gosu_texture_packer 0.1.1 → 0.1.2
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/CONTRIBUTING.md +7 -0
- data/README.md +7 -6
- data/examples/random_map.rb +67 -0
- data/examples/screenshots/random_map.png +0 -0
- data/gosu_texture_packer.gemspec +1 -0
- data/lib/gosu_texture_packer/tileset.rb +7 -2
- data/lib/gosu_texture_packer/version.rb +1 -1
- data/spec/files/ATTRIBUTION.md +3 -2
- data/spec/files/ground.json +492 -0
- data/spec/files/ground.png +0 -0
- metadata +22 -1
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: af48671121a22d94b41d7515d590a35d5cb69efa
|
4
|
+
data.tar.gz: 4a78ca27ca52ab4e8d9b0bb8d6318e09c8d26aec
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 1376f1f0358f8ca3f3550c92163a13ef170c208b451f7c43a6058e1ee4dea1d8606e82672a9b81abec3544890d22c19cf1a05a3f2d4dba7b5e717a9d56c15d01
|
7
|
+
data.tar.gz: b5a6936182ef3d2029e07ef4b7908e51557262f54fab2991ad7308191077affb0ff2a43969015f2e4fe6f34a6a985848c4c53fe1ba1a03655c20a1b0ca3fe2cb
|
data/CONTRIBUTING.md
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
Before you make a pull request:
|
2
|
+
|
3
|
+
- Make sure your code is formatted using [this ruby style guide](https://github.com/bbatsov/ruby-style-guide)
|
4
|
+
- Trim all trailing whitespace
|
5
|
+
- Run `rspec` to see if all old tests pass
|
6
|
+
- Add new tests for your code
|
7
|
+
- Consult [betterspecs.org](http://betterspecs.org/) when writing new tests
|
data/README.md
CHANGED
@@ -1,9 +1,9 @@
|
|
1
|
-
#
|
1
|
+
# gosu_texture_packer
|
2
2
|
|
3
3
|
[](http://badge.fury.io/rb/gosu_texture_packer)
|
4
4
|
[](https://codeclimate.com/github/spajus/gosu-texture-packer)
|
5
5
|
|
6
|
-
[
|
6
|
+
Ruby gem that provides [Texture Packer](http://www.codeandweb.com/texturepacker) support for [Gosu](https://github.com/jlnr/gosu) game engine.
|
7
7
|
|
8
8
|
## Installation
|
9
9
|
|
@@ -31,18 +31,19 @@ tile.draw(0, 0, 0) # tile is Gosu::Image
|
|
31
31
|
## Example Code
|
32
32
|
|
33
33
|
Run
|
34
|
-
[examples/tile_brush.rb](https://github.com/spajus/gosu-texture-packer/blob/master/examples/tile_brush.rb)
|
34
|
+
[examples/tile_brush.rb](https://github.com/spajus/gosu-texture-packer/blob/master/examples/tile_brush.rb) or [examples/random_map.rb](https://github.com/spajus/gosu-texture-packer/blob/master/examples/random_map.rb)
|
35
35
|
to see `gosu_texture_packer` in action.
|
36
36
|
|
37
|
-
Example
|
37
|
+
Example tilesets are loaded from
|
38
38
|
[spec/files](https://github.com/spajus/gosu-texture-packer/tree/master/spec/files) directory.
|
39
39
|
|
40
|
-
Click the window to draw random tiles.
|
41
|
-
|
42
40
|
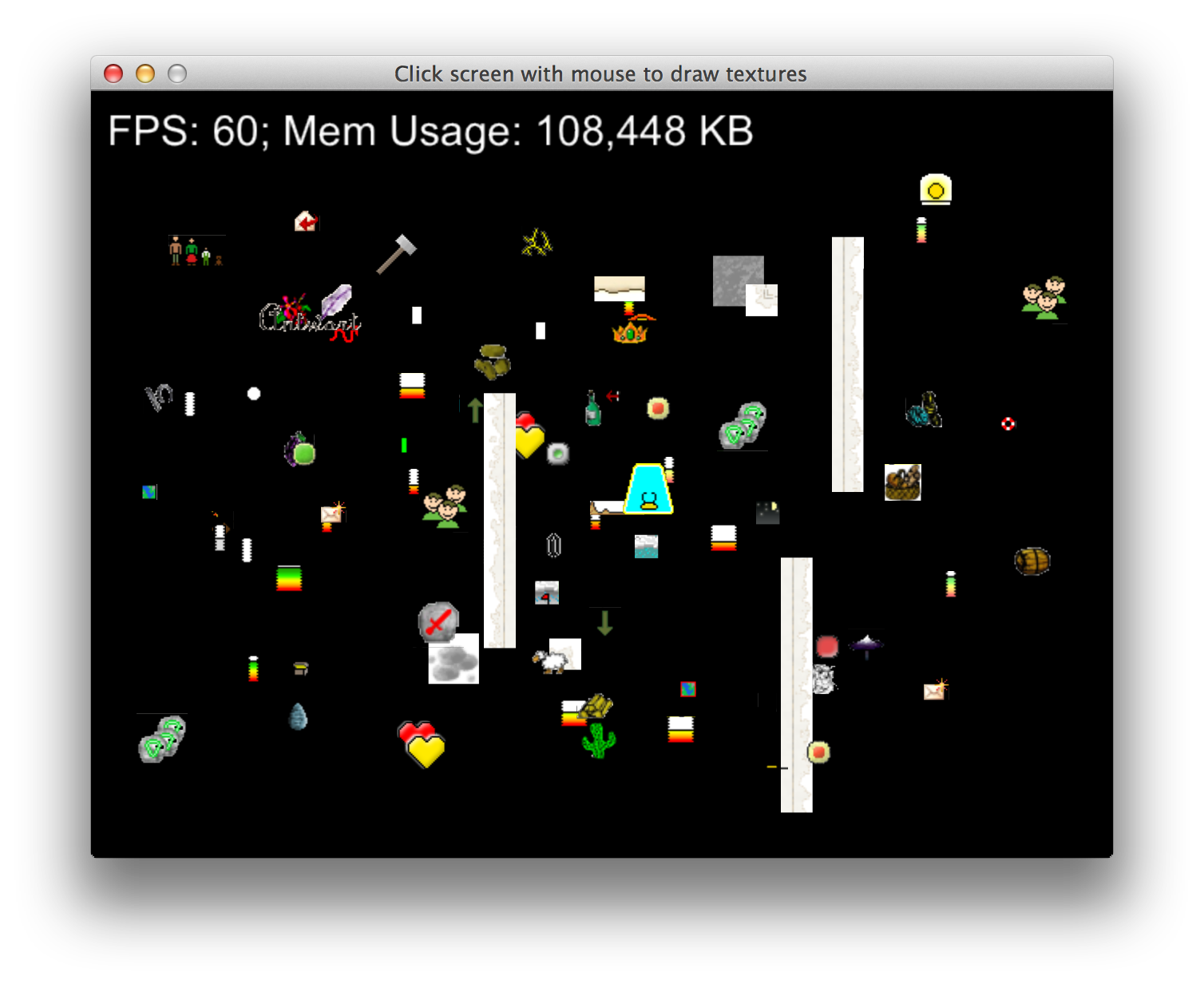
|
43
41
|
|
42
|
+
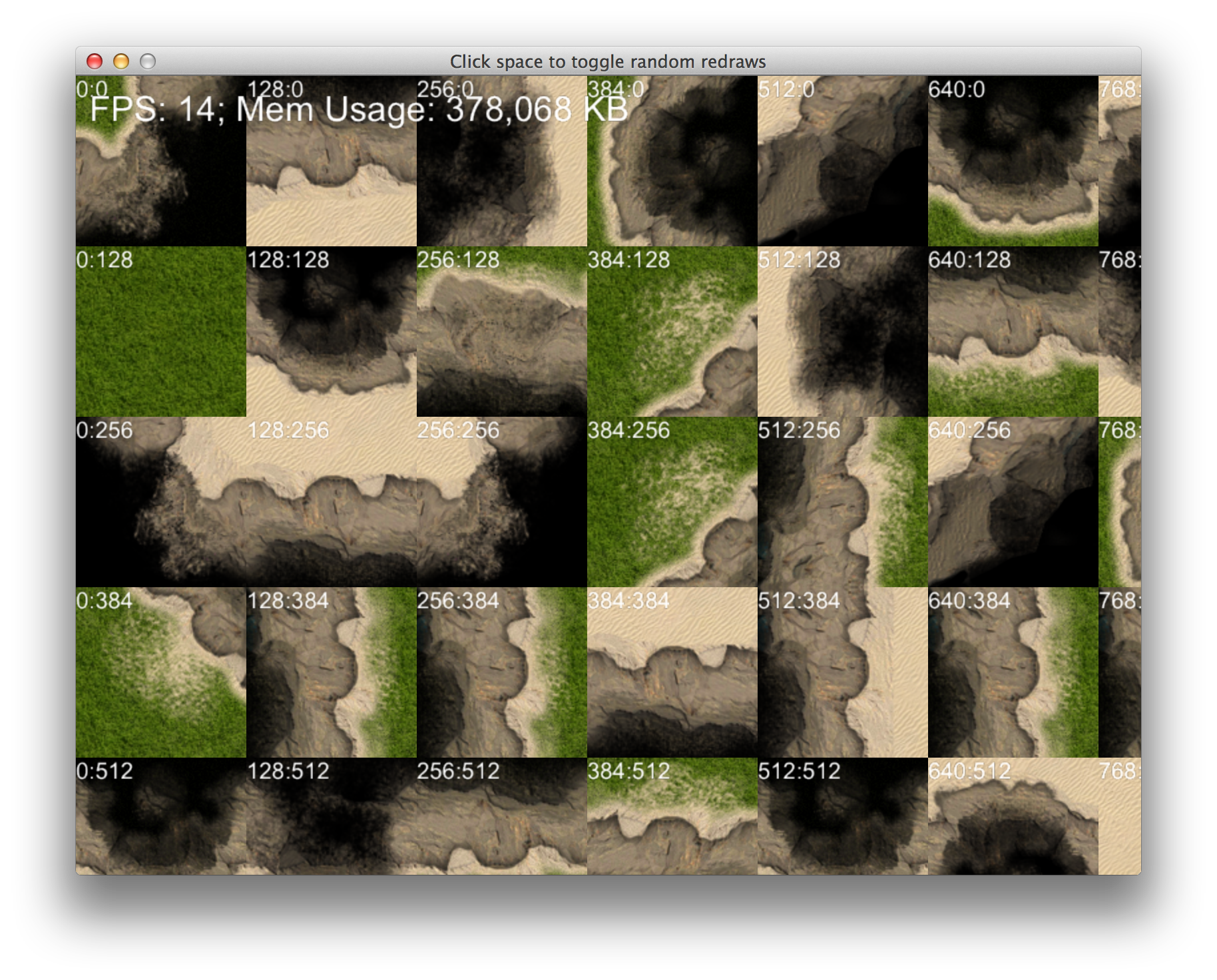
|
43
|
+
|
44
44
|
## Contributing
|
45
45
|
|
46
|
+
0. Read [CONTRIBUTING.md](https://github.com/spajus/gosu-texture-packer/blob/master/CONTRIBUTING.md)
|
46
47
|
1. Fork it ( https://github.com/[my-github-username]/gosu_texture_packer/fork )
|
47
48
|
2. Create your feature branch (`git checkout -b my-new-feature`)
|
48
49
|
3. Commit your changes (`git commit -am 'Add some feature'`)
|
@@ -0,0 +1,67 @@
|
|
1
|
+
ROOT_DIR = File.dirname(File.dirname(__FILE__))
|
2
|
+
|
3
|
+
# Add ../lib to load path
|
4
|
+
$:.unshift File.join(ROOT_DIR, 'lib')
|
5
|
+
|
6
|
+
require 'gosu'
|
7
|
+
require 'gosu_texture_packer'
|
8
|
+
|
9
|
+
class GameWindow < Gosu::Window
|
10
|
+
WIDTH = 800
|
11
|
+
HEIGHT = 600
|
12
|
+
TILE_SIZE = 128
|
13
|
+
def initialize
|
14
|
+
super(WIDTH, HEIGHT, false)
|
15
|
+
self.caption = 'Click space to toggle random redraws'
|
16
|
+
json_path = File.join(ROOT_DIR, 'spec', 'files', 'ground.json')
|
17
|
+
@tileset = Gosu::TexturePacker.load_json(self, json_path)
|
18
|
+
end
|
19
|
+
|
20
|
+
def needs_cursor?
|
21
|
+
true
|
22
|
+
end
|
23
|
+
|
24
|
+
def update
|
25
|
+
@fps = Gosu::Image.from_text(
|
26
|
+
self, "FPS: #{Gosu.fps}; Mem Usage: #{memory_usage}",
|
27
|
+
Gosu.default_font_name, 30)
|
28
|
+
end
|
29
|
+
|
30
|
+
def button_down(id)
|
31
|
+
close if id == Gosu::KbEscape
|
32
|
+
@redraw = !@redraw if id == Gosu::KbSpace
|
33
|
+
end
|
34
|
+
|
35
|
+
def needs_redraw?
|
36
|
+
if @redraw.nil?
|
37
|
+
@redraw = false
|
38
|
+
true
|
39
|
+
else
|
40
|
+
@redraw
|
41
|
+
end
|
42
|
+
end
|
43
|
+
|
44
|
+
def draw
|
45
|
+
(0..WIDTH / TILE_SIZE ).each do |x|
|
46
|
+
(0..HEIGHT / TILE_SIZE ).each do |y|
|
47
|
+
xx = x * (TILE_SIZE)
|
48
|
+
yy = y * (TILE_SIZE)
|
49
|
+
text = "#{xx}:#{yy}"
|
50
|
+
@tileset.frame(@tileset.frame_list.sample).draw(xx, yy, 0)
|
51
|
+
Gosu::Image.from_text(self, text, Gosu.default_font_name, 20).draw(xx, yy, 1)
|
52
|
+
end
|
53
|
+
end
|
54
|
+
@fps.draw(10, 10, 1)
|
55
|
+
end
|
56
|
+
|
57
|
+
private
|
58
|
+
|
59
|
+
def memory_usage
|
60
|
+
`ps -o rss= -p #{Process.pid}`
|
61
|
+
.chomp.gsub(/(\d)(?=(\d{3})+(\..*)?$)/,'\1,') + ' KB'
|
62
|
+
rescue
|
63
|
+
"Unavailable. Using Windows?"
|
64
|
+
end
|
65
|
+
end
|
66
|
+
|
67
|
+
GameWindow.new.show
|
Binary file
|
data/gosu_texture_packer.gemspec
CHANGED
@@ -1,4 +1,5 @@
|
|
1
1
|
require 'json'
|
2
|
+
require 'rmagick'
|
2
3
|
module Gosu
|
3
4
|
module TexturePacker
|
4
5
|
class Tileset
|
@@ -11,7 +12,7 @@ module Gosu
|
|
11
12
|
@window = window
|
12
13
|
@json = JSON.parse(File.read(json))
|
13
14
|
@source_dir = File.dirname(json)
|
14
|
-
@main_image =
|
15
|
+
@main_image = Magick::ImageList.new(image_file).first
|
15
16
|
@tile_cache = {}
|
16
17
|
end
|
17
18
|
|
@@ -24,7 +25,7 @@ module Gosu
|
|
24
25
|
unless tile
|
25
26
|
data = frames[name]
|
26
27
|
f = data['frame']
|
27
|
-
tile =
|
28
|
+
tile = build_tile(f)
|
28
29
|
@tile_cache[name] = tile
|
29
30
|
end
|
30
31
|
tile
|
@@ -32,6 +33,10 @@ module Gosu
|
|
32
33
|
|
33
34
|
private
|
34
35
|
|
36
|
+
def build_tile(f)
|
37
|
+
Gosu::Image.new(@window, @main_image, true, f['x'], f['y'], f['w'], f['h'])
|
38
|
+
end
|
39
|
+
|
35
40
|
def image_file
|
36
41
|
File.join(@source_dir, meta['image'])
|
37
42
|
end
|
data/spec/files/ATTRIBUTION.md
CHANGED
@@ -1,2 +1,3 @@
|
|
1
|
-
Art
|
2
|
-
|
1
|
+
Art sources:
|
2
|
+
- http://opengameart.org/content/zwischenwelt-tileset
|
3
|
+
- http://opengameart.org/content/ground-tileset-grass-sand
|
@@ -0,0 +1,492 @@
|
|
1
|
+
{"frames": {
|
2
|
+
|
3
|
+
"beach_bl.png":
|
4
|
+
{
|
5
|
+
"frame": {"x":896,"y":640,"w":128,"h":128},
|
6
|
+
"rotated": false,
|
7
|
+
"trimmed": false,
|
8
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
9
|
+
"sourceSize": {"w":128,"h":128}
|
10
|
+
},
|
11
|
+
"beach_bl_grass.png":
|
12
|
+
{
|
13
|
+
"frame": {"x":768,"y":640,"w":128,"h":128},
|
14
|
+
"rotated": false,
|
15
|
+
"trimmed": false,
|
16
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
17
|
+
"sourceSize": {"w":128,"h":128}
|
18
|
+
},
|
19
|
+
"beach_bm_01.png":
|
20
|
+
{
|
21
|
+
"frame": {"x":640,"y":896,"w":128,"h":128},
|
22
|
+
"rotated": false,
|
23
|
+
"trimmed": false,
|
24
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
25
|
+
"sourceSize": {"w":128,"h":128}
|
26
|
+
},
|
27
|
+
"beach_bm_01_grass.png":
|
28
|
+
{
|
29
|
+
"frame": {"x":640,"y":768,"w":128,"h":128},
|
30
|
+
"rotated": false,
|
31
|
+
"trimmed": false,
|
32
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
33
|
+
"sourceSize": {"w":128,"h":128}
|
34
|
+
},
|
35
|
+
"beach_bm_02.png":
|
36
|
+
{
|
37
|
+
"frame": {"x":640,"y":640,"w":128,"h":128},
|
38
|
+
"rotated": false,
|
39
|
+
"trimmed": false,
|
40
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
41
|
+
"sourceSize": {"w":128,"h":128}
|
42
|
+
},
|
43
|
+
"beach_bm_02_grass.png":
|
44
|
+
{
|
45
|
+
"frame": {"x":896,"y":512,"w":128,"h":128},
|
46
|
+
"rotated": false,
|
47
|
+
"trimmed": false,
|
48
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
49
|
+
"sourceSize": {"w":128,"h":128}
|
50
|
+
},
|
51
|
+
"beach_bm_03.png":
|
52
|
+
{
|
53
|
+
"frame": {"x":768,"y":512,"w":128,"h":128},
|
54
|
+
"rotated": false,
|
55
|
+
"trimmed": false,
|
56
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
57
|
+
"sourceSize": {"w":128,"h":128}
|
58
|
+
},
|
59
|
+
"beach_bm_03_grass.png":
|
60
|
+
{
|
61
|
+
"frame": {"x":640,"y":512,"w":128,"h":128},
|
62
|
+
"rotated": false,
|
63
|
+
"trimmed": false,
|
64
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
65
|
+
"sourceSize": {"w":128,"h":128}
|
66
|
+
},
|
67
|
+
"beach_bm_04.png":
|
68
|
+
{
|
69
|
+
"frame": {"x":512,"y":896,"w":128,"h":128},
|
70
|
+
"rotated": false,
|
71
|
+
"trimmed": false,
|
72
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
73
|
+
"sourceSize": {"w":128,"h":128}
|
74
|
+
},
|
75
|
+
"beach_bm_04_grass.png":
|
76
|
+
{
|
77
|
+
"frame": {"x":512,"y":768,"w":128,"h":128},
|
78
|
+
"rotated": false,
|
79
|
+
"trimmed": false,
|
80
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
81
|
+
"sourceSize": {"w":128,"h":128}
|
82
|
+
},
|
83
|
+
"beach_bm_05.png":
|
84
|
+
{
|
85
|
+
"frame": {"x":512,"y":640,"w":128,"h":128},
|
86
|
+
"rotated": false,
|
87
|
+
"trimmed": false,
|
88
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
89
|
+
"sourceSize": {"w":128,"h":128}
|
90
|
+
},
|
91
|
+
"beach_bm_05_grass.png":
|
92
|
+
{
|
93
|
+
"frame": {"x":512,"y":512,"w":128,"h":128},
|
94
|
+
"rotated": false,
|
95
|
+
"trimmed": false,
|
96
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
97
|
+
"sourceSize": {"w":128,"h":128}
|
98
|
+
},
|
99
|
+
"beach_br.png":
|
100
|
+
{
|
101
|
+
"frame": {"x":896,"y":384,"w":128,"h":128},
|
102
|
+
"rotated": false,
|
103
|
+
"trimmed": false,
|
104
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
105
|
+
"sourceSize": {"w":128,"h":128}
|
106
|
+
},
|
107
|
+
"beach_br_grass.png":
|
108
|
+
{
|
109
|
+
"frame": {"x":768,"y":384,"w":128,"h":128},
|
110
|
+
"rotated": false,
|
111
|
+
"trimmed": false,
|
112
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
113
|
+
"sourceSize": {"w":128,"h":128}
|
114
|
+
},
|
115
|
+
"beach_l_up_diagonal.png":
|
116
|
+
{
|
117
|
+
"frame": {"x":640,"y":384,"w":128,"h":128},
|
118
|
+
"rotated": false,
|
119
|
+
"trimmed": false,
|
120
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
121
|
+
"sourceSize": {"w":128,"h":128}
|
122
|
+
},
|
123
|
+
"beach_l_up_diagonal_grass.png":
|
124
|
+
{
|
125
|
+
"frame": {"x":512,"y":384,"w":128,"h":128},
|
126
|
+
"rotated": false,
|
127
|
+
"trimmed": false,
|
128
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
129
|
+
"sourceSize": {"w":128,"h":128}
|
130
|
+
},
|
131
|
+
"beach_l_up_diagonal_neighbour.png":
|
132
|
+
{
|
133
|
+
"frame": {"x":384,"y":896,"w":128,"h":128},
|
134
|
+
"rotated": false,
|
135
|
+
"trimmed": false,
|
136
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
137
|
+
"sourceSize": {"w":128,"h":128}
|
138
|
+
},
|
139
|
+
"beach_l_up_diagonal_neighbour_grass.png":
|
140
|
+
{
|
141
|
+
"frame": {"x":384,"y":768,"w":128,"h":128},
|
142
|
+
"rotated": false,
|
143
|
+
"trimmed": false,
|
144
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
145
|
+
"sourceSize": {"w":128,"h":128}
|
146
|
+
},
|
147
|
+
"beach_lm_01.png":
|
148
|
+
{
|
149
|
+
"frame": {"x":384,"y":640,"w":128,"h":128},
|
150
|
+
"rotated": false,
|
151
|
+
"trimmed": false,
|
152
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
153
|
+
"sourceSize": {"w":128,"h":128}
|
154
|
+
},
|
155
|
+
"beach_lm_01_grass.png":
|
156
|
+
{
|
157
|
+
"frame": {"x":384,"y":512,"w":128,"h":128},
|
158
|
+
"rotated": false,
|
159
|
+
"trimmed": false,
|
160
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
161
|
+
"sourceSize": {"w":128,"h":128}
|
162
|
+
},
|
163
|
+
"beach_lm_02.png":
|
164
|
+
{
|
165
|
+
"frame": {"x":384,"y":384,"w":128,"h":128},
|
166
|
+
"rotated": false,
|
167
|
+
"trimmed": false,
|
168
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
169
|
+
"sourceSize": {"w":128,"h":128}
|
170
|
+
},
|
171
|
+
"beach_lm_02_grass.png":
|
172
|
+
{
|
173
|
+
"frame": {"x":896,"y":256,"w":128,"h":128},
|
174
|
+
"rotated": false,
|
175
|
+
"trimmed": false,
|
176
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
177
|
+
"sourceSize": {"w":128,"h":128}
|
178
|
+
},
|
179
|
+
"beach_lm_03.png":
|
180
|
+
{
|
181
|
+
"frame": {"x":768,"y":256,"w":128,"h":128},
|
182
|
+
"rotated": false,
|
183
|
+
"trimmed": false,
|
184
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
185
|
+
"sourceSize": {"w":128,"h":128}
|
186
|
+
},
|
187
|
+
"beach_lm_03_grass.png":
|
188
|
+
{
|
189
|
+
"frame": {"x":640,"y":256,"w":128,"h":128},
|
190
|
+
"rotated": false,
|
191
|
+
"trimmed": false,
|
192
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
193
|
+
"sourceSize": {"w":128,"h":128}
|
194
|
+
},
|
195
|
+
"beach_lm_04.png":
|
196
|
+
{
|
197
|
+
"frame": {"x":512,"y":256,"w":128,"h":128},
|
198
|
+
"rotated": false,
|
199
|
+
"trimmed": false,
|
200
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
201
|
+
"sourceSize": {"w":128,"h":128}
|
202
|
+
},
|
203
|
+
"beach_lm_04_grass.png":
|
204
|
+
{
|
205
|
+
"frame": {"x":384,"y":256,"w":128,"h":128},
|
206
|
+
"rotated": false,
|
207
|
+
"trimmed": false,
|
208
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
209
|
+
"sourceSize": {"w":128,"h":128}
|
210
|
+
},
|
211
|
+
"beach_r_diagonal_neighbour.png":
|
212
|
+
{
|
213
|
+
"frame": {"x":256,"y":896,"w":128,"h":128},
|
214
|
+
"rotated": false,
|
215
|
+
"trimmed": false,
|
216
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
217
|
+
"sourceSize": {"w":128,"h":128}
|
218
|
+
},
|
219
|
+
"beach_r_diagonal_neighbour_grass.png":
|
220
|
+
{
|
221
|
+
"frame": {"x":256,"y":768,"w":128,"h":128},
|
222
|
+
"rotated": false,
|
223
|
+
"trimmed": false,
|
224
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
225
|
+
"sourceSize": {"w":128,"h":128}
|
226
|
+
},
|
227
|
+
"beach_r_down_diagonal.png":
|
228
|
+
{
|
229
|
+
"frame": {"x":256,"y":640,"w":128,"h":128},
|
230
|
+
"rotated": false,
|
231
|
+
"trimmed": false,
|
232
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
233
|
+
"sourceSize": {"w":128,"h":128}
|
234
|
+
},
|
235
|
+
"beach_r_down_diagonal_grass.png":
|
236
|
+
{
|
237
|
+
"frame": {"x":256,"y":512,"w":128,"h":128},
|
238
|
+
"rotated": false,
|
239
|
+
"trimmed": false,
|
240
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
241
|
+
"sourceSize": {"w":128,"h":128}
|
242
|
+
},
|
243
|
+
"beach_r_down_diagonal_neighbour.png":
|
244
|
+
{
|
245
|
+
"frame": {"x":256,"y":384,"w":128,"h":128},
|
246
|
+
"rotated": false,
|
247
|
+
"trimmed": false,
|
248
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
249
|
+
"sourceSize": {"w":128,"h":128}
|
250
|
+
},
|
251
|
+
"beach_r_down_diagonal_neighbour_grass.png":
|
252
|
+
{
|
253
|
+
"frame": {"x":256,"y":256,"w":128,"h":128},
|
254
|
+
"rotated": false,
|
255
|
+
"trimmed": false,
|
256
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
257
|
+
"sourceSize": {"w":128,"h":128}
|
258
|
+
},
|
259
|
+
"beach_r_up_diagonal.png":
|
260
|
+
{
|
261
|
+
"frame": {"x":896,"y":128,"w":128,"h":128},
|
262
|
+
"rotated": false,
|
263
|
+
"trimmed": false,
|
264
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
265
|
+
"sourceSize": {"w":128,"h":128}
|
266
|
+
},
|
267
|
+
"beach_r_up_diagonal_grass.png":
|
268
|
+
{
|
269
|
+
"frame": {"x":768,"y":128,"w":128,"h":128},
|
270
|
+
"rotated": false,
|
271
|
+
"trimmed": false,
|
272
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
273
|
+
"sourceSize": {"w":128,"h":128}
|
274
|
+
},
|
275
|
+
"beach_rm_01-22.png":
|
276
|
+
{
|
277
|
+
"frame": {"x":256,"y":128,"w":128,"h":128},
|
278
|
+
"rotated": false,
|
279
|
+
"trimmed": false,
|
280
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
281
|
+
"sourceSize": {"w":128,"h":128}
|
282
|
+
},
|
283
|
+
"beach_rm_01.png":
|
284
|
+
{
|
285
|
+
"frame": {"x":640,"y":128,"w":128,"h":128},
|
286
|
+
"rotated": false,
|
287
|
+
"trimmed": false,
|
288
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
289
|
+
"sourceSize": {"w":128,"h":128}
|
290
|
+
},
|
291
|
+
"beach_rm_01_grass-22.png":
|
292
|
+
{
|
293
|
+
"frame": {"x":384,"y":128,"w":128,"h":128},
|
294
|
+
"rotated": false,
|
295
|
+
"trimmed": false,
|
296
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
297
|
+
"sourceSize": {"w":128,"h":128}
|
298
|
+
},
|
299
|
+
"beach_rm_01_grass.png":
|
300
|
+
{
|
301
|
+
"frame": {"x":512,"y":128,"w":128,"h":128},
|
302
|
+
"rotated": false,
|
303
|
+
"trimmed": false,
|
304
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
305
|
+
"sourceSize": {"w":128,"h":128}
|
306
|
+
},
|
307
|
+
"beach_rm_02.png":
|
308
|
+
{
|
309
|
+
"frame": {"x":128,"y":896,"w":128,"h":128},
|
310
|
+
"rotated": false,
|
311
|
+
"trimmed": false,
|
312
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
313
|
+
"sourceSize": {"w":128,"h":128}
|
314
|
+
},
|
315
|
+
"beach_rm_02_grass.png":
|
316
|
+
{
|
317
|
+
"frame": {"x":128,"y":768,"w":128,"h":128},
|
318
|
+
"rotated": false,
|
319
|
+
"trimmed": false,
|
320
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
321
|
+
"sourceSize": {"w":128,"h":128}
|
322
|
+
},
|
323
|
+
"beach_rm_03.png":
|
324
|
+
{
|
325
|
+
"frame": {"x":128,"y":640,"w":128,"h":128},
|
326
|
+
"rotated": false,
|
327
|
+
"trimmed": false,
|
328
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
329
|
+
"sourceSize": {"w":128,"h":128}
|
330
|
+
},
|
331
|
+
"beach_rm_03_grass.png":
|
332
|
+
{
|
333
|
+
"frame": {"x":128,"y":512,"w":128,"h":128},
|
334
|
+
"rotated": false,
|
335
|
+
"trimmed": false,
|
336
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
337
|
+
"sourceSize": {"w":128,"h":128}
|
338
|
+
},
|
339
|
+
"beach_rm_04.png":
|
340
|
+
{
|
341
|
+
"frame": {"x":128,"y":384,"w":128,"h":128},
|
342
|
+
"rotated": false,
|
343
|
+
"trimmed": false,
|
344
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
345
|
+
"sourceSize": {"w":128,"h":128}
|
346
|
+
},
|
347
|
+
"beach_rm_04_grass.png":
|
348
|
+
{
|
349
|
+
"frame": {"x":128,"y":256,"w":128,"h":128},
|
350
|
+
"rotated": false,
|
351
|
+
"trimmed": false,
|
352
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
353
|
+
"sourceSize": {"w":128,"h":128}
|
354
|
+
},
|
355
|
+
"beach_rm_05.png":
|
356
|
+
{
|
357
|
+
"frame": {"x":128,"y":128,"w":128,"h":128},
|
358
|
+
"rotated": false,
|
359
|
+
"trimmed": false,
|
360
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
361
|
+
"sourceSize": {"w":128,"h":128}
|
362
|
+
},
|
363
|
+
"beach_rm_05_grass.png":
|
364
|
+
{
|
365
|
+
"frame": {"x":896,"y":0,"w":128,"h":128},
|
366
|
+
"rotated": false,
|
367
|
+
"trimmed": false,
|
368
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
369
|
+
"sourceSize": {"w":128,"h":128}
|
370
|
+
},
|
371
|
+
"beach_tl.png":
|
372
|
+
{
|
373
|
+
"frame": {"x":768,"y":0,"w":128,"h":128},
|
374
|
+
"rotated": false,
|
375
|
+
"trimmed": false,
|
376
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
377
|
+
"sourceSize": {"w":128,"h":128}
|
378
|
+
},
|
379
|
+
"beach_tl_grass.png":
|
380
|
+
{
|
381
|
+
"frame": {"x":640,"y":0,"w":128,"h":128},
|
382
|
+
"rotated": false,
|
383
|
+
"trimmed": false,
|
384
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
385
|
+
"sourceSize": {"w":128,"h":128}
|
386
|
+
},
|
387
|
+
"beach_tm_01.png":
|
388
|
+
{
|
389
|
+
"frame": {"x":512,"y":0,"w":128,"h":128},
|
390
|
+
"rotated": false,
|
391
|
+
"trimmed": false,
|
392
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
393
|
+
"sourceSize": {"w":128,"h":128}
|
394
|
+
},
|
395
|
+
"beach_tm_01_grass.png":
|
396
|
+
{
|
397
|
+
"frame": {"x":384,"y":0,"w":128,"h":128},
|
398
|
+
"rotated": false,
|
399
|
+
"trimmed": false,
|
400
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
401
|
+
"sourceSize": {"w":128,"h":128}
|
402
|
+
},
|
403
|
+
"beach_tm_02.png":
|
404
|
+
{
|
405
|
+
"frame": {"x":256,"y":0,"w":128,"h":128},
|
406
|
+
"rotated": false,
|
407
|
+
"trimmed": false,
|
408
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
409
|
+
"sourceSize": {"w":128,"h":128}
|
410
|
+
},
|
411
|
+
"beach_tm_02_grass.png":
|
412
|
+
{
|
413
|
+
"frame": {"x":128,"y":0,"w":128,"h":128},
|
414
|
+
"rotated": false,
|
415
|
+
"trimmed": false,
|
416
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
417
|
+
"sourceSize": {"w":128,"h":128}
|
418
|
+
},
|
419
|
+
"beach_tm_03.png":
|
420
|
+
{
|
421
|
+
"frame": {"x":0,"y":896,"w":128,"h":128},
|
422
|
+
"rotated": false,
|
423
|
+
"trimmed": false,
|
424
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
425
|
+
"sourceSize": {"w":128,"h":128}
|
426
|
+
},
|
427
|
+
"beach_tm_03_grass.png":
|
428
|
+
{
|
429
|
+
"frame": {"x":0,"y":768,"w":128,"h":128},
|
430
|
+
"rotated": false,
|
431
|
+
"trimmed": false,
|
432
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
433
|
+
"sourceSize": {"w":128,"h":128}
|
434
|
+
},
|
435
|
+
"beach_tm_04.png":
|
436
|
+
{
|
437
|
+
"frame": {"x":0,"y":640,"w":128,"h":128},
|
438
|
+
"rotated": false,
|
439
|
+
"trimmed": false,
|
440
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
441
|
+
"sourceSize": {"w":128,"h":128}
|
442
|
+
},
|
443
|
+
"beach_tm_04_grass.png":
|
444
|
+
{
|
445
|
+
"frame": {"x":0,"y":512,"w":128,"h":128},
|
446
|
+
"rotated": false,
|
447
|
+
"trimmed": false,
|
448
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
449
|
+
"sourceSize": {"w":128,"h":128}
|
450
|
+
},
|
451
|
+
"beach_tr.png":
|
452
|
+
{
|
453
|
+
"frame": {"x":0,"y":384,"w":128,"h":128},
|
454
|
+
"rotated": false,
|
455
|
+
"trimmed": false,
|
456
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
457
|
+
"sourceSize": {"w":128,"h":128}
|
458
|
+
},
|
459
|
+
"beach_tr_grass.png":
|
460
|
+
{
|
461
|
+
"frame": {"x":0,"y":256,"w":128,"h":128},
|
462
|
+
"rotated": false,
|
463
|
+
"trimmed": false,
|
464
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
465
|
+
"sourceSize": {"w":128,"h":128}
|
466
|
+
},
|
467
|
+
"grass.png":
|
468
|
+
{
|
469
|
+
"frame": {"x":0,"y":128,"w":128,"h":128},
|
470
|
+
"rotated": false,
|
471
|
+
"trimmed": false,
|
472
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
473
|
+
"sourceSize": {"w":128,"h":128}
|
474
|
+
},
|
475
|
+
"sand.png":
|
476
|
+
{
|
477
|
+
"frame": {"x":0,"y":0,"w":128,"h":128},
|
478
|
+
"rotated": false,
|
479
|
+
"trimmed": false,
|
480
|
+
"spriteSourceSize": {"x":0,"y":0,"w":128,"h":128},
|
481
|
+
"sourceSize": {"w":128,"h":128}
|
482
|
+
}},
|
483
|
+
"meta": {
|
484
|
+
"app": "http://www.texturepacker.com",
|
485
|
+
"version": "1.0",
|
486
|
+
"image": "ground.png",
|
487
|
+
"format": "RGBA8888",
|
488
|
+
"size": {"w":1024,"h":1024},
|
489
|
+
"scale": "1",
|
490
|
+
"smartupdate": "$TexturePacker:SmartUpdate:a4e7956c80b1dabce22dc97d34f9811b$"
|
491
|
+
}
|
492
|
+
}
|
Binary file
|
metadata
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: gosu_texture_packer
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.2
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Tomas Varaneckas
|
@@ -38,6 +38,20 @@ dependencies:
|
|
38
38
|
- - '>='
|
39
39
|
- !ruby/object:Gem::Version
|
40
40
|
version: '0'
|
41
|
+
- !ruby/object:Gem::Dependency
|
42
|
+
name: rmagick
|
43
|
+
requirement: !ruby/object:Gem::Requirement
|
44
|
+
requirements:
|
45
|
+
- - '>='
|
46
|
+
- !ruby/object:Gem::Version
|
47
|
+
version: '0'
|
48
|
+
type: :runtime
|
49
|
+
prerelease: false
|
50
|
+
version_requirements: !ruby/object:Gem::Requirement
|
51
|
+
requirements:
|
52
|
+
- - '>='
|
53
|
+
- !ruby/object:Gem::Version
|
54
|
+
version: '0'
|
41
55
|
- !ruby/object:Gem::Dependency
|
42
56
|
name: bundler
|
43
57
|
requirement: !ruby/object:Gem::Requirement
|
@@ -102,12 +116,15 @@ extensions: []
|
|
102
116
|
extra_rdoc_files: []
|
103
117
|
files:
|
104
118
|
- .gitignore
|
119
|
+
- CONTRIBUTING.md
|
105
120
|
- Gemfile
|
106
121
|
- Guardfile
|
107
122
|
- LICENSE
|
108
123
|
- LICENSE.txt
|
109
124
|
- README.md
|
110
125
|
- Rakefile
|
126
|
+
- examples/random_map.rb
|
127
|
+
- examples/screenshots/random_map.png
|
111
128
|
- examples/screenshots/tile_brush.png
|
112
129
|
- examples/tile_brush.rb
|
113
130
|
- gosu_texture_packer.gemspec
|
@@ -117,6 +134,8 @@ files:
|
|
117
134
|
- spec/files/ATTRIBUTION.md
|
118
135
|
- spec/files/_UI.json
|
119
136
|
- spec/files/_UI.png
|
137
|
+
- spec/files/ground.json
|
138
|
+
- spec/files/ground.png
|
120
139
|
- spec/gosu_texture_packer/tileset_spec.rb
|
121
140
|
- spec/gosu_texture_packer_spec.rb
|
122
141
|
- spec/spec_helper.rb
|
@@ -148,6 +167,8 @@ test_files:
|
|
148
167
|
- spec/files/ATTRIBUTION.md
|
149
168
|
- spec/files/_UI.json
|
150
169
|
- spec/files/_UI.png
|
170
|
+
- spec/files/ground.json
|
171
|
+
- spec/files/ground.png
|
151
172
|
- spec/gosu_texture_packer/tileset_spec.rb
|
152
173
|
- spec/gosu_texture_packer_spec.rb
|
153
174
|
- spec/spec_helper.rb
|