factory_girl 3.6.2 → 4.0.0.rc1
Sign up to get free protection for your applications and to get access to all the features.
- data/GETTING_STARTED.md +35 -43
- data/Gemfile.lock +1 -1
- data/README.md +0 -6
- data/features/step_definitions/database_steps.rb +2 -35
- data/features/step_definitions/factory_girl_steps.rb +0 -7
- data/features/support/factories.rb +1 -124
- data/gemfiles/3.0.gemfile.lock +1 -1
- data/gemfiles/3.1.gemfile.lock +1 -1
- data/gemfiles/3.2.gemfile.lock +1 -1
- data/lib/factory_girl.rb +1 -1
- data/lib/factory_girl/attribute_assigner.rb +2 -14
- data/lib/factory_girl/configuration.rb +8 -4
- data/lib/factory_girl/definition_proxy.rb +0 -4
- data/lib/factory_girl/syntax.rb +0 -11
- data/lib/factory_girl/version.rb +1 -1
- data/spec/acceptance/global_initialize_with_spec.rb +0 -2
- data/spec/acceptance/initialize_with_spec.rb +0 -44
- data/spec/acceptance/traits_spec.rb +0 -2
- data/spec/factory_girl/aliases_spec.rb +1 -1
- metadata +5 -24
- data/features/factory_girl_steps.feature +0 -241
- data/lib/factory_girl/decorator/invocation_ignorer.rb +0 -9
- data/lib/factory_girl/step_definitions.rb +0 -151
- data/lib/factory_girl/syntax/blueprint.rb +0 -40
- data/lib/factory_girl/syntax/generate.rb +0 -70
- data/lib/factory_girl/syntax/make.rb +0 -44
- data/lib/factory_girl/syntax/sham.rb +0 -47
- data/lib/factory_girl/syntax/vintage.rb +0 -130
- data/spec/acceptance/syntax/blueprint_spec.rb +0 -36
- data/spec/acceptance/syntax/generate_spec.rb +0 -61
- data/spec/acceptance/syntax/make_spec.rb +0 -54
- data/spec/acceptance/syntax/sham_spec.rb +0 -43
- data/spec/acceptance/syntax/vintage_spec.rb +0 -219
data/GETTING_STARTED.md
CHANGED
@@ -7,13 +7,13 @@ Update Your Gemfile
|
|
7
7
|
If you're using Rails, you'll need to change the required version of `factory_girl_rails`:
|
8
8
|
|
9
9
|
```ruby
|
10
|
-
gem "factory_girl_rails", "~>
|
10
|
+
gem "factory_girl_rails", "~> 4.0"
|
11
11
|
```
|
12
12
|
|
13
13
|
If you're *not* using Rails, you'll just have to change the required version of `factory_girl`:
|
14
14
|
|
15
15
|
```ruby
|
16
|
-
gem "factory_girl", "~>
|
16
|
+
gem "factory_girl", "~> 4.0"
|
17
17
|
```
|
18
18
|
|
19
19
|
JRuby users: FactoryGirl works with JRuby starting with 1.6.7.2 (latest stable, as per July 2012).
|
@@ -875,14 +875,41 @@ FactoryGirl.define do
|
|
875
875
|
end
|
876
876
|
```
|
877
877
|
|
878
|
-
When using `initialize_with`, attributes accessed from the `initialize_with`
|
879
|
-
block are assigned
|
880
|
-
|
878
|
+
When using `initialize_with`, attributes accessed from within the `initialize_with`
|
879
|
+
block are assigned *only* in the constructor; this equates to roughly the
|
880
|
+
following code:
|
881
881
|
|
882
|
-
|
882
|
+
```ruby
|
883
|
+
FactoryGirl.define do
|
884
|
+
factory :user do
|
885
|
+
initialize_with { new(name) }
|
886
|
+
|
887
|
+
name { 'value' }
|
888
|
+
end
|
889
|
+
end
|
890
|
+
|
891
|
+
FactoryGirl.build(:user)
|
892
|
+
# runs
|
893
|
+
User.new('value')
|
894
|
+
```
|
895
|
+
|
896
|
+
This prevents duplicate assignment; in versions of FactoryGirl before 4.0, it
|
897
|
+
would run this:
|
883
898
|
|
884
|
-
|
885
|
-
|
899
|
+
```ruby
|
900
|
+
FactoryGirl.define do
|
901
|
+
factory :user do
|
902
|
+
initialize_with { new(name) }
|
903
|
+
|
904
|
+
name { 'value' }
|
905
|
+
end
|
906
|
+
end
|
907
|
+
|
908
|
+
FactoryGirl.build(:user)
|
909
|
+
# runs
|
910
|
+
user = User.new('value')
|
911
|
+
user.name = 'value'
|
912
|
+
```
|
886
913
|
|
887
914
|
Custom Strategies
|
888
915
|
-----------------
|
@@ -1042,38 +1069,3 @@ config.after(:suite) do
|
|
1042
1069
|
puts @factory_girl_results
|
1043
1070
|
end
|
1044
1071
|
```
|
1045
|
-
|
1046
|
-
Cucumber Integration
|
1047
|
-
--------------------
|
1048
|
-
|
1049
|
-
factory\_girl ships with step definitions that make calling factories from Cucumber easier. To use them, add the following to features/support/env.rb:
|
1050
|
-
|
1051
|
-
```ruby
|
1052
|
-
require "factory_girl/step_definitions"
|
1053
|
-
```
|
1054
|
-
|
1055
|
-
Note: These step definitions are _deprecated_. Read why here:
|
1056
|
-
http://robots.thoughtbot.com/post/25650434584/writing-better-cucumber-scenarios-or-why-were
|
1057
|
-
|
1058
|
-
Alternate Syntaxes
|
1059
|
-
------------------
|
1060
|
-
|
1061
|
-
Users' tastes for syntax vary dramatically, but most users are looking for a
|
1062
|
-
common feature set. Because of this factory\_girl supports "syntax layers" which
|
1063
|
-
provide alternate interfaces. See Factory::Syntax for information about the
|
1064
|
-
various layers available. For example, the Machinist-style syntax is popular:
|
1065
|
-
|
1066
|
-
```ruby
|
1067
|
-
require "factory_girl/syntax/blueprint"
|
1068
|
-
require "factory_girl/syntax/make"
|
1069
|
-
require "factory_girl/syntax/sham"
|
1070
|
-
|
1071
|
-
Sham.email {|n| "#{n}@example.com" }
|
1072
|
-
|
1073
|
-
User.blueprint do
|
1074
|
-
name { "Billy Bob" }
|
1075
|
-
email { Sham.email }
|
1076
|
-
end
|
1077
|
-
|
1078
|
-
User.make(name: "Johnny")
|
1079
|
-
```
|
data/Gemfile.lock
CHANGED
data/README.md
CHANGED
@@ -62,12 +62,6 @@ factory_girl was written by Joe Ferris with contributions from several authors,
|
|
62
62
|
* Josh Clayton
|
63
63
|
* Thomas Walpole
|
64
64
|
|
65
|
-
The syntax layers are derived from software written by the following authors:
|
66
|
-
|
67
|
-
* Pete Yandell
|
68
|
-
* Rick Bradley
|
69
|
-
* Yossef Mendelssohn
|
70
|
-
|
71
65
|
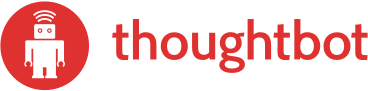
|
72
66
|
|
73
67
|
factory_girl is maintained and funded by [thoughtbot, inc](http://thoughtbot.com/community)
|
@@ -1,42 +1,9 @@
|
|
1
|
-
Then /^I should find the following for the last
|
2
|
-
model_class = model.camelize.constantize
|
3
|
-
last_instance = model_class.last or raise "No #{model.pluralize} exist"
|
1
|
+
Then /^I should find the following for the last category:$/ do |table|
|
4
2
|
table.hashes.first.each do |key, value|
|
5
|
-
|
3
|
+
Category.last.attributes[key].to_s.should == value
|
6
4
|
end
|
7
5
|
end
|
8
6
|
|
9
|
-
Then /^there should be (\d+) (.*)$/ do |count, model|
|
10
|
-
model_class = model.singularize.camelize.constantize
|
11
|
-
model_class.count.should == count.to_i
|
12
|
-
end
|
13
|
-
|
14
|
-
Then /^the post "([^"]*)" should (not )?have the following tags?:$/ do |post_title, negate, table|
|
15
|
-
post = Post.find_by_title!(post_title)
|
16
|
-
|
17
|
-
table.hashes.each do |row|
|
18
|
-
tag = Tag.find_by_name(row[:name])
|
19
|
-
|
20
|
-
if negate
|
21
|
-
post.tags.should_not include(tag)
|
22
|
-
else
|
23
|
-
post.tags.should include(tag)
|
24
|
-
end
|
25
|
-
end
|
26
|
-
end
|
27
|
-
|
28
|
-
Transform /^table:(?:.*,)?tags(?:,.*)?$/ do |table|
|
29
|
-
table.map_column!("tags") do |tags|
|
30
|
-
tags.split(',').map {|tag| Tag.find_by_name! tag.strip }
|
31
|
-
end
|
32
|
-
table
|
33
|
-
end
|
34
|
-
|
35
7
|
Before do
|
36
|
-
Post.delete_all
|
37
|
-
Tag.delete_all
|
38
|
-
User.delete_all
|
39
8
|
Category.delete_all
|
40
|
-
CategoryGroup.delete_all
|
41
9
|
end
|
42
|
-
|
@@ -27,13 +27,6 @@ When /^I create a "([^"]*)" instance from FactoryGirl$/ do |factory_name|
|
|
27
27
|
FactoryGirl.create(factory_name)
|
28
28
|
end
|
29
29
|
|
30
|
-
Given /^these super users exist:$/ do |table|
|
31
|
-
headers = table.headers + ["admin"]
|
32
|
-
rows = table.rows.map { |row| row + [true] }
|
33
|
-
new_table = Cucumber::Ast::Table.new([headers] + rows)
|
34
|
-
step %{the following person exists:}, new_table
|
35
|
-
end
|
36
|
-
|
37
30
|
When /^I find definitions$/ do
|
38
31
|
FactoryGirl.find_definitions
|
39
32
|
end
|
@@ -5,135 +5,12 @@ ActiveRecord::Base.establish_connection(
|
|
5
5
|
|
6
6
|
class CreateSchema < ActiveRecord::Migration
|
7
7
|
def self.up
|
8
|
-
create_table :posts, :force => true do |t|
|
9
|
-
t.integer :author_id
|
10
|
-
t.integer :category_id
|
11
|
-
t.string :title
|
12
|
-
t.string :body
|
13
|
-
end
|
14
|
-
|
15
|
-
create_table :category_groups, :force => true do |t|
|
16
|
-
t.string :name
|
17
|
-
end
|
18
|
-
|
19
8
|
create_table :categories, :force => true do |t|
|
20
|
-
t.integer :category_group_id
|
21
|
-
t.string :name
|
22
|
-
end
|
23
|
-
|
24
|
-
create_table :tags, :force => true do |t|
|
25
|
-
t.integer :post_id
|
26
9
|
t.string :name
|
27
10
|
end
|
28
|
-
|
29
|
-
create_table :users, :force => true do |t|
|
30
|
-
t.string :name
|
31
|
-
t.boolean :admin, :default => false, :null => false
|
32
|
-
end
|
33
11
|
end
|
34
12
|
end
|
35
13
|
|
36
14
|
CreateSchema.suppress_messages { CreateSchema.migrate(:up) }
|
37
15
|
|
38
|
-
class
|
39
|
-
end
|
40
|
-
|
41
|
-
class CategoryGroup < ActiveRecord::Base
|
42
|
-
end
|
43
|
-
|
44
|
-
class Category < ActiveRecord::Base
|
45
|
-
belongs_to :category_group
|
46
|
-
end
|
47
|
-
|
48
|
-
class Post < ActiveRecord::Base
|
49
|
-
belongs_to :author, :class_name => 'User'
|
50
|
-
belongs_to :category
|
51
|
-
has_many :tags
|
52
|
-
end
|
53
|
-
|
54
|
-
class Tag < ActiveRecord::Base
|
55
|
-
belongs_to :post
|
56
|
-
end
|
57
|
-
|
58
|
-
class NonActiveRecord
|
59
|
-
end
|
60
|
-
|
61
|
-
class SimpleColumn
|
62
|
-
def self.columns
|
63
|
-
[:name]
|
64
|
-
end
|
65
|
-
|
66
|
-
def save!
|
67
|
-
@@count ||= 0
|
68
|
-
@@count += 1
|
69
|
-
end
|
70
|
-
|
71
|
-
def self.count
|
72
|
-
@@count
|
73
|
-
end
|
74
|
-
end
|
75
|
-
|
76
|
-
class NamedAttributeModel
|
77
|
-
def self.attribute_names
|
78
|
-
%w(title)
|
79
|
-
end
|
80
|
-
|
81
|
-
attr_accessor :title
|
82
|
-
|
83
|
-
def save!
|
84
|
-
@@count ||= 0
|
85
|
-
@@count += 1
|
86
|
-
end
|
87
|
-
|
88
|
-
def self.count
|
89
|
-
@@count
|
90
|
-
end
|
91
|
-
end
|
92
|
-
|
93
|
-
FactoryGirl.define do
|
94
|
-
# To make sure the step defs work with an email
|
95
|
-
sequence :email do |n|
|
96
|
-
"email#{n}@example.com"
|
97
|
-
end
|
98
|
-
|
99
|
-
factory :user, :aliases => [:person] do
|
100
|
-
factory :admin_user do
|
101
|
-
admin true
|
102
|
-
end
|
103
|
-
end
|
104
|
-
|
105
|
-
factory :category do
|
106
|
-
name "programming"
|
107
|
-
category_group
|
108
|
-
end
|
109
|
-
|
110
|
-
factory :category_group do
|
111
|
-
name "tecnhology"
|
112
|
-
end
|
113
|
-
|
114
|
-
factory :post do
|
115
|
-
association :author, :factory => :user
|
116
|
-
category
|
117
|
-
end
|
118
|
-
|
119
|
-
factory :titled_post, :parent => :post do
|
120
|
-
title 'A Post with a Title'
|
121
|
-
end
|
122
|
-
|
123
|
-
factory :tag do
|
124
|
-
post
|
125
|
-
end
|
126
|
-
# This is here to ensure that factory step definitions don't raise for a non-AR factory
|
127
|
-
factory :non_active_record do
|
128
|
-
end
|
129
|
-
|
130
|
-
# This is here to make FG work with ORMs that have `columns => [:name, :admin, :etc]` on the class (Neo4j)
|
131
|
-
factory :simple_column do
|
132
|
-
end
|
133
|
-
|
134
|
-
factory :named_attribute_model do
|
135
|
-
end
|
136
|
-
end
|
137
|
-
|
138
|
-
require 'factory_girl/step_definitions'
|
139
|
-
|
16
|
+
class Category < ActiveRecord::Base; end
|
data/gemfiles/3.0.gemfile.lock
CHANGED
data/gemfiles/3.1.gemfile.lock
CHANGED
data/gemfiles/3.2.gemfile.lock
CHANGED
data/lib/factory_girl.rb
CHANGED
@@ -1,5 +1,6 @@
|
|
1
1
|
require 'set'
|
2
2
|
require 'active_support/core_ext/module/delegation'
|
3
|
+
require 'active_support/deprecation'
|
3
4
|
require 'active_support/notifications'
|
4
5
|
|
5
6
|
require 'factory_girl/definition_hierarchy'
|
@@ -41,7 +42,6 @@ require 'factory_girl/decorator/attribute_hash'
|
|
41
42
|
require 'factory_girl/decorator/class_key_hash'
|
42
43
|
require 'factory_girl/decorator/disallows_duplicates_registry'
|
43
44
|
require 'factory_girl/decorator/invocation_tracker'
|
44
|
-
require 'factory_girl/decorator/invocation_ignorer'
|
45
45
|
require 'factory_girl/decorator/new_constructor'
|
46
46
|
require 'factory_girl/version'
|
47
47
|
|
@@ -35,29 +35,17 @@ module FactoryGirl
|
|
35
35
|
end
|
36
36
|
|
37
37
|
def decorated_evaluator
|
38
|
-
|
38
|
+
Decorator::InvocationTracker.new(
|
39
39
|
Decorator::NewConstructor.new(@evaluator, @build_class)
|
40
40
|
)
|
41
41
|
end
|
42
42
|
|
43
|
-
def invocation_decorator
|
44
|
-
if FactoryGirl.duplicate_attribute_assignment_from_initialize_with
|
45
|
-
Decorator::InvocationIgnorer
|
46
|
-
else
|
47
|
-
Decorator::InvocationTracker
|
48
|
-
end
|
49
|
-
end
|
50
|
-
|
51
43
|
def methods_invoked_on_evaluator
|
52
44
|
method_tracking_evaluator.__invoked_methods__
|
53
45
|
end
|
54
46
|
|
55
47
|
def build_class_instance
|
56
|
-
@build_class_instance ||= method_tracking_evaluator.instance_exec(&@instance_builder)
|
57
|
-
if @instance_builder != FactoryGirl.constructor && FactoryGirl.duplicate_attribute_assignment_from_initialize_with
|
58
|
-
ActiveSupport::Deprecation.warn 'Accessing attributes from initialize_with when duplicate assignment is enabled is deprecated; use FactoryGirl.duplicate_attribute_assignment_from_initialize_with = false.', caller
|
59
|
-
end
|
60
|
-
end
|
48
|
+
@build_class_instance ||= method_tracking_evaluator.instance_exec(&@instance_builder)
|
61
49
|
end
|
62
50
|
|
63
51
|
def build_hash
|
@@ -3,8 +3,6 @@ module FactoryGirl
|
|
3
3
|
class Configuration
|
4
4
|
attr_reader :factories, :sequences, :traits, :strategies, :callback_names
|
5
5
|
|
6
|
-
attr_accessor :duplicate_attribute_assignment_from_initialize_with
|
7
|
-
|
8
6
|
def initialize
|
9
7
|
@factories = Decorator::DisallowsDuplicatesRegistry.new(Registry.new('Factory'))
|
10
8
|
@sequences = Decorator::DisallowsDuplicatesRegistry.new(Registry.new('Sequence'))
|
@@ -13,8 +11,6 @@ module FactoryGirl
|
|
13
11
|
@callback_names = Set.new
|
14
12
|
@definition = Definition.new
|
15
13
|
|
16
|
-
@duplicate_attribute_assignment_from_initialize_with = true
|
17
|
-
|
18
14
|
to_create {|instance| instance.save! }
|
19
15
|
initialize_with { new }
|
20
16
|
end
|
@@ -24,5 +20,13 @@ module FactoryGirl
|
|
24
20
|
def initialize_with(&block)
|
25
21
|
@definition.define_constructor(&block)
|
26
22
|
end
|
23
|
+
|
24
|
+
def duplicate_attribute_assignment_from_initialize_with
|
25
|
+
false
|
26
|
+
end
|
27
|
+
|
28
|
+
def duplicate_attribute_assignment_from_initialize_with=(value)
|
29
|
+
ActiveSupport::Deprecation.warn 'Assignment of duplicate_attribute_assignment_from_initialize_with is unnecessary as this is now default behavior in FactoryGirl 4.0; this line can be removed', caller
|
30
|
+
end
|
27
31
|
end
|
28
32
|
end
|
@@ -85,10 +85,6 @@ module FactoryGirl
|
|
85
85
|
@definition.declare_attribute(Declaration::Implicit.new(name, @definition, @ignore))
|
86
86
|
elsif args.first.respond_to?(:has_key?) && args.first.has_key?(:factory)
|
87
87
|
association(name, *args)
|
88
|
-
elsif FactoryGirl.callback_names.include?(name)
|
89
|
-
callback_when, callback_name = name.to_s.split('_', 2)
|
90
|
-
ActiveSupport::Deprecation.warn "Calling #{name} is deprecated; use the syntax #{callback_when}(:#{callback_name}) {}", caller
|
91
|
-
@definition.add_callback(Callback.new(name, block))
|
92
88
|
else
|
93
89
|
add_attribute(name, *args, &block)
|
94
90
|
end
|