doneski 0.1.3 → 0.1.4
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/README.md +48 -2
- data/bin/doneski +16 -12
- data/lib/doneski/task.rb +14 -1
- data/lib/doneski/tasks_collection.rb +20 -2
- data/lib/doneski/version.rb +1 -1
- metadata +3 -3
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 435913091b7bc929cf8a082042e8160ac6302cec
|
4
|
+
data.tar.gz: 7b045bc7e9d5994b6d2f034ace0771acbd4c19d7
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 6112609f0fbc13d2269760eb55d35d1630643829a99b4e6b174352e8dc76b4e64988f330ebab4c250de90c206c3ee80bb45558b65ae74362894a394f27c34b17
|
7
|
+
data.tar.gz: c4103d032e8d8a0ee91bfec312384cb7d585b11ee3f834888f6b52df816eeb2b4b45a21db51ea7ebe11cca42da69f3b469fc70ce551ff9ce6514c9b36e3e5683
|
data/README.md
CHANGED
@@ -1,9 +1,55 @@
|
|
1
1
|
# doneski
|
2
2
|
|
3
|
+
[](http://badge.fury.io/rb/doneski)
|
4
|
+
|
3
5
|
This is a quick CLI to manage tasks.
|
4
6
|
|
5
|
-
|
7
|
+
You can install the gem simply with:
|
8
|
+
|
9
|
+
```
|
10
|
+
$ gem install doneski
|
11
|
+
```
|
6
12
|
|
7
|
-
|
13
|
+
Simply run `doneski` to list your current tasks. You can add a task by calling `doneski -a The task title here`.
|
8
14
|
|
9
15
|
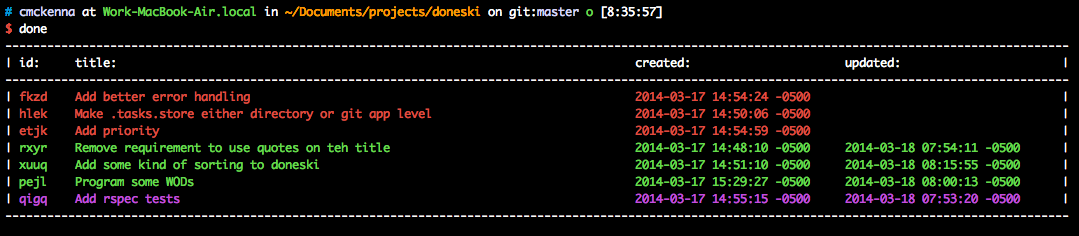
|
16
|
+
|
17
|
+
## Current functionality:
|
18
|
+
### Listing tasks
|
19
|
+
```
|
20
|
+
$ doneski -l [column to sort]
|
21
|
+
```
|
22
|
+
Example: `doneski -l title` will sort by title
|
23
|
+
|
24
|
+
### Adding tasks
|
25
|
+
```
|
26
|
+
$ doneski -a The task title goes here
|
27
|
+
```
|
28
|
+
This will add a new task with the title `The task title goes here` and move the task to the `:new` phase
|
29
|
+
|
30
|
+
### Removing tasks
|
31
|
+
```
|
32
|
+
$ doneski -r [id]
|
33
|
+
```
|
34
|
+
Example: `doneski -r xysf` will remove the task with the `id` of `xysf`
|
35
|
+
Example: `doneski -r` will remove ALL completed tasks
|
36
|
+
|
37
|
+
### Starting tasks
|
38
|
+
```
|
39
|
+
$ doneski -s id
|
40
|
+
```
|
41
|
+
Example: `doneski -s xysf` will move the task with the id `xysf` to the `:started` phase
|
42
|
+
|
43
|
+
### Finishing tasks
|
44
|
+
```
|
45
|
+
$ doneski -f id
|
46
|
+
```
|
47
|
+
Example: `doneski -f xysf` will finish the task with the id `xysf` to the `:completed` phase
|
48
|
+
|
49
|
+
### Getting help
|
50
|
+
```
|
51
|
+
$ doneski -h
|
52
|
+
```
|
53
|
+
This will display the help menu
|
54
|
+
|
55
|
+
Ruby 2.0 and 1.9.3 have been tested.
|
data/bin/doneski
CHANGED
@@ -2,36 +2,40 @@
|
|
2
2
|
require 'optparse'
|
3
3
|
require 'doneski'
|
4
4
|
|
5
|
-
@
|
6
|
-
@sort = :
|
5
|
+
@task_collection = TasksCollection.new
|
6
|
+
@sort = :priority
|
7
7
|
|
8
8
|
options = {}
|
9
9
|
|
10
10
|
optparse = OptionParser.new do |opts|
|
11
11
|
opts.banner = "Usage: doneski.rb"
|
12
12
|
|
13
|
-
opts.on('-l', '--list [sort]', 'Show all tasks. Optionally you can supply a column to sort by: id, title, date_created,
|
14
|
-
@sort = sort.downcase.to_sym if sort && ['id', 'title', 'date_created', 'stage'].include?(sort.downcase)
|
15
|
-
@
|
13
|
+
opts.on('-l', '--list [sort]', 'Show all tasks. Optionally you can supply a column to sort by: id, title, date_created, stage, priority') do |sort|
|
14
|
+
@sort = sort.downcase.to_sym if sort && ['id', 'title', 'date_created', 'stage', 'priority'].include?(sort.downcase)
|
15
|
+
@task_collection.all
|
16
16
|
end
|
17
17
|
|
18
18
|
opts.on('-r', '--remove [ID]', 'Clears all completed tasks from the list') do |id|
|
19
|
-
@
|
20
|
-
@
|
19
|
+
@task_collection.remove id: id if id
|
20
|
+
@task_collection.remove stage: Task::STATUS[:complete] unless id
|
21
21
|
end
|
22
22
|
|
23
23
|
opts.on('-a', '--add title', 'Add a new task') do |title|
|
24
|
-
@sort = :date_created
|
25
24
|
ARGV.unshift title
|
26
|
-
@
|
25
|
+
@task_collection.add("#{ARGV.join(' ')}")
|
27
26
|
end
|
28
27
|
|
29
28
|
opts.on('-s', '--start id', 'Start a task') do |id|
|
30
|
-
@
|
29
|
+
@task_collection.start(id)
|
31
30
|
end
|
32
31
|
|
33
32
|
opts.on('-f', '--finish id', "Finish a task") do |id|
|
34
|
-
@
|
33
|
+
@task_collection.finish(id)
|
34
|
+
end
|
35
|
+
|
36
|
+
opts.on('-p', '--priority id rating', "Set priority on a task (higher priority has more ++++)") do |id|
|
37
|
+
ARGV.unshift id
|
38
|
+
@task_collection.set_priority(ARGV[0], ARGV[1])
|
35
39
|
end
|
36
40
|
|
37
41
|
opts.on('-h', '--help', 'Display help') do
|
@@ -43,5 +47,5 @@ end
|
|
43
47
|
optparse.parse!
|
44
48
|
|
45
49
|
puts TasksCollection.display_header
|
46
|
-
puts @
|
50
|
+
puts @task_collection.sort(@sort)
|
47
51
|
puts TasksCollection.display_footer
|
data/lib/doneski/task.rb
CHANGED
@@ -10,6 +10,7 @@ class Task
|
|
10
10
|
@stage = options['stage'] || STATUS[:new]
|
11
11
|
@date_created = options['date_created'] || Time.now
|
12
12
|
@date_updated = options['date_updated'] || nil
|
13
|
+
@priority = options['priority'] || ''
|
13
14
|
end
|
14
15
|
|
15
16
|
def complete
|
@@ -17,6 +18,18 @@ class Task
|
|
17
18
|
@stage = STATUS[:complete]
|
18
19
|
end
|
19
20
|
|
21
|
+
def priority
|
22
|
+
!@priority.nil? ? -@priority.length : nil
|
23
|
+
end
|
24
|
+
|
25
|
+
def priority=(priority)
|
26
|
+
if priority.nil?
|
27
|
+
@priority = ''
|
28
|
+
else
|
29
|
+
@priority = priority.match(/\+{1,}/)[0] unless priority.nil?
|
30
|
+
end
|
31
|
+
end
|
32
|
+
|
20
33
|
def start
|
21
34
|
@date_updated = Time.now
|
22
35
|
@stage = STATUS[:started]
|
@@ -28,6 +41,6 @@ class Task
|
|
28
41
|
end
|
29
42
|
|
30
43
|
def to_s
|
31
|
-
"| \e[0;3#{stage.to_s}m#{id.to_s.ljust(8)}#{title.ljust(80)[0...80]}#{date_created.to_s.ljust(30)}#{date_updated.to_s.ljust(30)}\e[0m |"
|
44
|
+
"| \e[0;3#{stage.to_s}m#{id.to_s.ljust(8)}#{title.ljust(80)[0...80]}#{date_created.to_s.ljust(30)}#{date_updated.to_s.ljust(30)}#{@priority.ljust(10)}\e[0m |"
|
32
45
|
end
|
33
46
|
end
|
@@ -8,6 +8,7 @@ class TasksCollection
|
|
8
8
|
create unless File.exists? @@location
|
9
9
|
@yaml = YAML.load_file(@@location)
|
10
10
|
@tasks = @yaml ? @yaml['tasks'] : []
|
11
|
+
update_attributes(@tasks)
|
11
12
|
end
|
12
13
|
|
13
14
|
def all
|
@@ -23,6 +24,11 @@ class TasksCollection
|
|
23
24
|
store
|
24
25
|
end
|
25
26
|
|
27
|
+
def set_priority(id, priority)
|
28
|
+
@tasks.select{|task| task.match({id: id})}.first.priority = priority
|
29
|
+
store
|
30
|
+
end
|
31
|
+
|
26
32
|
def add(title)
|
27
33
|
@tasks << Task.new({'title' => title})
|
28
34
|
store
|
@@ -39,11 +45,11 @@ class TasksCollection
|
|
39
45
|
end
|
40
46
|
|
41
47
|
def self.display_header
|
42
|
-
"#{'-'*
|
48
|
+
"#{'-'*162}\n| #{'id:'.ljust(8)}#{'title:'.ljust(80)}#{'created:'.ljust(30)}#{'updated:'.ljust(30)}#{'priority:'.ljust(10)} |\n#{'-'*162}"
|
43
49
|
end
|
44
50
|
|
45
51
|
def self.display_footer
|
46
|
-
"#{'-'*
|
52
|
+
"#{'-'*162}"
|
47
53
|
end
|
48
54
|
|
49
55
|
private
|
@@ -59,4 +65,16 @@ private
|
|
59
65
|
File.open(@@location, 'w+'){|f| f.puts "# .task.store"}
|
60
66
|
end
|
61
67
|
|
68
|
+
def update_attributes(tasks)
|
69
|
+
tasks.each do |task|
|
70
|
+
attrs.each do |attr|
|
71
|
+
task.send((attr.to_s+"=").to_sym, '') unless task.send attr
|
72
|
+
end
|
73
|
+
end
|
74
|
+
end
|
75
|
+
|
76
|
+
def attrs
|
77
|
+
[:title, :date_created, :date_updated, :stage, :priority]
|
78
|
+
end
|
79
|
+
|
62
80
|
end
|
data/lib/doneski/version.rb
CHANGED
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: doneski
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.4
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Chad McKenna
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2014-03-
|
11
|
+
date: 2014-03-28 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: bundler
|
@@ -79,7 +79,7 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
79
79
|
version: '0'
|
80
80
|
requirements: []
|
81
81
|
rubyforge_project:
|
82
|
-
rubygems_version: 2.
|
82
|
+
rubygems_version: 2.2.2
|
83
83
|
signing_key:
|
84
84
|
specification_version: 4
|
85
85
|
summary: A simple task management CLI
|