feature_flags 0.0.1
Sign up to get free protection for your applications and to get access to all the features.
- data/.gitignore +17 -0
- data/Gemfile +4 -0
- data/LICENSE.txt +22 -0
- data/README.md +77 -0
- data/Rakefile +1 -0
- data/app/controllers/feature_flags_controller.rb +85 -0
- data/app/views/feature_flags/edit.html.erb +32 -0
- data/app/views/feature_flags/index.html.erb +52 -0
- data/app/views/feature_flags/new.html.erb +32 -0
- data/app/views/feature_flags/update.html.erb +0 -0
- data/feature_flags.gemspec +22 -0
- data/index.png +0 -0
- data/lib/feature_flags.rb +35 -0
- data/lib/feature_flags/configuration.rb +20 -0
- data/lib/feature_flags/engine.rb +5 -0
- data/lib/feature_flags/version.rb +3 -0
- data/lib/generators/feature_flags/install_generator.rb +40 -0
- data/lib/generators/feature_flags/templates/feature_flag.rb +5 -0
- data/lib/generators/feature_flags/templates/feature_flag_migrate.rb +13 -0
- data/lib/generators/feature_flags/templates/feature_flag_model.rb +7 -0
- data/new.png +0 -0
- metadata +99 -0
data/.gitignore
ADDED
data/Gemfile
ADDED
data/LICENSE.txt
ADDED
@@ -0,0 +1,22 @@
|
|
1
|
+
Copyright (c) 2013 pandurang
|
2
|
+
|
3
|
+
MIT License
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
6
|
+
a copy of this software and associated documentation files (the
|
7
|
+
"Software"), to deal in the Software without restriction, including
|
8
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
9
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
10
|
+
permit persons to whom the Software is furnished to do so, subject to
|
11
|
+
the following conditions:
|
12
|
+
|
13
|
+
The above copyright notice and this permission notice shall be
|
14
|
+
included in all copies or substantial portions of the Software.
|
15
|
+
|
16
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
17
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
18
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
19
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
20
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
21
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
22
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,77 @@
|
|
1
|
+
# FeatureFlags
|
2
|
+
|
3
|
+
Manage (turn on/off) different features in your rails app.
|
4
|
+
|
5
|
+
## Installation
|
6
|
+
|
7
|
+
Add this line to your application's Gemfile:
|
8
|
+
|
9
|
+
gem 'feature_flags'
|
10
|
+
|
11
|
+
And then execute:
|
12
|
+
|
13
|
+
$ bundle
|
14
|
+
|
15
|
+
Or install it yourself as:
|
16
|
+
|
17
|
+
$ gem install feature_flags
|
18
|
+
|
19
|
+
## Usage
|
20
|
+
|
21
|
+
with feature_flags gem you can easily manage different features in your rails application.You can turn on/off features.
|
22
|
+
|
23
|
+
|
24
|
+
rails generate feature_flags:install
|
25
|
+
|
26
|
+
this will generate 3 files,
|
27
|
+
1) initializer file in config/initializer/feature_flags.rb
|
28
|
+
2) migration file for Feature model
|
29
|
+
3) Feature.rb
|
30
|
+
then do
|
31
|
+
|
32
|
+
rake db:migrate
|
33
|
+
|
34
|
+
##############################################################################
|
35
|
+
|
36
|
+
To check whether paritcular feature is enabled or not use
|
37
|
+
|
38
|
+
FeatureFlags.enabled?(:feature_name)
|
39
|
+
|
40
|
+
this will check whether feature is enabled or not, and if its not present/created ,it will create that feature and set it to enabled by default.
|
41
|
+
|
42
|
+
##############################################################################
|
43
|
+
|
44
|
+
FeatureFlags.enable_all To enable all features in your app.
|
45
|
+
|
46
|
+
FeatureFlags.disable_all To disable all features in your app.
|
47
|
+
|
48
|
+
FeatureFlags.set_disabled(:feature_name) To disable feature in your app.
|
49
|
+
|
50
|
+
|
51
|
+
##############################################################################
|
52
|
+
|
53
|
+
In feature_flags.rb initializer file you can mention which layout to use for view
|
54
|
+
|
55
|
+
FeatureFlags.configure do |config|
|
56
|
+
config.layout = "application"
|
57
|
+
end
|
58
|
+
|
59
|
+
##############################################################################
|
60
|
+
|
61
|
+
|
62
|
+
Here are some screenshots,
|
63
|
+
main index view
|
64
|
+
|
65
|
+
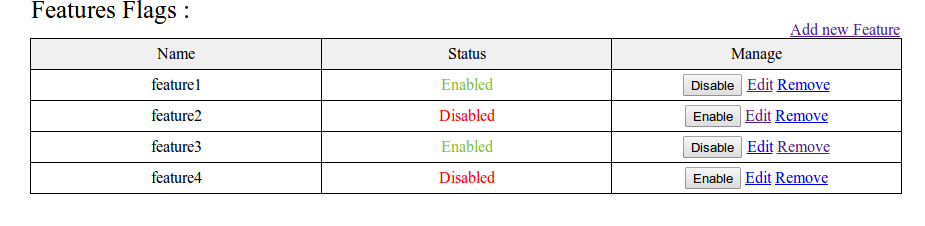
|
66
|
+
|
67
|
+
adding new feature to rails application
|
68
|
+
|
69
|
+
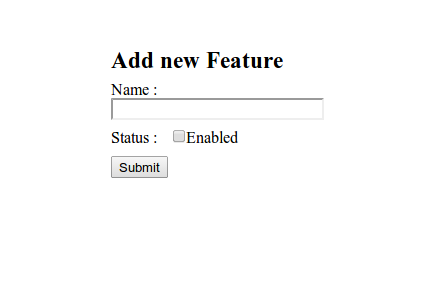
|
70
|
+
|
71
|
+
## Contributing
|
72
|
+
|
73
|
+
1. Fork it
|
74
|
+
2. Create your feature branch (`git checkout -b my-new-feature`)
|
75
|
+
3. Commit your changes (`git commit -am 'Add some feature'`)
|
76
|
+
4. Push to the branch (`git push origin my-new-feature`)
|
77
|
+
5. Create new Pull Request
|
data/Rakefile
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
require "bundler/gem_tasks"
|
@@ -0,0 +1,85 @@
|
|
1
|
+
class FeatureFlagsController < ApplicationController
|
2
|
+
layout FeatureFlags.configuration.layout.downcase
|
3
|
+
|
4
|
+
def index
|
5
|
+
@features = Feature.all
|
6
|
+
end
|
7
|
+
|
8
|
+
def new
|
9
|
+
@feature = Feature.new
|
10
|
+
end
|
11
|
+
|
12
|
+
def edit
|
13
|
+
@feature = Feature.find(params[:id])
|
14
|
+
end
|
15
|
+
|
16
|
+
def create
|
17
|
+
@features = Feature.all
|
18
|
+
@feature = Feature.new(params[:feature])
|
19
|
+
respond_to do |format|
|
20
|
+
if @feature.save
|
21
|
+
flash[:notice] = "#{@feature.name} feature successfully created"
|
22
|
+
format.html{
|
23
|
+
redirect_to feature_flags_url
|
24
|
+
}
|
25
|
+
else
|
26
|
+
flash[:error] = "#{@feature.name} feature could not be created"
|
27
|
+
format.html{
|
28
|
+
render :new
|
29
|
+
}
|
30
|
+
end
|
31
|
+
end
|
32
|
+
end
|
33
|
+
|
34
|
+
def update
|
35
|
+
@features = Feature.all
|
36
|
+
feature = Feature.find(params[:id])
|
37
|
+
|
38
|
+
respond_to do |format|
|
39
|
+
if feature.update_attributes(params[:feature])
|
40
|
+
flash[:notice] = "#{feature.name} feature successfully updated"
|
41
|
+
format.html{
|
42
|
+
redirect_to feature_flags_url
|
43
|
+
}
|
44
|
+
|
45
|
+
format.js{
|
46
|
+
render :json => {:status => true, :message => flash[:notice]}
|
47
|
+
}
|
48
|
+
else
|
49
|
+
flash[:error] = "#{feature.name} feature could not be updated"
|
50
|
+
format.html{
|
51
|
+
redirect_to feature_flags_url
|
52
|
+
}
|
53
|
+
format.js{
|
54
|
+
render :json => {:status => false, :message => flash[:error]}
|
55
|
+
}
|
56
|
+
end
|
57
|
+
end
|
58
|
+
end
|
59
|
+
|
60
|
+
def destroy
|
61
|
+
@features = Feature.all
|
62
|
+
feature = Feature.find(params[:id])
|
63
|
+
|
64
|
+
respond_to do |format|
|
65
|
+
if feature.destroy
|
66
|
+
flash[:notice] = "Feature successfully removed"
|
67
|
+
format.html{
|
68
|
+
redirect_to feature_flags_url
|
69
|
+
}
|
70
|
+
|
71
|
+
format.js{
|
72
|
+
render :json => {:status => true, :message => flash[:notice]}
|
73
|
+
}
|
74
|
+
else
|
75
|
+
flash[:error] = "This feature could not be removed"
|
76
|
+
format.html{
|
77
|
+
redirect_to feature_flags_url
|
78
|
+
}
|
79
|
+
format.js{
|
80
|
+
render :json => {:status => false, :message => flash[:error]}
|
81
|
+
}
|
82
|
+
end
|
83
|
+
end
|
84
|
+
end
|
85
|
+
end
|
@@ -0,0 +1,32 @@
|
|
1
|
+
<style>
|
2
|
+
.field{margin-top: 10px;}
|
3
|
+
.feature-div{padding-top: 100px;padding-left: 30%;padding-bottom: 100px;}
|
4
|
+
.errors{color: red;}
|
5
|
+
</style>
|
6
|
+
|
7
|
+
|
8
|
+
<div class="feature-div">
|
9
|
+
<p style="font-weight:bold;font-size:23px">Update Feature</p>
|
10
|
+
<%= form_for @feature, :url => feature_flag_path(@feature), :method => :put, :html => {:class => "feature-flag-form"} do |f| %>
|
11
|
+
<div class ="field">
|
12
|
+
<label>Name : </label> <br/>
|
13
|
+
<%= f.text_field :name %>
|
14
|
+
<div class ="errors">
|
15
|
+
<% if @feature.errors.any? %>
|
16
|
+
<% @feature.errors.full_messages.each do |error| %>
|
17
|
+
<%= error %>
|
18
|
+
<% end %>
|
19
|
+
<% end %>
|
20
|
+
</div>
|
21
|
+
|
22
|
+
</div>
|
23
|
+
<div class ="field">
|
24
|
+
<label>Status : </label>
|
25
|
+
<%= f.check_box :status %><label>Enabled </label> <br/>
|
26
|
+
</div>
|
27
|
+
<div class ="field">
|
28
|
+
<%= f.submit "Submit" %>
|
29
|
+
</div>
|
30
|
+
<% end %>
|
31
|
+
|
32
|
+
</div>
|
@@ -0,0 +1,52 @@
|
|
1
|
+
<style type="text/css">
|
2
|
+
th.bordered,td.bordered{height: 30px;border: 1px solid;text-align: center;vertical-align: middle;border-color: black;}
|
3
|
+
table#features tr th{background-color: #f0f0f0;width: 30%;}
|
4
|
+
#features{width: 90%;}
|
5
|
+
.page-header h2{font-size: 25px;margin-left: 50px;}
|
6
|
+
</style>
|
7
|
+
|
8
|
+
|
9
|
+
<div class="feature_flag">
|
10
|
+
|
11
|
+
<div class="page-header">
|
12
|
+
<h2>Features Flags :</h2>
|
13
|
+
</div>
|
14
|
+
|
15
|
+
</div>
|
16
|
+
<%= link_to "Add new Feature", new_feature_flag_path ,:style=>"float:right;margin-right: 50px;" %>
|
17
|
+
|
18
|
+
<div class="feature_flag">
|
19
|
+
<center>
|
20
|
+
<table id="features">
|
21
|
+
<thead>
|
22
|
+
<tr>
|
23
|
+
<th colspan=4 class="bordered headerTop">Name</th>
|
24
|
+
<th colspan=2 class="bordered headerTop">Status</th>
|
25
|
+
<th colspan=4 class="bordered headerTop">Manage</th>
|
26
|
+
</tr>
|
27
|
+
</thead>
|
28
|
+
<%if @features.any?%>
|
29
|
+
<tbody>
|
30
|
+
<% @features.each do |feature| %>
|
31
|
+
<tr>
|
32
|
+
<td colspan=4 class="bordered"> <%= feature.name %> </td>
|
33
|
+
<td colspan=2 class="bordered" style="color:<%= feature.status? ? '#84be40' : 'red' %>"><%= feature.status? ? "Enabled" : "Disabled" %>
|
34
|
+
<td colspan=4 class="bordered">
|
35
|
+
|
36
|
+
<%= form_for feature, :url => feature_flag_url(feature), :method => :put, :html => {:class => "feature-flag-form",:style => "display: inline-block"} do |f| %>
|
37
|
+
<%= f.hidden_field :status,:value=> !feature.status %>
|
38
|
+
<%= f.submit (feature.status? ? "Disable" : "Enable")%>
|
39
|
+
<% end %>
|
40
|
+
<%= link_to 'Edit', edit_feature_flag_path(feature)%>
|
41
|
+
|
42
|
+
<%= link_to 'Remove', feature_flag_path(feature), :method => :delete, :confirm => 'are you sure?' %>
|
43
|
+
|
44
|
+
</td>
|
45
|
+
</tr>
|
46
|
+
<% end %>
|
47
|
+
</tbody>
|
48
|
+
<% end %>
|
49
|
+
</table>
|
50
|
+
</center>
|
51
|
+
</div>
|
52
|
+
|
@@ -0,0 +1,32 @@
|
|
1
|
+
<style>
|
2
|
+
.field{margin-top: 10px;}
|
3
|
+
.feature-div{padding-top: 100px;padding-left: 30%;padding-bottom: 100px;}
|
4
|
+
.errors{color: red;}
|
5
|
+
</style>
|
6
|
+
|
7
|
+
|
8
|
+
<div class="feature-div">
|
9
|
+
<p style="font-weight:bold;font-size:23px">Add new Feature</p>
|
10
|
+
<%= form_for @feature, :url => feature_flags_path, :method => :post, :html => {:class => "feature-flag-form"} do |f| %>
|
11
|
+
<div class ="field">
|
12
|
+
<label>Name : </label> <br/>
|
13
|
+
<%= f.text_field :name %>
|
14
|
+
<div class ="errors">
|
15
|
+
<% if @feature.errors.any? %>
|
16
|
+
<% @feature.errors.full_messages.each do |error| %>
|
17
|
+
<%= error %>
|
18
|
+
<% end %>
|
19
|
+
<% end %>
|
20
|
+
</div>
|
21
|
+
|
22
|
+
</div>
|
23
|
+
<div class ="field">
|
24
|
+
<label>Status : </label>
|
25
|
+
<%= f.check_box :status %><label>Enabled </label> <br/>
|
26
|
+
</div>
|
27
|
+
<div class ="field">
|
28
|
+
<%= f.submit "Submit" %>
|
29
|
+
</div>
|
30
|
+
<% end %>
|
31
|
+
|
32
|
+
</div>
|
File without changes
|
@@ -0,0 +1,22 @@
|
|
1
|
+
# -*- encoding: utf-8 -*-
|
2
|
+
lib = File.expand_path('../lib', __FILE__)
|
3
|
+
$LOAD_PATH.unshift(lib) unless $LOAD_PATH.include?(lib)
|
4
|
+
require 'feature_flags/version'
|
5
|
+
|
6
|
+
Gem::Specification.new do |gem|
|
7
|
+
gem.name = "feature_flags"
|
8
|
+
gem.version = FeatureFlags::VERSION
|
9
|
+
gem.authors = ["pandurang"]
|
10
|
+
gem.email = ["pandurang.plw@gmail.com"]
|
11
|
+
gem.description = "Manage (turn on/off) different features in your rails app."
|
12
|
+
gem.summary = "Manage (turn on/off) different features in your rails app."
|
13
|
+
gem.homepage = "https://github.com/pandurang90/feature_flags"
|
14
|
+
|
15
|
+
gem.files = `git ls-files`.split("\n")
|
16
|
+
gem.executables = gem.files.grep(%r{^bin/}).map{ |f| File.basename(f) }
|
17
|
+
gem.test_files = gem.files.grep(%r{^(test|spec|features)/})
|
18
|
+
gem.require_paths = ["lib"]
|
19
|
+
|
20
|
+
gem.add_dependency "activesupport"
|
21
|
+
gem.add_dependency "rails"
|
22
|
+
end
|
data/index.png
ADDED
Binary file
|
@@ -0,0 +1,35 @@
|
|
1
|
+
|
2
|
+
require "feature_flags/version"
|
3
|
+
require "feature_flags/configuration"
|
4
|
+
require "feature_flags/engine"
|
5
|
+
require "active_support/dependencies"
|
6
|
+
|
7
|
+
module FeatureFlags
|
8
|
+
# Your code goes here...\
|
9
|
+
|
10
|
+
def self.enabled?(feature_name)
|
11
|
+
feature = Feature.where(:name => feature_name).first
|
12
|
+
|
13
|
+
if feature.present?
|
14
|
+
feature.status? ? true : false
|
15
|
+
elsif
|
16
|
+
Feature.create!(:name => feature_name, :status => true)
|
17
|
+
return true
|
18
|
+
end
|
19
|
+
|
20
|
+
end
|
21
|
+
|
22
|
+
def self.set_disabled(feature_name)
|
23
|
+
feature = Feature.where(:name => feature_name).first
|
24
|
+
(feature.present? && feature.update_attributes(:status => false)) ? true : false
|
25
|
+
end
|
26
|
+
|
27
|
+
def self.disable_all
|
28
|
+
Feature.update_all(:status => false)
|
29
|
+
end
|
30
|
+
|
31
|
+
def self.enable_all
|
32
|
+
Feature.update_all(:status => true)
|
33
|
+
end
|
34
|
+
|
35
|
+
end
|
@@ -0,0 +1,20 @@
|
|
1
|
+
module FeatureFlags
|
2
|
+
class Configuration
|
3
|
+
attr_accessor :layout, :filters
|
4
|
+
|
5
|
+
def initialize
|
6
|
+
layout = "application"
|
7
|
+
filters = []
|
8
|
+
end
|
9
|
+
|
10
|
+
end
|
11
|
+
|
12
|
+
class << self
|
13
|
+
attr_accessor :configuration
|
14
|
+
end
|
15
|
+
|
16
|
+
def self.configure
|
17
|
+
self.configuration ||= Configuration.new
|
18
|
+
yield(configuration) if block_given?
|
19
|
+
end
|
20
|
+
end
|
@@ -0,0 +1,40 @@
|
|
1
|
+
|
2
|
+
module FeatureFlags
|
3
|
+
module Generators
|
4
|
+
|
5
|
+
class MigrationNumber
|
6
|
+
def self.next_migration_number
|
7
|
+
unless @prev_migration_nr
|
8
|
+
@prev_migration_nr = Time.now.utc.strftime("%Y%m%d%H%M%S").to_i
|
9
|
+
else
|
10
|
+
@prev_migration_nr += 1
|
11
|
+
end
|
12
|
+
@prev_migration_nr.to_s
|
13
|
+
end
|
14
|
+
|
15
|
+
end
|
16
|
+
|
17
|
+
class InstallGenerator < Rails::Generators::Base
|
18
|
+
|
19
|
+
source_root File.expand_path("../templates", __FILE__)
|
20
|
+
desc "Creates feature_flags initializer, routes and copy locale
|
21
|
+
files to your application."
|
22
|
+
|
23
|
+
def create_initializer_file
|
24
|
+
template "feature_flag.rb", "config/initializers/feature_flags.rb"
|
25
|
+
#say("added initializer file", :green)
|
26
|
+
end
|
27
|
+
|
28
|
+
def setup_routes
|
29
|
+
route "resources :feature_flags"
|
30
|
+
end
|
31
|
+
|
32
|
+
def copy_feature_migration
|
33
|
+
template "feature_flag_migrate.rb", "db/migrate/#{MigrationNumber.next_migration_number}_create_features.rb"
|
34
|
+
template "feature_flag_model.rb", "app/models/feature.rb"
|
35
|
+
end
|
36
|
+
|
37
|
+
end
|
38
|
+
end
|
39
|
+
|
40
|
+
end
|
data/new.png
ADDED
Binary file
|
metadata
ADDED
@@ -0,0 +1,99 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: feature_flags
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.1
|
5
|
+
prerelease:
|
6
|
+
platform: ruby
|
7
|
+
authors:
|
8
|
+
- pandurang
|
9
|
+
autorequire:
|
10
|
+
bindir: bin
|
11
|
+
cert_chain: []
|
12
|
+
date: 2013-08-27 00:00:00.000000000 Z
|
13
|
+
dependencies:
|
14
|
+
- !ruby/object:Gem::Dependency
|
15
|
+
name: activesupport
|
16
|
+
requirement: !ruby/object:Gem::Requirement
|
17
|
+
none: false
|
18
|
+
requirements:
|
19
|
+
- - ! '>='
|
20
|
+
- !ruby/object:Gem::Version
|
21
|
+
version: '0'
|
22
|
+
type: :runtime
|
23
|
+
prerelease: false
|
24
|
+
version_requirements: !ruby/object:Gem::Requirement
|
25
|
+
none: false
|
26
|
+
requirements:
|
27
|
+
- - ! '>='
|
28
|
+
- !ruby/object:Gem::Version
|
29
|
+
version: '0'
|
30
|
+
- !ruby/object:Gem::Dependency
|
31
|
+
name: rails
|
32
|
+
requirement: !ruby/object:Gem::Requirement
|
33
|
+
none: false
|
34
|
+
requirements:
|
35
|
+
- - ! '>='
|
36
|
+
- !ruby/object:Gem::Version
|
37
|
+
version: '0'
|
38
|
+
type: :runtime
|
39
|
+
prerelease: false
|
40
|
+
version_requirements: !ruby/object:Gem::Requirement
|
41
|
+
none: false
|
42
|
+
requirements:
|
43
|
+
- - ! '>='
|
44
|
+
- !ruby/object:Gem::Version
|
45
|
+
version: '0'
|
46
|
+
description: Manage (turn on/off) different features in your rails app.
|
47
|
+
email:
|
48
|
+
- pandurang.plw@gmail.com
|
49
|
+
executables: []
|
50
|
+
extensions: []
|
51
|
+
extra_rdoc_files: []
|
52
|
+
files:
|
53
|
+
- .gitignore
|
54
|
+
- Gemfile
|
55
|
+
- LICENSE.txt
|
56
|
+
- README.md
|
57
|
+
- Rakefile
|
58
|
+
- app/controllers/feature_flags_controller.rb
|
59
|
+
- app/views/feature_flags/edit.html.erb
|
60
|
+
- app/views/feature_flags/index.html.erb
|
61
|
+
- app/views/feature_flags/new.html.erb
|
62
|
+
- app/views/feature_flags/update.html.erb
|
63
|
+
- feature_flags.gemspec
|
64
|
+
- index.png
|
65
|
+
- lib/feature_flags.rb
|
66
|
+
- lib/feature_flags/configuration.rb
|
67
|
+
- lib/feature_flags/engine.rb
|
68
|
+
- lib/feature_flags/version.rb
|
69
|
+
- lib/generators/feature_flags/install_generator.rb
|
70
|
+
- lib/generators/feature_flags/templates/feature_flag.rb
|
71
|
+
- lib/generators/feature_flags/templates/feature_flag_migrate.rb
|
72
|
+
- lib/generators/feature_flags/templates/feature_flag_model.rb
|
73
|
+
- new.png
|
74
|
+
homepage: https://github.com/pandurang90/feature_flags
|
75
|
+
licenses: []
|
76
|
+
post_install_message:
|
77
|
+
rdoc_options: []
|
78
|
+
require_paths:
|
79
|
+
- lib
|
80
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
81
|
+
none: false
|
82
|
+
requirements:
|
83
|
+
- - ! '>='
|
84
|
+
- !ruby/object:Gem::Version
|
85
|
+
version: '0'
|
86
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
87
|
+
none: false
|
88
|
+
requirements:
|
89
|
+
- - ! '>='
|
90
|
+
- !ruby/object:Gem::Version
|
91
|
+
version: '0'
|
92
|
+
requirements: []
|
93
|
+
rubyforge_project:
|
94
|
+
rubygems_version: 1.8.18
|
95
|
+
signing_key:
|
96
|
+
specification_version: 3
|
97
|
+
summary: Manage (turn on/off) different features in your rails app.
|
98
|
+
test_files: []
|
99
|
+
has_rdoc:
|