action_cable_client 1.0 → 1.1.0
Sign up to get free protection for your applications and to get access to all the features.
- checksums.yaml +4 -4
- data/CHANGELOG.md +1 -0
- data/README.md +21 -0
- data/lib/action_cable_client.rb +28 -11
- data/lib/action_cable_client/message.rb +4 -5
- data/lib/action_cable_client/version.rb +1 -1
- metadata +3 -3
checksums.yaml
CHANGED
@@ -1,7 +1,7 @@
|
|
1
1
|
---
|
2
2
|
SHA1:
|
3
|
-
metadata.gz:
|
4
|
-
data.tar.gz:
|
3
|
+
metadata.gz: 2061444f2909753d47a8f603b54d8be3009b298e
|
4
|
+
data.tar.gz: c52360502fc6582d1e46e7c2433a08fc3b6dd8da
|
5
5
|
SHA512:
|
6
|
-
metadata.gz:
|
7
|
-
data.tar.gz:
|
6
|
+
metadata.gz: 2244aa969d7c82bbb46e2f945ebe4499e556ffa70c43914579cb48333308b04e13ab92a2857df11d23c41bfac27978e6ccd0a2fe03f10f927f5456004cfc5d93
|
7
|
+
data.tar.gz: e79ce50f3b82c328b2af3d7076bdef524e8ceab8100638f3c8246000b2479043e768f7319cad71b8369bf3ef3e65952c6ca771d5837ebd04ea70d1f3121f8084
|
data/CHANGELOG.md
CHANGED
data/README.md
CHANGED
@@ -1,7 +1,10 @@
|
|
1
1
|
# Action Cable Client
|
2
|
+
[](https://badge.fury.io/rb/action_cable_client)
|
2
3
|
[](https://travis-ci.org/NullVoxPopuli/action_cable_client)
|
3
4
|
[](https://codeclimate.com/github/NullVoxPopuli/action_cable_client)
|
4
5
|
[](https://codeclimate.com/github/NullVoxPopuli/action_cable_client/coverage)
|
6
|
+
[](https://gemnasium.com/github.com/NullVoxPopuli/action_cable_client)
|
7
|
+
|
5
8
|
|
6
9
|
There are quite a few WebSocket Servers out there. The popular ones seem to be: [faye-websocket-ruby](https://github.com/faye/faye-websocket-ruby), [em-websockets](https://github.com/igrigorik/em-websocket). The client-only websocket gems are kinda hard to find, and are only raw websocket support. Rails has a thin layer on top of web sockets to help manage subscribing to channels and send/receive on channels.
|
7
10
|
|
@@ -34,8 +37,26 @@ end
|
|
34
37
|
|
35
38
|
This example is compatible with [this version of a small Rails app with Action Cable](https://github.com/NullVoxPopuli/mesh-relay/tree/2ed88928d91d82b88b7878fcb97e3bd81977cfe8)
|
36
39
|
|
40
|
+
|
41
|
+
|
37
42
|
The available hooks to tie in to are:
|
38
43
|
- `disconnected {}`
|
39
44
|
- `connected {}`
|
40
45
|
- `errored { |msg| }`
|
41
46
|
- `received { |msg }`
|
47
|
+
|
48
|
+
## Demo
|
49
|
+
|
50
|
+
[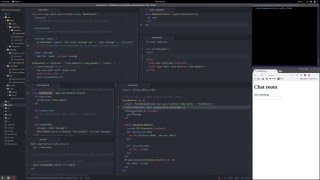](http://www.youtube.com/watch?v=x9D1wWsVHMY&hd=1)
|
51
|
+
|
52
|
+
Action Cable Client Demo on YouTube (1:41)
|
53
|
+
|
54
|
+
[Here is a set of files in a gist](https://gist.github.com/NullVoxPopuli/edfcbbe91a7877e445cbde84c7f05b37) that demonstrate how different `action_cable_client`s can communicate with eachother.
|
55
|
+
|
56
|
+
## Contributing
|
57
|
+
|
58
|
+
1. Fork it ( https://github.com/NullVoxPopuli/action_cable_client/fork )
|
59
|
+
2. Create your feature branch (`git checkout -b my-new-feature`)
|
60
|
+
3. Commit your changes (`git commit -am 'Add some feature'`)
|
61
|
+
4. Push to the branch (`git push origin my-new-feature`)
|
62
|
+
5. Create a new Pull Request
|
data/lib/action_cable_client.rb
CHANGED
@@ -17,13 +17,13 @@ class ActionCableClient
|
|
17
17
|
MESSAGE = 'message'
|
18
18
|
end
|
19
19
|
|
20
|
-
attr_reader :_websocket_client, :_uri, :_channel_name
|
20
|
+
attr_reader :_websocket_client, :_uri, :_channel_name, :_queued_send
|
21
21
|
attr_reader :_message_factory
|
22
22
|
# The queue should store entries in the format:
|
23
23
|
# [ action, data ]
|
24
24
|
attr_accessor :message_queue, :subscribed
|
25
25
|
|
26
|
-
|
26
|
+
alias subscribed? subscribed
|
27
27
|
|
28
28
|
def_delegator :_websocket_client, :disconnect, :disconnected
|
29
29
|
def_delegator :_websocket_client, :errback, :errored
|
@@ -33,9 +33,12 @@ class ActionCableClient
|
|
33
33
|
# @param [String] uri - e.g.: ws://domain:port
|
34
34
|
# @param [String] channel - the name of the channel on the Rails server
|
35
35
|
# e.g.: RoomChannel
|
36
|
-
|
36
|
+
# @param [Boolean] queued_send - optionally send messages after a ping
|
37
|
+
# is received, rather than instantly
|
38
|
+
def initialize(uri, channel = '', queued_send = false)
|
37
39
|
@_channel_name = channel
|
38
40
|
@_uri = uri
|
41
|
+
@_queued_send = queued_send
|
39
42
|
@message_queue = []
|
40
43
|
@subscribed = false
|
41
44
|
|
@@ -51,7 +54,11 @@ class ActionCableClient
|
|
51
54
|
# @param [String] action - how the message is being sent
|
52
55
|
# @param [Hash] data - the message to be sent to the channel
|
53
56
|
def perform(action, data)
|
54
|
-
|
57
|
+
if _queued_send
|
58
|
+
message_queue.push([action, data])
|
59
|
+
else
|
60
|
+
dispatch_message(action, data)
|
61
|
+
end
|
55
62
|
end
|
56
63
|
|
57
64
|
# callback for received messages as well as
|
@@ -67,7 +74,7 @@ class ActionCableClient
|
|
67
74
|
# puts message
|
68
75
|
# end
|
69
76
|
def received(skip_pings = true)
|
70
|
-
_websocket_client.stream do |
|
77
|
+
_websocket_client.stream do |message|
|
71
78
|
string = message.data
|
72
79
|
json = JSON.parse(string)
|
73
80
|
|
@@ -77,7 +84,8 @@ class ActionCableClient
|
|
77
84
|
else
|
78
85
|
yield(json)
|
79
86
|
end
|
80
|
-
|
87
|
+
|
88
|
+
deplete_queue if _queued_send
|
81
89
|
end
|
82
90
|
end
|
83
91
|
|
@@ -100,9 +108,7 @@ class ActionCableClient
|
|
100
108
|
# {"identifier" => "_ping","type" => "confirm_subscription"}
|
101
109
|
def check_for_subscribe_confirmation(message)
|
102
110
|
message_type = message[Message::TYPE_KEY]
|
103
|
-
if Message::TYPE_CONFIRM_SUBSCRIPTION == message_type
|
104
|
-
self.subscribed = true
|
105
|
-
end
|
111
|
+
self.subscribed = true if Message::TYPE_CONFIRM_SUBSCRIPTION == message_type
|
106
112
|
end
|
107
113
|
|
108
114
|
# {"identifier" => "_ping","message" => 1460201942}
|
@@ -118,8 +124,19 @@ class ActionCableClient
|
|
118
124
|
end
|
119
125
|
|
120
126
|
def deplete_queue
|
121
|
-
|
122
|
-
|
127
|
+
# if we haven't yet subscribed, don't deplete the queue
|
128
|
+
if subscribed?
|
129
|
+
# only try to send if we have messages to send
|
130
|
+
until message_queue.empty?
|
131
|
+
action, data = message_queue.pop
|
132
|
+
dispatch_message(action, data)
|
133
|
+
end
|
134
|
+
end
|
135
|
+
end
|
136
|
+
|
137
|
+
def dispatch_message(action, data)
|
138
|
+
# can't send messages if we aren't subscribed
|
139
|
+
if subscribed?
|
123
140
|
msg = _message_factory.create(Commands::MESSAGE, action, data)
|
124
141
|
send_msg(msg.to_json)
|
125
142
|
end
|
@@ -1,13 +1,12 @@
|
|
1
1
|
# frozen_string_literal: true
|
2
2
|
class ActionCableClient
|
3
3
|
class Message
|
4
|
-
IDENTIFIER_KEY = 'identifier'
|
5
|
-
IDENTIFIER_PING = '_ping'
|
4
|
+
IDENTIFIER_KEY = 'identifier'
|
5
|
+
IDENTIFIER_PING = '_ping'
|
6
6
|
# Type is never sent, but is received
|
7
7
|
# TODO: find a better place for this constant
|
8
|
-
TYPE_KEY = 'type'
|
9
|
-
TYPE_CONFIRM_SUBSCRIPTION = 'confirm_subscription'
|
10
|
-
|
8
|
+
TYPE_KEY = 'type'
|
9
|
+
TYPE_CONFIRM_SUBSCRIPTION = 'confirm_subscription'
|
11
10
|
|
12
11
|
attr_reader :_command, :_identifier, :_data
|
13
12
|
|
metadata
CHANGED
@@ -1,14 +1,14 @@
|
|
1
1
|
--- !ruby/object:Gem::Specification
|
2
2
|
name: action_cable_client
|
3
3
|
version: !ruby/object:Gem::Version
|
4
|
-
version:
|
4
|
+
version: 1.1.0
|
5
5
|
platform: ruby
|
6
6
|
authors:
|
7
7
|
- L. Preston Sego III
|
8
8
|
autorequire:
|
9
9
|
bindir: bin
|
10
10
|
cert_chain: []
|
11
|
-
date: 2016-04-
|
11
|
+
date: 2016-04-18 00:00:00.000000000 Z
|
12
12
|
dependencies:
|
13
13
|
- !ruby/object:Gem::Dependency
|
14
14
|
name: activesupport
|
@@ -129,5 +129,5 @@ rubyforge_project:
|
|
129
129
|
rubygems_version: 2.5.1
|
130
130
|
signing_key:
|
131
131
|
specification_version: 4
|
132
|
-
summary: ActionCableClient-1.0
|
132
|
+
summary: ActionCableClient-1.1.0
|
133
133
|
test_files: []
|