htk 0.0.1
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/.editorconfig +12 -0
- data/.gitignore +1 -0
- data/LICENSE.md +21 -0
- data/README.md +63 -0
- data/lib/apis.rb +31 -0
- metadata +78 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: a7dd939114342957941851759641e01558dcbcd9
|
4
|
+
data.tar.gz: 54b563d7b32b3b1bc9a3be0b35ee8e719164dd53
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 33df9f1d19af442a79ff2bb83a3b05520cad29d0ca5013f91840a41d695c9af1ffd935a996e6e4cac44b70ca39aec3d6ff944bfa06cd3d086668560380e30478
|
7
|
+
data.tar.gz: b171bc46628cf7208f31a41587ff7bdd3801737f411bbffe6eb1ded6a6eee5b1fd0d81cb7b28951f47a90b6075e1b95cfd227caf4b9eaf256ee3b95243563818
|
data/.editorconfig
ADDED
@@ -0,0 +1,12 @@
|
|
1
|
+
# See http://EditorConfig.org for details
|
2
|
+
|
3
|
+
# Top-most EditorConfig file
|
4
|
+
root = true
|
5
|
+
|
6
|
+
# Unix-style newlines with a newline ending every file
|
7
|
+
# Two space indent by default
|
8
|
+
[*]
|
9
|
+
end_of_line = lf
|
10
|
+
insert_final_newline = true
|
11
|
+
indent_style = space
|
12
|
+
indent_size = 2
|
data/.gitignore
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
local_settings.rb
|
data/LICENSE.md
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
MIT License
|
2
|
+
|
3
|
+
Copyright (c) 2019 Hacktoolkit
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in all
|
13
|
+
copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
21
|
+
SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,63 @@
|
|
1
|
+
# HTK for Ruby
|
2
|
+
|
3
|
+
A set of convenience utils for Ruby. A loosely inspired, not-quite-close-to-feature parity port of [`pyhtk-lite`](https://github.com/hacktoolkit/pyhtk-lite).
|
4
|
+
|
5
|
+
# Features
|
6
|
+
|
7
|
+
1. Debug via Slack using `::Htk::Utils.slack_debug('some debugging message')`. The best of `println` debugging, without the inconvenience of visually fishing for one message out of thousands of log lines.
|
8
|
+
|
9
|
+
# How to Use This Awesome?
|
10
|
+
|
11
|
+
1. Clone this repository into a directory named `htk` (preferred) or `htk_lite`
|
12
|
+
SSH: `git clone git@github.com:hacktoolkit/htk-rb.git htk`
|
13
|
+
HTTPS: `git clone https://github.com/hacktoolkit/htk-rb.git`
|
14
|
+
1. (Optional) Create a symlink to `htk` inside of your app's `lib/` directory.
|
15
|
+
1. Create `local_settings.rb` and add your [Slack incoming webhook](https://slack.com/apps/A0F7XDUAZ-incoming-webhooks) URL to `SLACK_WEBHOOK_URL`.
|
16
|
+
1. In your code, simply do:
|
17
|
+
```
|
18
|
+
::Htk::Utils.slack_debug('This is seriously awesome!')
|
19
|
+
::Htk::Utils.slack_debug('Yeah, no kidding.')
|
20
|
+
```
|
21
|
+
1. Check your Slack to verify that the message was posted. If not, perhaps your token was wrong, or the Slack integration was disabled.
|
22
|
+
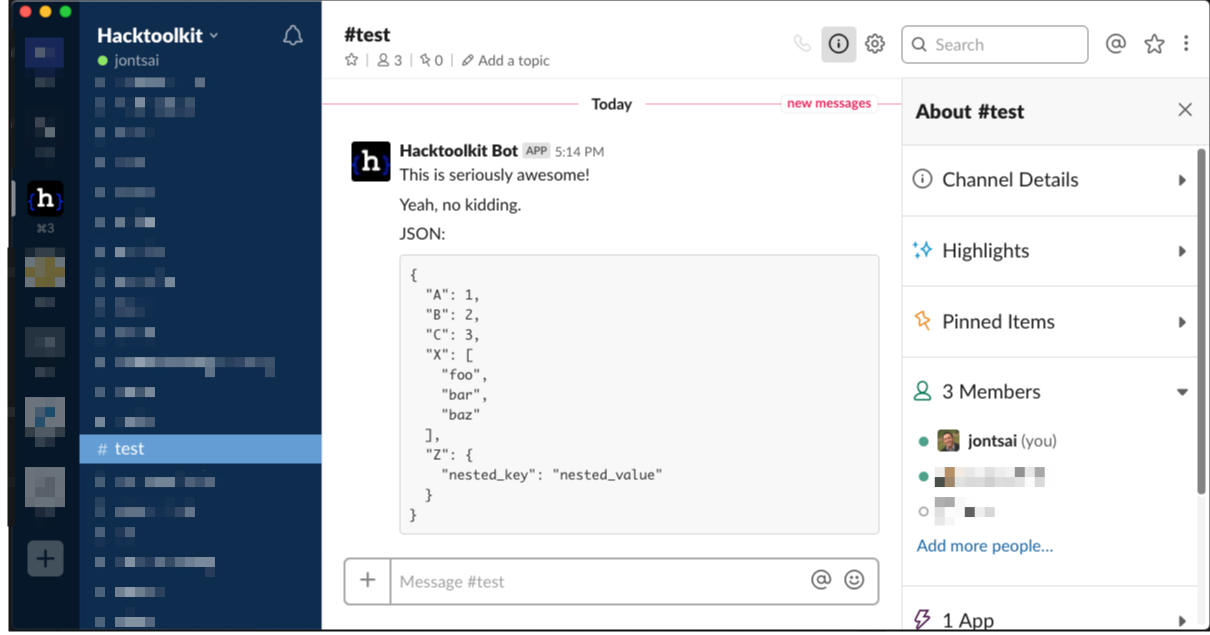
|
23
|
+
(Alternative link to screenshot above: https://cl.ly/436cfb4383a2)
|
24
|
+
1. Profit!
|
25
|
+
|
26
|
+
## Tips on Location of HTK Module
|
27
|
+
|
28
|
+
1. You can place it outside of your app directory tree, and then symlink it inside.
|
29
|
+
1. To not be nagged by the presence of the `htk` directory whenever you do `git status`, add `htk` to your `.git/info/exclude` file (like `.gitignore`, but only in your local repository, not checked in).
|
30
|
+
|
31
|
+
## Installation
|
32
|
+
|
33
|
+
Add this line to your application's Gemfile:
|
34
|
+
|
35
|
+
gem 'htk'
|
36
|
+
|
37
|
+
And then execute:
|
38
|
+
|
39
|
+
$ bundle
|
40
|
+
|
41
|
+
Or install it yourself as:
|
42
|
+
|
43
|
+
$ gem install htk
|
44
|
+
|
45
|
+
## Usage
|
46
|
+
|
47
|
+
TODO: Write usage instructions here
|
48
|
+
|
49
|
+
## Contributing
|
50
|
+
|
51
|
+
1. Fork it ( https://github.com/hacktoolkit/htk-rb/fork )
|
52
|
+
2. Create your feature branch (`git checkout -b my-new-feature`)
|
53
|
+
3. Commit your changes (`git commit -am 'Add some feature'`)
|
54
|
+
4. Push to the branch (`git push origin my-new-feature`)
|
55
|
+
5. Create a new Pull Request
|
56
|
+
|
57
|
+
# Authors and Maintainers
|
58
|
+
|
59
|
+
[Hacktoolkit](https://github.com/hacktoolkit) and [Jonathan Tsai](https://github.com/jontsai)
|
60
|
+
|
61
|
+
# License
|
62
|
+
|
63
|
+
MIT. See `LICENSE.md`
|
data/lib/apis.rb
ADDED
@@ -0,0 +1,31 @@
|
|
1
|
+
require_relative 'local_settings'
|
2
|
+
|
3
|
+
module Htk
|
4
|
+
module Apis
|
5
|
+
class Base
|
6
|
+
attr_accessor :name, :url
|
7
|
+
|
8
|
+
def initialize(name, url)
|
9
|
+
@name = name
|
10
|
+
@url = url
|
11
|
+
end
|
12
|
+
|
13
|
+
def connection(&block)
|
14
|
+
Faraday.new(url) do |faraday|
|
15
|
+
faraday.use ::Middleware::Mode
|
16
|
+
faraday.use ::Middleware::AuthParams
|
17
|
+
faraday.use ::Middleware::UseCanary
|
18
|
+
faraday.use ::Middleware::Timeout
|
19
|
+
faraday.use ::Middleware::RequestUuid
|
20
|
+
faraday.use ::Faraday::Request::Multipart
|
21
|
+
faraday.use ::Faraday::Request::UrlEncoded
|
22
|
+
faraday.use ::Faraday::Adapter::NetHttp
|
23
|
+
|
24
|
+
block.call(faraday) if block_given?
|
25
|
+
end
|
26
|
+
end
|
27
|
+
end
|
28
|
+
|
29
|
+
SlackDebug = Base.new('slack_debug', SLACK_WEBHOOK_URL)
|
30
|
+
end
|
31
|
+
end
|
metadata
ADDED
@@ -0,0 +1,78 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: htk
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.1
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Jonathan Tsai
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2020-12-16 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: bundler
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - "~>"
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '1.6'
|
20
|
+
type: :development
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - "~>"
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '1.6'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: rake
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - ">="
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '0'
|
34
|
+
type: :development
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - ">="
|
39
|
+
- !ruby/object:Gem::Version
|
40
|
+
version: '0'
|
41
|
+
description: A set of convenience utils for Ruby. A loosely inspired, not-quite-close-to-feature
|
42
|
+
parity port of [`pyhtk-lite`](https://github.com/hacktoolkit/pyhtk-lite).
|
43
|
+
email:
|
44
|
+
- hello@jontsaicom
|
45
|
+
executables: []
|
46
|
+
extensions: []
|
47
|
+
extra_rdoc_files: []
|
48
|
+
files:
|
49
|
+
- ".editorconfig"
|
50
|
+
- ".gitignore"
|
51
|
+
- LICENSE.md
|
52
|
+
- README.md
|
53
|
+
- lib/apis.rb
|
54
|
+
homepage: https://github.com/hacktoolkit/htk-rb
|
55
|
+
licenses:
|
56
|
+
- MIT
|
57
|
+
metadata: {}
|
58
|
+
post_install_message:
|
59
|
+
rdoc_options: []
|
60
|
+
require_paths:
|
61
|
+
- lib
|
62
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
63
|
+
requirements:
|
64
|
+
- - ">="
|
65
|
+
- !ruby/object:Gem::Version
|
66
|
+
version: '0'
|
67
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
68
|
+
requirements:
|
69
|
+
- - ">="
|
70
|
+
- !ruby/object:Gem::Version
|
71
|
+
version: '0'
|
72
|
+
requirements: []
|
73
|
+
rubyforge_project:
|
74
|
+
rubygems_version: 2.2.2
|
75
|
+
signing_key:
|
76
|
+
specification_version: 4
|
77
|
+
summary: A set of convenience utils for Ruby.
|
78
|
+
test_files: []
|