by default
|
114
|
+
|
115
|
+
date: #=> The date must be in yyyyMMdd format
|
116
|
+
|
117
|
+
time: #=> The time must be in HHmm format
|
118
|
+
|
119
|
+
time_is: #=> Does the time given relate to arrival or leaving time? Possible options: "departing" | "arriving". Set to Departing by default
|
120
|
+
|
121
|
+
journey_preference: #=> The journey preference eg possible options: "leastinterchange" | "leasttime" | "leastwalking"
|
122
|
+
|
123
|
+
mode: #=> The mode must be a comma separated list of modes. eg possible options: "public-bus,overground,train,tube,coach,dlr,cablecar,tram,river,walking,cycle"
|
124
|
+
|
125
|
+
accessibility_preference: #=> The accessibility preference must be a comma separated list eg. "noSolidStairs,noEscalators,noElevators,stepFreeToVehicle,stepFreeToPlatform"
|
126
|
+
|
127
|
+
from_name: #=> From name is the location name associated with a from coordinate
|
44
128
|
|
129
|
+
to_name: #=> To name is the label location associated with a to coordinate
|
130
|
+
|
131
|
+
via_name: #=> Via name is the location name associated with a via coordinate
|
132
|
+
|
133
|
+
max_transfer_minutes: #=> The max walking time in minutes for transfer eg. "120"
|
134
|
+
|
135
|
+
min_transfer_minutes: #=> The max walking time in minutes for journeys eg. "120"
|
136
|
+
|
137
|
+
walking_speed: #=> The walking speed. eg possible options: "slow" | "average" | "fast"
|
138
|
+
|
139
|
+
cycle_preference: #=> The cycle preference. eg possible options: "allTheWay" | "leaveAtStation" | "takeOnTransport" | "cycleHire"
|
140
|
+
|
141
|
+
adjustment: #=> Time adjustment command. eg possible options: "TripFirst" | "TripLast"
|
142
|
+
|
143
|
+
bike_proficiency: #=> A comma separated list of cycling proficiency levels. eg possible options: "easy,moderate,fast"
|
144
|
+
|
145
|
+
alternative_cycle: #=> Set to True to generate an additional journey consisting of cycling only, if possible. Default value is false. eg. alternative_cycle: true
|
146
|
+
|
147
|
+
alternative_walking: #=> Set to true to generate an additional journey consisting of walking only, if possible. Default value is false. eg. alternative_walking: true
|
148
|
+
|
149
|
+
apply_html_markup: #=> Flag to determine whether certain text (e.g. walking instructions) should be output with HTML tags or not.
|
150
|
+
|
151
|
+
```
|
45
152
|
|
46
153
|
## Contributing
|
47
154
|
|
data/lib/journey.rb
CHANGED
@@ -1,16 +1,22 @@
|
|
1
|
+
require_relative 'time_helpers'
|
2
|
+
|
1
3
|
module TFLJourneyPlanner
|
2
4
|
|
3
5
|
class Journey < RecursiveOpenStruct
|
6
|
+
|
7
|
+
include TimeHelpers
|
8
|
+
|
9
|
+
|
4
10
|
def instructions
|
5
11
|
array = []
|
6
12
|
legs.each do |leg|
|
7
13
|
if leg.instruction.steps.any?
|
8
|
-
leg.instruction.steps.each {|step| array << step.description}
|
14
|
+
leg.instruction.steps.each {|step| array << [ "#{prettify leg.departure_time} - #{prettify leg.arrival_time}", step.description]}
|
9
15
|
else
|
10
|
-
array << leg.instruction.summary + " / " + leg.instruction.detailed
|
16
|
+
array << ["#{prettify leg.departure_time} - #{prettify leg.arrival_time}", leg.instruction.summary + " / " + leg.instruction.detailed]
|
11
17
|
end
|
12
18
|
end
|
13
|
-
|
19
|
+
array.inject(Hash.new{ |h,k| h[k]=[] }){ |h,(k,v)| h[k] << v; h }
|
14
20
|
end
|
15
21
|
|
16
22
|
def map_path
|
data/lib/time_helpers.rb
ADDED
Binary file
|
data/spec/journey_spec.rb
CHANGED
@@ -7,12 +7,8 @@ describe TFLJourneyPlanner::Journey do
|
|
7
7
|
|
8
8
|
it "should return an array of instructions" do
|
9
9
|
VCR.use_cassette "hello", record: :none do
|
10
|
-
|
11
|
-
|
12
|
-
"H25 bus to Bedfont Library / H25 bus towards Hatton Cross",
|
13
|
-
"Continue along Staines Road for 64 metres (0 minutes, 57 seconds).",
|
14
|
-
"Turn left on to Grovestile Waye, continue for 95 metres (1 minute, 21 seconds)."]
|
15
|
-
expect(journeys[0].instructions).to eq array
|
10
|
+
hash = {"Sep 3 2014 16:58 - Sep 3 2014 17:03"=>["Continue along Fruen Road for 143 metres (2 minutes, 8 seconds).", "Turn right on to Bedfont Lane, continue for 172 metres (2 minutes, 33 seconds)."], "Sep 3 2014 17:03 - Sep 3 2014 17:06"=>["H25 bus to Bedfont Library / H25 bus towards Hatton Cross"], "Sep 3 2014 17:06 - Sep 3 2014 17:09"=>["Continue along Staines Road for 64 metres (0 minutes, 57 seconds).", "Turn left on to Grovestile Waye, continue for 95 metres (1 minute, 21 seconds)."]}
|
11
|
+
expect(journeys[0].instructions).to eq hash
|
16
12
|
end
|
17
13
|
end
|
18
14
|
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: journey_planner
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0.
|
4
|
+
version: 0.0.8
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- jpatel531
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2014-09-
|
11
|
+
date: 2014-09-05 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: bundler
|
@@ -56,6 +56,8 @@ files:
|
|
56
56
|
- lib/journey_planner/version.rb
|
57
57
|
- lib/results.rb
|
58
58
|
- lib/rubify_keys.rb
|
59
|
+
- lib/time_helpers.rb
|
60
|
+
- screenshots/jp_gmaps_ex.jpg
|
59
61
|
- spec/client_spec.rb
|
60
62
|
- spec/fixtures/tfl_jp_casettes/hello.yml
|
61
63
|
- spec/journey_spec.rb
|