jekyll-components 0.0.1 → 0.1.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +23 -2
- data/docs/creating-a-component.md +164 -0
- data/docs/introduction.md +45 -0
- data/examples/block_component.rb +22 -0
- data/examples/tag_component.rb +21 -0
- data/jekyll-components.gemspec +1 -1
- data/lib/jekyll/components/base.rb +7 -18
- data/lib/jekyll/components/version.rb +1 -1
- metadata +9 -5
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 4fafd5492ef563c250f16ad6c4c0e6aaef7c70eb
|
4
|
+
data.tar.gz: c88265b0c8a796f546c2a46c8fd76d206f7a6e14
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 7aca41a1e291457646bc52dde8017f81d7f3c60ba8bc4cd3b565b9cbd81807315e4e8f58d9918393716926ea637453c4c14d25afe1e0b05b4646cb7431875390
|
7
|
+
data.tar.gz: 9c521b6beb4993f0d0b89a16bfd17ea4aba4b4123e48906f5311a10b04fcf88ca6b45011278d0244bb91519bbfb55c008d4e43548b06a79b10869495e1111afe
|
data/README.md
CHANGED
@@ -1,8 +1,18 @@
|
|
1
|
-
# jekyll-components 🌟
|
1
|
+
# jekyll-components 🌟 [](https://travis-ci.org/helpscout/jekyll-components) [](https://badge.fury.io/rb/jekyll-components)
|
2
|
+
|
3
|
+
## DEPRECATED: Please use [jekyll-spark](https://github.com/helpscout/jekyll-spark) instead!!!
|
2
4
|
|
3
5
|
A Jekyll library for building component-based UI
|
4
6
|
|
5
|
-
|
7
|
+
This library was heavily inspired by view/component creation from modern Javascript libraries like [React](https://facebook.github.io/react/) and [Vue](https://vuejs.org/).
|
8
|
+
|
9
|
+
**Table of Contents**
|
10
|
+
|
11
|
+
- [Install](#install)
|
12
|
+
- [Documentation](#documenation)
|
13
|
+
- [Examples](#examples)
|
14
|
+
|
15
|
+
## Install
|
6
16
|
|
7
17
|
Add this line to your application's Gemfile:
|
8
18
|
|
@@ -19,3 +29,14 @@ Or install it yourself as:
|
|
19
29
|
```
|
20
30
|
gem install jekyll-components
|
21
31
|
```
|
32
|
+
|
33
|
+
|
34
|
+
|
35
|
+
## Documentation
|
36
|
+
|
37
|
+
**[View the docs](https://github.com/helpscout/jekyll-components/blob/master/docs/introduction.md)** to get started with Jekyll Components!
|
38
|
+
|
39
|
+
|
40
|
+
## Examples
|
41
|
+
|
42
|
+
**[View the starter](https://github.com/helpscout/jekyll-components/tree/master/examples)** Component view files.
|
@@ -0,0 +1,164 @@
|
|
1
|
+
# Creating a component
|
2
|
+
|
3
|
+
Let's create our first component under `_plugins/tags`.
|
4
|
+
|
5
|
+
We'll call it `napolean.rb`! Below is the [starting template](https://github.com/helpscout/jekyll-components/tree/master/examples) of any Jekyll Component:
|
6
|
+
|
7
|
+
```ruby
|
8
|
+
require "jekyll-components"
|
9
|
+
|
10
|
+
module Jekyll
|
11
|
+
# Create your component class
|
12
|
+
class NapoleanComponent < ComponentTag
|
13
|
+
def template(context)
|
14
|
+
render = %Q[
|
15
|
+
# Put markup here!
|
16
|
+
]
|
17
|
+
end
|
18
|
+
end
|
19
|
+
end
|
20
|
+
|
21
|
+
# Register your component with Liquid
|
22
|
+
Liquid::Template.register_tag(
|
23
|
+
"Napolean", # Namespace your component
|
24
|
+
Jekyll::NapoleanComponent, # Pass your newly created component class
|
25
|
+
)
|
26
|
+
```
|
27
|
+
|
28
|
+
### Rendering markup
|
29
|
+
|
30
|
+
Let's make this component render this GIF:
|
31
|
+
|
32
|
+
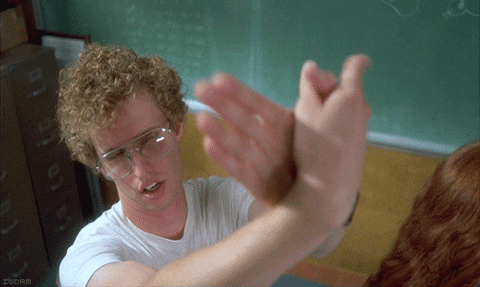
|
33
|
+
|
34
|
+
|
35
|
+
```ruby
|
36
|
+
require "jekyll-components"
|
37
|
+
|
38
|
+
module Jekyll
|
39
|
+
class NapoleanComponent < ComponentTag
|
40
|
+
def template(context)
|
41
|
+
render = %Q[
|
42
|
+
<div class="napolean">
|
43
|
+
<img src="https://media.giphy.com/media/9QbDWTcnq4wmc/giphy.gif">
|
44
|
+
</div>
|
45
|
+
]
|
46
|
+
end
|
47
|
+
end
|
48
|
+
end
|
49
|
+
|
50
|
+
Liquid::Template.register_tag(
|
51
|
+
"Napolean",
|
52
|
+
Jekyll::NapoleanComponent,
|
53
|
+
)
|
54
|
+
```
|
55
|
+
|
56
|
+
To use our tag component, all we have to do is use `{% Napolean %}` in our Jekyll `.html` or `.md` file:
|
57
|
+
|
58
|
+
```html
|
59
|
+
Check out this component!
|
60
|
+
{% Napolean %}
|
61
|
+
```
|
62
|
+
|
63
|
+
That's it!
|
64
|
+
|
65
|
+
|
66
|
+
### Adding props
|
67
|
+
|
68
|
+
"Props" are data that we can pass to our component.
|
69
|
+
|
70
|
+
For our example, let's provide the ability to adjust the width of our image as well as provide a caption:
|
71
|
+
|
72
|
+
```html
|
73
|
+
{% Napolean
|
74
|
+
width: "300"
|
75
|
+
caption: "Way to eat all the friggen chips Kip!"
|
76
|
+
%}
|
77
|
+
```
|
78
|
+
|
79
|
+
You can totally write everything in a single line (below). However, we recommend the above approach as it makes it much easier to add/remove/edit props.
|
80
|
+
|
81
|
+
```html
|
82
|
+
{% Napolean width: "300" caption: "Way to eat all the friggen chips Kip!" %}
|
83
|
+
```
|
84
|
+
|
85
|
+
In order to use our new `width` and `caption` props, we have to update our `template` method in our Napolean component. Prop data being passed to our component will be available in a `@prop` instance variable in our `.rb` file:
|
86
|
+
|
87
|
+
```ruby
|
88
|
+
require "jekyll-components"
|
89
|
+
|
90
|
+
module Jekyll
|
91
|
+
class NapoleanComponent < ComponentTag
|
92
|
+
def template(context)
|
93
|
+
# Declare props as variables
|
94
|
+
# Not necessary, but highly recommended
|
95
|
+
caption = @props["caption"]
|
96
|
+
width = @props["width"]
|
97
|
+
|
98
|
+
render = %Q[
|
99
|
+
<div class="napolean">
|
100
|
+
<img src="https://media.giphy.com/media/9QbDWTcnq4wmc/giphy.gif" width="#{width}">
|
101
|
+
#{caption}
|
102
|
+
</div>
|
103
|
+
]
|
104
|
+
end
|
105
|
+
end
|
106
|
+
end
|
107
|
+
|
108
|
+
Liquid::Template.register_tag(
|
109
|
+
"Napolean",
|
110
|
+
Jekyll::NapoleanComponent,
|
111
|
+
)
|
112
|
+
```
|
113
|
+
|
114
|
+
The resulting compiled markup will be:
|
115
|
+
|
116
|
+
```html
|
117
|
+
<div class="napolean">
|
118
|
+
<img src="https://media.giphy.com/media/9QbDWTcnq4wmc/giphy.gif" width="300">
|
119
|
+
Way to eat all the friggen chips Kip!
|
120
|
+
</div>
|
121
|
+
```
|
122
|
+
|
123
|
+
|
124
|
+
### Syntax
|
125
|
+
|
126
|
+
Below is a comparison between some syntax differences between Jekyll Components (Ruby) and React (ES6 Javascript):
|
127
|
+
|
128
|
+
| Ruby | Javascript (React) | Description |
|
129
|
+
| --- | --- | --- |
|
130
|
+
| `@props["key"]` | `this.props.key` | Component prop data. |
|
131
|
+
| `render =` | `render() { return ... } ` | Outputting the component's markup. |
|
132
|
+
| `%Q[ ... ]` | `` `...` `` | String interpolation wrapper. |
|
133
|
+
| `#{var}` | `${var} ` | String interpolated variable. |
|
134
|
+
|
135
|
+
|
136
|
+
|
137
|
+
## Creating a block component
|
138
|
+
|
139
|
+
Let's say we want to update our component to be a block instead. It'll make it more intuitive to add caption. Maybe something like:
|
140
|
+
|
141
|
+
```html
|
142
|
+
{% Napolean width: "300" %}
|
143
|
+
This tastes like the cow got into an onion patch.
|
144
|
+
{% endNapolean %}
|
145
|
+
```
|
146
|
+
|
147
|
+
It's pretty easy! The only thing we need to change in our `napolean.rb` file is the Class our component inherits from.
|
148
|
+
|
149
|
+
Before:
|
150
|
+
```ruby
|
151
|
+
class NapoleanComponent < ComponentTag
|
152
|
+
```
|
153
|
+
|
154
|
+
After:
|
155
|
+
```ruby
|
156
|
+
class NapoleanComponent < ComponentBlock
|
157
|
+
```
|
158
|
+
|
159
|
+
|
160
|
+
### That's it!
|
161
|
+
|
162
|
+
You've got the basics to create some awesome components in Jekyll.
|
163
|
+
|
164
|
+
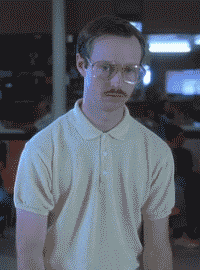
|
@@ -0,0 +1,45 @@
|
|
1
|
+
# Introduction
|
2
|
+
|
3
|
+
Components are `.rb` files that are added to your Jekyll project's default `_plugins/` directory:
|
4
|
+
|
5
|
+
```shell
|
6
|
+
my-jekyll/
|
7
|
+
└── _plugins/
|
8
|
+
└── *Add components here*
|
9
|
+
```
|
10
|
+
|
11
|
+
There are two types are Components:
|
12
|
+
|
13
|
+
**Tags**
|
14
|
+
|
15
|
+
These components are created using Liquid [Tags](http://www.rubydoc.info/github/Shopify/liquid/Liquid/Tag), and they do not contain content when used.
|
16
|
+
|
17
|
+
Example:
|
18
|
+
```html
|
19
|
+
{% Napolean id: "skillz" class: "nunchucks bow staff computer-hacking" %}
|
20
|
+
```
|
21
|
+
|
22
|
+
**Blocks**
|
23
|
+
|
24
|
+
These components are created using Liquid [Blocks](http://www.rubydoc.info/github/Shopify/liquid/Liquid/Block), and they **do** contain content when used.
|
25
|
+
|
26
|
+
Example:
|
27
|
+
```html
|
28
|
+
{% Napolean class: "chapstick" %}
|
29
|
+
But my lips hurt real bad!
|
30
|
+
{% endNapolean %}
|
31
|
+
```
|
32
|
+
|
33
|
+
Because of these types, we recommend you organize your components in your `_plugins/` directory into `tags` and `blocks` directories:
|
34
|
+
|
35
|
+
```shell
|
36
|
+
my-jekyll/
|
37
|
+
└── _plugins/
|
38
|
+
├── blocks/
|
39
|
+
└── tags/
|
40
|
+
```
|
41
|
+
|
42
|
+
|
43
|
+
### Up next
|
44
|
+
|
45
|
+
Learn how to [create a component](creating-a-component.md).
|
@@ -0,0 +1,22 @@
|
|
1
|
+
require "jekyll-components"
|
2
|
+
|
3
|
+
module Jekyll
|
4
|
+
class ExampleBlockComponent < ComponentBlock
|
5
|
+
def template(context)
|
6
|
+
# Declare props as variables here
|
7
|
+
content = @props["content"]
|
8
|
+
|
9
|
+
# Output rendered markup
|
10
|
+
render = %Q[
|
11
|
+
<div class="component">
|
12
|
+
#{content}
|
13
|
+
</div>
|
14
|
+
]
|
15
|
+
end
|
16
|
+
end
|
17
|
+
end
|
18
|
+
|
19
|
+
Liquid::Template.register_tag(
|
20
|
+
"ExampleBlock",
|
21
|
+
Jekyll::ExampleBlockComponent,
|
22
|
+
)
|
@@ -0,0 +1,21 @@
|
|
1
|
+
require "jekyll-components"
|
2
|
+
|
3
|
+
module Jekyll
|
4
|
+
class ExampleTagComponent < ComponentTag
|
5
|
+
def template(context)
|
6
|
+
# Declare props as variables here
|
7
|
+
|
8
|
+
# Output rendered markup
|
9
|
+
render = %Q[
|
10
|
+
<div class="component">
|
11
|
+
Example
|
12
|
+
</div>
|
13
|
+
]
|
14
|
+
end
|
15
|
+
end
|
16
|
+
end
|
17
|
+
|
18
|
+
Liquid::Template.register_tag(
|
19
|
+
"ExampleTag",
|
20
|
+
Jekyll::ExampleTagComponent,
|
21
|
+
)
|
data/jekyll-components.gemspec
CHANGED
@@ -28,7 +28,7 @@ Gem::Specification.new do |spec|
|
|
28
28
|
spec.add_development_dependency "minitest-reporters"
|
29
29
|
spec.add_development_dependency "minitest-profile"
|
30
30
|
spec.add_development_dependency "minitest", "~> 5.8"
|
31
|
-
spec.add_development_dependency "nokogiri", "~> 1.
|
31
|
+
spec.add_development_dependency "nokogiri", "~> 1.8.2"
|
32
32
|
spec.add_development_dependency "rspec-mocks"
|
33
33
|
spec.add_development_dependency "shoulda"
|
34
34
|
spec.add_development_dependency "kramdown"
|
@@ -47,7 +47,7 @@ module Jekyll
|
|
47
47
|
end
|
48
48
|
}
|
49
49
|
|
50
|
-
template
|
50
|
+
return template
|
51
51
|
end
|
52
52
|
|
53
53
|
def selector_data_props(attr = @attributes)
|
@@ -58,7 +58,7 @@ module Jekyll
|
|
58
58
|
end
|
59
59
|
}
|
60
60
|
|
61
|
-
template
|
61
|
+
return template
|
62
62
|
end
|
63
63
|
|
64
64
|
def selector_props(attr = @default_selector_attr)
|
@@ -66,7 +66,7 @@ module Jekyll
|
|
66
66
|
template += selector_data_props
|
67
67
|
template += selector_default_props(attr)
|
68
68
|
|
69
|
-
template
|
69
|
+
return template
|
70
70
|
end
|
71
71
|
|
72
72
|
def set_props(props = Hash.new)
|
@@ -98,15 +98,6 @@ module Jekyll
|
|
98
98
|
return content
|
99
99
|
end
|
100
100
|
|
101
|
-
def markdown_file?()
|
102
|
-
file = @context["page"]["name"]
|
103
|
-
if file
|
104
|
-
file.include?(".md") || file.include?(".markdown")
|
105
|
-
else
|
106
|
-
false
|
107
|
-
end
|
108
|
-
end
|
109
|
-
|
110
101
|
def render(context)
|
111
102
|
@context = context
|
112
103
|
@site = @context.registers[:site]
|
@@ -114,20 +105,18 @@ module Jekyll
|
|
114
105
|
serialize_data
|
115
106
|
output = template(context)
|
116
107
|
|
117
|
-
# if (markdown_file?)
|
118
|
-
# output = "{::nomarkdown type='html'}#{output}{:/nomarkdown}"
|
119
|
-
# end
|
120
|
-
|
121
108
|
if (output.instance_of?(String))
|
122
109
|
output = Liquid::Template.parse(output).render()
|
123
110
|
output = @@compressor.compress(unindent(output))
|
124
111
|
else
|
125
|
-
output
|
112
|
+
output = ""
|
126
113
|
end
|
114
|
+
|
115
|
+
return output
|
127
116
|
end
|
128
117
|
|
129
118
|
def template(context = @context)
|
130
|
-
context
|
119
|
+
return context
|
131
120
|
end
|
132
121
|
|
133
122
|
end
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: jekyll-components
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.0
|
4
|
+
version: 0.1.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- ItsJonQ
|
8
8
|
autorequire:
|
9
9
|
bindir: exe
|
10
10
|
cert_chain: []
|
11
|
-
date:
|
11
|
+
date: 2018-04-14 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: jekyll
|
@@ -114,14 +114,14 @@ dependencies:
|
|
114
114
|
requirements:
|
115
115
|
- - "~>"
|
116
116
|
- !ruby/object:Gem::Version
|
117
|
-
version: 1.
|
117
|
+
version: 1.8.2
|
118
118
|
type: :development
|
119
119
|
prerelease: false
|
120
120
|
version_requirements: !ruby/object:Gem::Requirement
|
121
121
|
requirements:
|
122
122
|
- - "~>"
|
123
123
|
- !ruby/object:Gem::Version
|
124
|
-
version: 1.
|
124
|
+
version: 1.8.2
|
125
125
|
- !ruby/object:Gem::Dependency
|
126
126
|
name: rspec-mocks
|
127
127
|
requirement: !ruby/object:Gem::Requirement
|
@@ -177,6 +177,10 @@ files:
|
|
177
177
|
- LICENSE.txt
|
178
178
|
- README.md
|
179
179
|
- Rakefile
|
180
|
+
- docs/creating-a-component.md
|
181
|
+
- docs/introduction.md
|
182
|
+
- examples/block_component.rb
|
183
|
+
- examples/tag_component.rb
|
180
184
|
- jekyll-components.gemspec
|
181
185
|
- lib/jekyll-components.rb
|
182
186
|
- lib/jekyll/components.rb
|
@@ -204,7 +208,7 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
204
208
|
version: '0'
|
205
209
|
requirements: []
|
206
210
|
rubyforge_project:
|
207
|
-
rubygems_version: 2.5.
|
211
|
+
rubygems_version: 2.4.5.1
|
208
212
|
signing_key:
|
209
213
|
specification_version: 4
|
210
214
|
summary: A Jekyll library for building component-based UI
|