author_engine 0.7.0 → 0.8.0
This diff represents the content of publicly available package versions that have been released to one of the supported registries. The information contained in this diff is provided for informational purposes only and reflects changes between package versions as they appear in their respective public registries.
- checksums.yaml +4 -4
- data/README.md +1 -1
- data/assets/ui/cursor.png +0 -0
- data/lib/author_engine/game/opal/exporter.rb +41 -13
- data/lib/author_engine/game/opal/game_runner.rb +70 -13
- data/lib/author_engine/game/opal/touch_button.rb +4 -13
- data/lib/author_engine/game/opal/touch_joystick.rb +7 -11
- data/lib/author_engine/version.rb +1 -1
- data/lib/author_engine/window.rb +3 -4
- data/screenshots/level_editor.png +0 -0
- metadata +4 -2
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA256:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: bd123dfe082801721b5f3a559180fb97ce3fea894f63e0f0d6c7694eb0cc2d5f
|
4
|
+
data.tar.gz: 945932ed00bb9287b2bb50e6f7135a35583e1a8d50624b1ea01632d7b76dcf62
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 9bde94c152e1f5722d047b1488a64e1126d58028dee38af826bbce70f2ce1153b31d71e85a5b2b9ae8fe2dfe6109bff92bd4685f4ef1145386de349cbce8951e
|
7
|
+
data.tar.gz: 3b6fa209262f49671634e9fcb0b2fea2556f1a893dd23950e9db634e8173f7c14f6aa8a018bd20acbe9323f72504a1d2348e3e48febcfc09b71a6ad0199fe361
|
data/README.md
CHANGED
@@ -22,7 +22,7 @@ A virtual console¹ that you code in Ruby.
|
|
22
22
|
## Sprite Editor
|
23
23
|

|
24
24
|
## Level Editor
|
25
|
-

|
26
26
|
## Code Editor
|
27
27
|
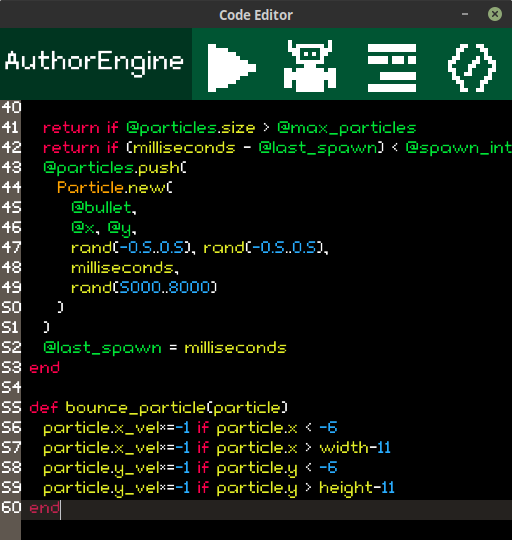
|
28
28
|
|
Binary file
|
@@ -1,5 +1,6 @@
|
|
1
1
|
require "opal"
|
2
2
|
require "fileutils"
|
3
|
+
require_relative "../../version"
|
3
4
|
|
4
5
|
class AuthorEngine
|
5
6
|
class OpalExporter
|
@@ -14,7 +15,7 @@ class AuthorEngine
|
|
14
15
|
return name.split("_").map {|n| n.capitalize}.join(" ")
|
15
16
|
end
|
16
17
|
|
17
|
-
# Rebuild opal runtime
|
18
|
+
# Rebuild opal runtime if it doesn't exist or if its out of date
|
18
19
|
def build_opal?
|
19
20
|
opal_runtime = "#{export_directory}/js/runtime.js"
|
20
21
|
|
@@ -29,6 +30,21 @@ class AuthorEngine
|
|
29
30
|
end
|
30
31
|
end
|
31
32
|
|
33
|
+
# Rebuild author_engine runtime if it doesn't exist or if its out of date
|
34
|
+
def build_authorengine?
|
35
|
+
authorengine_runtime = "#{export_directory}/js/author_engine.js"
|
36
|
+
|
37
|
+
if File.exists?(authorengine_runtime)
|
38
|
+
file = File.open(authorengine_runtime)
|
39
|
+
version = file.first.gsub("/", "").strip
|
40
|
+
file.close
|
41
|
+
|
42
|
+
AuthorEngine::VERSION != version
|
43
|
+
else
|
44
|
+
true
|
45
|
+
end
|
46
|
+
end
|
47
|
+
|
32
48
|
def stylesheet
|
33
49
|
%{
|
34
50
|
@font-face { font-family: Connection; src: url('fonts/Connection.otf'); }
|
@@ -42,7 +58,7 @@ body {
|
|
42
58
|
#canvas {
|
43
59
|
display: block;
|
44
60
|
margin: 0 auto;
|
45
|
-
|
61
|
+
cursor: none;
|
46
62
|
}
|
47
63
|
#loading {
|
48
64
|
font-family: Connection, sans-serif;
|
@@ -71,8 +87,6 @@ var projectString = `#{file}`;
|
|
71
87
|
|
72
88
|
def game_runtime
|
73
89
|
program = %{
|
74
|
-
# require "opal"
|
75
|
-
# require "opal-parser"
|
76
90
|
require "author_engine/opal"
|
77
91
|
|
78
92
|
`var callback = function(){
|
@@ -99,22 +113,31 @@ if (
|
|
99
113
|
opal_builder.build("opal")
|
100
114
|
opal_builder.build("opal-parser")
|
101
115
|
else
|
102
|
-
puts " Skipping Opal runtime. Already exists
|
116
|
+
puts " Skipping Opal runtime. Already exists and up to date (v#{Opal::VERSION})..."
|
103
117
|
end
|
104
118
|
|
105
|
-
|
106
|
-
|
107
|
-
|
108
|
-
game_builder.append_paths("#{base_path}")
|
119
|
+
author_engine_builder = nil
|
120
|
+
if build_authorengine?
|
121
|
+
puts " Building AuthorEngine runtime..."
|
109
122
|
|
110
|
-
|
123
|
+
author_engine_builder = Opal::Builder.new
|
124
|
+
base_path = File.expand_path("../../../..", __FILE__)
|
125
|
+
author_engine_builder.append_paths("#{base_path}")
|
126
|
+
|
127
|
+
author_engine_builder.build_require("author_engine/opal")
|
128
|
+
else
|
129
|
+
puts " Skipping AuthorEngine runtime. Already exists and up to date (v#{AuthorEngine::VERSION})..."
|
130
|
+
end
|
111
131
|
|
112
132
|
opal_builder_js = nil
|
113
133
|
if opal_builder
|
114
134
|
opal_runtime_js = opal_builder.build_str("", "(inline)").to_s
|
115
135
|
end
|
116
136
|
|
117
|
-
author_engine_js =
|
137
|
+
author_engine_js = nil
|
138
|
+
if author_engine_builder
|
139
|
+
author_engine_js = author_engine_builder.build_str(program, "(inline)").to_s
|
140
|
+
end
|
118
141
|
|
119
142
|
return {opal_runtime: opal_runtime_js, author_engine_runtime: author_engine_js}
|
120
143
|
end
|
@@ -124,6 +147,7 @@ if (
|
|
124
147
|
<!doctype html5>
|
125
148
|
<html>
|
126
149
|
<head>
|
150
|
+
<meta content="width=device-width, initial-scale=1" name="viewport" />
|
127
151
|
<meta charset="utf-8" />
|
128
152
|
<title>#{project_name} | AuthorEngine</title>
|
129
153
|
</head>
|
@@ -201,12 +225,16 @@ if (
|
|
201
225
|
end
|
202
226
|
end
|
203
227
|
|
228
|
+
puts " Building game..."
|
204
229
|
File.open("#{export_path}/game.js", "w") do |file|
|
205
230
|
file.write(project)
|
206
231
|
end
|
207
232
|
|
208
|
-
|
209
|
-
|
233
|
+
if hash[:author_engine_runtime]
|
234
|
+
File.open("#{export_path}/js/author_engine.js", "w") do |file|
|
235
|
+
file.write("// #{AuthorEngine::VERSION}\n")
|
236
|
+
file.write(hash[:author_engine_runtime])
|
237
|
+
end
|
210
238
|
end
|
211
239
|
|
212
240
|
fonts_path = "#{File.expand_path("../../../../../", __FILE__)}/assets/fonts"
|
@@ -34,7 +34,6 @@ class AuthorEngine
|
|
34
34
|
|
35
35
|
@game = Game.new(code: @save_file.code)
|
36
36
|
build_spritesheet_and_sprites_list
|
37
|
-
resize_canvas
|
38
37
|
|
39
38
|
@collision_detection = AuthorEngine::CollisionDetection.new(@sprites, @levels, @save_file.sprites)
|
40
39
|
@game.authorengine_collision_detection = @collision_detection
|
@@ -42,19 +41,20 @@ class AuthorEngine
|
|
42
41
|
@game.init
|
43
42
|
|
44
43
|
@show_touch_controls = false
|
45
|
-
@touch_joystick = TouchJoystick.new(
|
44
|
+
@touch_joystick = TouchJoystick.new(radius: 50)
|
46
45
|
@touch_buttons = []
|
47
46
|
@touch_buttons.push(
|
48
47
|
TouchButton.new(
|
49
|
-
label: "X", color: @game.red,
|
48
|
+
label: "X", color: @game.red, width: 50, height: 50, for_key: "x"
|
50
49
|
),
|
51
50
|
TouchButton.new(
|
52
|
-
label: "Y", color: @game.yellow,
|
51
|
+
label: "Y", color: @game.yellow, width: 50, height: 50, for_key: "y"
|
53
52
|
)
|
54
53
|
)
|
55
54
|
|
56
|
-
@fullscreen_button = TouchButton.new(label: "Fullscreen", color: @game.black,
|
55
|
+
@fullscreen_button = TouchButton.new(label: "Fullscreen", color: @game.black, width: 100, height: 50)
|
57
56
|
touch_handler_setup
|
57
|
+
resize_canvas
|
58
58
|
|
59
59
|
return self
|
60
60
|
end
|
@@ -72,9 +72,14 @@ class AuthorEngine
|
|
72
72
|
|
73
73
|
def run_game
|
74
74
|
`window.requestAnimationFrame(function() {#{run_game}})` # placed here to ensure next frame is called even if draw or update throw an error
|
75
|
-
|
76
|
-
|
77
|
-
|
75
|
+
width = `window.innerWidth`
|
76
|
+
height = `window.innerHeight`
|
77
|
+
game_width = 128 * @game.authorengine_scale
|
78
|
+
game_height = 128 * @game.authorengine_scale
|
79
|
+
|
80
|
+
area_width = (`window.innerWidth` - game_width) / 2
|
81
|
+
|
82
|
+
`#{@game.authorengine_canvas_context}.clearRect(#{area_width},0, #{game_width}, #{game_height})`
|
78
83
|
|
79
84
|
@counted_frames+=1
|
80
85
|
|
@@ -86,11 +91,9 @@ class AuthorEngine
|
|
86
91
|
|
87
92
|
|
88
93
|
if @sprites.size == (@spritesheet_width/@sprite_size)*(@spritesheet_height/@sprite_size)
|
89
|
-
width = 128 * @game.authorengine_scale
|
90
|
-
|
91
94
|
# `#{@game.authorengine_canvas_context}.setTransform(1, 0, 0, 1, 0, 0)`
|
92
95
|
`#{@game.authorengine_canvas_context}.save()`
|
93
|
-
`#{@game.authorengine_canvas_context}.translate(window.innerWidth/2 - #{
|
96
|
+
`#{@game.authorengine_canvas_context}.translate(window.innerWidth/2 - #{game_height/2}, 0)`
|
94
97
|
`#{@game.authorengine_canvas_context}.scale(#{@game.authorengine_scale}, #{@game.authorengine_scale})`
|
95
98
|
`#{@game.authorengine_canvas_context}.save()`
|
96
99
|
|
@@ -130,6 +133,59 @@ class AuthorEngine
|
|
130
133
|
end
|
131
134
|
|
132
135
|
def reposition_touch_controls
|
136
|
+
return nil unless @touch_joystick
|
137
|
+
|
138
|
+
width = `window.innerWidth`
|
139
|
+
height = `window.innerHeight`
|
140
|
+
game_width = 128 * @game.authorengine_scale
|
141
|
+
game_height = 128 * @game.authorengine_scale
|
142
|
+
|
143
|
+
# place controls under game
|
144
|
+
if width < height
|
145
|
+
area_width = width
|
146
|
+
area_height = height - game_height
|
147
|
+
|
148
|
+
puts "space: width #{area_width} x height #{area_height}"
|
149
|
+
|
150
|
+
@touch_joystick.x = @touch_joystick.radius + @touch_joystick.radius
|
151
|
+
@touch_joystick.y = game_height + area_height / 2
|
152
|
+
|
153
|
+
padding = 10
|
154
|
+
last_x = 20
|
155
|
+
@touch_buttons.reverse.each do |button|
|
156
|
+
button.x = width - (last_x + button.width)
|
157
|
+
button.y = (height - area_height) + area_height / 2 - button.height / 2
|
158
|
+
|
159
|
+
last_x += button.width + padding
|
160
|
+
end
|
161
|
+
|
162
|
+
@fullscreen_button.x = width - (width / 2 + @fullscreen_button.width / 2)
|
163
|
+
@fullscreen_button.y = height - @fullscreen_button.height
|
164
|
+
|
165
|
+
# place controls beside game
|
166
|
+
else
|
167
|
+
area_width = (`window.innerWidth` - game_width) / 2
|
168
|
+
area_height = game_height
|
169
|
+
|
170
|
+
puts "space: width #{area_width} x height #{area_height}"
|
171
|
+
|
172
|
+
@touch_joystick.x = @touch_joystick.radius + @touch_joystick.radius
|
173
|
+
@touch_joystick.y = game_height / 2
|
174
|
+
|
175
|
+
padding = 10
|
176
|
+
last_x = 50
|
177
|
+
@touch_buttons.reverse.each do |button|
|
178
|
+
button.x = width - (last_x + button.width)
|
179
|
+
button.y = game_height / 2 - button.height / 2
|
180
|
+
|
181
|
+
last_x += button.width + padding
|
182
|
+
end
|
183
|
+
|
184
|
+
@fullscreen_button.x = width - @fullscreen_button.width
|
185
|
+
@fullscreen_button.y = 0
|
186
|
+
end
|
187
|
+
|
188
|
+
return nil
|
133
189
|
end
|
134
190
|
|
135
191
|
def resize_canvas
|
@@ -137,9 +193,9 @@ class AuthorEngine
|
|
137
193
|
height = `window.innerHeight`
|
138
194
|
|
139
195
|
if width < height
|
140
|
-
@game.authorengine_scale =
|
196
|
+
@game.authorengine_scale = `#{width} / 128.0`
|
141
197
|
else
|
142
|
-
@game.authorengine_scale =
|
198
|
+
@game.authorengine_scale = `#{height} / 128.0`
|
143
199
|
end
|
144
200
|
|
145
201
|
`#{@game.authorengine_canvas}.width = #{width}`
|
@@ -150,6 +206,7 @@ class AuthorEngine
|
|
150
206
|
`#{@game.authorengine_canvas_context}.imageSmoothingEnabled = false`
|
151
207
|
|
152
208
|
reposition_touch_controls
|
209
|
+
`#{@game.authorengine_canvas_context}.clearRect(0, 0, window.innerWidth, window.innerHeight)`
|
153
210
|
return nil
|
154
211
|
end
|
155
212
|
|
@@ -1,9 +1,10 @@
|
|
1
1
|
class AuthorEngine
|
2
2
|
class TouchButton
|
3
|
-
|
4
|
-
|
3
|
+
attr_accessor :x, :y
|
4
|
+
attr_reader :width, :height
|
5
|
+
def initialize(label:, color:, x: 0, y: 0, width:, height:, for_key: nil, &block)
|
5
6
|
@label, @color, @x, @y, @width, @height = label, color, x, y, width, height
|
6
|
-
@
|
7
|
+
@for_key = for_key
|
7
8
|
@block = block
|
8
9
|
|
9
10
|
@buttons = AuthorEngine::Part::OpalInput::BUTTONS
|
@@ -12,16 +13,6 @@ class AuthorEngine
|
|
12
13
|
@game = AuthorEngine::GameRunner.instance.game
|
13
14
|
@game_width = 128 * @game.authorengine_scale
|
14
15
|
@game_x = `window.innerWidth/2 - #{@game_width/2}`
|
15
|
-
|
16
|
-
if @side == :left
|
17
|
-
@x = @game_x-@x
|
18
|
-
elsif @side == :right
|
19
|
-
@x = @game_x+@game_width+@x
|
20
|
-
else
|
21
|
-
raise "side must be :left or :right"
|
22
|
-
end
|
23
|
-
|
24
|
-
@y = `window.innerHeight/2 - #{height/2}` unless @y.is_a?(Numeric)
|
25
16
|
end
|
26
17
|
|
27
18
|
def draw
|
@@ -1,7 +1,8 @@
|
|
1
1
|
class AuthorEngine
|
2
2
|
class TouchJoystick
|
3
|
-
|
4
|
-
|
3
|
+
attr_accessor :x, :y, :radius
|
4
|
+
def initialize(x: 0, y: 0, radius:, background: nil, color: nil)
|
5
|
+
@x, @y, @radius, @background, @color = x, y, radius, background, color
|
5
6
|
|
6
7
|
@buttons = AuthorEngine::Part::OpalInput::BUTTONS
|
7
8
|
@key_states = AuthorEngine::Part::OpalInput::KEY_STATES
|
@@ -10,15 +11,6 @@ class AuthorEngine
|
|
10
11
|
@game_width = 128 * @game.authorengine_scale
|
11
12
|
@game_x = `window.innerWidth/2 - #{@game_width/2}`
|
12
13
|
|
13
|
-
if @side == :left
|
14
|
-
@x = @game_x-@x
|
15
|
-
elsif @side == :right
|
16
|
-
@x = @game_x+@game_width+@x
|
17
|
-
else
|
18
|
-
raise "side must be :left or :right"
|
19
|
-
end
|
20
|
-
|
21
|
-
@y = `window.innerHeight/2` unless @y.is_a?(Numeric)
|
22
14
|
@color = @game.dark_gray unless @color
|
23
15
|
@background = @game.light_gray unless @background
|
24
16
|
|
@@ -26,6 +18,10 @@ class AuthorEngine
|
|
26
18
|
end
|
27
19
|
|
28
20
|
def draw
|
21
|
+
#Clear
|
22
|
+
combo = @radius + @joystick_radius
|
23
|
+
`#{@game.authorengine_canvas_context}.clearRect(#{@x - combo}, #{@y - combo}, #{combo + combo}, #{combo + combo})`
|
24
|
+
|
29
25
|
# Background
|
30
26
|
`#{@game.authorengine_canvas_context}.fillStyle = #{@background}`
|
31
27
|
`#{@game.authorengine_canvas_context}.beginPath()`
|
data/lib/author_engine/window.rb
CHANGED
@@ -30,6 +30,8 @@ class AuthorEngine
|
|
30
30
|
|
31
31
|
@close_counter = 0
|
32
32
|
|
33
|
+
@cursor = AuthorEngine::Image.new("assets/ui/cursor.png", retro: true)
|
34
|
+
|
33
35
|
calculate_scale
|
34
36
|
setup
|
35
37
|
end
|
@@ -56,16 +58,13 @@ class AuthorEngine
|
|
56
58
|
|
57
59
|
def draw
|
58
60
|
@container.draw
|
61
|
+
@cursor.draw(mouse_x, mouse_y, Float::INFINITY, @square_scale, @square_scale) if @show_cursor
|
59
62
|
end
|
60
63
|
|
61
64
|
def update
|
62
65
|
@container.update
|
63
66
|
end
|
64
67
|
|
65
|
-
def needs_cursor?
|
66
|
-
@show_cursor
|
67
|
-
end
|
68
|
-
|
69
68
|
def lighten(color, amount = 25)
|
70
69
|
if defined?(color.alpha)
|
71
70
|
return Gosu::Color.rgba(color.red+amount, color.green+amount, color.blue+amount, color.alpha)
|
Binary file
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: author_engine
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version: 0.
|
4
|
+
version: 0.8.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- Cyberarm
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2019-11-
|
11
|
+
date: 2019-11-05 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: gosu
|
@@ -117,6 +117,7 @@ files:
|
|
117
117
|
- assets/fonts/SIL Open Font License.txt
|
118
118
|
- assets/ui/bucket_icon.png
|
119
119
|
- assets/ui/code_icon.png
|
120
|
+
- assets/ui/cursor.png
|
120
121
|
- assets/ui/error_icon.png
|
121
122
|
- assets/ui/level_icon.png
|
122
123
|
- assets/ui/loading_icon.png
|
@@ -166,6 +167,7 @@ files:
|
|
166
167
|
- lib/author_engine/views/sprite_editor.rb
|
167
168
|
- lib/author_engine/window.rb
|
168
169
|
- screenshots/code_editor.png
|
170
|
+
- screenshots/level_editor.png
|
169
171
|
- screenshots/play.png
|
170
172
|
- screenshots/sprite_editor.png
|
171
173
|
homepage: https://github.com/cyberarm/author_engineV2
|