c64asm 0.4.1
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +7 -0
- data/.gitignore +4 -0
- data/.yardopts +4 -0
- data/LICENSE.txt +23 -0
- data/README.md +126 -0
- data/Rakefile +6 -0
- data/c64asm.gemspec +19 -0
- data/examples/04.png +0 -0
- data/examples/04.prg +0 -0
- data/examples/hello_world.png +0 -0
- data/examples/hello_world.rb +30 -0
- data/lib/c64asm/asm.rb +751 -0
- data/lib/c64asm/basic.rb +132 -0
- data/lib/c64asm/data.rb +221 -0
- data/lib/c64asm/version.rb +7 -0
- data/lib/c64asm.rb +5 -0
- data/tools/make-data.rb +80 -0
- metadata +64 -0
checksums.yaml
ADDED
@@ -0,0 +1,7 @@
|
|
1
|
+
---
|
2
|
+
SHA1:
|
3
|
+
metadata.gz: 0da94793e9c3a04fdfd00747a09e1fb494c34f67
|
4
|
+
data.tar.gz: e85f96c230799224cee34a7a72d77f8d0c781bd8
|
5
|
+
SHA512:
|
6
|
+
metadata.gz: 515a5a8ed205f58676f5ace0c58a2ee1b465f508b2b32f2957f43540c2071d4d651daa55274e9b0635bf11dc125726630b058b1206b1f72c75d879c34bbfb7a2
|
7
|
+
data.tar.gz: 1705421b94cd83b4e8ebe53d3ccf402ea779ec09bd440ad48d27457a94c57381e0c7f2fd5773deabc6d51b9a84a78bdefb89af7224c491af449da94031681ff6
|
data/.gitignore
ADDED
data/.yardopts
ADDED
data/LICENSE.txt
ADDED
@@ -0,0 +1,23 @@
|
|
1
|
+
Copyright (c) 2012 - 2014, Piotr S. Staszewski
|
2
|
+
All rights reserved.
|
3
|
+
|
4
|
+
Redistribution and use in source and binary forms, with or without
|
5
|
+
modification, are permitted provided that the following conditions are met:
|
6
|
+
|
7
|
+
1. Redistributions of source code must retain the above copyright notice, this
|
8
|
+
list of conditions and the following disclaimer.
|
9
|
+
|
10
|
+
2. Redistributions in binary form must reproduce the above copyright notice,
|
11
|
+
this list of conditions and the following disclaimer in the documentation
|
12
|
+
and/or other materials provided with the distribution.
|
13
|
+
|
14
|
+
THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
|
15
|
+
ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
|
16
|
+
WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
|
17
|
+
DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE
|
18
|
+
FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
|
19
|
+
DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
|
20
|
+
SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER
|
21
|
+
CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY,
|
22
|
+
OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
|
23
|
+
OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
|
data/README.md
ADDED
@@ -0,0 +1,126 @@
|
|
1
|
+
# c64asm [](http://rubydoc.info/gems/c64asm/frames)
|
2
|
+
|
3
|
+
A MOS6502 assembler as a Ruby DLS, with focus on the Commodore 64.
|
4
|
+
|
5
|
+
Features:
|
6
|
+
|
7
|
+
- You write MOS6502 assembly naturally but still have full power of Ruby (it's awesome)
|
8
|
+
- Macros with variables as the basic building block
|
9
|
+
- C64 BASIC compiler built in
|
10
|
+
- Data driven with a generating tool, I *didn't write* data.rb
|
11
|
+
- Can output a source code representation (that I believe I've based upon some well known and established assembler)
|
12
|
+
- Can output a disassembler-like output, useful for cycle counting
|
13
|
+
|
14
|
+
Bugs:
|
15
|
+
- No test suite
|
16
|
+
- I believe my 'scrape opcodes table' approach missed some edge cases
|
17
|
+
|
18
|
+
Todo:
|
19
|
+
- More C64 helpers, e.g. bank switching helpers and a hash of named addresses
|
20
|
+
- Not far away from adding a basic CPU simulator (note: this is far from an emulator)
|
21
|
+
|
22
|
+
*I've written the bulk of it more than two years ago. I got to a rather cosy state of overall functionality, but I believe it misses some edge-cases. Please feel free to go nuts hacking it!*
|
23
|
+
|
24
|
+
# Showcase
|
25
|
+
|
26
|
+
Got the gem, in the examples folder:
|
27
|
+
|
28
|
+
$ cat hello_world.rb
|
29
|
+
#!/usr/bin/env ruby
|
30
|
+
# encoding: utf-8
|
31
|
+
# See LICENSE.txt for licensing information.
|
32
|
+
|
33
|
+
$LOAD_PATH.unshift(File.dirname(__FILE__) + '/../lib')
|
34
|
+
require 'c64asm'
|
35
|
+
|
36
|
+
hello_world = C64Asm::Macro.new do
|
37
|
+
block C64Asm::Basic.new('10 sys 49153').code
|
38
|
+
|
39
|
+
align 0xc000 # 49153
|
40
|
+
jsr 0xe544 # clear
|
41
|
+
ldx.d 0 # string index
|
42
|
+
label :load
|
43
|
+
lda.ax :msg # load character
|
44
|
+
cmp.d 35 # hash is the end of line
|
45
|
+
beq :finish # if so we're done
|
46
|
+
jsr 0xffd2 # chrout
|
47
|
+
inx # increment index
|
48
|
+
jmp :load # if not load the next character
|
49
|
+
label :finish
|
50
|
+
rts # back to basic(s)
|
51
|
+
label :msg
|
52
|
+
data "HELLO WORLD#"
|
53
|
+
end
|
54
|
+
|
55
|
+
code = hello_world.call # compile the macro
|
56
|
+
puts code.dump # print detailed
|
57
|
+
#puts code.to_source # just the source code
|
58
|
+
code.write!('hello_world.prg') # save to a standard .prg file
|
59
|
+
|
60
|
+
|
61
|
+
Let's run it:
|
62
|
+
|
63
|
+
$ ./hello_world.rb
|
64
|
+
$0801 .block
|
65
|
+
$0801 .block
|
66
|
+
$0801 * = $801
|
67
|
+
$0801 .. .. .. .byte $3,$8,$a,$0,$9e,$20,$34,$39,$31,$35,$33,$0,$0,$0
|
68
|
+
$080f .bend
|
69
|
+
$080F * = $c000
|
70
|
+
$C000 20 44 E5 jsr $e544
|
71
|
+
$C003 A2 00 ldx #$0
|
72
|
+
$C005 load
|
73
|
+
$C005 BD 14 C0 lda msg,x
|
74
|
+
$C008 C9 23 cmp #$23
|
75
|
+
$C00A F0 07 beq finish
|
76
|
+
$C00C 20 D2 FF jsr $ffd2
|
77
|
+
$C00F E8 inx
|
78
|
+
$C010 4C 05 C0 jmp load
|
79
|
+
$C013 finish
|
80
|
+
$C013 60 rts
|
81
|
+
$C014 msg
|
82
|
+
$C014 .. .. .. .text "HELLO WORLD#"
|
83
|
+
$c020 .bend
|
84
|
+
|
85
|
+
And run the compiled .prg with VICE:
|
86
|
+
|
87
|
+
$ x64 hello_world.prg
|
88
|
+
|
89
|
+
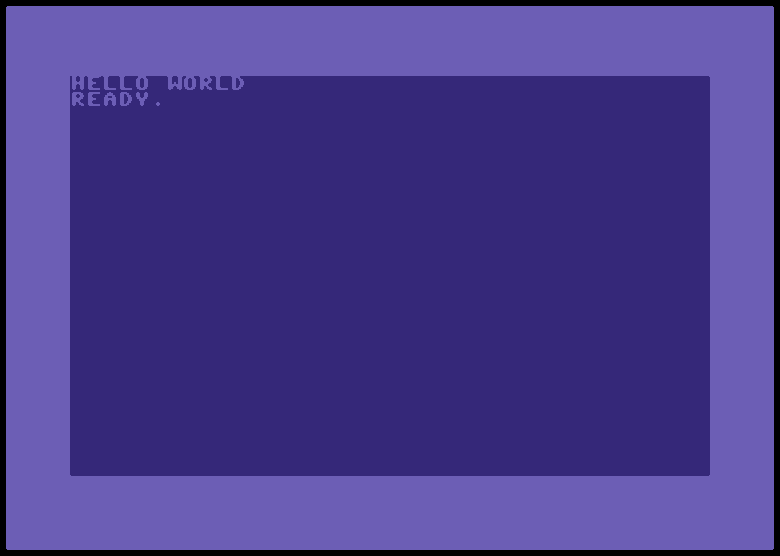
|
90
|
+
|
91
|
+
- - -
|
92
|
+
|
93
|
+
And an old demo screenshot, source not distributed 'cause it sucks, but it does compile under this gem:
|
94
|
+
|
95
|
+
$ ruby 04.rb.3
|
96
|
+
$ x64 04.prg
|
97
|
+
|
98
|
+
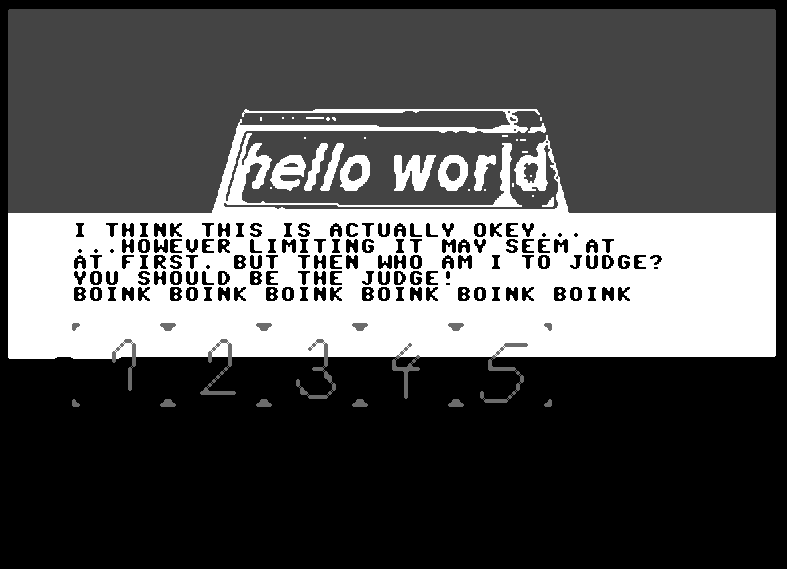
|
99
|
+
|
100
|
+
And it moves and stuff (no sound though), you can grab the .prg [here](https://raw.github.com/drbig/c64asm/master/examples/04.prg) and see the whole thing. The big ugly numbers are sprites - I assume the point was to make them move and be interesting, oh well.
|
101
|
+
|
102
|
+
Also my raster bar code is flawed.
|
103
|
+
|
104
|
+
## Additional notes
|
105
|
+
|
106
|
+
First compiler. Also I believe that'd be my second/third non-trivial Ruby project. I'm also by no means a C64 hacker, but I appreciate the platform very much. It was fun, even this get-this-public-now work.
|
107
|
+
|
108
|
+
This project has been inspired by a blog post of person that did a NES assembler in Common Lisp (kudos to the author!).
|
109
|
+
|
110
|
+
## Contributing
|
111
|
+
|
112
|
+
Follow the usual GitHub development model:
|
113
|
+
|
114
|
+
1. Clone the repository
|
115
|
+
2. Make your changes on a separate branch
|
116
|
+
4. Make a pull request
|
117
|
+
|
118
|
+
See licensing for legalese.
|
119
|
+
|
120
|
+
## Licensing
|
121
|
+
|
122
|
+
Standard two-clause BSD license, see LICENSE.txt for details.
|
123
|
+
|
124
|
+
Any contributions will be licensed under the same conditions.
|
125
|
+
|
126
|
+
Copyright (c) 2012 - 2014 Piotr S. Staszewski
|
data/Rakefile
ADDED
data/c64asm.gemspec
ADDED
@@ -0,0 +1,19 @@
|
|
1
|
+
require File.expand_path('../lib/c64asm/version', __FILE__)
|
2
|
+
|
3
|
+
Gem::Specification.new do |s|
|
4
|
+
s.name = 'c64asm'
|
5
|
+
s.version = C64Asm::VERSION
|
6
|
+
s.date = Time.now
|
7
|
+
|
8
|
+
s.summary = %q{Data-driven verbose DSL (almost dis-)assembler for MOS6502 with focus on Commoder 64}
|
9
|
+
s.description = %q{Already two-year-old project inspirated by the NES assembler written in Lisp. The assembly DSL lets you easily unroll loops, create screen maps on the fly and just hack the thing. As you write a plain .prg file you may also dump the code to a very verbose format to ponder the machine code.}
|
10
|
+
s.license = 'BSD'
|
11
|
+
s.authors = ['Piotr S. Staszewski']
|
12
|
+
s.email = 'p.staszewski@gmail.com'
|
13
|
+
s.homepage = 'https://github.com/drbig/c64asm'
|
14
|
+
|
15
|
+
s.files = `git ls-files`.split("\n")
|
16
|
+
s.require_paths = ['lib']
|
17
|
+
|
18
|
+
s.required_ruby_version = '>= 1.8.7' # rather tentative
|
19
|
+
end
|
data/examples/04.png
ADDED
Binary file
|
data/examples/04.prg
ADDED
Binary file
|
Binary file
|
@@ -0,0 +1,30 @@
|
|
1
|
+
#!/usr/bin/env ruby
|
2
|
+
# encoding: utf-8
|
3
|
+
# See LICENSE.txt for licensing information.
|
4
|
+
|
5
|
+
$LOAD_PATH.unshift(File.dirname(__FILE__) + '/../lib')
|
6
|
+
require 'c64asm'
|
7
|
+
|
8
|
+
hello_world = C64Asm::Macro.new do
|
9
|
+
block C64Asm::Basic.new('10 sys 49153').code
|
10
|
+
|
11
|
+
align 0xc000 # 49153
|
12
|
+
jsr 0xe544 # clear
|
13
|
+
ldx.d 0 # string index
|
14
|
+
label :load
|
15
|
+
lda.ax :msg # load character
|
16
|
+
cmp.d 35 # hash is the end of line
|
17
|
+
beq :finish # if so we're done
|
18
|
+
jsr 0xffd2 # chrout
|
19
|
+
inx # increment index
|
20
|
+
jmp :load # if not load the next character
|
21
|
+
label :finish
|
22
|
+
rts # back to basic(s)
|
23
|
+
label :msg
|
24
|
+
data "HELLO WORLD#"
|
25
|
+
end
|
26
|
+
|
27
|
+
code = hello_world.call # compile the macro
|
28
|
+
puts code.dump # print detailed
|
29
|
+
#puts code.to_source # just the source code
|
30
|
+
code.write!('hello_world.prg') # save to a standard .prg file
|