formulaic 0.3.0 → 0.4.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/.ruby-version +1 -1
- data/.travis.yml +3 -2
- data/README.md +14 -4
- data/lib/formulaic.rb +11 -0
- data/lib/formulaic/dsl.rb +4 -1
- data/lib/formulaic/errors.rb +1 -0
- data/lib/formulaic/form.rb +2 -1
- data/lib/formulaic/inputs/input.rb +1 -1
- data/lib/formulaic/inputs/string_input.rb +24 -5
- data/lib/formulaic/label.rb +1 -1
- data/lib/formulaic/version.rb +1 -1
- data/spec/features/fill_in_user_form_spec.rb +28 -3
- data/spec/fixtures/user_form.html +8 -0
- data/spec/formulaic/dsl_spec.rb +9 -1
- data/spec/formulaic/label_spec.rb +4 -4
- data/spec/spec_helper.rb +20 -3
- data/spec/support/models/user.rb +5 -0
- metadata +5 -3
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 5aac19cb8a6553a97ce1cf6fd5685efe3d9670cd
|
4
|
+
data.tar.gz: 4b2936160b65052f3eaf8f11fed542104ef86c2e
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 8aeb601d65baf8db3eb4a524b64917ca82ffc172aa3827c60c96beb2802974a6907c5569bb07d048af4f7e741f97794d89e07958902a6348c5fb123e280629f2
|
7
|
+
data.tar.gz: 9821a23573b2387dde20940b476ef9e2247177c565aaeacc218deaf4f18058858d237cdc5bdb1aa926122603e9a7876ad2db857cc63804aa8f87f2ba8fa51568
|
data/.ruby-version
CHANGED
@@ -1 +1 @@
|
|
1
|
-
2.
|
1
|
+
2.4.0
|
data/.travis.yml
CHANGED
data/README.md
CHANGED
@@ -122,7 +122,7 @@ You may have attributes included in your `User` factory that don’t pertain to
|
|
122
122
|
sign up:
|
123
123
|
|
124
124
|
```ruby
|
125
|
-
fill_form(:user, attributes_for(:user).slice(sign_up_attributes))
|
125
|
+
fill_form(:user, attributes_for(:user).slice(*sign_up_attributes))
|
126
126
|
|
127
127
|
# ...
|
128
128
|
def sign_up_attributes
|
@@ -142,6 +142,11 @@ it knows to pass directly to `fill_in` rather than trying to find a translation.
|
|
142
142
|
You’ll need to find submit buttons yourself since `submit` is a thin wrapper
|
143
143
|
around `I18n.t`.
|
144
144
|
|
145
|
+
Formulaic assumes your forms don't use AJAX, setting the wait time to 0. This can be configured using:
|
146
|
+
```ruby
|
147
|
+
Formulaic.default_wait_time = 5
|
148
|
+
```
|
149
|
+
|
145
150
|
## Known Limitations
|
146
151
|
|
147
152
|
* Formulaic currently supports the following mappings from the `#class` of the
|
@@ -171,8 +176,13 @@ around `I18n.t`.
|
|
171
176
|
|
172
177
|
## About
|
173
178
|
|
174
|
-
Formulaic is maintained by [Caleb Thompson]
|
175
|
-
|
176
|
-
[contributors]
|
179
|
+
Formulaic is maintained by [Caleb Thompson][caleb]
|
180
|
+
and thoughtbot's [Austin Ruby on Rails development team][team],
|
181
|
+
with the help of community [contributors].
|
182
|
+
Thank you!
|
183
|
+
|
184
|
+
[caleb]: http://github.com/calebthompson
|
185
|
+
[team]: https://thoughtbot.com/austin?utm_source=github
|
186
|
+
[contributors]: http://github.com/thoughtbot/formulaic/contributors
|
177
187
|
|
178
188
|
[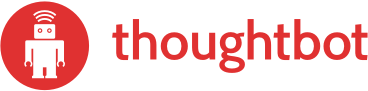](http://thoughtbot.com)
|
data/lib/formulaic.rb
CHANGED
@@ -7,4 +7,15 @@ require 'formulaic/form'
|
|
7
7
|
require 'formulaic/dsl'
|
8
8
|
|
9
9
|
module Formulaic
|
10
|
+
class << self
|
11
|
+
attr_accessor :default_wait_time
|
12
|
+
|
13
|
+
def configure
|
14
|
+
yield self
|
15
|
+
end
|
16
|
+
end
|
17
|
+
end
|
18
|
+
|
19
|
+
Formulaic.configure do |config|
|
20
|
+
config.default_wait_time = 0
|
10
21
|
end
|
data/lib/formulaic/dsl.rb
CHANGED
@@ -16,7 +16,10 @@ module Formulaic
|
|
16
16
|
end
|
17
17
|
|
18
18
|
def submit(model_class, action = :create)
|
19
|
-
I18n.t
|
19
|
+
I18n.t "#{model_class}.#{action}",
|
20
|
+
scope: [:helpers, :submit],
|
21
|
+
model: model_class.to_s.humanize,
|
22
|
+
default: action
|
20
23
|
end
|
21
24
|
end
|
22
25
|
end
|
data/lib/formulaic/errors.rb
CHANGED
data/lib/formulaic/form.rb
CHANGED
@@ -8,7 +8,8 @@ module Formulaic
|
|
8
8
|
DateTime => Formulaic::Inputs::DateTimeInput,
|
9
9
|
Array => Formulaic::Inputs::ArrayInput,
|
10
10
|
String => Formulaic::Inputs::StringInput,
|
11
|
-
|
11
|
+
Symbol => Formulaic::Inputs::StringInput,
|
12
|
+
1.class => Formulaic::Inputs::StringInput,
|
12
13
|
Float => Formulaic::Inputs::StringInput,
|
13
14
|
TrueClass => Formulaic::Inputs::BooleanInput,
|
14
15
|
FalseClass => Formulaic::Inputs::BooleanInput,
|
@@ -2,22 +2,41 @@ module Formulaic
|
|
2
2
|
module Inputs
|
3
3
|
class StringInput < Input
|
4
4
|
def fill
|
5
|
-
if page.has_selector?(:fillable_field, label)
|
5
|
+
if page.has_selector?(:fillable_field, label, wait: Formulaic.default_wait_time)
|
6
6
|
fill_in(label, with: value)
|
7
|
-
elsif page.has_selector?(:radio_button, label)
|
7
|
+
elsif page.has_selector?(:radio_button, label, wait: Formulaic.default_wait_time)
|
8
8
|
choose(value)
|
9
|
-
elsif has_option_in_select?(value, label)
|
10
|
-
select(value, from: label)
|
9
|
+
elsif has_option_in_select?(translate_option(value), label)
|
10
|
+
select(translate_option(value), from: label)
|
11
11
|
else
|
12
12
|
raise Formulaic::InputNotFound.new(%[Unable to find input "#{label}".])
|
13
13
|
end
|
14
14
|
end
|
15
15
|
|
16
16
|
def has_option_in_select?(option, select)
|
17
|
-
find(:select, select)
|
17
|
+
element = find(:select, select)
|
18
|
+
if ! element.has_selector?(:option, option, wait: Formulaic.default_wait_time)
|
19
|
+
raise Formulaic::OptionForSelectInputNotFound.new(%[Unable to find option with text matching "#{option}".])
|
20
|
+
end
|
21
|
+
true
|
18
22
|
rescue Capybara::ElementNotFound
|
19
23
|
false
|
20
24
|
end
|
25
|
+
|
26
|
+
private
|
27
|
+
|
28
|
+
def translate_option(option)
|
29
|
+
I18n.t(lookup_paths_for_option.first,
|
30
|
+
scope: :'simple_form.options', default: lookup_paths_for_option)
|
31
|
+
end
|
32
|
+
|
33
|
+
def lookup_paths_for_option
|
34
|
+
[
|
35
|
+
:"#{label.model_name}.#{label.attribute}.#{value}",
|
36
|
+
:"defaults.#{label.attribute}.#{value}",
|
37
|
+
value.to_s,
|
38
|
+
]
|
39
|
+
end
|
21
40
|
end
|
22
41
|
end
|
23
42
|
end
|
data/lib/formulaic/label.rb
CHANGED
data/lib/formulaic/version.rb
CHANGED
@@ -2,9 +2,6 @@ require 'spec_helper'
|
|
2
2
|
require 'pathname'
|
3
3
|
|
4
4
|
describe 'Fill in user form' do
|
5
|
-
|
6
|
-
before(:all) { load_translations }
|
7
|
-
|
8
5
|
it 'finds and fills text fields' do
|
9
6
|
visit 'user_form'
|
10
7
|
form = Formulaic::Form.new(:user, :new, name: 'George')
|
@@ -14,6 +11,14 @@ describe 'Fill in user form' do
|
|
14
11
|
expect(input(:user, :name).value).to eq 'George'
|
15
12
|
end
|
16
13
|
|
14
|
+
it 'finds and fills text fields with symbol values' do
|
15
|
+
visit 'user_form'
|
16
|
+
form = Formulaic::Form.new(:user, :new, name: :George)
|
17
|
+
form.fill
|
18
|
+
|
19
|
+
expect(input(:user, :name).value).to eq 'George'
|
20
|
+
end
|
21
|
+
|
17
22
|
it 'finds and fills a password field' do
|
18
23
|
visit 'user_form'
|
19
24
|
form = Formulaic::Form.new(:user, :new, password: 'supersecr3t')
|
@@ -224,4 +229,24 @@ describe 'Fill in user form' do
|
|
224
229
|
|
225
230
|
expect(page.find('#user_friend_ids_1')).to be_checked
|
226
231
|
end
|
232
|
+
|
233
|
+
it 'raises an useful error when option not found for select' do
|
234
|
+
visit 'user_form'
|
235
|
+
|
236
|
+
form = Formulaic::Form.new(:user, :new, role: :unknown)
|
237
|
+
|
238
|
+
expect { form.fill }
|
239
|
+
.to raise_error(
|
240
|
+
Formulaic::OptionForSelectInputNotFound,
|
241
|
+
%[Unable to find option with text matching "unknown".])
|
242
|
+
end
|
243
|
+
|
244
|
+
it 'translate option for select when translation is available' do
|
245
|
+
visit 'user_form'
|
246
|
+
|
247
|
+
form = Formulaic::Form.new(:user, :new, role: :admin)
|
248
|
+
form.fill
|
249
|
+
|
250
|
+
expect(page).to have_select('user_role', selected: 'Administrator')
|
251
|
+
end
|
227
252
|
end
|
@@ -302,5 +302,13 @@
|
|
302
302
|
</select>
|
303
303
|
</div>
|
304
304
|
<div class="input boolean required user_terms_of_service"><input name="user[terms_of_service]" type="hidden" value="0"><input class="boolean required" id="user_terms_of_service" name="user[terms_of_service]" type="checkbox" value="1"><label class="boolean required" for="user_terms_of_service"><abbr title="required">*</abbr> I agree to the Terms of Service</label></div>
|
305
|
+
<div class="input select required user_role">
|
306
|
+
<label class="select required" for="user_role"><abbr title="required">*</abbr> Role</label>
|
307
|
+
<select class="select required" name="user[role]" id="user_role">
|
308
|
+
<option value=""></option>
|
309
|
+
<option selected="selected" value="member">Member</option>
|
310
|
+
<option value="admin">Administrator</option>
|
311
|
+
</select>
|
312
|
+
</div>
|
305
313
|
<input name="commit" type="submit" value="Register">
|
306
314
|
</form>
|
data/spec/formulaic/dsl_spec.rb
CHANGED
@@ -49,10 +49,16 @@ describe Formulaic::Dsl do
|
|
49
49
|
end
|
50
50
|
|
51
51
|
describe 'submit' do
|
52
|
-
it 'finds a submit label' do
|
52
|
+
it 'finds a custom submit label' do
|
53
53
|
I18n.backend.store_translations(:en, { helpers: { submit: { user: { create: 'Create user' } } } })
|
54
54
|
|
55
55
|
expect(object_with_dsl.submit(:user)).to eq 'Create user'
|
56
|
+
|
57
|
+
I18n.backend.store_translations(:en, { helpers: { submit: { user: { create: nil } } } })
|
58
|
+
end
|
59
|
+
|
60
|
+
it 'finds the default submit label' do
|
61
|
+
expect(object_with_dsl.submit(:user)).to eq 'Create User'
|
56
62
|
end
|
57
63
|
end
|
58
64
|
|
@@ -67,6 +73,8 @@ describe Formulaic::Dsl do
|
|
67
73
|
expect(Formulaic::Form).to have_received(:new)
|
68
74
|
.with(:model, :new, attributes: :values)
|
69
75
|
expect(object_with_dsl).to have_received(:click_on).with('Create model')
|
76
|
+
|
77
|
+
I18n.backend.store_translations(:en, { helpers: { submit: { model: { create: nil } } } })
|
70
78
|
end
|
71
79
|
end
|
72
80
|
|
@@ -13,6 +13,8 @@ describe Formulaic::Label do
|
|
13
13
|
I18n.backend.store_translations(:en, { simple_form: { labels: { user: { name: "Translated" } } } } )
|
14
14
|
|
15
15
|
expect(label(:user, :name)).to eq("Translated")
|
16
|
+
|
17
|
+
I18n.backend.store_translations(:en, { simple_form: { labels: { user: { name: nil } } } } )
|
16
18
|
end
|
17
19
|
|
18
20
|
it "should leave cases alone" do
|
@@ -23,10 +25,8 @@ describe Formulaic::Label do
|
|
23
25
|
expect(label(:student, "Course selection")).to eq "Course selection"
|
24
26
|
end
|
25
27
|
|
26
|
-
|
27
|
-
|
28
|
-
attribute.to_s.humanize
|
29
|
-
end
|
28
|
+
it "humanizes attribute if no translation is found and human_attribute_name is not available" do
|
29
|
+
expect(label(:student, :course_selection)).to eq "Course selection"
|
30
30
|
end
|
31
31
|
|
32
32
|
def label(model_name, attribute, action = :new)
|
data/spec/spec_helper.rb
CHANGED
@@ -1,5 +1,7 @@
|
|
1
1
|
require 'formulaic'
|
2
2
|
|
3
|
+
Dir[File.expand_path('../support/**/*.rb', __FILE__)].each { |f| require f }
|
4
|
+
|
3
5
|
module SpecHelper
|
4
6
|
def input(model, field)
|
5
7
|
page.find("##{model}_#{field}")
|
@@ -16,8 +18,14 @@ module SpecHelper
|
|
16
18
|
end
|
17
19
|
end
|
18
20
|
|
19
|
-
def
|
21
|
+
def reset_and_load_translations
|
22
|
+
I18n.reload!
|
20
23
|
I18n.backend.store_translations(:en, YAML.load(<<-TRANSLATIONS))
|
24
|
+
helpers:
|
25
|
+
submit:
|
26
|
+
create: 'Create %{model}'
|
27
|
+
update: 'Update %{model}'
|
28
|
+
submit: 'Save %{model}'
|
21
29
|
simple_form:
|
22
30
|
labels:
|
23
31
|
user:
|
@@ -35,6 +43,11 @@ module SpecHelper
|
|
35
43
|
phone: Phone Number
|
36
44
|
terms_of_service: I agree to the Terms of Service
|
37
45
|
url: Website
|
46
|
+
options:
|
47
|
+
user:
|
48
|
+
role:
|
49
|
+
admin: Administrator
|
50
|
+
member: Member
|
38
51
|
TRANSLATIONS
|
39
52
|
I18n.backend.store_translations(:es, YAML.load(<<-TRANSLATIONS))
|
40
53
|
date:
|
@@ -56,6 +69,10 @@ module SpecHelper
|
|
56
69
|
end
|
57
70
|
end
|
58
71
|
|
59
|
-
RSpec.configure do |
|
60
|
-
|
72
|
+
RSpec.configure do |config|
|
73
|
+
config.include SpecHelper
|
74
|
+
|
75
|
+
config.before(:each) do
|
76
|
+
reset_and_load_translations
|
77
|
+
end
|
61
78
|
end
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: formulaic
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.
|
4
|
+
version: 0.4.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Caleb Thompson
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date:
|
11
|
+
date: 2017-02-06 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: capybara
|
@@ -154,6 +154,7 @@ files:
|
|
154
154
|
- spec/formulaic/form_spec.rb
|
155
155
|
- spec/formulaic/label_spec.rb
|
156
156
|
- spec/spec_helper.rb
|
157
|
+
- spec/support/models/user.rb
|
157
158
|
homepage: https://github.com/thoughtbot/formulaic
|
158
159
|
licenses:
|
159
160
|
- MIT
|
@@ -174,7 +175,7 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
174
175
|
version: '0'
|
175
176
|
requirements: []
|
176
177
|
rubyforge_project:
|
177
|
-
rubygems_version: 2.
|
178
|
+
rubygems_version: 2.6.8
|
178
179
|
signing_key:
|
179
180
|
specification_version: 4
|
180
181
|
summary: Simplify form filling with Capybara
|
@@ -188,3 +189,4 @@ test_files:
|
|
188
189
|
- spec/formulaic/form_spec.rb
|
189
190
|
- spec/formulaic/label_spec.rb
|
190
191
|
- spec/spec_helper.rb
|
192
|
+
- spec/support/models/user.rb
|