cocoacache 0.1.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +7 -0
- data/LICENSE +21 -0
- data/README.md +76 -0
- data/bin/cocoacache +6 -0
- data/lib/cocoacache.rb +6 -0
- data/lib/cocoacache/command.rb +69 -0
- data/lib/cocoacache/core.rb +59 -0
- data/lib/cocoacache/version.rb +3 -0
- metadata +65 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA256:
|
3
|
+
metadata.gz: 79c19952c9762997d1df2764df553418753291713e056fb46ac78f4f475aaa52
|
4
|
+
data.tar.gz: dc8dd66718d9397267977d9b5a45fdeb64415cbbc1e8bbdd4222c7e5af84ea02
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: dd858d97aa764dedcb54f8d98a0934c65c496abb36184d6f1a1a348569fa08dac3c1395b90959d598d46eb2633754c77f85bc9fac124e5ff981e6c3dc105da4c
|
7
|
+
data.tar.gz: be5221d30aac81c3b9c1a5cdfd9ab365640a8e559df07a7f29d0c34515832e87af8eecca181ebfb23ce69b059fba41a31470ef9602c4a1b9afe546fb1c736f0d
|
data/LICENSE
ADDED
@@ -0,0 +1,21 @@
|
|
1
|
+
The MIT License (MIT)
|
2
|
+
|
3
|
+
Copyright (c) 2019 Suyeol Jeon (xoul.kr)
|
4
|
+
|
5
|
+
Permission is hereby granted, free of charge, to any person obtaining a copy
|
6
|
+
of this software and associated documentation files (the "Software"), to deal
|
7
|
+
in the Software without restriction, including without limitation the rights
|
8
|
+
to use, copy, modify, merge, publish, distribute, sublicense, and/or sell
|
9
|
+
copies of the Software, and to permit persons to whom the Software is
|
10
|
+
furnished to do so, subject to the following conditions:
|
11
|
+
|
12
|
+
The above copyright notice and this permission notice shall be included in all
|
13
|
+
copies or substantial portions of the Software.
|
14
|
+
|
15
|
+
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR
|
16
|
+
IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY,
|
17
|
+
FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE
|
18
|
+
AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER
|
19
|
+
LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM,
|
20
|
+
OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE
|
21
|
+
SOFTWARE.
|
data/README.md
ADDED
@@ -0,0 +1,76 @@
|
|
1
|
+
# CocoaCache
|
2
|
+
|
3
|
+
[](https://rubygems.org/gems/cocoacache)
|
4
|
+
[](https://travis-ci.org/devxoul/CocoaCache)
|
5
|
+
[](https://codecov.io/gh/devxoul/CocoaCache)
|
6
|
+
|
7
|
+
Partial CocoaPods Specs cache for the faster CI build.
|
8
|
+
|
9
|
+
## Background
|
10
|
+
|
11
|
+
It takes several minutes updating CocoaPods Specs repository while building a project on a CI server. In order to prevent from updating Specs repository, you have to cache the entire Specs repository located in `~/.coccoapods/repos/master`. But this is too large to cache in the CI server. CocoaCache helps to cache specific Pod specs to prevent from updating the Specs repository.
|
12
|
+
|
13
|
+
## Concepts
|
14
|
+
|
15
|
+
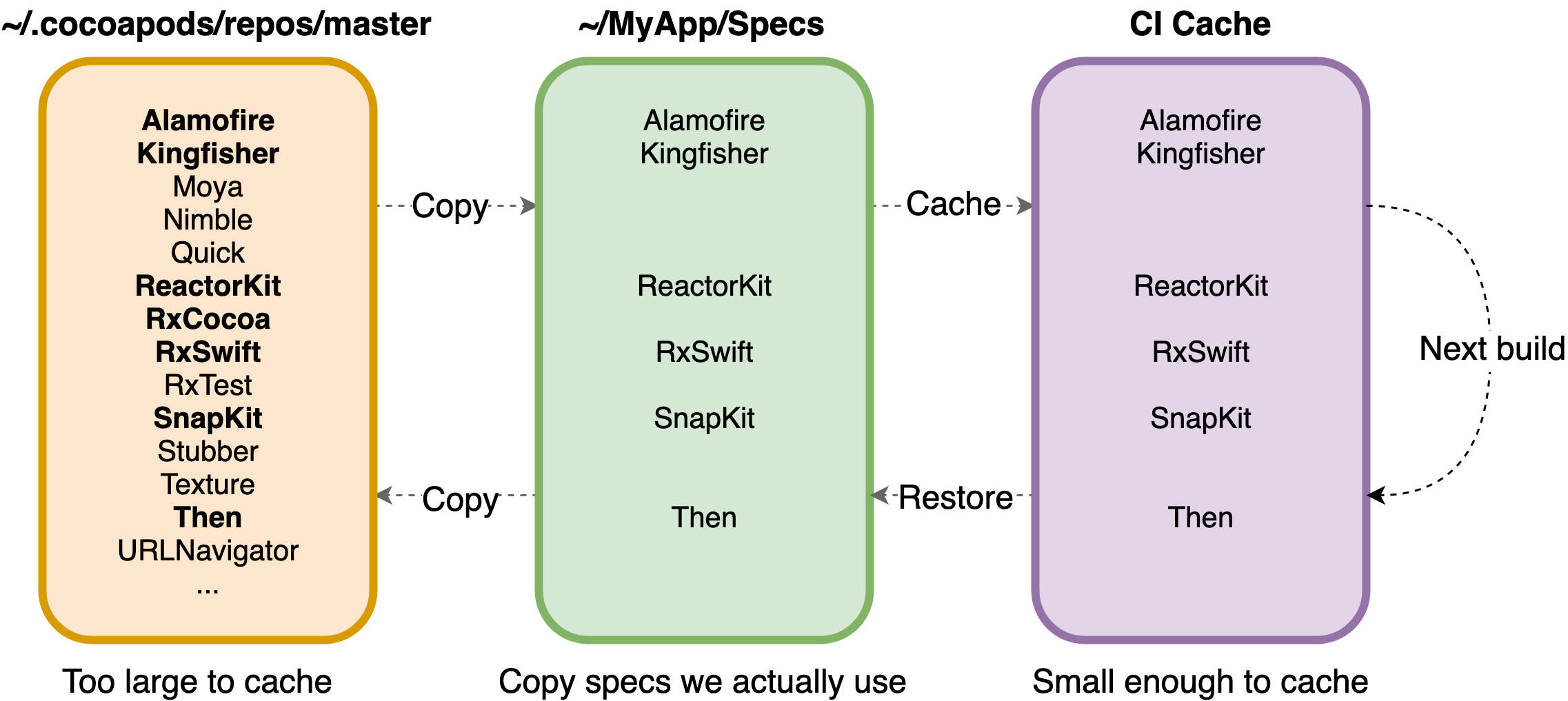
|
16
|
+
|
17
|
+
### Saving Cache (Previous Build)
|
18
|
+
|
19
|
+
1. Find Pods from `Podfile.lock` to cache.
|
20
|
+
2. Copy the specific Specs from the origin Specs directory to `./Specs`.
|
21
|
+
```
|
22
|
+
$HOME/.cocoapods/repos/master/Specs/7/7/7/ReactorKit -> ./Specs/7/7/7/ReactorKit
|
23
|
+
```
|
24
|
+
3. Cache `./Specs` to the CI server.
|
25
|
+
|
26
|
+
### Restoring Cache (Next Build)
|
27
|
+
|
28
|
+
1. Restore the `./Spec` directory from the CI server.
|
29
|
+
2. Find Pods from `Podfile.lock` to restore.
|
30
|
+
3. Copy the cached Specs back to the origin Specs directory from `./Specs`.
|
31
|
+
```
|
32
|
+
./Specs/7/7/7/ReactorKit -> $HOME/.cocoapods/repos/master/Specs/7/7/7/ReactorKit
|
33
|
+
```
|
34
|
+
|
35
|
+
## Installation
|
36
|
+
|
37
|
+
```console
|
38
|
+
$ gem install cocoacache
|
39
|
+
```
|
40
|
+
|
41
|
+
## Usage
|
42
|
+
|
43
|
+
```
|
44
|
+
Usage: cocoacache COMMAND [options]
|
45
|
+
|
46
|
+
Commands:
|
47
|
+
save Copy specs from the origin Specs to cache directory.
|
48
|
+
restore Copy the cached Specs back to the origin directory.
|
49
|
+
|
50
|
+
Options:
|
51
|
+
--origin <value> The origin Specs directory. Defaults to $HOME/.cocoapods/repos/master/Specs
|
52
|
+
--cache <value> Where to cache the Specs. Defaults to ~/Specs
|
53
|
+
--podfile <value> The path for the Podfile.lock. Defaults to ~/Podfile.lock
|
54
|
+
```
|
55
|
+
|
56
|
+
## Configuration
|
57
|
+
|
58
|
+
### Travis
|
59
|
+
|
60
|
+
**`.travis.yml`**
|
61
|
+
|
62
|
+
```yml
|
63
|
+
cache:
|
64
|
+
- directories:
|
65
|
+
- Specs
|
66
|
+
|
67
|
+
before_install:
|
68
|
+
- ruby scripts/cache_cocoapods.rb restore
|
69
|
+
|
70
|
+
before_cache:
|
71
|
+
- ruby scripts/cache_cocoapods.rb save
|
72
|
+
```
|
73
|
+
|
74
|
+
## License
|
75
|
+
|
76
|
+
**CocoaCache** is under MIT license. See the [LICENSE] file for more info.
|
data/bin/cocoacache
ADDED
data/lib/cocoacache.rb
ADDED
@@ -0,0 +1,69 @@
|
|
1
|
+
require "colored2"
|
2
|
+
|
3
|
+
module CocoaCache
|
4
|
+
class Command
|
5
|
+
def self.run(core_factory, argv)
|
6
|
+
case argv[0]
|
7
|
+
when "save"
|
8
|
+
core = self.get_core(core_factory, argv)
|
9
|
+
core.save()
|
10
|
+
|
11
|
+
when "restore"
|
12
|
+
core = self.get_core(core_factory, argv)
|
13
|
+
core.restore()
|
14
|
+
|
15
|
+
when "--version"
|
16
|
+
puts CocoaCache::VERSION
|
17
|
+
|
18
|
+
else
|
19
|
+
self.help
|
20
|
+
end
|
21
|
+
end
|
22
|
+
|
23
|
+
def self.get_core(factory, argv)
|
24
|
+
return factory.new(
|
25
|
+
:origin_specs_dir => (
|
26
|
+
self.parse_argument(argv, "--origin") \
|
27
|
+
or "$HOME/.cocoapods/repos/master/Specs"
|
28
|
+
),
|
29
|
+
:cache_specs_dir => (
|
30
|
+
self.parse_argument(argv, "--cache") \
|
31
|
+
or "Specs"
|
32
|
+
),
|
33
|
+
:podfile_path => (
|
34
|
+
self.parse_argument(argv, "--podfile") \
|
35
|
+
or "Podfile.lock"
|
36
|
+
),
|
37
|
+
)
|
38
|
+
end
|
39
|
+
|
40
|
+
def self.help
|
41
|
+
puts <<~HELP
|
42
|
+
Usage: cocoacache COMMAND [options]
|
43
|
+
|
44
|
+
Commands:
|
45
|
+
save Copy specs from the origin Specs to cache directory.
|
46
|
+
restore Copy the cached Specs back to the origin directory.
|
47
|
+
|
48
|
+
Options:
|
49
|
+
--origin <value> The origin Specs directory. Defaults to $HOME/.cocoapods/repos/master/Specs
|
50
|
+
--cache <value> Where to cache the Specs. Defaults to ~/Specs
|
51
|
+
--podfile <value> The path for the Podfile.lock. Defaults to ~/Podfile.lock
|
52
|
+
HELP
|
53
|
+
end
|
54
|
+
|
55
|
+
def self.parse_argument(argv, name)
|
56
|
+
index = argv.index(name)
|
57
|
+
if index.nil?
|
58
|
+
return nil
|
59
|
+
end
|
60
|
+
|
61
|
+
value = argv[index + 1]
|
62
|
+
if value.nil? or value.start_with?('--')
|
63
|
+
raise Exception("[!] Insufficient value for option '#{name}'".red)
|
64
|
+
end
|
65
|
+
|
66
|
+
return value
|
67
|
+
end
|
68
|
+
end
|
69
|
+
end
|
@@ -0,0 +1,59 @@
|
|
1
|
+
require "digest"
|
2
|
+
require "yaml"
|
3
|
+
|
4
|
+
module CocoaCache
|
5
|
+
class Core
|
6
|
+
attr_accessor :mute
|
7
|
+
|
8
|
+
def initialize(origin_specs_dir:, cache_specs_dir:, podfile_path:)
|
9
|
+
@origin_specs_dir = origin_specs_dir
|
10
|
+
@cache_specs_dir = cache_specs_dir
|
11
|
+
@podfile_path = podfile_path
|
12
|
+
end
|
13
|
+
|
14
|
+
def save()
|
15
|
+
pods = get_pods()
|
16
|
+
pods.each do |pod|
|
17
|
+
origin_path = get_origin_path(pod)
|
18
|
+
cache_path = get_cache_path(pod)
|
19
|
+
copy_dir(:from => origin_path, :to => cache_path)
|
20
|
+
end
|
21
|
+
end
|
22
|
+
|
23
|
+
def restore()
|
24
|
+
pods = get_pods()
|
25
|
+
pods.each do |pod|
|
26
|
+
origin_path = get_origin_path(pod)
|
27
|
+
cache_path = get_cache_path(pod)
|
28
|
+
copy_dir(:from => cache_path, :to => origin_path)
|
29
|
+
end
|
30
|
+
end
|
31
|
+
|
32
|
+
def copy_dir(from:, to:)
|
33
|
+
log "Copy #{from} -> #{to}"
|
34
|
+
`mkdir -p #{to} && cp -R #{from} #{to}/../`
|
35
|
+
end
|
36
|
+
|
37
|
+
def get_pods()
|
38
|
+
lockfile = YAML.load(File.read(@podfile_path))
|
39
|
+
pods = lockfile["SPEC REPOS"]["https://github.com/cocoapods/specs.git"]
|
40
|
+
return pods
|
41
|
+
end
|
42
|
+
|
43
|
+
def get_origin_path(pod)
|
44
|
+
return File.join(@origin_specs_dir, *get_shard_prefixes(pod), pod)
|
45
|
+
end
|
46
|
+
|
47
|
+
def get_cache_path(pod)
|
48
|
+
return File.join(@cache_specs_dir, *get_shard_prefixes(pod), pod)
|
49
|
+
end
|
50
|
+
|
51
|
+
def get_shard_prefixes(pod)
|
52
|
+
return Digest::MD5.hexdigest(pod)[0...3].split("")
|
53
|
+
end
|
54
|
+
|
55
|
+
def log(*strings)
|
56
|
+
puts strings.join(" ") if not @mute
|
57
|
+
end
|
58
|
+
end
|
59
|
+
end
|
metadata
ADDED
@@ -0,0 +1,65 @@
|
|
1
|
+
--- !ruby/object:Gem::Specification
|
2
|
+
name: cocoacache
|
3
|
+
version: !ruby/object:Gem::Version
|
4
|
+
version: 0.1.0
|
5
|
+
platform: ruby
|
6
|
+
authors:
|
7
|
+
- Suyeol Jeon
|
8
|
+
autorequire:
|
9
|
+
bindir: bin
|
10
|
+
cert_chain: []
|
11
|
+
date: 2019-06-25 00:00:00.000000000 Z
|
12
|
+
dependencies:
|
13
|
+
- !ruby/object:Gem::Dependency
|
14
|
+
name: colored2
|
15
|
+
requirement: !ruby/object:Gem::Requirement
|
16
|
+
requirements:
|
17
|
+
- - "~>"
|
18
|
+
- !ruby/object:Gem::Version
|
19
|
+
version: '3.1'
|
20
|
+
type: :runtime
|
21
|
+
prerelease: false
|
22
|
+
version_requirements: !ruby/object:Gem::Requirement
|
23
|
+
requirements:
|
24
|
+
- - "~>"
|
25
|
+
- !ruby/object:Gem::Version
|
26
|
+
version: '3.1'
|
27
|
+
description: Partial CocoaPods Specs cache for the faster CI build. It helps to cache
|
28
|
+
specific Pod specs to prevent from updatingthe Specs repository.
|
29
|
+
email: devxoul@gmail.com
|
30
|
+
executables:
|
31
|
+
- cocoacache
|
32
|
+
extensions: []
|
33
|
+
extra_rdoc_files: []
|
34
|
+
files:
|
35
|
+
- LICENSE
|
36
|
+
- README.md
|
37
|
+
- bin/cocoacache
|
38
|
+
- lib/cocoacache.rb
|
39
|
+
- lib/cocoacache/command.rb
|
40
|
+
- lib/cocoacache/core.rb
|
41
|
+
- lib/cocoacache/version.rb
|
42
|
+
homepage: https://github.com/devxoul/CocoaCache
|
43
|
+
licenses:
|
44
|
+
- MIT
|
45
|
+
metadata: {}
|
46
|
+
post_install_message:
|
47
|
+
rdoc_options: []
|
48
|
+
require_paths:
|
49
|
+
- lib
|
50
|
+
required_ruby_version: !ruby/object:Gem::Requirement
|
51
|
+
requirements:
|
52
|
+
- - ">="
|
53
|
+
- !ruby/object:Gem::Version
|
54
|
+
version: 2.2.2
|
55
|
+
required_rubygems_version: !ruby/object:Gem::Requirement
|
56
|
+
requirements:
|
57
|
+
- - ">="
|
58
|
+
- !ruby/object:Gem::Version
|
59
|
+
version: '0'
|
60
|
+
requirements: []
|
61
|
+
rubygems_version: 3.0.3
|
62
|
+
signing_key:
|
63
|
+
specification_version: 4
|
64
|
+
summary: Partial CocoaPods Specs cache for the faster CI build
|
65
|
+
test_files: []
|