dingbot 0.2.3 → 0.2.5
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +5 -5
- data/README.md +5 -3
- data/lib/dingbot/client.rb +22 -3
- data/lib/dingbot/configuration.rb +3 -2
- data/lib/dingbot/error.rb +0 -14
- data/lib/dingbot/message/action_card.rb +33 -9
- data/lib/dingbot/version.rb +1 -1
- data/lib/dingbot.rb +1 -1
- metadata +3 -4
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
|
-
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: d5786404b17bd985d218ca86427d9295b8aa0e3c62e5de0d1a9a711bbc68b912
|
4
|
+
data.tar.gz: f1b404b5ef785d3239f9e8dcbfffea5fac403d117537b89b1122a01a1b42f006
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 43acda49cef2e0496a98cdff8136fd2e8cf58a247fa78bcf850690d71443aebff784d5dd79ab895df3e378ab4b125dd810f359dd62b93f0d75cd4e1fe98a6751
|
7
|
+
data.tar.gz: d4f9c6a4cabee5ebaf06a0b3837fa2c584440d18d3e6676bc51fd9197e3b50f428b7f657ef66bcd9b45c8545841440bcdc2d9a3afcfbccf23d7f3fbb427b6a20
|
data/README.md
CHANGED
@@ -4,7 +4,7 @@
|
|
4
4
|
[](https://raw.githubusercontent.com/thierryxing/dingtalk-bot/master/LICENSE.txt)
|
5
5
|
|
6
6
|
DingTalk Bot是阿里钉钉自定义机器人的Ruby库
|
7
|
-
官方文档:[阿里钉钉自定义机器人](https://
|
7
|
+
官方文档:[阿里钉钉自定义机器人](https://developers.dingtalk.com/document/robots/robot-overview).
|
8
8
|
|
9
9
|
|
10
10
|
## 安装
|
@@ -28,12 +28,14 @@ gem 'dingbot'
|
|
28
28
|
# 全局配置方式
|
29
29
|
DingBot.configure do |config|
|
30
30
|
config.endpoint = 'https://oapi.dingtalk.com/robot/send' # API endpoint URL, default: ENV['DINGTALK_API_ENDPOINT'] or https://oapi.dingtalk.com/robot/send
|
31
|
-
config.access_token = '
|
31
|
+
config.access_token = 'your access token' # access token, default: ENV['DINGTALK_ACCESS_TOKEN']
|
32
|
+
config.secret = "your sign secret" # sign secret
|
32
33
|
end
|
33
34
|
|
34
35
|
# 局部配置方式
|
35
36
|
DingBot.endpoint='https://oapi.dingtalk.com/robot/send'
|
36
|
-
DingBot.access_token = '
|
37
|
+
DingBot.access_token = 'your access token'
|
38
|
+
DingBot.secret = "your sign secret" # sign secret
|
37
39
|
```
|
38
40
|
|
39
41
|
发送消息
|
data/lib/dingbot/client.rb
CHANGED
@@ -1,4 +1,5 @@
|
|
1
1
|
require 'httparty'
|
2
|
+
require 'json'
|
2
3
|
require 'dingbot/configuration'
|
3
4
|
require 'dingbot/message/base'
|
4
5
|
require 'dingbot/message/text'
|
@@ -12,7 +13,8 @@ module DingBot
|
|
12
13
|
format :json
|
13
14
|
headers "Content-Type" => "application/json"
|
14
15
|
|
15
|
-
attr_accessor :access_token
|
16
|
+
# attr_accessor :access_token
|
17
|
+
# attr_accessor :secret
|
16
18
|
|
17
19
|
# @private
|
18
20
|
attr_accessor(*Configuration::VALID_OPTIONS_KEYS)
|
@@ -43,7 +45,20 @@ module DingBot
|
|
43
45
|
end
|
44
46
|
|
45
47
|
def send_msg(message)
|
46
|
-
|
48
|
+
query = {
|
49
|
+
access_token: @access_token,
|
50
|
+
}
|
51
|
+
|
52
|
+
if !@secret.nil? and !@secret.empty?
|
53
|
+
timestamp = (Time.now.to_f * 1000).to_i
|
54
|
+
|
55
|
+
query.merge!({
|
56
|
+
timestamp: timestamp,
|
57
|
+
sign: Base64.encode64(OpenSSL::HMAC.digest(OpenSSL::Digest.new('sha256'), @secret, "#{timestamp}\n#{@secret}")).strip
|
58
|
+
})
|
59
|
+
end
|
60
|
+
|
61
|
+
validate self.class.post(@endpoint, { query: query, body: message.to_json })
|
47
62
|
end
|
48
63
|
|
49
64
|
def send_text(content)
|
@@ -83,8 +98,12 @@ module DingBot
|
|
83
98
|
end
|
84
99
|
|
85
100
|
fail error_klass.new(response) if error_klass
|
86
|
-
|
87
101
|
parsed = response.parsed_response
|
102
|
+
|
103
|
+
body = JSON.parse(response.body)
|
104
|
+
errcode = body["errcode"]
|
105
|
+
fail body["errmsg"] if errcode != 0
|
106
|
+
|
88
107
|
parsed.client = self if parsed.respond_to?(:client=)
|
89
108
|
parsed.parse_headers!(response.headers) if parsed.respond_to?(:parse_headers!)
|
90
109
|
parsed
|
@@ -1,8 +1,8 @@
|
|
1
1
|
module DingBot
|
2
2
|
# Defines constants and methods related to configuration.
|
3
3
|
module Configuration
|
4
|
-
# An array of valid keys in the options hash when configuring a
|
5
|
-
VALID_OPTIONS_KEYS = [:endpoint, :access_token, :httparty].freeze
|
4
|
+
# An array of valid keys in the options hash when configuring a DingBot::API.
|
5
|
+
VALID_OPTIONS_KEYS = [:endpoint, :access_token, :httparty, :secret].freeze
|
6
6
|
|
7
7
|
# The user agent that will be sent to the API endpoint if none is set.
|
8
8
|
DEFAULT_USER_AGENT = "DingBot Ruby Gem #{DingBot::VERSION}".freeze
|
@@ -34,6 +34,7 @@ module DingBot
|
|
34
34
|
def reset
|
35
35
|
self.endpoint = ENV['DINGTALK_ENDPOINT'] || DEFAULT_ENDPOINT
|
36
36
|
self.access_token = ENV['DINGTALK_ACCESS_TOKEN']
|
37
|
+
self.secret = ENV['DINGTALK_SECRET']
|
37
38
|
end
|
38
39
|
|
39
40
|
end
|
data/lib/dingbot/error.rb
CHANGED
@@ -36,23 +36,9 @@ module DingBot
|
|
36
36
|
message = parsed_response.message || parsed_response.error
|
37
37
|
|
38
38
|
"Server responded with code #{@response.code}, message: " \
|
39
|
-
"#{handle_message(message)}. " \
|
40
39
|
"Request URI: #{@response.request.base_uri}#{@response.request.path}"
|
41
40
|
end
|
42
41
|
|
43
|
-
# Handle error response message in case of nested hashes
|
44
|
-
def handle_message(message)
|
45
|
-
case message
|
46
|
-
when SentryApi::ObjectifiedHash
|
47
|
-
message.to_h.sort.map do |key, val|
|
48
|
-
"'#{key}' #{(val.is_a?(Hash) ? val.sort.map {|k, v| "(#{k}: #{v.join(' ')})"} : val).join(' ')}"
|
49
|
-
end.join(', ')
|
50
|
-
when Array
|
51
|
-
message.join(' ')
|
52
|
-
else
|
53
|
-
message
|
54
|
-
end
|
55
|
-
end
|
56
42
|
end
|
57
43
|
|
58
44
|
# Raised when API endpoint returns the HTTP status code 400.
|
@@ -2,14 +2,35 @@ module DingBot
|
|
2
2
|
module Message
|
3
3
|
|
4
4
|
# ActionCard基类
|
5
|
+
# {
|
6
|
+
# "msgtype": "actionCard",
|
7
|
+
# "actionCard": {
|
8
|
+
# "title": "乔布斯 20 年前想打造一间苹果咖啡厅,而它正是 Apple Store 的前身",
|
9
|
+
# "text": "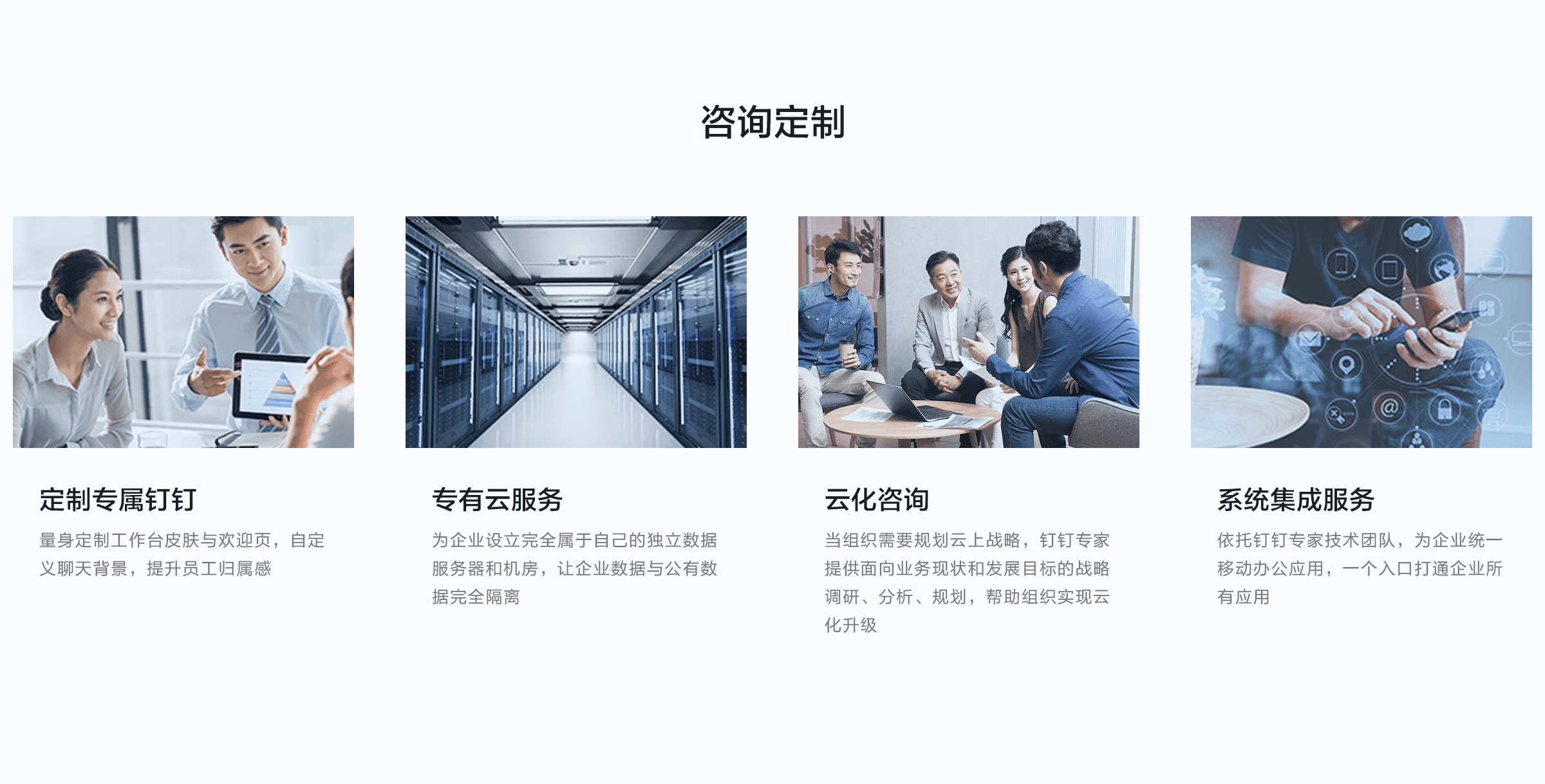 \n\n #### 乔布斯 20 年前想打造的苹果咖啡厅 \n\n Apple Store 的设计正从原来满满的科技感走向生活化,而其生活化的走向其实可以追溯到 20 年前苹果一个建立咖啡馆的计划",
|
10
|
+
# "btnOrientation": "0",
|
11
|
+
# "btns": [
|
12
|
+
# {
|
13
|
+
# "title": "内容不错",
|
14
|
+
# "actionURL": "https://www.dingtalk.com/"
|
15
|
+
# },
|
16
|
+
# {
|
17
|
+
# "title": "不感兴趣",
|
18
|
+
# "actionURL": "https://www.dingtalk.com/"
|
19
|
+
# }
|
20
|
+
# ]
|
21
|
+
# }
|
22
|
+
# "at":{
|
23
|
+
# "atMobiles":["137188xxxxx"]
|
24
|
+
# }
|
25
|
+
# }
|
5
26
|
class ActionCard < Base
|
6
|
-
attr_accessor :title, :text, :btn_orientation, :
|
27
|
+
attr_accessor :title, :text, :btn_orientation, :at_mobiles
|
7
28
|
|
8
|
-
def initialize(title='', text='', btn_orientation='0',
|
29
|
+
def initialize(title='', text='', btn_orientation='0', at_mobiles=[])
|
9
30
|
@title = title
|
10
31
|
@text = text
|
11
32
|
@btn_orientation = btn_orientation
|
12
|
-
@
|
33
|
+
@at_mobiles = at_mobiles
|
13
34
|
end
|
14
35
|
|
15
36
|
def msg_type
|
@@ -17,13 +38,16 @@ module DingBot
|
|
17
38
|
end
|
18
39
|
|
19
40
|
def body_params
|
20
|
-
super.merge(
|
41
|
+
super.merge({
|
21
42
|
actionCard: {
|
22
43
|
title: @title,
|
23
44
|
text: @text,
|
24
|
-
hideAvatar: @hide_avatar,
|
25
45
|
btnOrientation: @btn_orientation,
|
46
|
+
},
|
47
|
+
at: {
|
48
|
+
atMobiles: @at_mobiles,
|
26
49
|
}
|
50
|
+
}
|
27
51
|
)
|
28
52
|
end
|
29
53
|
end
|
@@ -32,8 +56,8 @@ module DingBot
|
|
32
56
|
class WholeActionCard < ActionCard
|
33
57
|
attr_accessor :single_title, :single_url
|
34
58
|
|
35
|
-
def initialize(title='', text='', single_title='', single_url='',
|
36
|
-
super(title, text, btn_orientation,
|
59
|
+
def initialize(title='', text='', single_title='', single_url='', at_mobiles=[], btn_orientation='0')
|
60
|
+
super(title, text, btn_orientation, at_mobiles)
|
37
61
|
@single_title = single_title
|
38
62
|
@single_url = single_url
|
39
63
|
end
|
@@ -53,8 +77,8 @@ module DingBot
|
|
53
77
|
class IndependentActionCard < ActionCard
|
54
78
|
attr_accessor :buttons
|
55
79
|
|
56
|
-
def initialize(title='', text='', buttons=[],
|
57
|
-
super(title, text, btn_orientation,
|
80
|
+
def initialize(title='', text='', buttons=[], at_mobiles=[], btn_orientation='0')
|
81
|
+
super(title, text, btn_orientation, at_mobiles)
|
58
82
|
@buttons = buttons
|
59
83
|
end
|
60
84
|
|
data/lib/dingbot/version.rb
CHANGED
data/lib/dingbot.rb
CHANGED
@@ -38,7 +38,7 @@ module DingBot
|
|
38
38
|
#
|
39
39
|
# @return [Array<Symbol>]
|
40
40
|
def self.actions
|
41
|
-
hidden = /access_token|post|validate|httparty/
|
41
|
+
hidden = /access_token|post|validate|httparty|secret/
|
42
42
|
(DingBot::Client.instance_methods - Object.methods).reject {|e| e[hidden]}
|
43
43
|
end
|
44
44
|
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: dingbot
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.2.
|
4
|
+
version: 0.2.5
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Thierry Xing
|
8
8
|
autorequire:
|
9
9
|
bindir: exe
|
10
10
|
cert_chain: []
|
11
|
-
date:
|
11
|
+
date: 2021-09-09 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: httparty
|
@@ -133,8 +133,7 @@ required_rubygems_version: !ruby/object:Gem::Requirement
|
|
133
133
|
- !ruby/object:Gem::Version
|
134
134
|
version: '0'
|
135
135
|
requirements: []
|
136
|
-
|
137
|
-
rubygems_version: 2.6.14
|
136
|
+
rubygems_version: 3.0.3
|
138
137
|
signing_key:
|
139
138
|
specification_version: 4
|
140
139
|
summary: 钉钉自定义机器人Ruby库
|