factory_girl_web 0.0.3
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/MIT-LICENSE +20 -0
- data/README.md +35 -0
- data/Rakefile +24 -0
- data/app/controllers/factory_girl_web/application_controller.rb +5 -0
- data/app/controllers/factory_girl_web/fixtures_controller.rb +25 -0
- data/app/helpers/factory_girl_web/application_helper.rb +4 -0
- data/app/models/factory_girl_web/fixture.rb +51 -0
- data/app/views/factory_girl_web/fixtures/index.html.slim +16 -0
- data/app/views/layouts/factory_girl_web/application.html.slim +36 -0
- data/app/views/shared/factory_girl_web/_footer.html.slim +4 -0
- data/app/views/shared/factory_girl_web/_header.html.slim +4 -0
- data/config/routes.rb +4 -0
- data/lib/factory_girl_web.rb +6 -0
- data/lib/factory_girl_web/engine.rb +9 -0
- data/lib/factory_girl_web/version.rb +3 -0
- metadata +101 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: 2780d583007ee4c5b842fb8b2f0568fcc7bffe7b
|
4
|
+
data.tar.gz: fbff6d19661a88533ac9f7ee2667fd0944d38d6d
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 16ec7608aaa9e829c735ffa09a1fd61b4814ab622ea9641656b655d8051dd2634734a96f4fadc3069fc6f1470e8f85c6b0ff8a18bb4a8b44db5fc2bb6db83fc6
|
7
|
+
data.tar.gz: 62376102a16bf44b1d1edd862aaf7ee1d06c898bc411934ffb295f791fb25e3abde5aecc860244e8875d1972f22cc5882775e9cf16cf209c7406cbcb3188d962
|
data/MIT-LICENSE
ADDED
@@ -0,0 +1,20 @@
|
|
1
|
+
Copyright 2016 Yoshiyuki Hirano
|
2
|
+
|
3
|
+
Permission is hereby granted, free of charge, to any person obtaining
|
4
|
+
a copy of this software and associated documentation files (the
|
5
|
+
"Software"), to deal in the Software without restriction, including
|
6
|
+
without limitation the rights to use, copy, modify, merge, publish,
|
7
|
+
distribute, sublicense, and/or sell copies of the Software, and to
|
8
|
+
permit persons to whom the Software is furnished to do so, subject to
|
9
|
+
the following conditions:
|
10
|
+
|
11
|
+
The above copyright notice and this permission notice shall be
|
12
|
+
included in all copies or substantial portions of the Software.
|
13
|
+
|
14
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND,
|
15
|
+
EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF
|
16
|
+
MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND
|
17
|
+
NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE
|
18
|
+
LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION
|
19
|
+
OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION
|
20
|
+
WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,35 @@
|
|
1
|
+
# factory_girl_web
|
2
|
+
|
3
|
+
A web interface for factory_girl
|
4
|
+
|
5
|
+
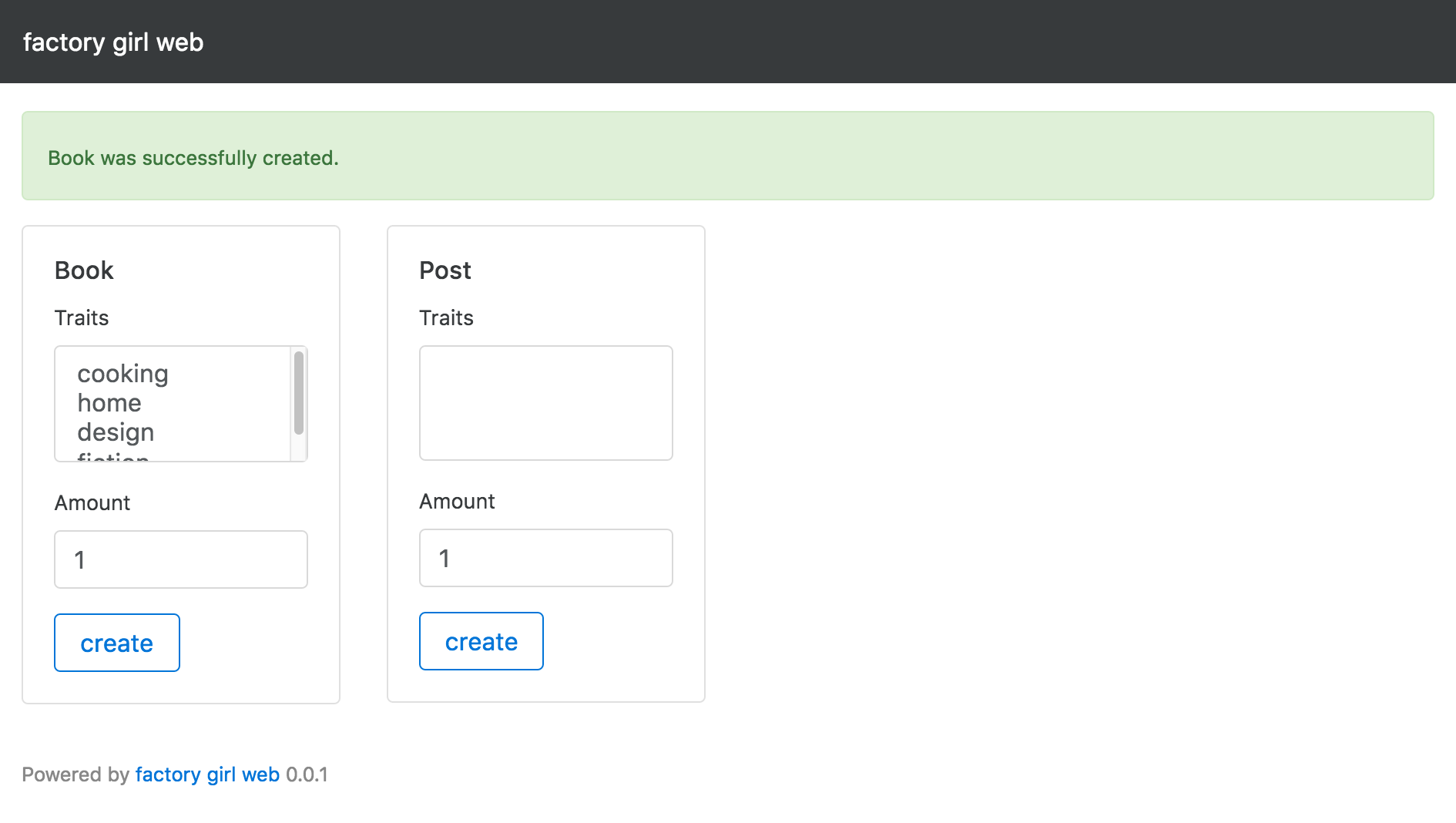
|
6
|
+
|
7
|
+
## Installation
|
8
|
+
|
9
|
+
First add the gem to your development environment and run the `bundle` command to install it.
|
10
|
+
|
11
|
+
```ruby
|
12
|
+
gem 'factory_girl_web', group: :development
|
13
|
+
```
|
14
|
+
|
15
|
+
## Usage
|
16
|
+
|
17
|
+
Add to your routes.rb:
|
18
|
+
|
19
|
+
```ruby
|
20
|
+
Your::App.routes.draw do
|
21
|
+
if Rails.env.development?
|
22
|
+
mount FactoryGirlWeb::Engine, at: '/factory_girl_web'
|
23
|
+
end
|
24
|
+
end
|
25
|
+
```
|
26
|
+
|
27
|
+
Then visit `http://localhost:3000/factory_girl_web`, you can create defined factories through web interface.
|
28
|
+
|
29
|
+
## Contributing
|
30
|
+
|
31
|
+
1. Fork it
|
32
|
+
2. Create your feature branch (`git checkout -b my-new-feature`)
|
33
|
+
3. Commit your changes (`git commit -am 'Add some feature'`)
|
34
|
+
4. Push to the branch (`git push origin my-new-feature`)
|
35
|
+
5. Create new Pull Request
|
data/Rakefile
ADDED
@@ -0,0 +1,24 @@
|
|
1
|
+
begin
|
2
|
+
require 'bundler/setup'
|
3
|
+
rescue LoadError
|
4
|
+
puts 'You must `gem install bundler` and `bundle install` to run rake tasks'
|
5
|
+
end
|
6
|
+
|
7
|
+
require 'rdoc/task'
|
8
|
+
|
9
|
+
RDoc::Task.new(:rdoc) do |rdoc|
|
10
|
+
rdoc.rdoc_dir = 'rdoc'
|
11
|
+
rdoc.title = 'FactoryGirlWeb'
|
12
|
+
rdoc.options << '--line-numbers'
|
13
|
+
rdoc.rdoc_files.include('README.rdoc')
|
14
|
+
rdoc.rdoc_files.include('lib/**/*.rb')
|
15
|
+
end
|
16
|
+
|
17
|
+
|
18
|
+
|
19
|
+
load 'rails/tasks/statistics.rake'
|
20
|
+
|
21
|
+
|
22
|
+
|
23
|
+
Bundler::GemHelper.install_tasks
|
24
|
+
|
@@ -0,0 +1,25 @@
|
|
1
|
+
require_dependency "factory_girl_web/application_controller"
|
2
|
+
|
3
|
+
module FactoryGirlWeb
|
4
|
+
class FixturesController < ApplicationController
|
5
|
+
def index
|
6
|
+
@fixtures = Fixture.all
|
7
|
+
end
|
8
|
+
|
9
|
+
def create
|
10
|
+
fixture = Fixture.new(permitted_params)
|
11
|
+
|
12
|
+
if fixture.create
|
13
|
+
redirect_to :back, notice: format("%{model} was successfully created.", model: fixture.name_i18n)
|
14
|
+
else
|
15
|
+
redirect_to :back, alert: fixture.errors.full_messages.to_sentence
|
16
|
+
end
|
17
|
+
end
|
18
|
+
|
19
|
+
private
|
20
|
+
|
21
|
+
def permitted_params
|
22
|
+
params.require(:fixture).permit(:name, :traits, :amount)
|
23
|
+
end
|
24
|
+
end
|
25
|
+
end
|
@@ -0,0 +1,51 @@
|
|
1
|
+
module FactoryGirlWeb
|
2
|
+
class Fixture
|
3
|
+
include ActiveModel::Model
|
4
|
+
|
5
|
+
attr_accessor :name, :traits, :amount
|
6
|
+
|
7
|
+
delegate :factories, to: :class
|
8
|
+
|
9
|
+
with_options presence: true do
|
10
|
+
validates :name
|
11
|
+
validates :amount, numericality: { only_integer: true, greater_than: 0 }
|
12
|
+
end
|
13
|
+
|
14
|
+
def create
|
15
|
+
return unless valid?
|
16
|
+
|
17
|
+
ActiveRecord::Base.transaction do
|
18
|
+
FactoryGirl.create_list(name.to_sym, amount.to_i, *formatted_traits)
|
19
|
+
end
|
20
|
+
end
|
21
|
+
|
22
|
+
def name_i18n
|
23
|
+
name.to_s.classify.safe_constantize.try(:model_name).try(:human) || name
|
24
|
+
end
|
25
|
+
|
26
|
+
def defined_traits
|
27
|
+
factories[name] || []
|
28
|
+
end
|
29
|
+
|
30
|
+
private
|
31
|
+
|
32
|
+
def formatted_traits
|
33
|
+
return [] if traits.blank?
|
34
|
+
traits.map(&:to_sym)
|
35
|
+
end
|
36
|
+
|
37
|
+
class << self
|
38
|
+
def all
|
39
|
+
factories.keys.map { |name| new(name: name, amount: 1) }
|
40
|
+
end
|
41
|
+
|
42
|
+
def factories
|
43
|
+
@factories ||= begin
|
44
|
+
FactoryGirl.factories.sort_by(&:name).each_with_object(HashWithIndifferentAccess.new) do |factory, h|
|
45
|
+
h[factory.name] = factory.defined_traits.map(&:name)
|
46
|
+
end
|
47
|
+
end
|
48
|
+
end
|
49
|
+
end
|
50
|
+
end
|
51
|
+
end
|
@@ -0,0 +1,16 @@
|
|
1
|
+
- @fixtures.each_slice(4) do |fixtures|
|
2
|
+
.row
|
3
|
+
- fixtures.each do |fixture|
|
4
|
+
.col-md-3
|
5
|
+
.card
|
6
|
+
.card-block
|
7
|
+
h6.card-title = fixture.name_i18n
|
8
|
+
= form_for fixture, url: fixtures_path do |f|
|
9
|
+
= f.hidden_field :name
|
10
|
+
.form-group
|
11
|
+
= f.label :traits
|
12
|
+
= f.select :traits, fixture.defined_traits, {}, multiple: true, size: 3, include_blank: false, class: "form-control"
|
13
|
+
.form-group
|
14
|
+
= f.label :amount
|
15
|
+
= f.number_field :amount, min: 1, required: true, class: "form-control"
|
16
|
+
= f.button :create, class: "btn btn-outline-primary"
|
@@ -0,0 +1,36 @@
|
|
1
|
+
doctype html
|
2
|
+
html
|
3
|
+
head
|
4
|
+
title factory girl web
|
5
|
+
meta name="viewport" content="width=device-width,initial-scale=1"
|
6
|
+
= csrf_meta_tags
|
7
|
+
= stylesheet_link_tag "//maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.4/css/bootstrap.min.css"
|
8
|
+
css:
|
9
|
+
#main {
|
10
|
+
margin-top: 4.5rem;
|
11
|
+
}
|
12
|
+
.card {
|
13
|
+
font-size: 85%;
|
14
|
+
overflow: hidden;
|
15
|
+
}
|
16
|
+
select.form-control {
|
17
|
+
overflow: auto;
|
18
|
+
}
|
19
|
+
footer {
|
20
|
+
padding: 1.5rem 0;
|
21
|
+
font-size: 80%;
|
22
|
+
color: #888;
|
23
|
+
}
|
24
|
+
body
|
25
|
+
= render partial: "shared/factory_girl_web/header"
|
26
|
+
|
27
|
+
.container-fluid#main
|
28
|
+
- if notice
|
29
|
+
.alert.alert-success role="alert"
|
30
|
+
small = notice
|
31
|
+
- if alert
|
32
|
+
.alert.alert-warning role="alert"
|
33
|
+
small = alert
|
34
|
+
= yield
|
35
|
+
|
36
|
+
= render partial: "shared/factory_girl_web/footer"
|
data/config/routes.rb
ADDED
metadata
ADDED
@@ -0,0 +1,101 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: factory_girl_web
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.0.3
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Yoshiyuki Hirano
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2016-09-18 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: rails
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - ">="
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '4.2'
|
20
|
+
type: :runtime
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - ">="
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '4.2'
|
27
|
+
- !ruby/object:Gem::Dependency
|
28
|
+
name: slim
|
29
|
+
requirement: !ruby/object:Gem::Requirement
|
30
|
+
requirements:
|
31
|
+
- - ">="
|
32
|
+
- !ruby/object:Gem::Version
|
33
|
+
version: '0'
|
34
|
+
type: :runtime
|
35
|
+
prerelease: false
|
36
|
+
version_requirements: !ruby/object:Gem::Requirement
|
37
|
+
requirements:
|
38
|
+
- - ">="
|
39
|
+
- !ruby/object:Gem::Version
|
40
|
+
version: '0'
|
41
|
+
- !ruby/object:Gem::Dependency
|
42
|
+
name: factory_girl_rails
|
43
|
+
requirement: !ruby/object:Gem::Requirement
|
44
|
+
requirements:
|
45
|
+
- - ">="
|
46
|
+
- !ruby/object:Gem::Version
|
47
|
+
version: '0'
|
48
|
+
type: :development
|
49
|
+
prerelease: false
|
50
|
+
version_requirements: !ruby/object:Gem::Requirement
|
51
|
+
requirements:
|
52
|
+
- - ">="
|
53
|
+
- !ruby/object:Gem::Version
|
54
|
+
version: '0'
|
55
|
+
description: A web interface for factory_girl
|
56
|
+
email:
|
57
|
+
- yhirano@me.com
|
58
|
+
executables: []
|
59
|
+
extensions: []
|
60
|
+
extra_rdoc_files: []
|
61
|
+
files:
|
62
|
+
- MIT-LICENSE
|
63
|
+
- README.md
|
64
|
+
- Rakefile
|
65
|
+
- app/controllers/factory_girl_web/application_controller.rb
|
66
|
+
- app/controllers/factory_girl_web/fixtures_controller.rb
|
67
|
+
- app/helpers/factory_girl_web/application_helper.rb
|
68
|
+
- app/models/factory_girl_web/fixture.rb
|
69
|
+
- app/views/factory_girl_web/fixtures/index.html.slim
|
70
|
+
- app/views/layouts/factory_girl_web/application.html.slim
|
71
|
+
- app/views/shared/factory_girl_web/_footer.html.slim
|
72
|
+
- app/views/shared/factory_girl_web/_header.html.slim
|
73
|
+
- config/routes.rb
|
74
|
+
- lib/factory_girl_web.rb
|
75
|
+
- lib/factory_girl_web/engine.rb
|
76
|
+
- lib/factory_girl_web/version.rb
|
77
|
+
homepage: https://github.com/yhirano55/factory_girl_web
|
78
|
+
licenses:
|
79
|
+
- MIT
|
80
|
+
metadata: {}
|
81
|
+
post_install_message:
|
82
|
+
rdoc_options: []
|
83
|
+
require_paths:
|
84
|
+
- lib
|
85
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
86
|
+
requirements:
|
87
|
+
- - ">="
|
88
|
+
- !ruby/object:Gem::Version
|
89
|
+
version: '0'
|
90
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
91
|
+
requirements:
|
92
|
+
- - ">="
|
93
|
+
- !ruby/object:Gem::Version
|
94
|
+
version: '0'
|
95
|
+
requirements: []
|
96
|
+
rubyforge_project:
|
97
|
+
rubygems_version: 2.5.1
|
98
|
+
signing_key:
|
99
|
+
specification_version: 4
|
100
|
+
summary: A web interface for factory_girl
|
101
|
+
test_files: []
|