diff_matcher 2.0.1 → 2.1.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- data/.rspec +1 -0
- data/README.md +118 -5
- data/TODO.txt +14 -0
- data/doc/diff_matcher.gif +0 -0
- data/lib/diff_matcher/difference.rb +75 -27
- data/lib/diff_matcher/version.rb +1 -1
- data/spec/diff_matcher/difference_spec.rb +212 -31
- metadata +5 -3
data/.rspec
ADDED
@@ -0,0 +1 @@
|
|
1
|
+
--color
|
data/README.md
CHANGED
@@ -20,11 +20,11 @@ actual == expected # when expected is anything else
|
|
20
20
|
Example:
|
21
21
|
|
22
22
|
``` ruby
|
23
|
-
|
24
|
-
|
25
|
-
|
26
|
-
|
27
|
-
|
23
|
+
puts DiffMatcher::difference(
|
24
|
+
{ :a=>{ :a1=>11 }, :b=>[ 21, 22 ], :c=>/\d/, :d=>Fixnum, :e=>lambda { |x| (4..6).include? x } },
|
25
|
+
{ :a=>{ :a1=>10, :a2=>12 }, :b=>[ 21 ], :c=>'3' , :d=>4 , :e=>5 },
|
26
|
+
:color_scheme=>:white_background
|
27
|
+
)
|
28
28
|
```
|
29
29
|
|
30
30
|
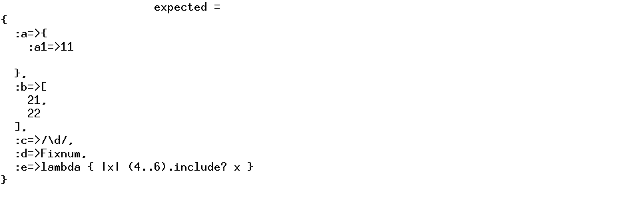
|
@@ -87,6 +87,7 @@ When `actual` is missing one of the `expected` values
|
|
87
87
|
``` ruby
|
88
88
|
puts DiffMatcher::difference([1, 2], [1])
|
89
89
|
# => [
|
90
|
+
# => 1
|
90
91
|
# => - 2
|
91
92
|
# => ]
|
92
93
|
# => Where, - 1 missing
|
@@ -97,11 +98,55 @@ When `actual` has additional values to the `expected`
|
|
97
98
|
``` ruby
|
98
99
|
puts DiffMatcher::difference([1], [1, 2])
|
99
100
|
# => [
|
101
|
+
# => 1
|
100
102
|
# => + 2
|
101
103
|
# => ]
|
102
104
|
# => Where, + 1 additional
|
103
105
|
```
|
104
106
|
|
107
|
+
|
108
|
+
When `expected` can take multiple forms use a `Matcher`
|
109
|
+
|
110
|
+
``` ruby
|
111
|
+
puts DiffMatcher::difference(DiffMatcher::Matcher[Fixnum,Float], "3")
|
112
|
+
- Float+ "3"
|
113
|
+
Where, - 1 missing, + 1 additional
|
114
|
+
```
|
115
|
+
|
116
|
+
|
117
|
+
When `actual` is an array of unknown size use an `AllMatcher` to match
|
118
|
+
against *all* the elements in the array.
|
119
|
+
|
120
|
+
``` ruby
|
121
|
+
puts DiffMatcher::difference(DiffMatcher::AllMatcher[Fixnum], [1, 2, "3"])
|
122
|
+
[
|
123
|
+
: 1,
|
124
|
+
: 2,
|
125
|
+
- Fixnum+ "3"
|
126
|
+
]
|
127
|
+
Where, - 1 missing, + 1 additional, : 2 match_class
|
128
|
+
```
|
129
|
+
|
130
|
+
|
131
|
+
When `actual` is an array of unknown size *and* `expected` can take
|
132
|
+
multiple forms use a `Matcher` inside of an `AllMatcher` to match
|
133
|
+
against *all* the elements in the array in any of the forms.
|
134
|
+
|
135
|
+
``` ruby
|
136
|
+
puts DiffMatcher::difference(
|
137
|
+
DiffMatcher::AllMatcher[
|
138
|
+
DiffMatcher::Matcher[Fixnum, Float]
|
139
|
+
],
|
140
|
+
[1, 2.00, "3"]
|
141
|
+
)
|
142
|
+
[
|
143
|
+
| 1,
|
144
|
+
| 2.0,
|
145
|
+
- Float+ "3"
|
146
|
+
]
|
147
|
+
Where, - 1 missing, + 1 additional, | 2 match_matcher
|
148
|
+
```
|
149
|
+
|
105
150
|
### Options
|
106
151
|
|
107
152
|
`:ignore_additional=>true` will match even if `actual` has additional items
|
@@ -182,6 +227,74 @@ DiffMatcher can match using not only regexes but classes and procs.
|
|
182
227
|
And the difference string that it outputs can be formatted in several ways as needed.
|
183
228
|
|
184
229
|
|
230
|
+
Use with rspec
|
231
|
+
---
|
232
|
+
To use with rspec create the following custom matcher:
|
233
|
+
|
234
|
+
``` ruby
|
235
|
+
require 'diff_matcher'
|
236
|
+
|
237
|
+
module RSpec
|
238
|
+
module Matchers
|
239
|
+
class BeMatching
|
240
|
+
include BaseMatcher
|
241
|
+
|
242
|
+
def initialize(expected, opts)
|
243
|
+
@expected = expected
|
244
|
+
@opts = opts.update(:color_enabled=>RSpec::configuration.color_enabled?)
|
245
|
+
end
|
246
|
+
|
247
|
+
def matches?(actual)
|
248
|
+
@difference = DiffMatcher::Difference.new(expected, actual, @opts)
|
249
|
+
@difference.matching?
|
250
|
+
end
|
251
|
+
|
252
|
+
def failure_message_for_should
|
253
|
+
@difference.to_s
|
254
|
+
end
|
255
|
+
end
|
256
|
+
|
257
|
+
def be_matching(expected, opts={})
|
258
|
+
Matchers::BeMatching.new(expected, opts)
|
259
|
+
end
|
260
|
+
end
|
261
|
+
end
|
262
|
+
```
|
263
|
+
|
264
|
+
And use it with:
|
265
|
+
|
266
|
+
``` ruby
|
267
|
+
describe "hash matcher" do
|
268
|
+
subject { { :a=>1, :b=>2, :c=>'3', :d=>4, :e=>"additional stuff" } }
|
269
|
+
let(:expected) { { :a=>1, :b=>Fixnum, :c=>/[0-9]/, :d=>lambda { |x| (3..5).include?(x) } } }
|
270
|
+
|
271
|
+
it { should be_matching(expected, :ignore_additional=>true) }
|
272
|
+
it { should be_matching(expected) }
|
273
|
+
end
|
274
|
+
```
|
275
|
+
|
276
|
+
Will result in:
|
277
|
+
|
278
|
+
```
|
279
|
+
Failures:
|
280
|
+
|
281
|
+
1) hash matcher
|
282
|
+
Failure/Error: it { should be_matching(expected) }
|
283
|
+
{
|
284
|
+
:a=>1,
|
285
|
+
:b=>: 2,
|
286
|
+
:c=>~ (3),
|
287
|
+
:d=>{ 4,
|
288
|
+
+ :e=>"additional stuff"
|
289
|
+
}
|
290
|
+
Where, + 1 additional, ~ 1 match_regexp, : 1 match_class, { 1 match_proc
|
291
|
+
# ./hash_matcher_spec.rb:6:in `block (2 levels) in <top (required)>'
|
292
|
+
|
293
|
+
Finished in 0.00601 seconds
|
294
|
+
2 examples, 1 failure
|
295
|
+
```
|
296
|
+
|
297
|
+
|
185
298
|
Contributing
|
186
299
|
---
|
187
300
|
|
data/TODO.txt
ADDED
@@ -0,0 +1,14 @@
|
|
1
|
+
Fri Aug 12 2011
|
2
|
+
- match against class Pattern < Hash which has an :optional_keys attr (or :required_keys?)
|
3
|
+
- array patterns with min/max ? (keys 0..n required?)
|
4
|
+
|
5
|
+
|
6
|
+
Also, the words `diff` and `match` seem to get thrown around and
|
7
|
+
interchanged a lot.
|
8
|
+
I think I should make it clear that `diff_matcher` was built as a differ
|
9
|
+
that outputs a textual diff based on what it matched.
|
10
|
+
ie. by matching `actual` against an `expected` value/pattern/class/proc.
|
11
|
+
|
12
|
+
Wed Dec 14 2011
|
13
|
+
- underline
|
14
|
+
- string diff
|
data/doc/diff_matcher.gif
CHANGED
Binary file
|
@@ -5,6 +5,50 @@ module DiffMatcher
|
|
5
5
|
difference.matching? ? nil : difference.to_s
|
6
6
|
end
|
7
7
|
|
8
|
+
class Matcher
|
9
|
+
attr_reader :expecteds
|
10
|
+
|
11
|
+
def self.[](*expected)
|
12
|
+
new(*expected)
|
13
|
+
end
|
14
|
+
|
15
|
+
def initialize(*expected)
|
16
|
+
@expecteds = [expected].flatten
|
17
|
+
@opts = {}
|
18
|
+
end
|
19
|
+
|
20
|
+
def |(other)
|
21
|
+
#"(#{expecteds.join(",")}|#{other.expecteds.join(",")})"
|
22
|
+
tap { @expecteds += other.expecteds }
|
23
|
+
end
|
24
|
+
|
25
|
+
def expected(expected, actual)
|
26
|
+
expected
|
27
|
+
end
|
28
|
+
|
29
|
+
def diff(actual, opts={})
|
30
|
+
dif = nil
|
31
|
+
@expecteds.any? { |e|
|
32
|
+
d = DiffMatcher::Difference.new(expected(e, actual), actual, opts)
|
33
|
+
dif = d.matching? ? nil : d.dif
|
34
|
+
d.matching?
|
35
|
+
}
|
36
|
+
dif
|
37
|
+
end
|
38
|
+
end
|
39
|
+
|
40
|
+
class NotAnArray < Exception; end
|
41
|
+
class AllMatcher < Matcher
|
42
|
+
def expected(expected, actual)
|
43
|
+
[expected]*actual.size
|
44
|
+
end
|
45
|
+
|
46
|
+
def diff(actual, opts={})
|
47
|
+
raise NotAnArray unless actual.is_a?(Array)
|
48
|
+
super
|
49
|
+
end
|
50
|
+
end
|
51
|
+
|
8
52
|
class Difference
|
9
53
|
RESET = "\e[0m"
|
10
54
|
BOLD = "\e[1m"
|
@@ -16,28 +60,25 @@ module DiffMatcher
|
|
16
60
|
MAGENTA = "\e[35m"
|
17
61
|
CYAN = "\e[36m"
|
18
62
|
|
19
|
-
|
20
|
-
:default=>{
|
63
|
+
DEFAULT_COLOR_SCHEME = {
|
21
64
|
:missing => [RED , "-"],
|
22
65
|
:additional => [YELLOW, "+"],
|
23
66
|
:match_value => [nil , nil],
|
24
67
|
:match_regexp => [GREEN , "~"],
|
25
68
|
:match_class => [BLUE , ":"],
|
69
|
+
:match_matcher => [BLUE , "|"],
|
26
70
|
:match_proc => [CYAN , "{"]
|
27
|
-
},
|
28
|
-
:white_background=> {
|
29
|
-
:missing => [RED , "-"],
|
30
|
-
:additional => [MAGENTA, "+"],
|
31
|
-
:match_value => [nil , nil],
|
32
|
-
:match_regexp => [GREEN , "~"],
|
33
|
-
:match_class => [BLUE , ":"],
|
34
|
-
:match_proc => [CYAN , "{"]
|
35
|
-
}
|
36
71
|
}
|
37
72
|
|
38
|
-
|
73
|
+
COLOR_SCHEMES = {
|
74
|
+
:default => DEFAULT_COLOR_SCHEME,
|
75
|
+
:white_background => DEFAULT_COLOR_SCHEME.merge(
|
76
|
+
:additional => [MAGENTA, "+"]
|
77
|
+
)
|
78
|
+
}
|
39
79
|
|
40
80
|
def initialize(expected, actual, opts={})
|
81
|
+
@opts = opts
|
41
82
|
@ignore_additional = opts[:ignore_additional]
|
42
83
|
@quiet = opts[:quiet]
|
43
84
|
@color_enabled = opts[:color_enabled] || !!opts[:color_scheme]
|
@@ -69,6 +110,10 @@ module DiffMatcher
|
|
69
110
|
end
|
70
111
|
end
|
71
112
|
|
113
|
+
def dif
|
114
|
+
@difference
|
115
|
+
end
|
116
|
+
|
72
117
|
private
|
73
118
|
|
74
119
|
def item_types
|
@@ -87,16 +132,16 @@ module DiffMatcher
|
|
87
132
|
@matches_shown ||= lambda {
|
88
133
|
ret = []
|
89
134
|
unless @quiet
|
90
|
-
ret += [:match_class, :match_proc, :match_regexp]
|
135
|
+
ret += [:match_matcher, :match_class, :match_proc, :match_regexp]
|
91
136
|
ret += [:match_value]
|
92
137
|
end
|
93
138
|
ret
|
94
139
|
}.call
|
95
140
|
end
|
96
141
|
|
97
|
-
def difference(expected, actual
|
142
|
+
def difference(expected, actual)
|
98
143
|
if actual.is_a? expected.class
|
99
|
-
left = diff(expected, actual
|
144
|
+
left = diff(expected, actual)
|
100
145
|
right = diff(actual, expected)
|
101
146
|
items_to_s(
|
102
147
|
expected,
|
@@ -105,18 +150,17 @@ module DiffMatcher
|
|
105
150
|
}
|
106
151
|
)
|
107
152
|
else
|
108
|
-
difference_to_s(expected, actual
|
153
|
+
difference_to_s(expected, actual)
|
109
154
|
end
|
110
155
|
end
|
111
156
|
|
112
|
-
def diff(expected, actual
|
157
|
+
def diff(expected, actual)
|
113
158
|
if expected.is_a?(Hash)
|
114
159
|
expected.keys.inject({}) { |h, k|
|
115
|
-
h.update(k => actual.has_key?(k) ? difference(actual[k], expected[k]
|
160
|
+
h.update(k => actual.has_key?(k) ? difference(actual[k], expected[k]) : expected[k])
|
116
161
|
}
|
117
162
|
elsif expected.is_a?(Array)
|
118
163
|
expected, actual = [expected, actual].map { |x| x.each_with_index.inject({}) { |h, (v, i)| h.update(i=>v) } }
|
119
|
-
#diff(expected, actual, reverse) # XXX - is there a test case for this?
|
120
164
|
diff(expected, actual)
|
121
165
|
else
|
122
166
|
actual
|
@@ -151,10 +195,13 @@ module DiffMatcher
|
|
151
195
|
|
152
196
|
def match?(expected, actual)
|
153
197
|
case expected
|
154
|
-
when
|
155
|
-
|
156
|
-
|
157
|
-
|
198
|
+
when Matcher
|
199
|
+
d = expected.diff(actual, @opts)
|
200
|
+
[d.nil? , :match_matcher, d]
|
201
|
+
when Class ; [actual.is_a?(expected) , :match_class ]
|
202
|
+
when Proc ; [expected.call(actual) , :match_proc ]
|
203
|
+
when Regexp ; [actual.is_a?(String) && actual.match(expected) , :match_regexp ]
|
204
|
+
else [actual == expected , :match_value ]
|
158
205
|
end
|
159
206
|
end
|
160
207
|
|
@@ -178,12 +225,13 @@ module DiffMatcher
|
|
178
225
|
markup(match_type, actual) if matches_shown.include?(match_type)
|
179
226
|
end
|
180
227
|
|
181
|
-
def difference_to_s(expected, actual
|
182
|
-
match, match_type = match?(
|
228
|
+
def difference_to_s(expected, actual)
|
229
|
+
match, match_type, d = match?(expected, actual)
|
183
230
|
if match
|
184
|
-
match_to_s(expected, actual, match_type)
|
231
|
+
match_to_s(expected, actual.inspect, match_type)
|
185
232
|
else
|
186
|
-
|
233
|
+
match_type == :match_matcher ? d :
|
234
|
+
"#{markup(:missing, expected.inspect)}#{markup(:additional, actual.inspect)}"
|
187
235
|
end
|
188
236
|
end
|
189
237
|
|
data/lib/diff_matcher/version.rb
CHANGED
@@ -12,34 +12,115 @@ def fix_EOF_problem(s)
|
|
12
12
|
s.gsub("\n#{" " * indentation}", "\n").strip
|
13
13
|
end
|
14
14
|
|
15
|
-
describe "DiffMatcher::difference(expected, actual, opts)" do
|
16
|
-
subject { DiffMatcher::difference(expected, actual, opts) }
|
17
15
|
|
18
|
-
|
19
|
-
|
20
|
-
|
21
|
-
|
22
|
-
|
23
|
-
|
24
|
-
|
16
|
+
shared_examples_for "a matcher" do |expected, expected2, same, different, difference, opts|
|
17
|
+
opts ||= {}
|
18
|
+
context "with #{opts.size > 0 ? opts_to_s(opts) : "no opts"}" do
|
19
|
+
describe "diff(#{same.inspect}#{opts_to_s(opts)})" do
|
20
|
+
let(:expected ) { expected }
|
21
|
+
let(:expected2) { expected }
|
22
|
+
let(:actual ) { same }
|
23
|
+
let(:opts ) { opts }
|
25
24
|
|
26
|
-
|
27
|
-
|
25
|
+
it { should be_nil }
|
26
|
+
end
|
28
27
|
|
29
|
-
|
30
|
-
|
31
|
-
|
32
|
-
|
33
|
-
|
34
|
-
|
35
|
-
|
36
|
-
|
37
|
-
should =~ difference :
|
38
|
-
should == fix_EOF_problem(difference)
|
39
|
-
} if RUBY_1_9
|
40
|
-
end
|
28
|
+
describe "diff(#{different.inspect}#{opts_to_s(opts)})" do
|
29
|
+
let(:expected ) { expected }
|
30
|
+
let(:expected2) { expected }
|
31
|
+
let(:actual ) { different }
|
32
|
+
let(:opts ) { opts }
|
33
|
+
|
34
|
+
it { should_not be_nil } unless RUBY_1_9
|
35
|
+
it { should == fix_EOF_problem(difference)+"\n" } if RUBY_1_9
|
41
36
|
end
|
42
37
|
end
|
38
|
+
end
|
39
|
+
|
40
|
+
|
41
|
+
describe DiffMatcher::Matcher do
|
42
|
+
expected, expected2, same, different, difference =
|
43
|
+
{:nombre => String , :edad => Integer },
|
44
|
+
{:name => String , :age => Integer },
|
45
|
+
{:name => "Peter" , :age => 21 },
|
46
|
+
{:name => 21 , :age => 21 },
|
47
|
+
<<-EOF
|
48
|
+
{
|
49
|
+
:name=>\e[31m- \e[1mString\e[0m\e[33m+ \e[1m21\e[0m,
|
50
|
+
:age=>\e[34m: \e[1m21\e[0m
|
51
|
+
}
|
52
|
+
EOF
|
53
|
+
|
54
|
+
describe "DiffMatcher::Matcher[expected, expected2]," do
|
55
|
+
subject { DiffMatcher::Matcher[expected, expected2].diff(actual) }
|
56
|
+
|
57
|
+
it_behaves_like "a matcher", expected, expected2, same, different, difference
|
58
|
+
end
|
59
|
+
|
60
|
+
describe "DiffMatcher::Matcher[expected] | DiffMatcher::Matcher[expected2])" do
|
61
|
+
subject { (DiffMatcher::Matcher[expected] | DiffMatcher::Matcher[expected2]).diff(actual) }
|
62
|
+
|
63
|
+
it_behaves_like "a matcher", expected, expected2, same, different, difference
|
64
|
+
end
|
65
|
+
end
|
66
|
+
|
67
|
+
|
68
|
+
describe "DiffMatcher::AllMatcher[expected]" do
|
69
|
+
let(:all_matcher) { DiffMatcher::AllMatcher[expected] }
|
70
|
+
let(:expected ) { 1 }
|
71
|
+
|
72
|
+
describe "#diff(actual)" do
|
73
|
+
subject { all_matcher.diff(actual) }
|
74
|
+
|
75
|
+
context "when all match" do
|
76
|
+
let(:actual) { [1, 1, 1] }
|
77
|
+
|
78
|
+
it { should eql nil }
|
79
|
+
end
|
80
|
+
|
81
|
+
context "when not all match" do
|
82
|
+
let(:actual) { [1, 2, 1] }
|
83
|
+
|
84
|
+
it { should eql "[\n 1,\n \e[31m- \e[1m1\e[0m\e[33m+ \e[1m2\e[0m,\n 1\n]\n" }
|
85
|
+
end
|
86
|
+
|
87
|
+
context "when actual is not an array" do
|
88
|
+
let(:actual) { 'a' }
|
89
|
+
|
90
|
+
it { expect { subject }.to raise_error(DiffMatcher::NotAnArray) }
|
91
|
+
end
|
92
|
+
end
|
93
|
+
end
|
94
|
+
|
95
|
+
|
96
|
+
shared_examples_for "a diff matcher" do |expected, same, different, difference, opts|
|
97
|
+
opts ||= {}
|
98
|
+
context "with #{opts.size > 0 ? opts_to_s(opts) : "no opts"}" do
|
99
|
+
describe "difference(#{expected.inspect}, #{same.inspect}#{opts_to_s(opts)})" do
|
100
|
+
let(:expected) { expected }
|
101
|
+
let(:actual ) { same }
|
102
|
+
let(:opts ) { opts }
|
103
|
+
|
104
|
+
it { should be_nil }
|
105
|
+
end
|
106
|
+
|
107
|
+
describe "difference(#{expected.inspect}, #{different.inspect}#{opts_to_s(opts)})" do
|
108
|
+
let(:expected) { expected }
|
109
|
+
let(:actual ) { different }
|
110
|
+
let(:opts ) { opts }
|
111
|
+
|
112
|
+
it { should_not be_nil } unless RUBY_1_9
|
113
|
+
it {
|
114
|
+
difference.is_a?(Regexp) ?
|
115
|
+
should =~ difference :
|
116
|
+
should == fix_EOF_problem(difference)
|
117
|
+
} if RUBY_1_9
|
118
|
+
end
|
119
|
+
end
|
120
|
+
end
|
121
|
+
|
122
|
+
describe "DiffMatcher::difference(expected, actual, opts)" do
|
123
|
+
subject { DiffMatcher::difference(expected, actual, opts) }
|
43
124
|
|
44
125
|
describe "when expected is an instance," do
|
45
126
|
context "of Fixnum," do
|
@@ -228,9 +309,7 @@ describe "DiffMatcher::difference(expected, actual, opts)" do
|
|
228
309
|
Where, - 1 missing, + 1 additional
|
229
310
|
EOF
|
230
311
|
end
|
231
|
-
end
|
232
312
|
|
233
|
-
describe "when expected is," do
|
234
313
|
context "a Regex," do
|
235
314
|
expected, same, different =
|
236
315
|
/[a-z]/,
|
@@ -253,9 +332,7 @@ describe "DiffMatcher::difference(expected, actual, opts)" do
|
|
253
332
|
EOF
|
254
333
|
end
|
255
334
|
end
|
256
|
-
end
|
257
335
|
|
258
|
-
describe "when expected is," do
|
259
336
|
context "a proc," do
|
260
337
|
expected, same, different =
|
261
338
|
lambda { |x| [FalseClass, TrueClass].include? x.class },
|
@@ -264,6 +341,110 @@ describe "DiffMatcher::difference(expected, actual, opts)" do
|
|
264
341
|
|
265
342
|
it_behaves_like "a diff matcher", expected, same, different,
|
266
343
|
/- #<Proc.*?>\+ \"true\"\nWhere, - 1 missing, \+ 1 additional/
|
344
|
+
|
345
|
+
context "that defines another diff matcher" do
|
346
|
+
expected, same, different =
|
347
|
+
lambda { |array| array.all? { |item| DiffMatcher::Difference.new(String, item).matching? } },
|
348
|
+
["A", "B", "C"],
|
349
|
+
["A", "B", 0 ]
|
350
|
+
|
351
|
+
it_behaves_like "a diff matcher", expected, same, different,
|
352
|
+
/- #<Proc.*?>\+ \[\"A\", \"B\", 0\]\nWhere, - 1 missing, \+ 1 additional/
|
353
|
+
end
|
354
|
+
end
|
355
|
+
|
356
|
+
context "a DiffMatcher::Matcher," do
|
357
|
+
expected, same, different =
|
358
|
+
DiffMatcher::Matcher[String],
|
359
|
+
"a",
|
360
|
+
1
|
361
|
+
|
362
|
+
it_behaves_like "a diff matcher", expected, same, different,
|
363
|
+
<<-EOF
|
364
|
+
- String+ 1
|
365
|
+
Where, - 1 missing, + 1 additional
|
366
|
+
EOF
|
367
|
+
|
368
|
+
context "or-ed with another DiffMatcher::Matcher," do
|
369
|
+
expected, same, different =
|
370
|
+
DiffMatcher::Matcher[Fixnum] | DiffMatcher::Matcher[String],
|
371
|
+
"a",
|
372
|
+
1.0
|
373
|
+
|
374
|
+
it_behaves_like "a diff matcher", expected, same, different,
|
375
|
+
<<-EOF
|
376
|
+
- String+ 1.0
|
377
|
+
Where, - 1 missing, + 1 additional
|
378
|
+
EOF
|
379
|
+
end
|
380
|
+
end
|
381
|
+
|
382
|
+
context "a DiffMatcher::AllMatcher," do
|
383
|
+
expected, same, different =
|
384
|
+
DiffMatcher::AllMatcher[String],
|
385
|
+
%w(ay be ci),
|
386
|
+
["a", 2, "c"]
|
387
|
+
|
388
|
+
it_behaves_like "a diff matcher", expected, same, different,
|
389
|
+
<<-EOF
|
390
|
+
[
|
391
|
+
: "a",
|
392
|
+
- String+ 2,
|
393
|
+
: "c"
|
394
|
+
]
|
395
|
+
Where, - 1 missing, + 1 additional, : 2 match_class
|
396
|
+
EOF
|
397
|
+
|
398
|
+
end
|
399
|
+
end
|
400
|
+
|
401
|
+
context "a DiffMatcher::AllMatcher using an or-ed DiffMatcher::Matcher," do
|
402
|
+
expected, same, different =
|
403
|
+
DiffMatcher::AllMatcher[ DiffMatcher::Matcher[Fixnum, Float] ],
|
404
|
+
[1, 2.0, 3],
|
405
|
+
[1, "2", 3]
|
406
|
+
|
407
|
+
it_behaves_like "a diff matcher", expected, same, different,
|
408
|
+
<<-EOF
|
409
|
+
[
|
410
|
+
| 1,
|
411
|
+
- Float+ "2",
|
412
|
+
| 3
|
413
|
+
]
|
414
|
+
Where, - 1 missing, + 1 additional, | 2 match_matcher
|
415
|
+
EOF
|
416
|
+
|
417
|
+
context "more complex", do
|
418
|
+
expected, same, different =
|
419
|
+
DiffMatcher::AllMatcher[
|
420
|
+
DiffMatcher::Matcher[
|
421
|
+
{nombre:String, edad:Fixnum},
|
422
|
+
{name:String, age:Fixnum}
|
423
|
+
]
|
424
|
+
],
|
425
|
+
[
|
426
|
+
{name: "Alice", age: 10},
|
427
|
+
{name: "Bob" , age: 20},
|
428
|
+
{name: "Con" , age: 30}
|
429
|
+
],
|
430
|
+
[
|
431
|
+
{name: "Alice", age: 10 },
|
432
|
+
{name: "Bob" , age: nil},
|
433
|
+
{nombre: "Con" , edad: 30 }
|
434
|
+
]
|
435
|
+
|
436
|
+
it_behaves_like "a diff matcher", expected, same, different,
|
437
|
+
<<-EOF
|
438
|
+
[
|
439
|
+
| {:name=>"Alice", :age=>10},
|
440
|
+
{
|
441
|
+
:name=>: "Bob",
|
442
|
+
:age=>- Fixnum+ nil
|
443
|
+
},
|
444
|
+
| {:nombre=>"Con", :edad=>30}
|
445
|
+
]
|
446
|
+
Where, - 1 missing, + 1 additional, : 1 match_class, | 2 match_matcher
|
447
|
+
EOF
|
267
448
|
end
|
268
449
|
end
|
269
450
|
|
@@ -279,7 +460,7 @@ describe "DiffMatcher::difference(expected, actual, opts)" do
|
|
279
460
|
[
|
280
461
|
- 1+ 0,
|
281
462
|
2,
|
282
|
-
~ (3),
|
463
|
+
~ "(3)",
|
283
464
|
: 4,
|
284
465
|
{ 5
|
285
466
|
]
|
@@ -303,7 +484,7 @@ describe "DiffMatcher::difference(expected, actual, opts)" do
|
|
303
484
|
[
|
304
485
|
- 1+ 0,
|
305
486
|
2,
|
306
|
-
~ (3),
|
487
|
+
~ "(3)",
|
307
488
|
: 4,
|
308
489
|
{ 5
|
309
490
|
]
|
@@ -317,7 +498,7 @@ describe "DiffMatcher::difference(expected, actual, opts)" do
|
|
317
498
|
\e[0m[
|
318
499
|
\e[0m \e[31m- \e[1m1\e[0m\e[33m+ \e[1m0\e[0m,
|
319
500
|
\e[0m 2,
|
320
|
-
\e[0m \e[32m~ \e[0m\e[32m(\e[1m3\e[0m\e[32m)\e[0m\e[0m,
|
501
|
+
\e[0m \e[32m~ \e[0m"\e[32m(\e[1m3\e[0m\e[32m)\e[0m"\e[0m,
|
321
502
|
\e[0m \e[34m: \e[1m4\e[0m,
|
322
503
|
\e[0m \e[36m{ \e[1m5\e[0m
|
323
504
|
\e[0m]
|
@@ -330,7 +511,7 @@ describe "DiffMatcher::difference(expected, actual, opts)" do
|
|
330
511
|
\e[0m[
|
331
512
|
\e[0m \e[31m- \e[1m1\e[0m\e[35m+ \e[1m0\e[0m,
|
332
513
|
\e[0m 2,
|
333
|
-
\e[0m \e[32m~ \e[0m\e[32m(\e[1m3\e[0m\e[32m)\e[0m\e[0m,
|
514
|
+
\e[0m \e[32m~ \e[0m"\e[32m(\e[1m3\e[0m\e[32m)\e[0m"\e[0m,
|
334
515
|
\e[0m \e[34m: \e[1m4\e[0m,
|
335
516
|
\e[0m \e[36m{ \e[1m5\e[0m
|
336
517
|
\e[0m]
|
metadata
CHANGED
@@ -2,7 +2,7 @@
|
|
2
2
|
name: diff_matcher
|
3
3
|
version: !ruby/object:Gem::Version
|
4
4
|
prerelease:
|
5
|
-
version: 2.0
|
5
|
+
version: 2.1.0
|
6
6
|
platform: ruby
|
7
7
|
authors:
|
8
8
|
- Playup
|
@@ -10,7 +10,7 @@ autorequire:
|
|
10
10
|
bindir: bin
|
11
11
|
cert_chain: []
|
12
12
|
|
13
|
-
date:
|
13
|
+
date: 2012-01-13 00:00:00 +11:00
|
14
14
|
default_executable:
|
15
15
|
dependencies: []
|
16
16
|
|
@@ -32,12 +32,14 @@ extensions: []
|
|
32
32
|
extra_rdoc_files: []
|
33
33
|
|
34
34
|
files:
|
35
|
+
- .rspec
|
35
36
|
- .travis.yml
|
36
37
|
- CHANGELOG.md
|
37
38
|
- Gemfile
|
38
39
|
- License.txt
|
39
40
|
- README.md
|
40
41
|
- Rakefile
|
42
|
+
- TODO.txt
|
41
43
|
- diff_matcher.gemspec
|
42
44
|
- doc/diff_matcher.gif
|
43
45
|
- doc/example_output.png
|
@@ -60,7 +62,7 @@ required_ruby_version: !ruby/object:Gem::Requirement
|
|
60
62
|
requirements:
|
61
63
|
- - ">="
|
62
64
|
- !ruby/object:Gem::Version
|
63
|
-
hash: -
|
65
|
+
hash: -331059827
|
64
66
|
segments:
|
65
67
|
- 0
|
66
68
|
version: "0"
|