black_hole_struct 0.1.1 → 0.1.2
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +41 -2
- data/lib/black_hole_struct.rb +41 -5
- data/test/black_hole_struct_test.rb +34 -2
- metadata +2 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 0e6b867238881473e5302a2d846b0f761ede511e
|
4
|
+
data.tar.gz: b28833bb76c5b7fe5e51a21a94f515cba7eb99e9
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 30c0f323a16358dedfbae9eb464a86db214bf39ae7664e89b64f15f5805ff8e163746c68f9830ec764f4ae79564f98eb0440486740939ebba3d4e0a8f425d12b
|
7
|
+
data.tar.gz: 5ce7b1f60843969f3af2842f66c49162e92e3fad1b8201426ac1f8cd9b71eb0dd23d3cf504ebced1da41e2ad01e8868a4aa96b01c5c8f72f4440fc9f647d12dc
|
data/README.md
CHANGED
@@ -1,7 +1,12 @@
|
|
1
|
+
[](https://circleci.com/gh/mickey/black-hole-struct/tree/master)
|
2
|
+
|
1
3
|
# BlackHoleStruct
|
2
4
|
|
3
|
-
**BlackHoleStruct** is a data structure similar to an `OpenStruct
|
4
|
-
infinite chaining of attributes or [autovivification](https://en.wikipedia.org/wiki/Autovivification)
|
5
|
+
**BlackHoleStruct** is a data structure similar to an `OpenStruct` that allows:
|
6
|
+
- infinite chaining of attributes or [autovivification](https://en.wikipedia.org/wiki/Autovivification)
|
7
|
+
- deep merging of BlackHoleStruct/Hash
|
8
|
+
|
9
|
+
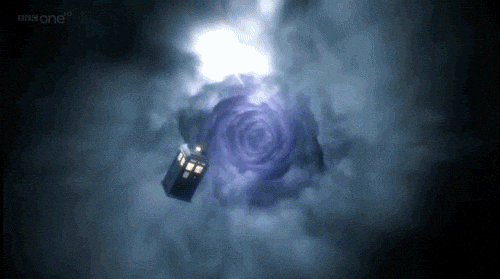
|
5
10
|
|
6
11
|
## Installation
|
7
12
|
|
@@ -30,8 +35,42 @@ config.dashboard.time.to = "now"
|
|
30
35
|
puts config.dashboard.theme # "white"
|
31
36
|
puts config.dashboard.time # #<BlackHoleStruct :from="now-1h" :to="now">
|
32
37
|
puts config.dashboard.time.from # "now-1h"
|
38
|
+
|
39
|
+
config[:connection][:host] = "localhost"
|
40
|
+
config[:connection][:port] = 3000
|
41
|
+
|
42
|
+
puts config.to_h
|
43
|
+
# {
|
44
|
+
# connection: {
|
45
|
+
# host: "localhost",
|
46
|
+
# port: 3000
|
47
|
+
# }
|
48
|
+
# dashboard: {
|
49
|
+
# theme: "white",
|
50
|
+
# time: {
|
51
|
+
# from: "now-1h",
|
52
|
+
# to: "now"
|
53
|
+
# }
|
54
|
+
# }
|
55
|
+
# }
|
56
|
+
|
57
|
+
config = BlackHoleStruct.new(theme: "white", connection: {port: 3000})
|
58
|
+
config.deep_merge!(connection: {host: 'localhost'})
|
59
|
+
puts config.to_h
|
60
|
+
# {
|
61
|
+
# connection: {
|
62
|
+
# host: "localhost",
|
63
|
+
# port: 3000
|
64
|
+
# }
|
65
|
+
# theme: "white"
|
66
|
+
# }
|
67
|
+
|
33
68
|
```
|
34
69
|
|
70
|
+
## Is it any good
|
71
|
+
|
72
|
+
[Yes](https://news.ycombinator.com/item?id=3067434)
|
73
|
+
|
35
74
|
## Advanced usage
|
36
75
|
|
37
76
|
Check the [documentation](http://www.rubydoc.info/github/mickey/black-hole-struct/master/BlackHoleStruct).
|
data/lib/black_hole_struct.rb
CHANGED
@@ -2,7 +2,7 @@
|
|
2
2
|
# infinite chaining of attributes or [autovivification](https://en.wikipedia.org/wiki/Autovivification).
|
3
3
|
class BlackHoleStruct
|
4
4
|
# Current version
|
5
|
-
VERSION = "0.1.
|
5
|
+
VERSION = "0.1.2"
|
6
6
|
|
7
7
|
# BlackHoleStruct can be optionally initialized with a Hash
|
8
8
|
# @param [Hash] hash Initialize with a hash
|
@@ -56,18 +56,49 @@ class BlackHoleStruct
|
|
56
56
|
@table.each_pair
|
57
57
|
end
|
58
58
|
|
59
|
-
#
|
60
|
-
#
|
61
|
-
#
|
62
|
-
|
59
|
+
# Returns a new hash with self and other_hash merged.
|
60
|
+
# @param [Hash] other_hash
|
61
|
+
# @return the final hash
|
62
|
+
def merge(other_hash)
|
63
|
+
self.dup.merge!(other_hash)
|
64
|
+
end
|
65
|
+
|
66
|
+
# Same as merge, but modifies self.
|
63
67
|
# @param [Hash] other_hash
|
64
68
|
# @return the final hash
|
65
69
|
def merge!(other_hash)
|
66
70
|
# no deep merge
|
67
71
|
@table = self.to_h.merge!(other_hash)
|
72
|
+
self
|
68
73
|
end
|
69
74
|
alias :update :merge!
|
70
75
|
|
76
|
+
# Returns a new hash with self and other_hash merged recursively.
|
77
|
+
# It only merges Hash recursively.
|
78
|
+
# @param [Hash] other_hash
|
79
|
+
# @return the final hash
|
80
|
+
def deep_merge(other_hash)
|
81
|
+
self.dup.deep_merge!(other_hash)
|
82
|
+
end
|
83
|
+
|
84
|
+
# Same as deep_merge, but modifies self.
|
85
|
+
# @param [Hash] other_hash
|
86
|
+
# @return the final hash
|
87
|
+
def deep_merge!(other_hash)
|
88
|
+
other_hash.each_pair do |current_key, other_value|
|
89
|
+
this_value = @table[current_key.to_sym]
|
90
|
+
|
91
|
+
if (this_value.is_a?(Hash) || this_value.is_a?(self.class)) &&
|
92
|
+
(other_value.is_a?(Hash) || other_value.is_a?(self.class))
|
93
|
+
@table[current_key.to_sym] = this_value.deep_merge(other_value)
|
94
|
+
else
|
95
|
+
@table[current_key.to_sym] = other_value
|
96
|
+
end
|
97
|
+
end
|
98
|
+
|
99
|
+
self
|
100
|
+
end
|
101
|
+
|
71
102
|
# Converts self to the hash
|
72
103
|
# @return [Hash]
|
73
104
|
def to_h
|
@@ -89,6 +120,11 @@ class BlackHoleStruct
|
|
89
120
|
|
90
121
|
private
|
91
122
|
|
123
|
+
def initialize_copy(other)
|
124
|
+
super
|
125
|
+
@table = @table.clone
|
126
|
+
end
|
127
|
+
|
92
128
|
def method_missing(name, *args)
|
93
129
|
if @table[name.to_sym]
|
94
130
|
@table[name.to_sym]
|
@@ -89,12 +89,44 @@ class BlackHoleStructTest < Minitest::Test
|
|
89
89
|
}
|
90
90
|
end
|
91
91
|
|
92
|
-
def test_merge
|
92
|
+
def test_merge!
|
93
93
|
@subject.theme = "white"
|
94
94
|
@subject.merge!(size: 5)
|
95
95
|
|
96
|
+
assert_equal @subject.to_h, { theme: "white", size: 5 }
|
97
|
+
end
|
98
|
+
|
99
|
+
def test_merge
|
100
|
+
@subject.theme = "white"
|
101
|
+
merged = @subject.merge(size: 5)
|
102
|
+
|
103
|
+
assert_equal merged.to_h, { theme: "white", size: 5 }
|
104
|
+
assert_equal @subject.to_h, { theme: "white" }
|
105
|
+
end
|
106
|
+
|
107
|
+
def test_deep_merge!
|
108
|
+
@subject.theme = "white"
|
109
|
+
@subject.connection.port = 3000
|
110
|
+
@subject.deep_merge!(connection: { host: '127.0.0.1' })
|
111
|
+
|
112
|
+
assert_equal @subject.to_h, {
|
113
|
+
theme: "white",
|
114
|
+
connection: { port: 3000, host: '127.0.0.1' }
|
115
|
+
}
|
116
|
+
end
|
117
|
+
|
118
|
+
def test_deep_merge
|
119
|
+
@subject.theme = "white"
|
120
|
+
@subject.connection.port = 3000
|
121
|
+
merged = @subject.deep_merge(connection: { host: '127.0.0.1' })
|
122
|
+
|
123
|
+
assert_equal merged.to_h, {
|
124
|
+
theme: "white",
|
125
|
+
connection: { port: 3000, host: '127.0.0.1' }
|
126
|
+
}
|
96
127
|
assert_equal @subject.to_h, {
|
97
|
-
theme: "white",
|
128
|
+
theme: "white",
|
129
|
+
connection: { port: 3000 }
|
98
130
|
}
|
99
131
|
end
|
100
132
|
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: black_hole_struct
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.1.
|
4
|
+
version: 0.1.2
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Michael Bensoussan
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2017-01-
|
11
|
+
date: 2017-01-03 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: minitest
|